Sdet
100+ Sdet Interview Questions and Answers
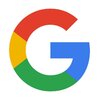
Asked in Google

Q. Given an array of numbers, replace each number with the product of all the numbers in the array except the number itself without using division.
Replace each number in an array with the product of all other numbers without using division.
Iterate through the array and calculate the product of all numbers to the left of the current index.
Then, iterate through the array again and calculate the product of all numbers to the right of the current index.
Multiply the left and right products to get the final product and replace the current index with it.
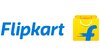
Asked in Flipkart

Q. Write a program to find the angle between the hour and minute hands of a clock, given the time as input. The expected output is the angle in degrees.
Program to find the angle between the hands of a clock given the time.
Calculate the angle of the hour hand from 12 o'clock position
Calculate the angle of the minute hand from 12 o'clock position
Calculate the absolute difference between the two angles
If the difference is greater than 180 degrees, subtract it from 360 degrees
Return the absolute value of the difference as the angle between the hands
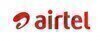
Asked in Bharti Airtel

Q. After clicking the submit button, a blank page appears. How would you debug this issue to find the root cause?
Investigate the blank page issue after clicking the submit button to identify potential causes and solutions.
Check for JavaScript errors in the console that may prevent page rendering.
Verify if the form submission is correctly handled by the backend server.
Inspect network requests to see if the submission returns a valid response.
Ensure that the URL is correctly defined and not leading to a 404 error.
Look for any infinite loops or redirects in the code that could cause a blan...read more
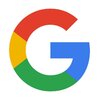
Asked in Google

Q. Write a program to find the depth of a binary search tree without using recursion.
Program to find depth of binary search tree without recursion
Use a stack to keep track of nodes and their depths
Iteratively traverse the tree and update the maximum depth
Return the maximum depth once traversal is complete
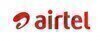
Asked in Bharti Airtel

Q. Write a program to reverse the string. Input: I LOVE JAVA. Output: A VAJE VOLI
This program reverses the order of words in a string while also reversing each word's characters.
Split the input string into words: 'I LOVE JAVA' -> ['I', 'LOVE', 'JAVA']
Reverse each word: 'I' -> 'I', 'LOVE' -> 'EVOL', 'JAVA' -> 'AVAJ'
Reverse the order of words: ['AVAJ', 'EVOL', 'I'] -> 'AVAJ EVOL I'
Final output: 'AVAJ EVOL I'
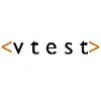
Asked in vTEST

Q. Tell us about a time you faced a disagreement with your team on a testing strategy. How did you handle it and what was the outcome?
Faced a disagreement on testing strategy; facilitated discussion, aligned goals, and reached a consensus that improved our testing process.
Identified the disagreement during a sprint planning meeting regarding automated vs. manual testing.
Facilitated a discussion to understand each team member's perspective and concerns.
Presented data on the efficiency of automated testing for regression scenarios, highlighting time savings.
Proposed a hybrid approach that combined both strate...read more
Sdet Jobs
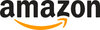
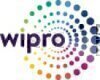
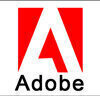
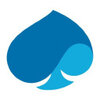
Asked in Capgemini Engineering

Q. Do you use the console to locate elements in a web page?
Yes, I use console to locate elements in a web page for debugging and testing purposes.
Yes, I use console commands like document.querySelector() or document.getElementById() to locate elements on a web page.
Console is helpful for quickly testing and verifying element selectors before implementing them in automated tests.
Using console to locate elements can help in identifying issues with element selection and improve test script efficiency.
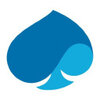
Asked in Capgemini Engineering

Q. Do you use the console to locate elements on a web page?
Yes, I use the console to locate elements in web pages for debugging and testing purposes.
I use the console to inspect elements and identify unique attributes like IDs, classes, or XPath.
I can use commands like document.getElementById(), document.querySelector(), or $() to locate elements.
I also use the console to test CSS selectors and verify if elements are being correctly identified.
Share interview questions and help millions of jobseekers 🌟
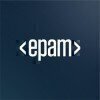
Asked in EPAM Systems

Q. Write an extended method that accepts a locator and timeout. If the method is visible before the timeout, return success; otherwise, return failure.
Create a method to check if element is visible within a specified timeout
Create a method that accepts a locator and timeout as parameters
Use a loop to check if the element is visible within the specified timeout
Return success if the element is visible before timeout, else return failure
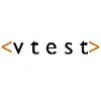
Asked in vTEST

Q. Can you walk us through your approach to testing a [specific product/feature]? How would you prioritize testcases if you had limited time?
I prioritize testing based on risk, user impact, and critical functionality to ensure quality within limited time.
Identify critical functionalities: Focus on features that are essential for the product's core purpose, e.g., login for an e-commerce site.
Assess risk: Evaluate which areas are most likely to fail or have the highest impact on users, such as payment processing.
User impact: Prioritize tests that affect the largest number of users, like UI elements on the homepage.
U...read more
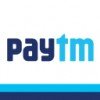
Asked in Paytm

