Top 250 Algorithms Interview Questions and Answers
Updated 12 Jul 2025
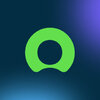
Asked in ServiceNow

Q. Set Matrix Zeros Problem Statement
Given an N x M
integer matrix, if an element is 0, set its entire row and column to 0's, and return the matrix. Specifically, if a cell has a value 0 (i.e., matrix[i][j] == 0
), change all cells i...read more
To solve the Set Matrix Zeros problem, we can use O(1) space by utilizing the first row and column to store information about zeros in the rest of the matrix.
Iterate through the matrix and use the first row and column to mark rows and columns that ne...read more
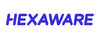
Asked in Hexaware Technologies

Q. Intersection of Two Arrays II
Given two integer arrays ARR1
and ARR2
of size N
and M
respectively, find the intersection of these arrays. An intersection refers to elements that appear in both arrays.
Note:
Input arrays/lists can ...read more
Find the intersection of two integer arrays in the order they appear in the first array.
Iterate through the first array and store elements in a hashmap with their frequencies.
Iterate through the second array and check if the element exists in the has...read more
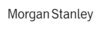
Asked in Morgan Stanley

Q. Max Element After Update Operations
Given an array A
of size N
, initialized with all zeros, and another array ARR
of M
pairs of integers representing operations. Each operation consists of a range where each element within that ra...read more
Find the maximum element in an array after performing a series of increment operations on specified ranges.
Initialize an array of size N with all zeros
Iterate through the operations and increment elements within specified ranges
Return the maximum ele...read more
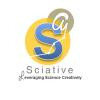
Asked in Sciative Solutions

Q. How would you approach the problem of tracing all squares on a chessboard using valid Knight moves, without repeating any square?
Tracing all squares in a chess board by valid moves of a Knight without repeating any square.
Create a 2D array to represent the chess board.
Start from any square and mark it as visited.
Generate all possible moves of a Knight from the current square.
C...read more
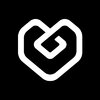
Asked in Fynd

Q. Write a program to find the count of words in a sentence using a Map data structure.
Count words in a sentence using Map
Split the sentence into an array of words
Create a Map object
Loop through the array and add each word as a key to the map with a value of 1
If the word already exists in the map, increment its value by 1
Return the map
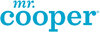
Asked in Mr Cooper

Q. Connect Ropes Problem Statement
Given a number of ropes denoted as 'N' and an array containing the lengths of these ropes, your task is to connect the ropes into one single rope. The cost to connect two ropes is determined by the ...read more
The task is to find the minimum cost required to connect all the ropes by summing their lengths.
Iterate through the ropes and connect the two shortest ropes at each step to minimize cost
Use a priority queue to efficiently find the shortest ropes
Keep ...read more
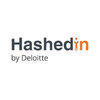
Asked in HashedIn by Deloitte

Q. Write code to find the maximum length subarray matching the given sum.
Code to find max length subarray matching the given sum
Use a sliding window approach to iterate through the array
Keep track of the current sum and the maximum length subarray
If the current sum exceeds the given sum, move the window's left pointer
If t...read more
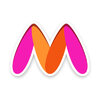
Asked in Myntra

Q. Explain all the search algorithms you know, including their space and time complexities.
Explanation of search algorithms with their space and time complexities.
Linear Search - O(n) time complexity, O(1) space complexity
Binary Search - O(log n) time complexity, O(1) space complexity
Jump Search - O(√n) time complexity, O(1) space complexi...read more
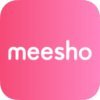
Asked in Meesho

Q. Array Intersection Problem Statement
Given two integer arrays/ lists ARR1
and ARR2
of sizes N
and M
respectively, you are required to determine their intersection. An intersection is defined as the set of common values existing in...read more
The task is to find the intersection of two integer arrays/lists.
Read the number of test cases
For each test case, read the size and elements of the first array/list
Read the size and elements of the second array/list
Find the intersection of the two ar...read more
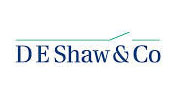
Asked in DE Shaw

Q. Write an algorithm to find the absolute max subsequence of an array containing both positive and negative numbers in O(n) time?
Algorithm to find absolute max subsequence of an array with positive and negative numbers in O(n) time.
Initialize max_so_far and max_ending_here as 0
Loop through the array and for each element, add it to max_ending_here
If max_ending_here becomes nega...read more
Algorithms Jobs
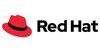
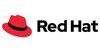
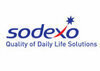
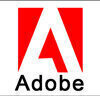
Asked in Adobe

