Senior Android Developer
100+ Senior Android Developer Interview Questions and Answers
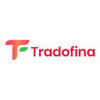
Asked in Tradofina

Q. 1 . What are SOLID principles? 2. Kotlin Flows 3. What is MVVM 4. Performance Optimization
SOLID principles are five design principles that help developers create maintainable and scalable software systems.
Single Responsibility Principle (SRP): A class should have only one reason to change, meaning it should only have one job. For example, a User class should only handle user data, not user authentication.
Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification. For instance, you can add new features through inheritanc...read more
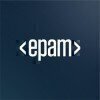
Asked in EPAM Systems

Q. What is the root class in Java and what is it used for?
The root class in Java is the Object class, which is the parent class for all other classes in Java.
The Object class is used to provide common methods and behaviors that are inherited by all other classes.
It defines methods like toString(), equals(), and hashCode() that can be overridden by subclasses.
All classes in Java implicitly extend the Object class, either directly or indirectly.
For example, the String class extends the Object class.
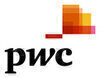
Asked in PwC

Q. What Android application components did you use in your previous project? Give an example.
The Android application components used in the previous project include activities, services, broadcast receivers, and content providers.
Activities: Used for the user interface and interaction with the user. Example: MainActivity.java
Services: Used for background tasks or long-running operations. Example: DownloadService.java
Broadcast Receivers: Used for system-wide events or notifications. Example: ConnectivityReceiver.java
Content Providers: Used for data management and shar...read more
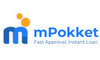
Asked in mPokket

Q. Difference between Authentication and Authorisation. What Coroutine makes it different than threads. Small DS algorithm. Practical usage of viewmodel and coroutine. What is livedata.
Authentication is the process of verifying the identity of a user, while authorization is the process of granting access to specific resources.
Authentication ensures that the user is who they claim to be, usually through credentials like username and password.
Authorization determines what actions a user is allowed to perform on a system or resource.
Coroutines are lightweight threads that allow for asynchronous programming in a more sequential and structured manner.
Coroutines ...read more
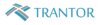
Asked in Trantor

Q. Given a Kotlin extension function, what could be the output?
Kotlin extension functions allow adding new functions to existing classes without modifying their source code.
Extension functions are defined outside the class they extend.
They can be called on the object of the class they extend.
They can access the properties and functions of the class they extend.

Asked in MatchMove

Q. Advantage of MVC, MVP, MVVM Glide vs Picasso Bitmap Sampling what is viewStub how to achieve fix size in Recyclerview. Inline vs normal function. Work request in Work manager
MVC, MVP, MVVM are design patterns for organizing code. Glide and Picasso are image loading libraries. Bitmap sampling is resizing images. ViewStub is a lightweight view. RecyclerView can achieve fixed size with setHasFixedSize(true). Inline functions are defined within a class. WorkManager can schedule background tasks.
MVC, MVP, MVVM are design patterns for organizing code
Glide and Picasso are popular image loading libraries
Bitmap sampling is resizing images to reduce memory...read more
Senior Android Developer Jobs

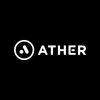
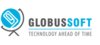
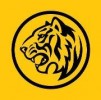
Asked in Maybank

Q. What is push notification and how implemented in android
Push notification is a message that pops up on a mobile device. It can be implemented in Android using Firebase Cloud Messaging (FCM) or Google Cloud Messaging (GCM).
Push notifications are messages sent from a server to a client app that wakes up the app to inform the user of new messages or events.
In Android, push notifications can be implemented using Firebase Cloud Messaging (FCM) or Google Cloud Messaging (GCM) APIs.
Developers need to set up a server to send push notifica...read more
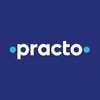
Asked in Practo

