Java Developer
2000+ Java Developer Interview Questions and Answers
Asked in IQ Accencis

Packages in Java help organize code, prevent naming conflicts, and provide access control.
Organize code into logical groups for easier maintenance and readability
Prevent naming conflicts by using unique package names
Provide access control by using access modifiers like public, private, protected, and default
Facilitate reusability by allowing classes to be easily imported and used in other packages
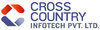
Asked in Cross Country Infotech

Q. Do you have any Java certifications?
No, I don't have any Java certificate.
No, I don't possess any Java certification.
Unfortunately, I haven't obtained any Java certificate.
Regrettably, I do not hold any Java certification.
Asked in Systech ERP

Q. 1.why we go with oops concept? 2.what is polymorphism? 3.what is JVM? 4.what is the Database connection coding in JAVA? 5.what is JOIN(QUERY)? 6.what is TRIGGER? 7.what is PROCEDURE? 8.what is Hashmap? 9.what i...
read moreBasic Java and Query questions for Java Developer position.
OOPs concept helps in creating modular, reusable and maintainable code.
Polymorphism is the ability of an object to take on many forms.
JVM stands for Java Virtual Machine, which executes Java bytecode.
Database connection coding in Java involves creating a connection object, setting connection properties, and executing SQL queries.
JOIN is used to combine rows from two or more tables based on a related column between the...read more
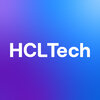
Asked in HCLTech

Q. 1)Compare two string using Java 8 features without comparator and comparable 2) Given an array to print non duplicate in the array 3) Solid principles 4) Stereo type annotations 5) how to make list immutable 6)...
read moreThe interview questions cover a range of topics related to Java development, including Java 8 features, data structures, annotations, and database triggers.
Use Java 8 features like streams and lambda expressions to compare two strings without using comparator or comparable.
To print non-duplicate elements in an array, use a HashSet to store unique elements and then iterate through the array to check for duplicates.
Solid principles refer to a set of design principles for object...read more
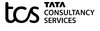
Asked in TCS

Q. Where did you implement Executor service in your project?
Implemented Executor service in the backend for parallel processing.
Implemented ExecutorService in the backend to handle multiple requests concurrently.
Used ExecutorService to execute multiple tasks in parallel.
Implemented ExecutorService to improve the performance of the application.
Used ExecutorService to manage thread pools and execute tasks asynchronously.
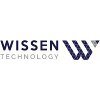
Asked in Wissen Technology

Q. Write a Java program using multithreading to print the following output for n=10: Thread-1 : 1 Thread-2 : 2 Thread-3 : 3 Thread-1 : 4 Thread-2 : 5 Thread-3 : 6 Thread-1 : 7 Thread-2 : 8 Thread-3 : 9 Thread-1 :...
read moreA Java program using multithreading to print numbers from 1 to 10 in a specific pattern.
Create three threads and assign them to print numbers in a specific pattern
Use synchronization to ensure the correct order of printing
Use a loop to iterate from 1 to 10 and assign the numbers to the threads
Print the thread name and the assigned number in the desired format
Java Developer Jobs
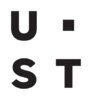
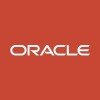
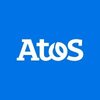
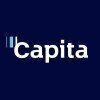
Asked in Capita

Some standard Java pre-defined functional interfaces include Function, Consumer, Predicate, and Supplier.
Function: Represents a function that accepts one argument and produces a result. Example: Function<Integer, String>
Consumer: Represents an operation that accepts a single input argument and returns no result. Example: Consumer<String>
Predicate: Represents a predicate (boolean-valued function) of one argument. Example: Predicate<Integer>
Supplier: Represents a supplier of re...read more
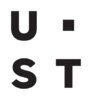
Asked in UST

Spring Boot offers annotations like @SpringBootApplication, @RestController, @Autowired, @Component, @RequestMapping, etc.
@SpringBootApplication - Used to mark the main class of a Spring Boot application.
@RestController - Used to define a class as a controller in a RESTful web service.
@Autowired - Used for automatic dependency injection.
@Component - Used to indicate that a class is a Spring component.
@RequestMapping - Used to map web requests to specific handler methods.
Share interview questions and help millions of jobseekers 🌟
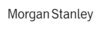
Asked in Morgan Stanley