Q. Given an array of integers nums and an integer k, return the total number of subarrays whose sum equals to k.
Given an array of integers, find if a subarray exists whose sum equals to k.
Use a hashmap to store the prefix sum and its frequency.
Iterate through the array and check if the difference between current prefix sum and k exists in the hashmap.
If it exi...read more
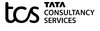
Asked in TCS

Q. Write a program to find even and odd numbers using lambda expressions.
Program to find even and odd number using lambda expression
Create a list of numbers
Use lambda expression to filter even and odd numbers
Print the even and odd numbers
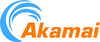
Asked in Akamai Technologies

Q. Explain BFS and DFS.
BFS and DFS are graph traversal algorithms used to search for nodes in a graph.
BFS stands for Breadth First Search and explores all the nodes at the current depth before moving to the next level.
DFS stands for Depth First Search and explores as far a...read more
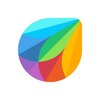
Asked in Freshworks

Q. Maximum Subarray Sum Queries
You are provided with an array of ‘N’ integers and ‘Q’ queries. Each query requires calculating the maximum subarray sum in a specified range of the array.
Input:
The first line contains ‘T’, represent...read more
Implement a function to calculate maximum subarray sum queries in a given range of an array.
Iterate through each query and calculate the maximum subarray sum within the specified range using Kadane's algorithm.
Keep track of the maximum sum found so f...read more
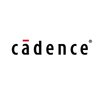
Asked in Cadence Design Systems

Q. Count Palindromic Substrings Problem Statement
Given a string STR
, determine the total number of palindromic substrings within STR
.
Input:
The first line contains an integer 't' representing the number of test cases. For each test...read more
Count the total number of palindromic substrings in a given string.
Iterate through each character in the string and expand around it to find palindromic substrings.
Use dynamic programming to store previously calculated palindromic substrings.
Consider...read more
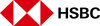
Asked in HSBC Group

Q. Search in a 2D Matrix
Given a 2D matrix MAT
of size M x N, where M and N represent the number of rows and columns respectively. Each row is sorted in non-decreasing order, and the first element of each row is greater than the last...read more
Implement a function to search for a given integer in a 2D matrix with specific properties.
Iterate through each row of the matrix and perform a binary search on each row to find the target integer.
Since the rows are sorted, binary search can be appli...read more
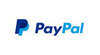
Asked in PayPal

Q. How do you detect a cycle in a graph?
To find a cycle in a graph, use depth-first search (DFS) and keep track of visited nodes.
Implement DFS algorithm to traverse the graph
Maintain a visited array to keep track of visited nodes
If a visited node is encountered again during DFS, a cycle ex...read more
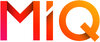
Asked in MiQ Digital

Q. How many swapping operations are required to sort the array [8,22,7,9,31,19,5,13] using the bubble sort algorithm?
The number of swaps required to sort an array using bubblesort
Bubble sort compares adjacent elements and swaps them if they are in the wrong order
Count the number of swaps required to sort the given array
The given array requires 19 swaps to be sorted...read more
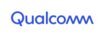
Asked in Qualcomm

Q. What is the Min-Cut placement algorithm? Given some block sizes, use the algorithm to place them on a given chip area.
Min-Cut placement algorithm is used to place blocks on a given chip area.
Min-Cut algorithm partitions the chip into two parts and minimizes the cut between them
It is a graph-based algorithm that uses a flow network to represent the chip and its block...read more
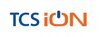
Asked in TCS iON

Q. GCD (Greatest Common Divisor) Problem Statement
You are given two numbers, X
and Y
. Your task is to determine the greatest common divisor of these two numbers.
The Greatest Common Divisor (GCD) of two integers is the largest posit...read more
The greatest common divisor (GCD) of two numbers is the largest positive integer that divides both numbers without leaving a remainder.
Use Euclidean algorithm to find GCD efficiently
GCD(X, Y) = GCD(Y, X % Y)
Repeat until Y becomes 0, then X is the GCD
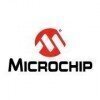
Asked in Microchip Technology

Q. Given an integer, how would you determine if it is a power of 2?
Check if a number is a power of 2.
A number is a power of 2 if it is greater than 0 and has only one bit set to 1.
Use bitwise operations to check if the number is a power of 2.
For example, 4 (100 in binary) is a power of 2, while 6 (110 in binary) is ...read more
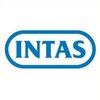
Asked in Intas Pharmaceuticals

Q. What is the key to removing duplicates?
The key to remove duplicates is to iterate through the array and keep track of unique elements.
Iterate through the array and compare each element with the rest of the elements.
If a duplicate is found, remove it from the array.
Keep track of unique ele...read more
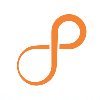
Asked in Persistent Systems

