Senior Android Developer
100+ Senior Android Developer Interview Questions and Answers
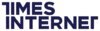
Asked in Times Internet

Q. Binary Array Sorting Problem Statement
You are provided with a binary array, i.e., an array containing only 0s and 1s. Your task is to sort this binary array and return it after sorting.
Input:
The first line ...read more
Yes, the binary array sorting problem can be solved in linear time and constant space by using a two-pointer approach.
Use two pointers, one starting from the beginning of the array and the other starting from the end.
Swap 0s to the left side and 1s to the right side by incrementing and decrementing the pointers accordingly.
Continue this process until the two pointers meet in the middle of the array.
Example: Input: [1, 0, 1, 0, 1], Output: [0, 0, 1, 1, 1]
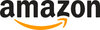
Asked in Amazon

Q. Maximum Subarray Sum Problem Statement
Given an array arr
of length N
consisting of integers, find the sum of the subarray (including empty subarray) with the maximum sum among all subarrays.
Explanation:
A sub...read more
Find the sum of the subarray with the maximum sum among all subarrays in a given array.
Iterate through the array and keep track of the maximum sum subarray encountered so far.
Use Kadane's algorithm to efficiently find the maximum subarray sum.
Consider the case where all elements in the array are negative.
Handle the case where the array contains only one element.
Senior Android Developer Interview Questions and Answers for Freshers
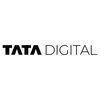
Asked in Tata Digital

Q. what is daemon Thread? what is data Class? Garbage Collector? Activity Lifecycle? what is Fragment? what took you used for App Debugging ? how to revert changes pushed in GIT? write down example of Data class i...
read moreQuestions related to Android development concepts and practices.
Daemon thread is a low priority thread that runs in the background.
Data class is a class that is used to hold data and provides default implementations of common methods like equals, hashCode, and toString.
Garbage collector is a mechanism that automatically frees up memory by removing objects that are no longer in use.
Activity lifecycle refers to the various states an activity can be in, such as onCreate, onStart...read more
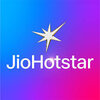
Asked in JioStar

Q. How would you design an app with a configurable UI where the UI configurations come from an API?
Design an Android app with a dynamic UI based on API configurations for flexible user experiences.
Use a JSON schema to define UI components and their properties.
Implement a factory pattern to create UI elements based on the configuration.
Utilize RecyclerView for dynamic lists, allowing for different item types.
Incorporate data binding to link UI components with data models seamlessly.
Consider using a library like Jetpack Compose for a more declarative UI approach.
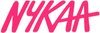
Asked in Nykaa

Q. What is a Service? How can a notification in a Foreground Service interact with other components e.g. buttons in a music player?
A Service is a component that runs in the background to perform long-running operations. A Foreground Service is a Service that has a notification to show the user that it is running.
A Service is used to perform long-running operations in the background, such as playing music in a music player app.
A Foreground Service is a type of Service that has a notification to indicate to the user that it is running and to give it higher priority, making it less likely to be killed by th...read more
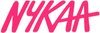
Asked in Nykaa

Q. Which data structure is used to store views in a layout?
The data structure used to store views in a layout is a tree structure.
Views in a layout are stored in a hierarchical tree structure.
Each view is a node in the tree, with parent-child relationships defining the layout.
Examples include LinearLayout, RelativeLayout, and ConstraintLayout.
Senior Android Developer Jobs

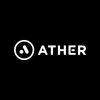
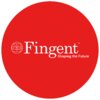
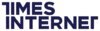
Asked in Times Internet

ViewModel in Android is a class that is responsible for preparing and managing data for an activity or fragment.
ViewModel is part of the Android Architecture Components and is used to store and manage UI-related data in a lifecycle-conscious way.
It survives configuration changes such as screen rotations and retains data during the lifecycle of the activity or fragment.
ViewModel should never hold a reference to a view or any class that has a reference to the activity context t...read more
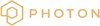
Asked in Photon Interactive

