Mern Full Stack Developer
100+ Mern Full Stack Developer Interview Questions and Answers
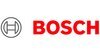
Asked in Bosch

Q. What is the best way to save large files (e.g., photos) into a MongoDB database?
Use GridFS to save large files in MongoDB database.
GridFS is a specification for storing and retrieving large files in MongoDB.
It divides the file into smaller chunks and stores them as separate documents.
GridFS provides a way to query and retrieve the file in chunks or as a whole.
To save a large file, use the GridFS API provided by the MongoDB driver.
Example: db.fs.files.insert({filename: 'photo.jpg', contentType: 'image/jpeg'})
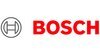
Asked in Bosch

Q. What are the request, response, and next parameters in an Express function?
Request, response and next are parameters in Express function for handling HTTP requests and responses.
Request object represents the HTTP request and contains properties for the request query string, parameters, body, HTTP headers, etc.
Response object represents the HTTP response that an Express app sends when it receives an HTTP request.
Next function is a middleware function that passes control to the next middleware function in the stack.
Request, response and next are commo...read more
Mern Full Stack Developer Interview Questions and Answers for Freshers
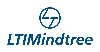
Asked in LTIMindtree

Q. Write a program to count the number of vowels in a string.
Program to count the number of vowels in a string.
Loop through each character in the string and check if it is a vowel.
Use a counter to keep track of the number of vowels found.
Consider both uppercase and lowercase vowels.
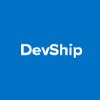
Asked in Devship

Q. Have you worked on any file upload tasks previously? Explain the process and how files are saved in the database and the retrieval process.
Yes, I have experience with file upload tasks. Files are saved in DB by storing the file path and retrieval is done by fetching the file path from the DB.
Used multer middleware in Node.js to handle file uploads
Saved file path in MongoDB using Mongoose schema
Retrieved file by querying the database for the file path
Implemented file upload functionality in a project using React for the front end
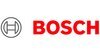
Asked in Bosch

Q. What is the difference between microservice and monolithic architecture?
Microservices are small, independent services that communicate with each other, while monolithic architecture is a single, unified system.
Microservices are loosely coupled and can be developed and deployed independently
Monolithic architecture is tightly coupled and requires the entire system to be deployed together
Microservices allow for better scalability and fault tolerance
Monolithic architecture is simpler to develop and test
Examples of microservices include Netflix, Amazo...read more
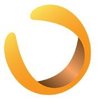
Asked in Stark Digital Media Services

Q. If a client reports receiving a 500 error, how would you approach diagnosing and resolving the issue?
Diagnosing a 500 error involves checking server logs, reviewing code, and testing API endpoints to identify the root cause.
Check Server Logs: Look for error messages in server logs (e.g., Node.js logs) to pinpoint the issue, such as uncaught exceptions.
Review Recent Changes: Identify any recent code changes or deployments that might have introduced the error, such as new middleware or routes.
Test API Endpoints: Use tools like Postman to test API endpoints directly and see if ...read more
Mern Full Stack Developer Jobs
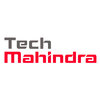
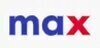
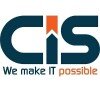
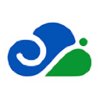
Asked in Enovate IT Outsourcing

Q. Create a React table with a button. When clicked, the button should route to another page, trigger an API call, and display the data in a tabular format using React.js.
Create a react table with a button to route to another page, trigger an API, and display data in tabular format.
Create a React component for the table
Add a button to the table rows
Implement routing using React Router
Handle button click event to navigate to another page
On the new page, trigger an API call using Axios or Fetch
Retrieve the data from the API response
Display the data in tabular format using HTML table or a UI library like Material-UI
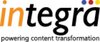
Asked in Integra Software Services

