Software Developer Intern
1000+ Software Developer Intern Interview Questions and Answers
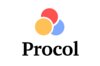
Asked in Procol

Q. Power of a Number Problem
You are given two integers, X
and N
. Your task is to compute the value of X
raised to the power of N
(i.e., X^N
).
Input:
The first line contains an integer T
, the number of test cases....read more
Compute the value of X raised to the power of N for given integers X and N.
Read the number of test cases T
For each test case, read the values of X and N
Calculate X^N for each test case
Print the result of X^N for each test case
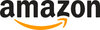
Asked in Amazon

Q. Simplify Directory Path Problem Statement
You are provided with a directory path in Unix-style notation, and your task is to simplify it according to given rules.
In a Unix-style file system:
- A dot (.) refers ...read more
The task is to simplify a given Unix-style directory path and determine the final destination.
Replace multiple slashes with a single slash
Handle dot (.) by ignoring it
Handle double dot (..) by removing the previous directory from the path
Ensure the simplified path starts with a slash and does not have a trailing slash
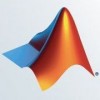
Asked in MathWorks

Q. Car Pooling Capacity Problem
You are a cab driver with a car that initially has 'C' empty seats. The car moves in a straight line towards the forward direction only. Your job is to determine if it is possible t...read more
Determine if it is possible to handle all passenger trips within the car capacity without exceeding it at any point.
Iterate through each trip and keep track of the total number of passengers in the car at each point.
Check if the total number of passengers exceeds the car capacity at any point, return 'False'. Otherwise, return 'True'.
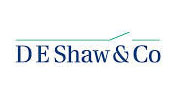
Asked in DE Shaw

Q. Minimum Removals Problem Statement
Given an integer array ARR
of size N
and an integer K
, determine the minimum number of elements that need to be removed so that the difference between the maximum and minimum ...read more
Find the minimum number of elements to remove from an array to satisfy a given condition.
Calculate the initial difference between the maximum and minimum elements in the array.
Iterate through the array and remove elements to minimize the difference.
Return the count of elements removed as the answer.
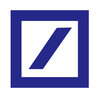
Asked in Deutsche Bank

Q. Next Smaller Palindrome Problem Statement
You are provided with a palindrome number 'N' presented as a string 'S'. The task is to determine the largest palindrome number that is strictly less than 'N'.
Input:
T...read more
Given a palindrome number 'N' as a string, find the largest palindrome number strictly less than 'N'.
Iterate from the middle towards the start and end of the number to find the next smaller palindrome.
Handle cases where the middle digit is 0 by borrowing from adjacent digits.
Ensure the resulting number does not have leading zeros, except when the answer is 0.

Asked in VMware Software

Q. Reverse the String Problem Statement
You are given a string STR
which contains alphabets, numbers, and special characters. Your task is to reverse the string.
Example:
Input:
STR = "abcde"
Output:
"edcba"
Input...read more
Reverse a given string containing alphabets, numbers, and special characters.
Iterate through the string from the end to the beginning and append each character to a new string.
Use built-in functions like reverse() or slicing to reverse the string.
Handle special characters and numbers while reversing the string.
Ensure to consider the constraints on the input string length and number of test cases.
Software Developer Intern Jobs
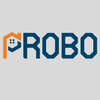
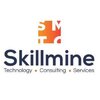
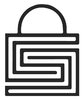
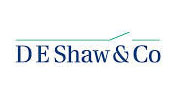
Asked in DE Shaw

Q. Water Equalization Problem Statement
You are provided with an array ARR
of positive integers. Each integer represents the number of liters of water in a bucket. The goal is to make the liters of water in every ...read more
Given an array of water buckets, find the minimum liters of water to remove to make all buckets equal.
Iterate through the array to find the maximum frequency of a water amount
Calculate the total liters to be removed by subtracting the maximum frequency from the total number of buckets
Return the total liters to be removed as the answer
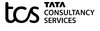
Asked in TCS

Q. Find Duplicates in an Array
Given an array ARR
of size 'N', where each integer is in the range from 0 to N - 1, identify all elements that appear more than once.
Return the duplicate elements in any order. If n...read more
Find duplicates in an array of integers within a specified range.
Iterate through the array and keep track of the count of each element using a hashmap.
Return elements with count greater than 1 as duplicates.
Time complexity can be optimized to O(N) using a HashSet to store seen elements.
Share interview questions and help millions of jobseekers 🌟
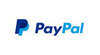
Asked in PayPal