Q. Write a function to find the vowels in a string and convert them to uppercase.
Convert vowels in a string to uppercase
Iterate through each character in the string
Check if the character is a vowel (a, e, i, o, u)
If it is a vowel, convert it to uppercase
Share interview questions and help millions of jobseekers 🌟
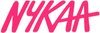
Asked in Nykaa

Q. What functions would you add to an Image Loader library?
Functions to add in an Image Loader library for Senior Android Developer role
Support for loading images from different sources like network, local storage, and resources
Caching mechanism to improve performance and reduce network calls
Ability to handle image loading in different formats like JPEG, PNG, GIF, etc.
Support for placeholder images while the actual image is being loaded
Error handling for failed image loading requests
Option to customize image loading behavior like res...read more
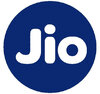
Asked in Jio

Q. 1. What is happen when App is launch on Android 2. Internal working of MVVM 3. System design for 2 page App that has one listing and detail page 4. Clean Architecture 5. How garbage collector works 6. Move even...
read moreAnswers to various technical questions related to Android development.
1. When an app is launched on Android, the system creates a new instance of the Activity class for the main activity and loads the layout.
2. MVVM (Model-View-ViewModel) is a design pattern that separates the UI, business logic, and data. The View interacts with the ViewModel to update the data.
3. For a 2-page app with a listing and detail page, you can design a navigation flow using activities or fragments....read more
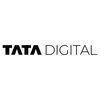
Asked in Tata Digital

Q. Write a program to print prime numbers between 100 and 100,000.
Print prime numbers between 100 to 1,00,000
Start with a loop from 100 to 100000
Check if the current number is prime or not
If prime, print the number
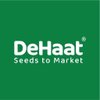
Asked in DeHaat

Q. What is an activity, service, view model, live data and jetpack compose
Activity, service, view model, live data, and Jetpack Compose are key components in Android development.
Activity: Represents a single screen with a user interface. Example: MainActivity.
Service: Performs long-running operations in the background without a user interface. Example: MusicPlayerService.
View Model: Stores and manages UI-related data in a lifecycle-conscious way. Example: MainViewModel.
Live Data: Data holder class that is lifecycle-aware and ensures UI updates only...read more
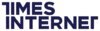
Asked in Times Internet

Q. Data structure and algorithm : find maximum sum subarray. Separate zeroes and ones in array. Android questions : viewmodel under the hood, recycler view under the hood, implement viewmodel.
Questions on data structure, algorithm, and Android development.
To find maximum sum subarray, use Kadane's algorithm
To separate zeroes and ones in array, use two pointers approach
ViewModel is a class that stores and manages UI-related data
RecyclerView is a flexible view for providing a limited window into a large data set
To implement ViewModel, extend ViewModel class and override onCleared() method
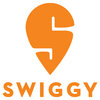
Asked in Swiggy

RecyclerView is a flexible view for providing a limited window into a large data set.
RecyclerView recycles views to improve performance and memory usage.
It uses a LayoutManager to organize and position items within the view.
Adapter provides data to be displayed in the RecyclerView.
ItemDecoration allows for custom drawing of item decorations like borders or dividers.
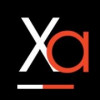
Asked in Xapads Media

Q. How can you recover the uninstall rate of an Android app?
To recover uninstall rate of an android app, analyze user feedback, improve app performance, and offer incentives.
Analyze user feedback to identify reasons for uninstallation
Improve app performance to reduce crashes and bugs
Offer incentives such as discounts or exclusive content to encourage reinstallation
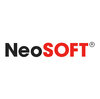
Asked in NeoSOFT

Q. What are the reasons for using that, and what are its pros and cons?
Using dependency injection in Android development can improve code maintainability and testability.
Pros: easier to manage dependencies, promotes code reusability, facilitates unit testing
Cons: initial setup can be complex, may introduce overhead in smaller projects
Example: Using Dagger 2 for dependency injection in an Android project
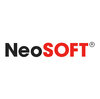
Asked in NeoSOFT