Q. Explain the Jenkins setup process, how to configure a job, and how to update the team with reports.
Jenkins setup involves configuring jobs and updating reports for the team.
Install Jenkins on a server or local machine
Create a new job by selecting 'New Item' and choosing the job type
Configure the job by setting up build triggers, source code management, build steps, and post-build actions
Add plugins for reporting tools like JUnit or HTML Publisher to generate reports
Update the report by viewing the job's build history and clicking on the specific build to see the report
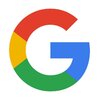
Asked in Google

Q. Create a cache with fast look up that only stores the N most recently accessed items
Create a cache with fast look up that only stores the N most recently accessed items
Implement a hash table with doubly linked list to store the items
Use a counter to keep track of the most recently accessed items
When the cache is full, remove the least recently accessed item
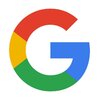
Asked in Google

Q. Describe recursive mergesort and its runtime. Write an iterative version in C++/Java/Python
Recursive mergesort divides array into halves, sorts them and merges them back. O(nlogn) runtime.
Divide array into halves recursively
Sort each half recursively using mergesort
Merge the sorted halves back together
Runtime is O(nlogn)
Iterative version can be written using a stack or queue
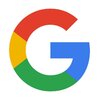
Asked in Google

Q. Given an array of integers heights representing the histogram's bar height where the width of each bar is 1, return the area of the largest rectangle in the histogram. Solve it in linear time.
Find the maximum rectangle (in terms of area) under a histogram in linear time
Use a stack to keep track of the bars in the histogram
For each bar, calculate the area of the rectangle it can form
Pop the bars from the stack until a smaller bar is encountered
Keep track of the maximum area seen so far
Return the maximum area
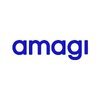
Asked in Amagi Media Labs

Q. How would you automate the API for retrieving possible AWS service IP allocations?
To automate API for retrieving AWS service IP allocations, we can use AWS CLI or SDKs.
Use AWS CLI to retrieve IP allocations for a specific service
Use AWS SDKs to programmatically retrieve IP allocations
Create automated tests to ensure IP allocations are correct
Use tools like Postman to test API endpoints
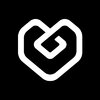
Asked in Fynd

Q. How will you handle/locate dynamic web elements in a web table?
To handle dynamic web elements in a web table, I will use techniques like XPath, CSS selectors, and dynamic element identification.
Use XPath to locate elements based on their attributes or position in the DOM
Utilize CSS selectors to target specific elements based on their styling
Implement dynamic element identification techniques to handle elements that change on page reloads or updates
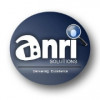
Asked in Anri Solutions HR Services

Q. What is the method to find the second largest number in a given set of data?
To find the second largest number, iterate through the data while tracking the largest and second largest values.
Initialize two variables: 'largest' and 'secondLargest' to negative infinity.
Iterate through each number in the dataset.
If the current number is greater than 'largest', update 'secondLargest' to 'largest' and then update 'largest' to the current number.
If the current number is less than 'largest' but greater than 'secondLargest', update 'secondLargest' to the curre...read more
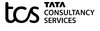
Asked in TCS

Q. What is the difference between an Array and an ArrayList?
Array is a fixed-size data structure while ArrayList is a dynamic-size data structure in Java.
Array is a static data structure with a fixed size, while ArrayList is a dynamic data structure that can grow or shrink in size.
Arrays can hold primitive data types and objects, while ArrayList can only hold objects.
Arrays require a specified size during initialization, while ArrayList can dynamically resize itself.
Arrays use square brackets [] for declaration, while ArrayList is a p...read more
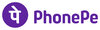
Asked in PhonePe

Q. What approach would you take to design an automation framework?
Approach for designing an automation framework
Identify the scope and requirements of the framework
Choose a suitable programming language and tools
Design the framework architecture and modules
Implement the framework and write test scripts
Integrate the framework with CI/CD pipeline
Continuously maintain and update the framework
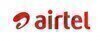
Asked in Bharti Airtel

Q. Write a program to count the occurrences of each character in a string.
A program to count the characters in a string
Iterate through each character in the string and increment the count for each character
Use a hashmap to store the count of each character
Handle edge cases like empty string or null input
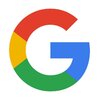
Asked in Google

Q. How would you efficiently implement three stacks using a single array?
Implement 3 stacks in a single array efficiently
Divide the array into 3 equal parts
Use pointers to keep track of top of each stack
Implement push and pop operations for each stack
Handle stack overflow and underflow cases
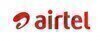
Asked in Bharti Airtel

