Top 250 Frontend Development Interview Questions and Answers
Updated 4 Jul 2025
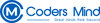
Asked in Coders Mind and 3 others

Q. What is CSS3?
CSS3 is the latest version of Cascading Style Sheets used for styling web pages.
CSS3 introduces new features like animations, transitions, and gradients.
It also includes new selectors like :nth-child() and :not().
CSS3 supports media queries for respo...read more
Asked in Encyclofoodia and 10 others

Q. Explain the concept of Virtual DOM.
Virtual DOM is a lightweight representation of the actual DOM, optimizing updates and rendering in web applications.
The Virtual DOM is a JavaScript object that mirrors the structure of the actual DOM.
When changes occur, a new Virtual DOM is created a...read more
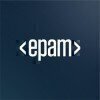
Asked in EPAM Systems

Q. How can we adapt the frontend to different device views?
Responsive design using media queries and flexible layouts to adapt frontend to different devices.
Use media queries in CSS to apply different styles based on screen size
Utilize responsive frameworks like Bootstrap or Foundation for pre-built responsi...read more
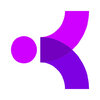

Q. Explain props and events in Vue.js.
Props are used to pass data from parent to child components, while events are used to communicate from child to parent components.
Props are read-only and cannot be modified by the child component
Events are emitted using $emit() method and can be list...read more
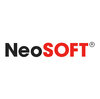
Asked in NeoSOFT

Q. What is the viewport?
The viewport is the visible area of a web page displayed on a device's screen.
It determines the layout and dimensions of the content on the screen.
It can be adjusted using meta tags in the HTML head section.
It is important for responsive design and m...read more
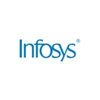
Asked in Infosys

Q. How do you handle drop-down menus in testing?
To handle drop-down, identify the element, select the option, and verify the selection.
Identify the drop-down element using locators like ID, class, or XPath.
Use Selenium's Select class to select the desired option.
Verify the selection using assertio...read more
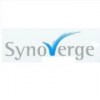
Asked in Synoverge Technologies

Q. Tell me about JSX.
JSX is a syntax extension for JavaScript used with React to describe what the UI should look like.
JSX stands for JavaScript XML
It allows developers to write HTML-like code in JavaScript
JSX elements are transpiled into regular JavaScript function call...read more
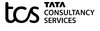
Asked in TCS

Q. Create a page that moves
Create a page that moves
Use CSS animations or transitions to create movement
Consider using JavaScript for more complex animations
Experiment with different types of movement such as sliding, fading, or rotating
Asked in Zomnor Worknet

Q. What is DOM in frontend development?
DOM stands for Document Object Model, a programming interface for web documents.
DOM is a tree-like structure that represents the HTML elements of a webpage.
It allows developers to manipulate the content, structure, and style of a webpage using script...read more
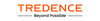
Asked in Tredence

Q. How do you change all element background colors to blue using jQuery?
Use jQuery to change the background color of all ul elements to blue.
Use the jQuery selector to select all ul elements
Use the css() method to change the background color to blue
Frontend Development Jobs
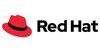
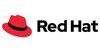
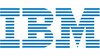
Asked in Lixil Window Systems

Q. What is window load
Window load refers to the time it takes for a webpage to fully load all its content.
It is a measure of the time it takes for a webpage to load completely
It includes all the resources on the page such as images, scripts, and stylesheets
A slow window l...read more
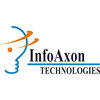
Asked in InfoAxon Technologies

Q. How do you achieve responsiveness in a web page?
Achieve responsiveness in web page by using media queries, flexible layouts, and fluid images.
Use CSS media queries to apply different styles based on screen size
Create flexible layouts using CSS Grid or Flexbox
Use relative units like percentages and...read more
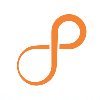
Asked in Persistent Systems

Q. Development about backend and front end
Backend development focuses on server-side logic and databases, while front end development focuses on user interface and client-side functionality.
Backend development involves writing server-side code using languages like Java, Python, or Node.js.
Fr...read more
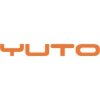
Asked in Yuto Printing and Packaging

Q. How do you create a dynamic page?
A dynamic page can be created by using HTML, CSS, and JavaScript to add interactive elements and update content dynamically.
Use HTML to structure the page and define the content.
Apply CSS to style the page and make it visually appealing.
Utilize JavaS...read more
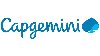
Asked in Capgemini

Q. What are Pure Components in React JS?
Pure Components in React JS are components that do not re-render unless their props have changed.
Pure Components extend React.Component and implement shouldComponentUpdate method for shallow comparison of props and state.
Pure Components are more effi...read more
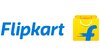
Asked in Flipkart

Q. How do you manage large datasets on the front end?
Large data on front end can be managed by implementing pagination, lazy loading, virtual scrolling, and data caching.
Implement pagination to display data in smaller chunks, reducing load time and improving performance.
Use lazy loading to only load da...read more
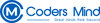
Asked in Coders Mind and 3 others

Q. What is CSS3?
CSS3 is the latest version of Cascading Style Sheets used for styling web pages.
CSS3 introduces new features like animations, transitions, and gradients.
It also includes new selectors like :nth-child() and :not().
CSS3 supports media queries for respo...read more
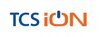
Asked in TCS iON