Q. What is the output of the program when the expression is evaluated as 0 divided by 7?
The output of the program when 0 is divided by 7 is 0.
Division of 0 by any number results in 0.
In programming languages, dividing by 0 usually results in an error or undefined behavior.
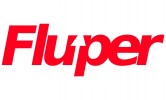
Asked in Fluper

Q. What is Google firebase? How to integrate FCM. why do you like not
Firebase is a mobile and web application development platform developed by Google. FCM is Firebase Cloud Messaging, a messaging service for Android, iOS, and web applications.
Firebase provides a range of services including authentication, real-time database, cloud storage, and hosting.
FCM allows developers to send notifications and messages to users on Android, iOS, and web applications.
To integrate FCM, developers need to add the Firebase SDK to their app and configure the F...read more
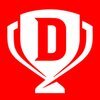
Asked in Dream11

Q. Given an array containing only 1s and 0s, find the largest subarray with an equal number of 1s and 0s.
Find largest subarray with equal number of 1s and 0s
Use a hashmap to store the count of 1s and 0s encountered so far
If the count of 1s and 0s is equal at any point, calculate the length of subarray
Keep track of the maximum length subarray found so far
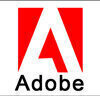
Asked in Adobe

Q. How do you detect a loop in a linked list?
To detect loop in linked list, use Floyd's cycle-finding algorithm.
Create two pointers, slow and fast, and initialize both to the head of the linked list.
Move slow pointer by one node and fast pointer by two nodes.
If there is a loop, the two pointers will eventually meet.
If there is no loop, the fast pointer will reach the end of the linked list.
Time complexity: O(n), Space complexity: O(1)
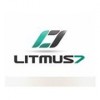
Asked in Litmus7 Systems Consulting

Q. What are the concepts of threads in Java?
Threads in Java allow for concurrent execution of code, enabling multitasking and improving performance.
Threads are lightweight sub-processes within a process.
They allow for concurrent execution of code, enabling multitasking.
Threads share the same memory space, allowing for efficient communication and data sharing.
Examples include creating threads using the Thread class or implementing the Runnable interface.
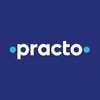
Asked in Practo

Q. What is the space and time complexity, and how can it be improved?
Understanding space and time complexity is crucial for optimizing algorithms in Android development.
Time complexity measures the time an algorithm takes to complete as a function of input size. Example: O(n) for linear search.
Space complexity measures the amount of memory an algorithm uses relative to input size. Example: O(1) for in-place sorting.
Improving time complexity can involve using more efficient algorithms, like switching from O(n^2) bubble sort to O(n log n) quicks...read more
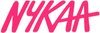
Asked in Nykaa

Q. Why do we prefer the use of ConstraintLayout?
ConstraintLayout is preferred for its flexibility, efficiency, and ease of use in creating complex layouts.
Allows for creating complex layouts with a flat view hierarchy, improving performance
Supports responsive design with constraints that adapt to different screen sizes
Easier to use and understand compared to other layout options like RelativeLayout
Provides tools like Layout Editor in Android Studio for visual editing of layouts
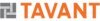
Asked in Tavant Technologies

Q. MVVM and MVP diff, Fragment1 and Fragment2 passing values using ViewModel flow, after navigation, what are the viewmodel process, Unit testing oriented viewmodel, livedata and repositories.
MVVM and MVP are architectural patterns; ViewModels facilitate data sharing between Fragments, enhancing unit testing and data management.
MVVM (Model-View-ViewModel) separates UI logic from business logic, while MVP (Model-View-Presenter) emphasizes a more direct interaction between View and Presenter.
In MVVM, ViewModels hold UI-related data and can be shared between Fragments, allowing for seamless data passing using LiveData.
Example: Fragment1 updates a LiveData in ViewMode...read more
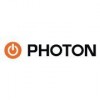
Asked in Photon Technologies