Q. Maximum Difference Problem Statement
Given an array ARR
of N
elements, your task is to find the maximum difference between any two elements in ARR
.
If the maximum difference is even, print EVEN; if it is odd, p...read more
Find the maximum difference between any two elements in an array and determine if it is even or odd.
Iterate through the array to find the maximum and minimum elements.
Calculate the difference between the maximum and minimum elements.
Check if the difference is even or odd and return the result.
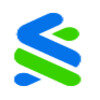
Asked in Standard Chartered

Q. Ways To Make Coin Change
Given an infinite supply of coins of varying denominations, determine the total number of ways to make change for a specified value using these coins. If it's not possible to make the c...read more
The task is to find the total number of ways to make change for a specified value using given denominations.
Use dynamic programming to keep track of the number of ways to make change for each value up to the target value.
Iterate through each denomination and update the number of ways to make change for each value based on the current denomination.
The final answer will be the number of ways to make change for the target value.
Consider edge cases such as when the target value i...read more
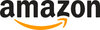
Asked in Amazon

Q. Add Two Numbers Represented by Linked Lists
Your task is to find the sum list of two numbers represented by linked lists and return the head of the sum list.
Explanation:
The sum list should be a linked list re...read more
Add two numbers represented by linked lists and return the head of the sum list.
Traverse both linked lists simultaneously while keeping track of carry from previous sum
Create a new linked list to store the sum of the two numbers
Handle cases where one linked list is longer than the other by padding with zeros
Update the sum and carry values accordingly while iterating through the linked lists
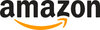
Asked in Amazon

Q. Anagram Pairs Verification Problem
Your task is to determine if two given strings are anagrams of each other. Two strings are considered anagrams if you can rearrange the letters of one string to form the other...read more
The task is to determine whether two given strings form an anagram pair or not.
Anagrams are words or names that can be formed by rearranging the letters of another word
Check if the two strings have the same characters with the same frequency
Convert the strings to character arrays and sort them
Compare the sorted arrays to check if they are equal
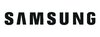
Asked in Samsung

Q. Count Leaf Nodes in a Binary Tree
Count the number of leaf nodes present in a given binary tree. A binary tree is a data structure where each node has at most two children, known as the left child and the right...read more
Count the number of leaf nodes in a binary tree where each node has at most two children.
Traverse the binary tree and count nodes with both children as NULL.
Use recursion to check each node in the tree.
Leaf nodes have no child nodes.
Example: For input 20 10 35 5 15 30 42 -1 13 -1 -1 -1 -1 -1 -1 -1, output should be 4.
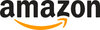
Asked in Amazon

Q. Longest Common Subsequence Problem Statement
Given two strings, S
and T
with respective lengths M
and N
, your task is to determine the length of their longest common subsequence.
A subsequence is a sequence tha...read more
The task is to find the length of the longest common subsequence between two given strings.
A subsequence is a string that can be derived from another string by deleting some or no characters without changing the order of the remaining characters.
We can solve this problem using dynamic programming.
Create a 2D array to store the lengths of the longest common subsequences for all possible prefixes of the two strings.
Iterate through the strings and fill the array based on the fol...read more
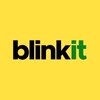
Asked in Blinkit

Q. Maximum Possible Time Problem Statement
Given an array ARR
consisting of exactly four integer digits, your task is to form the maximum possible valid time in a 24-hour format using those digits.
Explanation:
Th...read more
Given an array of four digits, form the maximum valid 24-hour time. Return -1 if not possible.
Iterate through all permutations of the digits to find the maximum valid time.
Check if the time is valid (between 00:00 and 23:59) before returning.
Handle cases where no valid time can be generated by returning -1.
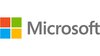
Asked in Microsoft Corporation

Q. Mean, Median, Mode Calculation
You are given an array 'ARR' consisting of 'N' integers. Your task is to calculate the three statistical measures for the given array:
- Mean - Implement the function
mean()
to cal...read more
Implement functions to calculate mean, median, and mode of an array of integers.
Implement a function mean() to calculate the mean of the array by summing all elements and dividing by the total count.
Implement a function median() to calculate the median of the array by sorting the array and finding the middle element(s).
Implement a function mode() to find the mode of the array by counting frequencies and returning the smallest element with the highest frequency.
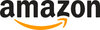
Asked in Amazon