Q. What is the execution order of setImmediate, setInterval, console.log, and process.nextTick in Node.js?
In Node.js, the execution order of setImmediate, setInterval, console.log, and process.nextTick follows the event loop phases.
process.nextTick: Executes immediately after the current operation completes, before any I/O tasks.
console.log: Runs synchronously in the current phase of the event loop, outputting to the console.
setImmediate: Executes after the current event loop cycle, allowing I/O operations to complete first.
setInterval: Scheduled to run at specified intervals, bu...read more
Share interview questions and help millions of jobseekers 🌟
Asked in Magic Software Enterprizes

Q. What Object-Oriented Programming (OOP) paradigms are you familiar with, and could you provide a code snippet that demonstrates one of them?
I am familiar with OOP paradigms like encapsulation, inheritance, and polymorphism. Here's a code snippet demonstrating encapsulation.
Encapsulation: Bundling data and methods that operate on the data within one unit (class).
Inheritance: Creating new classes based on existing ones to promote code reuse.
Polymorphism: Allowing methods to do different things based on the object it is acting upon.
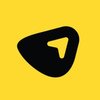
Asked in Uplers

Q. Can you outline a simple strategy for fallback error handling in a Node.js application using async/await?
Use try-catch blocks to handle errors in async/await functions in Node.js applications.
Wrap the async function in a try block and catch any errors using a catch block.
Use the throw keyword to throw custom errors within the async function.
Handle promise rejections using the catch block of the async/await function.
Use the finally block to execute cleanup code regardless of whether an error occurred or not.
Asked in Lakhera Global Services

Q. What is the event loop, how does it work, and can you provide a practical example?
Event loop is a mechanism in JavaScript that allows for asynchronous operations to be executed in a non-blocking way.
Event loop continuously checks the call stack for any functions that need to be executed.
If the call stack is empty, the event loop checks the callback queue for any functions that are waiting to be executed.
The event loop then moves functions from the callback queue to the call stack for execution.
Practical example: setTimeout function in JavaScript, where the...read more
Asked in Bonami Software

Q. Write a program to find the sum and average of the digits that appear in the string, ignoring all other characters. For example, given the string 'PYnative29@#8496'.
Program to find sum and average of digits in a string, ignoring other characters.
Iterate through each character in the string and check if it is a digit.
If it is a digit, convert it to an integer and add it to the sum.
Keep track of the count of digits to calculate the average at the end.
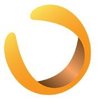
Asked in Stark Digital Media Services

Q. How do you stay informed about the latest trends and technologies in web development?
I stay updated on web development trends through various resources, including blogs, podcasts, and online communities.
Follow Industry Blogs: Websites like Smashing Magazine and CSS-Tricks provide insights into the latest web technologies and best practices.
Listen to Podcasts: Shows like 'JavaScript Jabber' and 'ShopTalk Show' discuss current trends and tools in web development.
Participate in Online Communities: Engaging in forums like Stack Overflow and Reddit helps me learn ...read more
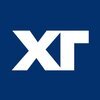
Asked in Xicom Technologies

Q. What is react how it is different from html and angular
React is a JavaScript library for building user interfaces, while HTML is a markup language and Angular is a front-end framework.
React is a JavaScript library for building interactive user interfaces.
HTML is a markup language used for creating the structure of web pages.
Angular is a front-end framework that provides a more structured approach to building web applications.
React uses a virtual DOM for faster rendering, while Angular uses two-way data binding.
React components ar...read more
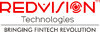
Asked in REDVision Global Technologies

Q. Node is single-threaded, then how do you achieve asynchronous behavior?
Node.js achieves asynchronous behavior through event-driven architecture and non-blocking I/O operations.
Node.js uses event-driven architecture to handle asynchronous operations.
It utilizes non-blocking I/O operations to allow multiple operations to be executed concurrently.
Node.js uses callbacks, promises, and async/await to manage asynchronous code execution.
Example: Using callbacks in Node.js to handle asynchronous file operations.
Asked in Magic Software Enterprizes