Q. Explain WorkManager and how it is implemented.
Work manager is an Android library that manages background tasks efficiently.
Work manager is part of Android Jetpack and is used for deferrable background tasks.
It provides a way to schedule tasks that need to run even if the app is in the background or not running.
Work manager handles task constraints, retries, and backoff policies automatically.
Tasks can be one-time or periodic, with support for specifying constraints like network availability or charging status.
Example: Wo...read more
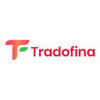
Asked in Tradofina

Q. 5. What is Profiler 6. What is Unit Testing 7. Code Coverage 8. Dependency Injection 9. Advantages of Kotilin
Key concepts for Android development include performance profiling, testing, code quality, dependency management, and Kotlin advantages.
Profiler: Android Profiler is a tool that helps monitor the performance of an app, including CPU, memory, network usage, and energy consumption.
Unit Testing: This involves testing individual components of the application in isolation to ensure they work as intended, using frameworks like JUnit.
Code Coverage: A measure of how much of the code ...read more
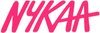
Asked in Nykaa

Q. What are content providers? What are its uses?
Content providers are components in Android that manage access to a structured set of data. They are used to share data between apps.
Content providers allow apps to securely share data with other apps
They provide a standard interface for querying and modifying data
Content providers are often used to access data from databases or files
Examples include the Contacts Provider for accessing contact information and the Media Provider for accessing media files
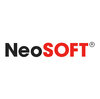
Asked in NeoSOFT

Q. What are coroutines, scope functions, and visibility modifiers?
Coroutines, scope functions, and visibility modifiers are key concepts in Kotlin programming for Android development.
Coroutines are a way to perform asynchronous programming in a sequential manner. They allow for non-blocking operations.
Scope functions are functions that allow you to execute a block of code within the context of an object. Examples include 'let', 'apply', 'run', 'also', and 'with'.
Visibility modifiers control the visibility of classes, interfaces, functions, ...read more
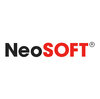
Asked in NeoSOFT

Q. What is the MVVM (Model-View-ViewModel) architectural pattern?
MVVM is an architectural pattern that separates the user interface from the business logic and data handling in Android development.
Model represents the data and business logic of the application.
View is responsible for displaying the UI elements and sending user interactions to the ViewModel.
ViewModel acts as a mediator between the Model and the View, handling the communication and data flow.
MVVM helps in achieving separation of concerns, making code more modular and easier ...read more
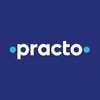
Asked in Practo

Q. Describe how you would design the search page for a video streaming app.
The search page of a video streaming app should allow users to easily search for and discover content.
Include a search bar at the top for users to enter keywords or phrases
Display relevant search results in a grid or list format
Provide filters or sorting options to refine search results
Include thumbnails, titles, and brief descriptions of the search results
Allow users to click on search results to view more details or start streaming
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
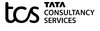
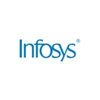
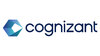
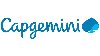
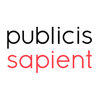
Top Interview Questions for Senior Android Developer Related Skills
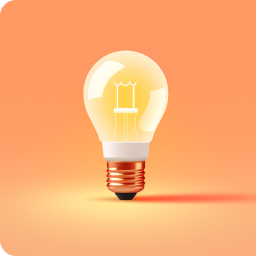
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
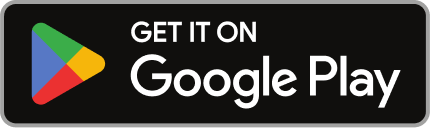
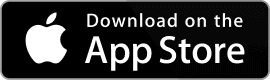
Reviews
Interviews
Salaries
Users
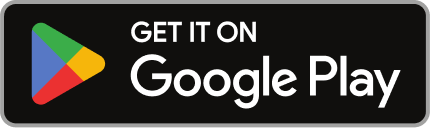
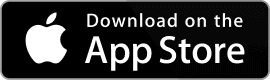