Q. Ninja's Jump Task
The Ninja has been given a challenge by his master to reach the last stone. These stones are represented as an array of numbers. The Ninja can jump using either odd-numbered or even-numbered j...read more
Find the number of starting indices from which a Ninja can reach the last stone by following specific jump rules.
Iterate through the array and keep track of the possible jumps for each index based on the rules provided.
Use dynamic programming or a stack to efficiently calculate the number of starting indices.
Consider edge cases where some indices may have no possible jumps.
Example: For the input [10, 13, 12, 14, 15], the Ninja can start from indices 0 and 1 to reach the last ...read more
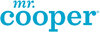
Asked in Mr Cooper

Q. Sum of LCM Problem Statement
Given an integer 'N', calculate and print the sum of the least common multiples (LCM) for each integer from 1 to N with N.
The sum is represented as:LCM(1, N) + LCM(2, N) + ... + LC...read more
Calculate and print the sum of least common multiples (LCM) for each integer from 1 to N with N.
Iterate from 1 to N and calculate LCM of each number with N
Sum up all the calculated LCMs to get the final result
Implement a function to calculate LCM of two numbers
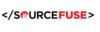
Asked in Sourcefuse Technologies

Q. Count Ways to Climb Stairs Problem
Given a staircase with a certain number of steps, you start at the 0th step, and your goal is to reach the Nth step. At every step, you have the option to move either one step...read more
Count the number of distinct ways to climb stairs by moving either one step or two steps at a time.
Use dynamic programming to solve the problem efficiently.
Keep track of the number of ways to reach each step by considering the number of ways to reach the previous two steps.
Return the result modulo 10^9+7 to handle large outputs.
Example: For N=4, ways to climb are {0,1,2,3,4}, {0,1,3,4}, {0,2,4}. Total 3 ways.
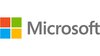
Asked in Microsoft Corporation

Q. Find All Triplets with Zero Sum
Given an array Arr
consisting of N
integers, find all distinct triplets in the array that sum up to zero.
Explanation:
An array is said to have a triplet {Arr[i], Arr[j], Arr[k]}...read more
The task is to find all distinct triplets in an array that add up to zero.
Iterate through the array and fix the first element of the triplet.
Use two pointers approach to find the other two elements that sum up to the negative of the fixed element.
Skip duplicate elements to avoid duplicate triplets.
Return the list of triplets found.
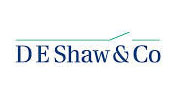
Asked in DE Shaw

Q. Majority Element - II Problem Statement
Given an array/list ARR
of integers with length 'N', identify all elements that appear more than floor(N/3)
times within the array/list.
Input:
T (number of test cases)
Fo...read more
Identify elements in an array that appear more than floor(N/3) times using efficient counting methods.
Use a HashMap to count occurrences of each element in the array.
Calculate the threshold as floor(N/3) to determine majority elements.
Iterate through the HashMap to collect elements that exceed the threshold.
Example: For array [3, 2, 3], floor(3/3) = 1, so 3 is a majority element.
Example: For array [1, 1, 2, 2, 3, 3], floor(6/3) = 2, so 1, 2, and 3 are majority elements.

Asked in JPMorgan Chase & Co.

Q. Minimum Travel Cost Problem
You are given a country called 'Ninjaland' with 'N' states, numbered from 1 to 'N'. These states are connected by 'M' bidirectional roads, each with a specified travel cost. The aim ...read more
The task is to select 'N' - 1 roads in a country with 'N' states, such that the tourist bus can travel to every state at least once at minimum cost.
The problem can be solved using a minimum spanning tree algorithm, such as Kruskal's algorithm or Prim's algorithm.
Create a graph representation of the country using the given roads and their costs.
Apply the minimum spanning tree algorithm to find the minimum cost roads that connect all the states.
Print the selected roads and thei...read more
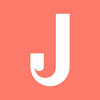
Asked in Jupiter Money

Q. Partial BST Problem Statement
Check if a given binary tree is a Partial Binary Search Tree (BST). A Partial BST adheres to the following properties:
- The left subtree of a node contains only nodes with data les...read more
Check if a binary tree is a Partial Binary Search Tree (BST) based on specific properties.
Traverse the tree in level order and check if each node satisfies the properties of a Partial BST.
Use recursion to check if the left and right subtrees are also Partial BSTs.
Compare the data of each node with its children to ensure the BST properties are maintained.
Example: For input 1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1, the output should be true.
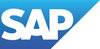
Asked in SAP