Q. What is minification?
Minification is the process of removing unnecessary characters from code without affecting its functionality.
Minification reduces file size by removing comments, whitespace, and renaming variables.
It helps improve website loading speed and performanc...read more
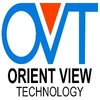
Asked in OVT India and 3 others

Q. What is reflow?
Reflow is a process of melting and re-solidifying solder paste to create a permanent bond between components and PCB.
Reflow is a critical step in surface mount technology (SMT) assembly.
It involves heating the PCB and components to a specific tempera...read more
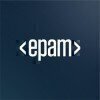
Asked in EPAM Systems

Q. How can we adapt the frontend to different device views?
Responsive design using media queries and flexible layouts to adapt frontend to different devices.
Use media queries in CSS to apply different styles based on screen size
Utilize responsive frameworks like Bootstrap or Foundation for pre-built responsi...read more
Asked in MaaxtreeM

Q. How do you retrieve the selected value from a dropdown list?
To get the selected drop down list value, use the getSelectedValue() method.
Use the getSelectedValue() method to get the selected value from the drop down list.
Identify the drop down list element using its ID or name.
Call the getSelectedValue() metho...read more
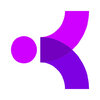

Q. Explain props and events in Vue.js.
Props are used to pass data from parent to child components, while events are used to communicate from child to parent components.
Props are read-only and cannot be modified by the child component
Events are emitted using $emit() method and can be list...read more
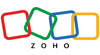
Asked in Zoho

Q. What is a critical rendering path?
The sequence of steps a browser takes to convert HTML, CSS, and JavaScript into a rendered page.
Includes parsing HTML, constructing the DOM tree, calculating styles, and executing JavaScript.
Optimizing the critical rendering path can improve page loa...read more
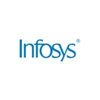
Asked in Infosys

Q. How do you handle drop-down menus in testing?
To handle drop-down, identify the element, select the option, and verify the selection.
Identify the drop-down element using locators like ID, class, or XPath.
Use Selenium's Select class to select the desired option.
Verify the selection using assertio...read more
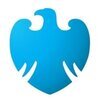
Asked in Barclays Global Service Centre

Q. Create an accordion component.
An accordion is a UI component that expands and collapses content sections when clicked.
Use HTML, CSS, and JavaScript to create the accordion
Each section should have a header and content that can be toggled
Utilize event listeners to handle the click ...read more
Asked in Huntington Bank

Q. How do you create a hyperlink in Razor?
To create a hyperlink in Razor, use the Html.ActionLink method.
Use @Html.ActionLink() method in Razor to create a hyperlink
Specify the link text, action method, controller name, and route values as parameters
Example: @Html.ActionLink("Click here", "I...read more
Asked in Athamas Technologies

Q. Create a simple drop-down menu.
Create a simple drop down menu using HTML and CSS
Use element to define the options in the menu
Style the drop down menu using CSS to customize its appearance
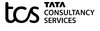
Asked in TCS

Q. Create a page that moves
Create a page that moves
Use CSS animations or transitions to create movement
Consider using JavaScript for more complex animations
Experiment with different types of movement such as sliding, fading, or rotating

Asked in Elucidata

Q. Describe how you would design a navigation bar for a website.
A clean and user-friendly navbar design for easy navigation on a website
Include important links such as Home, About Us, Services, Contact
Use clear and concise labels for each navigation item
Consider using dropdown menus for subcategories
Ensure the na...read more
Asked in Zomnor Worknet

Q. What is DOM in frontend development?
DOM stands for Document Object Model, a programming interface for web documents.
DOM is a tree-like structure that represents the HTML elements of a webpage.
It allows developers to manipulate the content, structure, and style of a webpage using script...read more
Top Interview Questions for Related Skills
Interview Experiences of Popular Companies
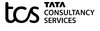
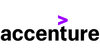
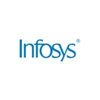
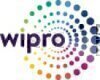
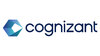
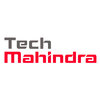
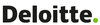
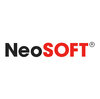
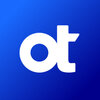
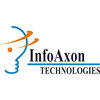
Interview Questions of Frontend Development Related Designations
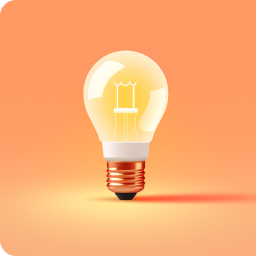
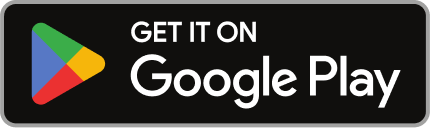
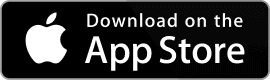
Reviews
Interviews
Salaries
Users
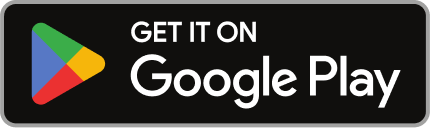
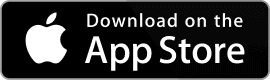