Q. What is the difference between an interface and a type in programming?
Interfaces define contracts for classes, while types are more flexible and can represent various structures in programming.
Interfaces can only describe object shapes, while types can describe primitive types, unions, and intersections.
Example of an interface: `interface User { name: string; age: number; }`.
Example of a type: `type ID = string | number;` allows for multiple types.
Interfaces support declaration merging, meaning you can define the same interface multiple times a...read more
Asked in Magic Software Enterprizes

Q. What is the method to find the second largest salary in a database?
To find the second largest salary, use SQL queries or programming logic to filter and sort salary data.
Use SQL: SELECT DISTINCT salary FROM employees ORDER BY salary DESC LIMIT 1 OFFSET 1;
Use subquery: SELECT MAX(salary) FROM employees WHERE salary < (SELECT MAX(salary) FROM employees);
In programming: Sort the salary array and access the second last element.
Asked in Magic Software Enterprizes

Q. What occurs behind the scenes when a web search is conducted?
A web search involves indexing, querying, and retrieving relevant results from vast databases.
1. User Input: The process starts when a user enters a query into a search engine.
2. Query Processing: The search engine processes the query to understand intent and context.
3. Indexing: Search engines maintain an index of web pages, which is a structured database of content.
4. Ranking Algorithms: Algorithms evaluate and rank pages based on relevance, authority, and other factors.
5. ...read more
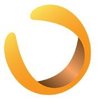
Asked in Stark Digital Media Services

Q. What testing libraries did you use in frontend and backend applications?
I utilize various testing libraries for both frontend and backend to ensure code quality and functionality.
Frontend: I commonly use Jest for unit testing React components.
Frontend: React Testing Library helps in testing component behavior and user interactions.
Backend: For Node.js, I use Mocha and Chai for unit and integration testing.
Backend: Supertest is useful for testing HTTP endpoints in Express applications.

Asked in Vue Data Technologies

Q. Find the last non-repeating character from the string "Hello".
Find the last non-repetitive character in a given string
Iterate through the string from right to left
Check if the character is not repeated before it
Return the first non-repeated character found
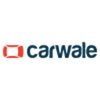
Asked in Carwale

Q. Given an array of integers, find the next greater element for each element in the array.
Use stack to find next greater element for each element in the array
Create an empty stack to store indices of elements
Iterate through the array and for each element, pop elements from stack until finding a greater element
Store the next greater element in a result array
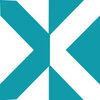
Asked in Excellence Technologies

Q. Write a program to find the second largest number in an array without using built-in methods like sort, array, or max.
This program finds the second largest number in an array without using built-in methods.
Initialize two variables, 'first' and 'second', to hold the largest and second largest values.
Iterate through the array and update 'first' and 'second' accordingly.
If a number is greater than 'first', update 'second' to 'first' and then update 'first' to the new number.
If a number is less than 'first' but greater than 'second', update 'second' to this number.
Example: For the array [3, 5, 1...read more
Asked in Lakhera Global Services

Q. What are asynchronous and synchronous operations? Provide a practical example.
Asynchronous and synchronous refer to different ways of executing code. Asynchronous allows multiple tasks to be executed simultaneously, while synchronous executes tasks one after the other.
Synchronous code executes tasks sequentially, waiting for each task to finish before moving on to the next one.
Asynchronous code allows tasks to be executed concurrently, without waiting for each task to finish before starting the next one.
Example: Synchronous code would be like waiting f...read more
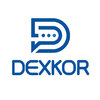
Asked in DexKor

Q. Can you explain what you know about DexKor's products and services?
DexKor offers innovative solutions in the healthcare sector, focusing on diagnostic tools and data management.
DexKor specializes in advanced diagnostic products for healthcare providers.
Their services include data management solutions that enhance patient care.
Examples of products include lab testing kits and software for patient data analysis.
They aim to improve efficiency and accuracy in medical diagnostics.
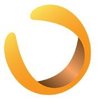
Asked in Stark Digital Media Services