Q. Subarray Sums I Problem Statement
You are provided with an array of positive integers ARR
that represents the strengths of different “jutsus” (ninja techniques). You are also given the strength of the enemy S
, ...read more
Count the number of subarrays whose combined strength matches the given enemy strength.
Iterate through all subarrays and calculate their sum to check if it matches the enemy strength.
Use two pointers technique to efficiently find subarrays with sum equal to the enemy strength.
Consider edge cases like when the enemy strength is 0 or when all elements in the array are equal to the enemy strength.
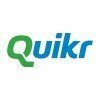
Asked in Quikr

Q. Subsequences of String Problem Statement
You are provided with a string 'STR'
that consists of lowercase English letters ranging from 'a' to 'z'. Your task is to determine all non-empty possible subsequences of...read more
Generate all possible subsequences of a given string.
Use recursion to generate all possible subsequences by including or excluding each character in the string.
Maintain the order of characters while generating subsequences.
Handle base cases where the string is empty or has only one character.
Store the generated subsequences in an array of strings.
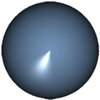
Asked in HPC Sphere

Q. Valid Stack Permutation Problem
Determine if one array is a valid stack permutation of another given array. An array is a valid stack permutation of another if by performing some sequence of push and pop operat...read more
Determine if one array is a valid stack permutation of another given array by performing push and pop operations.
Check if the elements in the 'FIRST' array can be obtained by performing push and pop operations on the 'OTHER' array.
Maintain a stack to simulate the push and pop operations.
If the 'FIRST' array can be obtained, output 'YES'; otherwise, output 'NO'.
Asked in Imaginnovate

Q. How do we link external CSS and JS files to HTML?
External CSS and JS files can be linked to HTML using the <link> and <script> tags respectively.
Use the <link> tag with the 'rel' attribute set to 'stylesheet' to link external CSS files.
Use the <script> tag with the 'src' attribute to link external JS files.
Place the <link> and <script> tags within the <head> section of the HTML document.
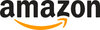
Asked in Amazon

Q. Clone Linked List with Random Pointer Problem Statement
Given a linked list where each node has two pointers: one pointing to the next node and another which can point randomly to any node in the list or null, ...read more
Cloning a linked list with random pointers by creating new nodes rather than copying references.
Create a deep copy of the linked list by iterating through the original list and creating new nodes with the same values.
Update the random pointers of the new nodes by mapping the original node's random pointer index to the corresponding new node.
Ensure the cloned linked list is an exact copy of the original by validating the values and random pointers.
The cloning process can be ac...read more
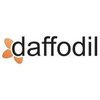
Asked in Daffodil Software

Q. Find the Longest Palindromic Substring
Given a string ‘S’ composed of lowercase English letters, your task is to identify the longest palindromic substring within ‘S’.
If there are multiple longest palindromic ...read more
Find the longest palindromic substring in a given string, returning the rightmost one if multiple substrings are of the same length.
Use dynamic programming to check for palindromes within the string.
Start by checking for palindromes of length 1 and 2, then expand to longer substrings.
Keep track of the longest palindromic substring found so far and return the rightmost one if multiple substrings are of the same length.
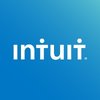
Asked in Intuit

Q. Grid Satisfaction Problem
In this problem, you are given a grid of size N*M containing two types of people: type ‘A’ and type ‘B’. You are given the number of people of each type: 'countA' denotes the number of...read more
Given a grid with type A and B people, maximize satisfaction based on neighbors.
Start with type A people for maximum satisfaction
Optimally place people to maximize satisfaction
Consider satisfaction levels for each type of person and their neighbors
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
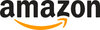
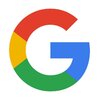

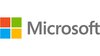
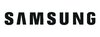
Top Interview Questions for Software Developer Intern Related Skills
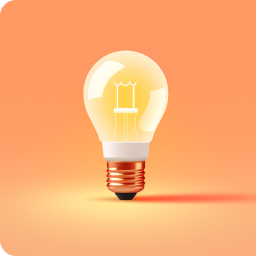
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
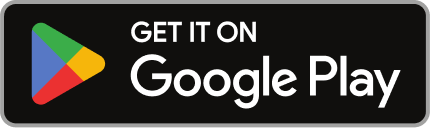
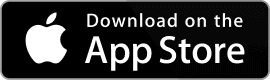
Reviews
Interviews
Salaries
Users
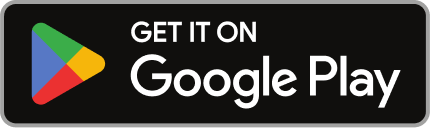
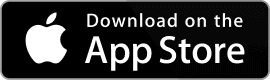