Q. Write Selenium code to automate the 'Add to Cart' feature on Amazon.
Use Selenium to automate adding items to cart on Amazon website.
Locate the 'Add to Cart' button on the product page using Selenium's findElement method
Click on the 'Add to Cart' button to add the item to the cart
Verify that the item has been successfully added to the cart by checking the cart contents
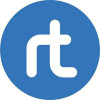
Asked in rtCamp Solutions

Q. What is the difference between Git and GitHub?
Git is a version control system used for tracking changes in code, while GitHub is a platform for hosting Git repositories and collaborating on code.
Git is a distributed version control system that allows developers to track changes in code and collaborate with others.
GitHub is a web-based platform that provides hosting for Git repositories, allowing developers to store, share, and collaborate on code.
Git can be used locally on a developer's machine, while GitHub is a cloud-b...read more
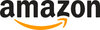
Asked in Amazon

Q. Implement a stack using two queues.
Create a stack using 2 given queues.
Push elements into one queue until it is full.
When the first queue is full, push new elements into the second queue.
To pop an element, remove all elements from the non-empty queue except the last one.
Switch the non-empty queue to the other one when it becomes empty.
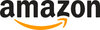
Asked in Amazon

Q. What current technologies are you working with?
I am currently working with Java and Selenium for test automation.
Using Java programming language for writing test scripts
Using Selenium WebDriver for automating web applications
Integrating with CI/CD tools like Jenkins for continuous testing
Working with frameworks like TestNG for test management
Using tools like Maven for project management
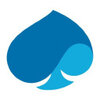
Asked in Capgemini Engineering

Q. How do you automate email and one-time passcode testing?
Automating email and one time passcode involves using automation tools and scripting to send emails and generate passcodes.
Use automation tools like Selenium or Puppeteer to automate the process of logging into email accounts and sending emails.
Utilize scripting languages like Python or JavaScript to generate one time passcodes and send them via email.
Implement API calls to interact with email servers and send emails programmatically.
Consider using third-party services like T...read more
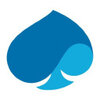
Asked in Capgemini Engineering

Q. How do you compare two databases and two files?
Comparing two databases and two files involves analyzing their structure, content, and integrity.
Compare the schema of the databases to ensure they have the same tables, columns, and relationships.
Check the data in the databases and files to identify any discrepancies or missing information.
Use checksums or hash functions to compare the contents of the files for differences.
Consider using tools like SQL queries, file comparison software, or scripting languages for efficient c...read more
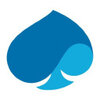
Asked in Capgemini Engineering

Q. How do you exit recursion in programming?
To exit recursion in programming, a base case is defined to stop the recursive calls.
Define a base case that will stop the recursive calls.
Ensure that the base case is reached during the recursion.
Return a value or perform an action when the base case is met.
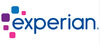
Asked in Experian

Q. How would you integrate a Selenium framework with Jenkins?
Integrating Selenium framework with Jenkins involves setting up Jenkins job to run Selenium tests.
Install Jenkins on the server
Install necessary plugins like Selenium plugin
Create a Jenkins job for running Selenium tests
Configure the job to execute Selenium tests using the Selenium framework
Set up build triggers and post-build actions as needed

Asked in PayU Payments

Q. WHAT IS OOPS, INHERITANCE, STATIC DYNAMIC BINDING
OOPS is Object-Oriented Programming, Inheritance is the ability of a class to inherit properties and behavior from another class, Static Binding is resolved at compile time, Dynamic Binding is resolved at runtime.
OOPS stands for Object-Oriented Programming, which is a programming paradigm based on the concept of objects.
Inheritance is a feature in OOP that allows a class to inherit properties and behavior from another class.
Static Binding is also known as early binding, where...read more
Interview Experiences of Popular Companies
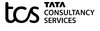
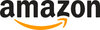
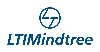
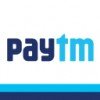
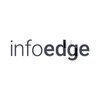
Top Interview Questions for Sdet Related Skills
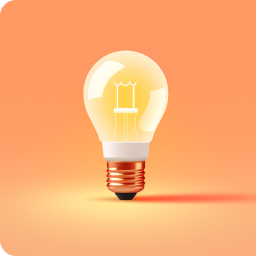
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
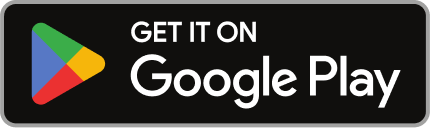
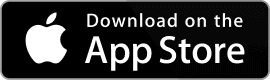
Reviews
Interviews
Salaries
Users
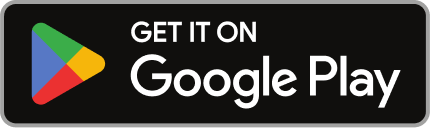
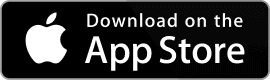