Q. Index of First Occurrence Problem Statement
Given two strings A
and B
, determine the index of the first occurrence of A
in B
. If A
is not present in B
, return -1.
Example:
Input:
A = "bc", B = "abcddbc"
Output:
1
Explanation:
The ...read more
Find the index of the first occurrence of string A in string B.
Iterate through string B and check if a substring of length equal to A matches A.
Return the index of the first occurrence of A in B, or -1 if not found.
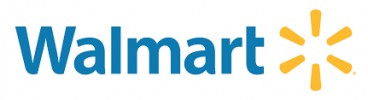
Asked in Walmart

Q. Calculate Score of Balanced Parentheses
In this intellectual game, Ninja is provided with a string of balanced parentheses called STR
. The aim is to calculate the score based on specific game rules. If Ninja wins the game by corre...read more
Calculate the score of a string of balanced parentheses based on specific game rules.
Iterate through the string and keep track of the score based on the rules provided
Use a stack to keep track of the scores of valid parentheses expressions
For each '(...read more
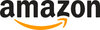
Asked in Amazon

Q. Connect Ropes with Minimum Cost
Given 'N' ropes, each having different lengths, your task is to connect these ropes into one single rope. The cost to connect two particular ropes is equal to the sum of their lengths. Please determ...read more
The task is to connect N ropes into one rope with minimum cost.
Sort the array of rope lengths in ascending order.
Initialize a variable to keep track of the total cost.
While there are more than one rope, take the two shortest ropes and connect them.
Ad...read more

Asked in JPMorgan Chase & Co.

Q. Find Permutation Problem Statement
Given an integer N
, determine an array of size 2 * N
that satisfies the following conditions:
- Each number from
1
toN
appears exactly twice in the array. - The distance between the second and firs...read more
The task is to find a permutation array of size 2*N with specific conditions.
Create an array of size 2*N to store the permutation.
Ensure each number from 1 to N appears exactly twice in the array.
Check that the distance between the second and first o...read more
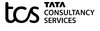
Asked in TCS

Q. What are the different types of complexities?
There are various types of complexities, such as time complexity, space complexity, algorithmic complexity, and computational complexity.
Time complexity refers to the amount of time taken by an algorithm to run, often measured in terms of the input s...read more
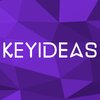
Asked in Keyideas

Q. What is the best approach to find the missing number from a set of consecutive n numbers?
One approach is to calculate the sum of all numbers in the set and then subtract the sum of the given numbers to find the missing number.
Calculate the sum of all numbers in the set using the formula n*(n+1)/2, where n is the total number of elements ...read more
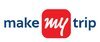
Asked in MakeMyTrip

Q. Maximum Sum of Products for Array Rotations
You are given an array ARR
consisting of N
elements. Your task is to determine the maximum value of the summation of i * ARR[i]
among all possible rotations of ARR
. Rotations can be eith...read more
Find the maximum sum of products for array rotations.
Iterate through all possible rotations of the array and calculate the sum of products for each rotation.
Keep track of the maximum sum of products found so far.
Return the maximum sum of products obt...read more
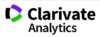
Asked in Clarivate

Q. Best Time to Buy and Sell Stock II Problem Statement
Given the stock prices for a certain number of days, represented as an array, determine the maximum profit you can achieve. You may perform as many transactions as you like, but...read more
The problem involves finding the maximum profit that can be achieved by buying and selling stocks on different days.
Iterate through the array of stock prices and find the local minima and maxima to calculate profit
Keep track of the total profit by ad...read more
Top Interview Questions for Related Skills
Interview Experiences of Popular Companies
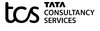
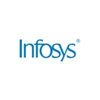
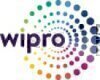
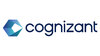
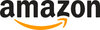
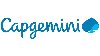
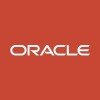
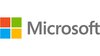
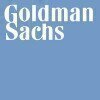
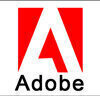
Interview Questions of Algorithms Related Designations
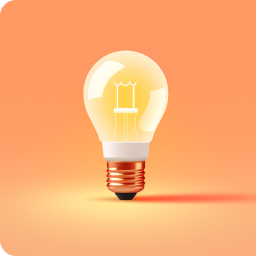
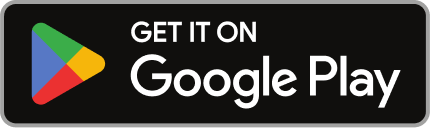
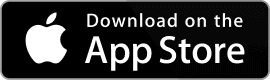
Reviews
Interviews
Salaries
Users
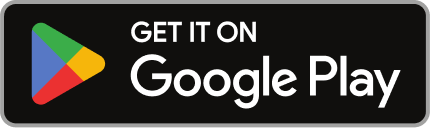
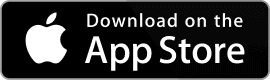