Q. How do you manage re-rendering in application workflows?
Managing re-rendering involves optimizing component updates and state management in React applications.
Use React's built-in hooks like useMemo and useCallback to memoize values and functions, preventing unnecessary re-renders.
Implement shouldComponentUpdate or React.memo for class components and functional components respectively to control rendering.
Leverage state management libraries like Redux or Context API to manage global state, reducing prop drilling and re-renders.
Spl...read more
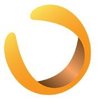
Asked in Stark Digital Media Services

Q. How do you optimize the performance of a web application?
Optimizing web application performance involves techniques to enhance speed, responsiveness, and overall user experience.
Minification and Compression: Use tools like UglifyJS and Gzip to reduce file sizes of CSS and JavaScript, improving load times.
Lazy Loading: Implement lazy loading for images and components to load only what is necessary, reducing initial load time.
Code Splitting: Use dynamic imports in React to split code into smaller chunks, loading only the required cod...read more
Asked in BlueWebSpark

Q. What is the process and structure for building a scalable and robust application using the MERN stack?
Building a scalable MERN stack application involves structured architecture, efficient state management, and robust API design.
Use a modular architecture: Break down the application into reusable components (e.g., separate folders for models, routes, controllers).
Implement state management: Use Redux or Context API for managing global state in React applications.
Design RESTful APIs: Ensure your Express.js APIs follow REST principles for better scalability and maintainability....read more
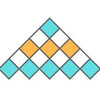
Asked in InterviewBit

Q. What are the different sorting techniques available in JavaScript?
Some of the sorting techniques available in JavaScript include bubble sort, insertion sort, selection sort, merge sort, and quick sort.
Bubble sort: repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
Insertion sort: builds the final sorted array one item at a time by inserting each element into its correct position.
Selection sort: repeatedly finds the minimum element from the unsorted part of the array and swaps it with...read more
Asked in Optimal Virtual Employee

Q. new es6 features, difference between regular and arrow functions, difference between promises and async/await, implementation of time-complexity in javascript context, et.
ES6 features, arrow functions, promises vs async/await, time complexity in JavaScript.
ES6 features include arrow functions, template literals, destructuring, spread/rest operators, etc.
Arrow functions have a more concise syntax and do not bind their own 'this' value.
Promises are objects representing the eventual completion or failure of an asynchronous operation.
Async/await is a syntactic sugar for working with promises, making asynchronous code look synchronous.
Time complexi...read more
Asked in Mypcot Infotech

Q. Given an array of strings, how would you remove the numbers from each string?
Remove numbers from strings in an array, returning only the alphabetic characters.
Use a regular expression to identify and remove digits. Example: 'abc123' becomes 'abc'.
Iterate through the array of strings and apply the regex to each string.
Return a new array containing the cleaned strings without numbers. Example: ['hello1', 'world2'] becomes ['hello', 'world'].
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
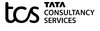
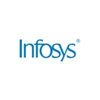
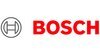
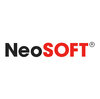
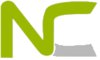
Top Interview Questions for Mern Full Stack Developer Related Skills
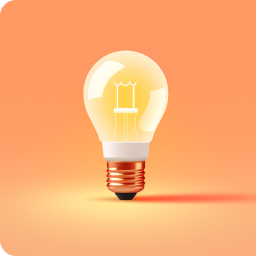
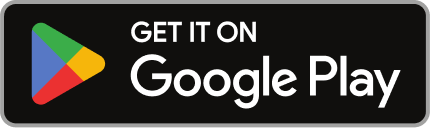
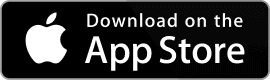
Reviews
Interviews
Salaries
Users
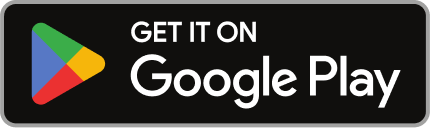
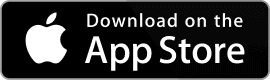