Q. Solve a two-pointer array question on the whiteboard.
Two-pointer technique efficiently solves array problems by using two indices to traverse and manipulate elements.
The two-pointer technique is useful for problems involving sorted arrays, such as finding pairs that sum to a target.
Example: Given an array [1, 2, 3, 4, 5] and target 6, use pointers to find pairs (1, 5), (2, 4), (3, 3).
It can also be used for removing duplicates from a sorted array by maintaining one pointer for unique elements.
Example: For [1, 1, 2, 2, 3], use o...read more
Share interview questions and help millions of jobseekers 🌟
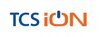
Asked in TCS iON

Q. You are given an integer array nums. You are initially positioned at the array's first index, and each element in the array represents your maximum jump length at that position. Return true if you can reach the...
read moreDetermine if you can jump to the last index of an array based on jump lengths.
The problem involves an array where each element represents the maximum jump length from that position.
You can use a greedy approach to track the furthest index you can reach as you iterate through the array.
Example: For array [2,3,1,1,4], you can jump to index 4 (last index) from index 0.
If at any point the current index exceeds the furthest reachable index, return false.
Example: For array [3,2,1,0...read more
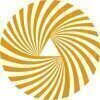
Asked in Altimetrik

Q. What is Flow, Channel diffrent between StateFlow and SharedFlow
Flow is a cold asynchronous data stream, Channel is a hot asynchronous data stream. StateFlow is a hot asynchronous data stream with a state, SharedFlow is a hot asynchronous data stream without a state.
Flow is a cold asynchronous data stream that emits values one by one.
Channel is a hot asynchronous data stream that can have multiple subscribers.
StateFlow is a hot asynchronous data stream that retains the most recent value and emits it to new subscribers.
SharedFlow is a hot ...read more
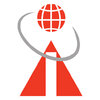
Asked in Calibrage Info Systems

Q. What are the differences between Activity, Fragment, and View? When should each be used?
Activities are standalone components, Fragments are modular sections of UI, and Views are UI elements within them.
Activity: Represents a single screen with a user interface. Example: MainActivity in a messaging app.
Fragment: A reusable portion of UI within an Activity. Example: ChatFragment displaying messages.
View: The basic building block for UI components. Example: TextView, Button, etc.
Use Activity for full-screen interfaces, Fragment for modular UI, and View for individu...read more
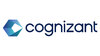
Asked in Cognizant

Q. How do you handle multiple view types in RecyclerView?
Multiple views in RecyclerView can be handled by using different view types and view holders.
Use getItemViewType() method to return different view types based on position
Create multiple view holders for each view type
Bind data to each view holder based on its type
Example: Creating a chat app with different message types like text, image, and video
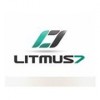
Asked in Litmus7 Systems Consulting

Q. Volatile keyword uses, refied keyword and its use in generics
Volatile keyword ensures visibility of changes to variables across threads. Reified keyword is used to access type information at runtime in generics.
Volatile keyword is used to indicate that a variable's value will be modified by different threads.
It ensures that changes made by one thread to a volatile variable are visible to other threads.
Reified keyword is used in generics to access type information at runtime, which is not possible with regular generics due to type erasu...read more
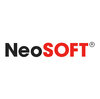
Asked in NeoSOFT

Q. What are the different types of interfaces?
Different types of interfaces include user interfaces, hardware interfaces, and software interfaces.
User interfaces: allow users to interact with the system, such as graphical user interfaces (GUI) and command-line interfaces (CLI)
Hardware interfaces: connect hardware components to the system, such as USB, HDMI, and Ethernet ports
Software interfaces: define how software components interact with each other, such as application programming interfaces (APIs) and network protocol...read more
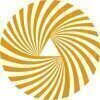
Asked in Altimetrik

Q. What is inline function and highorder function?
Inline functions are functions that are expanded in place at the call site, while high-order functions are functions that can take other functions as parameters or return them.
Inline functions are expanded in place at the call site to improve performance.
High-order functions can take other functions as parameters or return them.
Example of high-order function: map() function in Kotlin.
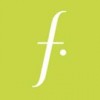
Asked in Falabella