Overriding equals and hashCode methods in Java is important for proper object comparison and hashing.
Equals method is used to compare two objects for equality, while hashCode method is used to generate a unique integer value for an object.
By overriding equals and hashCode methods, we can ensure that objects are compared based on their actual content rather than memory address.
This is crucial for collections like HashMap and HashSet, where proper implementation of equals and h...read more
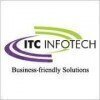
Asked in ITC Infotech

Q. How do you change the background color of a div element?
Use CSS to change the background color of a div element.
Use the 'background-color' property in CSS to specify the color.
You can use color names, hex codes, RGB values, or HSL values to set the background color.
Example: div { background-color: blue; }
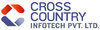
Asked in Cross Country Infotech

Q. When is memory allocated: when a class is created, when an instance is created, or at another time?
Memory is allocated when an instance of a class is created.
Memory is allocated dynamically when an object is instantiated.
Each instance of a class has its own memory allocation.
Memory allocation occurs at runtime, not during class creation.
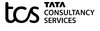
Asked in TCS

Q. What is daemon thread? How it help in multithreading?
Daemon thread is a low priority thread that runs in the background and provides services to other threads.
Daemon threads are used for tasks that don't require user interaction or input.
They are automatically terminated when all non-daemon threads have completed.
Examples include garbage collection, logging, and monitoring.
They can be created using setDaemon() method.
Daemon threads should not be used for tasks that require data consistency or integrity.
They can help in improvin...read more
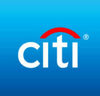
Asked in Citicorp

Q. 1) How do you achieve concurrency in your current job, and write code for it? 2) Write code for Rest API using Springboot, need to write Entity, Service, Repository, and Controller classes? 3)Volatile keywords,...
read moreAnswers to common Java Developer interview questions
1) Concurrency is achieved using multithreading in Java. Use threads, executors, or synchronized blocks. Example: using ExecutorService to run multiple tasks concurrently.
2) Rest API in Springboot: Entity class defines database table, Service class contains business logic, Repository class interacts with database, Controller class handles HTTP requests.
3) Volatile keyword provides visibility guarantee but does not support sy...read more
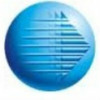
Asked in Protechsoft Technologies

Q. Write a program for different types of books (Paperback, E-book, Audiobook) using Inheritance Concept.
Program for different types of books using Inheritance Concept
Create a Book class as the parent class
Create Paperbook, E-book, and Audiobook classes as child classes
Inherit properties and methods from the Book class
Add unique properties and methods to each child class
Example: Paperbook class can have a property for number of pages
Example: E-book class can have a property for file format
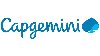
Asked in Capgemini

Java 8 introduced several new features including lambda expressions, functional interfaces, streams, and the new Date and Time API.
Lambda expressions: Allow you to treat functionality as a method argument.
Functional interfaces: Interfaces with a single abstract method, used for lambda expressions.
Streams: Provide a new abstraction to work with sequences of elements.
Date and Time API: Improved API for handling date and time operations.
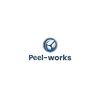
Asked in Peel-works

Q. What is the difference between a local variable, a static variable, and an instance variable?
Local, static, and instance variables differ in their scope and lifetime.
Local variables are declared inside a method and have a limited scope.
Static variables belong to the class and are shared among all instances.
Instance variables are unique to each object and can be accessed using the object reference.
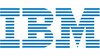
Asked in IBM

Q. How do you make a class immutable?
To make a class immutable, use final keyword, make all fields private, provide no setter methods, and ensure deep copy of mutable fields.
Use final keyword to prevent subclassing
Make all fields private to restrict direct access
Provide no setter methods to prevent modification
Ensure deep copy of mutable fields to avoid sharing references
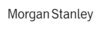
Asked in Morgan Stanley

Synchronized Collection is thread-safe but may have performance issues, while Concurrent Collection is optimized for concurrent access.
Synchronized Collection uses synchronized keyword to achieve thread-safety, while Concurrent Collection uses non-blocking algorithms like CAS (Compare and Swap).
Synchronized Collection locks the entire collection during modification, leading to potential performance bottlenecks, while Concurrent Collection allows multiple threads to access and...read more
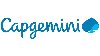
Asked in Capgemini

Q. What are the internal partitions of the Java Virtual Machine?
Internal partitions of Java Virtual Machine
Java Virtual Machine has three internal partitions: Heap, Stack, and Method Area
Heap is used for dynamic memory allocation of objects and arrays
Stack is used for storing method frames and local variables
Method Area is used for storing class-level data such as method code and static variables
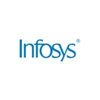
Asked in Infosys