Q. What are coroutines, and how do they work internally?
Coroutines are a concurrency design pattern in Kotlin that allow for asynchronous programming.
Coroutines are lightweight threads that can be used to perform non-blocking operations.
They are based on the concept of suspending functions, which can be paused and resumed.
Coroutines use a dispatcher to determine which thread or thread pool to run on.
They can be used to simplify asynchronous code and avoid callback hell.
Coroutines can be used with various libraries and frameworks, ...read more
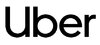
Asked in Uber

Q. Design a question that checks OOPS concepts and data structures.
Design a library management system using OOP principles and appropriate data structures.
Use classes for Book, Member, and Library to encapsulate related data and behaviors.
Implement inheritance for different types of books (e.g., Fiction, Non-Fiction).
Utilize a HashMap to store books for quick lookup by ISBN.
Implement a queue for managing book requests from members.
Use ArrayList to maintain a dynamic list of members.
Asked in SugarBox Networks

Q. How did you solve a particular problem regarding Android development?
I solved a problem by implementing a custom view to display complex data in a more user-friendly way.
Identified the specific requirements and limitations of the problem
Researched and experimented with different solutions, including using third-party libraries
Implemented a custom view with optimized performance and user experience
Tested the solution thoroughly to ensure it met the desired functionality
Asked in SugarBox Networks

Q. What is the difference between a Service and an IntentService?
Service is a base class for Android services that can run in the background, while IntentService is a subclass of Service that handles asynchronous requests on demand.
Service is a base class for Android services that can run indefinitely in the background, while IntentService is a subclass of Service that handles asynchronous requests one at a time.
Service does not have a default worker thread, so you need to manage the thread manually, whereas IntentService creates a worker ...read more
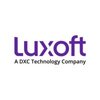
Asked in Luxoft

Q. What is Car PropertyManager and how is it used in AOSP?
Car propertymanager is a component in AOSP that manages properties related to car-specific features.
Car propertymanager is used to handle properties like car make, model, year, etc.
It is mostly used in Android Automotive OS for managing car-specific settings and configurations.
Developers can use car propertymanager to access and modify car-related properties in their apps.
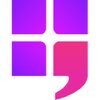
Asked in AppyHigh

Q. Explain the Room database and its queries, and design a database for an app.
Room Database is an abstraction layer over SQLite for Android, simplifying data persistence with a robust ORM framework.
Room provides an easy way to manage SQLite databases in Android apps.
It uses annotations to define entities, DAOs, and database classes.
Example of an entity: @Entity(tableName = 'users') public class User { ... }
Queries are defined in DAO interfaces using @Query annotation.
Example of a query: @Query('SELECT * FROM users WHERE id = :userId') User getUserById(...read more
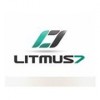
Asked in Litmus7 Systems Consulting

Q. What are the pros and cons of multi-module architecture in Android?
Multi module architecture in Android involves breaking down an app into multiple modules for better organization and scalability.
Pros: better organization, easier maintenance, faster build times, code reusability
Cons: increased complexity, potential for dependency issues, learning curve for developers
Example: Breaking down an app into separate modules for UI, networking, and data storage can make it easier to work on different parts of the app independently.

Asked in CureSkin

Q. Given a range of numbers from x to y, how would you count the numbers that end with 2, 3, or 7?
Count pretty numbers ending with 2, 3, or 7 within a given range.
Iterate through the range from x to y and check if each number ends with 2, 3, or 7.
Increment a counter for each pretty number found.
Return the total count of pretty numbers within the range.
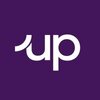
Asked in Upstox

Q. What is FnO and how did you implement this feature?
FnO stands for Futures and Options, a type of financial derivative. Implemented feature by integrating real-time market data and enabling users to trade contracts.
FnO is a type of financial derivative where parties agree to buy or sell an asset at a specified price on a future date.
Implemented feature by integrating real-time market data feeds to provide up-to-date information on contract prices.
Enabled users to trade futures and options contracts through the app, with featur...read more
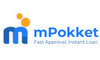
Asked in mPokket

Q. Write a high-level design for the given app feature.
Design a feature for an app
Identify the purpose and goals of the feature
Define the user flow and interactions
Consider the technical requirements and constraints
Design the UI/UX
Plan the data model and storage
Consider scalability and performance
Implement and test the feature
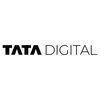
Asked in Tata Digital

Q. What is IPC and RPC?
IPC stands for Inter-Process Communication and RPC stands for Remote Procedure Call.
IPC is a mechanism that allows communication between processes running on the same or different devices.
RPC is a protocol that enables one program to request a service from another program on a remote machine.
IPC can be achieved through shared memory, message passing, and pipes.
RPC uses a client-server model and can be implemented using various protocols such as HTTP, TCP, and UDP.
Both IPC and...read more
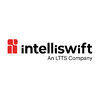
Asked in Intelliswift - An LTTS Company

Q. In Compose UI, how do you prevent recomposition?
In Compose UI, recomposition can be minimized using various techniques to optimize performance and maintain UI state.
Use `remember` to store state across recompositions: `val count = remember { mutableStateOf(0) }`.
Leverage `derivedStateOf` to create derived states that only recompute when their dependencies change.
Utilize `key` in lists to help Compose identify items and avoid unnecessary recompositions: `LazyColumn { items(itemsList, key = { it.id }) { ... } }`.
Break down c...read more
Asked in SugarBox Networks

Q. What are the different launch modes in Android?
Different launch modes in Android control how a new instance of an activity is associated with the current task.
Standard - The default launch mode, creates a new instance of the activity in the task.
SingleTop - If an instance of the activity already exists at the top of the task, it will not be recreated.
SingleTask - A new task will always be created and a new instance of the activity will be launched in it.
SingleInstance - The activity will be the only one in the task, no ot...read more

Asked in Money View

Q. Kotlin Scope functions are extension functions. Write an extension function for let.
Kotlin's 'let' is a scope function that allows executing a block of code on a nullable object.
1. 'let' is called on an object and provides it as 'it' inside the block.
2. It is useful for null safety, allowing operations on non-null objects.
3. Example: val result = myString?.let { it.length } // returns length if myString is not null.
4. It can be chained with other scope functions for cleaner code.
5. 'let' can also be used to transform data and return a new value.

Asked in Testbook.com

Q. What are Dispatchers in Kotlin Coroutines?
Dispatchers in Kotlin Coroutines are responsible for determining which thread or threads the coroutines should run on.
Dispatchers help manage the execution of coroutines on different threads.
There are different types of Dispatchers like Default, IO, Main, and Unconfined.
Example: launch(Dispatchers.IO) { // perform network operations }
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
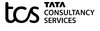
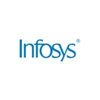
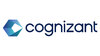
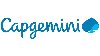
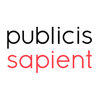
Top Interview Questions for Senior Android Developer Related Skills
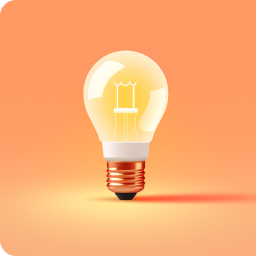
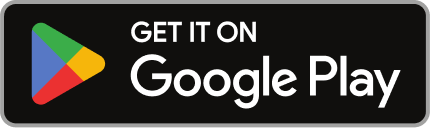
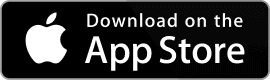
Reviews
Interviews
Salaries
Users
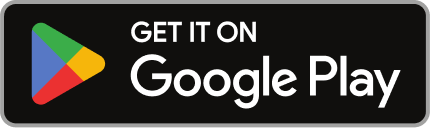
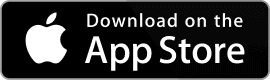