A View is a complete page while a Partial View is a reusable component in MVC.
A View represents a complete page in MVC which can include layout, content, and other views.
A Partial View is a reusable component that can be rendered within a View or another Partial View.
Partial Views are useful for creating modular and reusable components in MVC applications.
Example: A View may contain the overall layout of a website, while a Partial View may represent a sidebar or a navigation ...read more
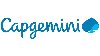
Asked in Capgemini

Q. Why are Java Strings immutable?
Java Strings are immutable to ensure data integrity and security.
Immutable strings prevent accidental modification of data.
String pooling optimizes memory usage by reusing existing strings.
Immutable strings are thread-safe, simplifying concurrent programming.
String immutability allows for efficient caching and hashing.
Immutable strings enable safe sharing of string references.
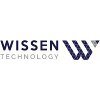
Asked in Wissen Technology

Q. How to create Thread in Java and What are the ways?
Threads in Java allow concurrent execution of multiple tasks. They can be created in multiple ways.
Using the Thread class
Implementing the Runnable interface
Using the Executor framework
Using the Callable interface with ExecutorService
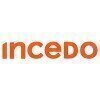
Asked in Incedo

Q. What is the difference between HashMap and Hashtable?
Hashtable is synchronized and does not allow null keys or values, while HashMap is not synchronized and allows null keys and values.
Hashtable is thread-safe, while HashMap is not.
Hashtable does not allow null keys or values, while HashMap allows null keys and values.
Hashtable is slower than HashMap due to synchronization.
Hashtable is a legacy class, while HashMap is part of the Java Collections Framework.
Hashtable is recommended to be used in multi-threaded environments, whil...read more
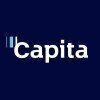
Asked in Capita

Session interface in Hibernate is used to create, read, update, and delete persistent objects.
Session interface is used to interact with the database in Hibernate.
It represents a single-threaded unit of work.
It provides methods for CRUD operations like save, update, delete, and get.
Session is created using SessionFactory object.
Example: Session session = sessionFactory.openSession();
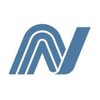
Asked in Netcracker Technology

Comparable is an interface used for natural ordering, while Comparator is an interface used for custom ordering in Java.
Comparable interface is used to define the natural ordering of objects. It is implemented by the class whose objects are to be compared.
Comparator interface is used to define custom ordering of objects. It is implemented by a separate class.
Comparable interface has a single method, compareTo(), which compares the current object with another object.
Comparator...read more
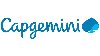
Asked in Capgemini

Q. Explain public static void main(String args[]) in Java.
The public static void main method is the entry point of a Java program.
The main method is declared with the public access modifier, allowing it to be accessed from anywhere.
The static keyword allows the main method to be called without creating an instance of the class.
The void keyword indicates that the main method does not return any value.
The main method takes an array of strings (args[]) as a parameter, which can be used to pass command-line arguments to the program.
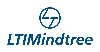
Asked in LTIMindtree

Static is used to define class-level variables and methods, while final is used to define constants that cannot be changed.
Static variables belong to the class itself, while final variables are constants that cannot be modified.
Static methods can be called without creating an instance of the class, while final methods cannot be overridden.
Static keyword is used for memory management, while final keyword is used for defining constants.
Example: static int count = 0; final doubl...read more

Asked in CitiusTech

Azure and AWS are two major cloud service providers offering similar services but with some differences in features and pricing.
AWS is the oldest and most widely used cloud service provider, while Azure is catching up quickly.
Azure is known for its strong integration with Microsoft products and services, while AWS has a wider range of third-party integrations.
AWS has a larger global presence with more data centers worldwide compared to Azure.
Pricing models differ between the ...read more
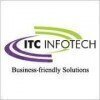
Asked in ITC Infotech

Q. What is a session in Java?
Session in Java is a way to store information about a user across multiple requests.
Session is used to maintain stateful information about a user.
It is created when a user first accesses a web application and remains active until the user logs out or the session times out.
Session data is stored on the server and can be accessed by multiple requests from the same user.
It is commonly used for user authentication, shopping carts, and personalization.
The HttpSession interface in ...read more
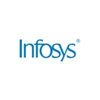
Asked in Infosys

Q. What is java and what is inheritance and what is oops concepts and what is method
Java is an object-oriented programming language. Inheritance is a mechanism to create new classes based on existing ones. OOPs is a programming paradigm. Method is a block of code that performs a specific task.
Java is a high-level, class-based, and object-oriented programming language.
Inheritance is a mechanism in which one class acquires the properties and behaviors of another class.
OOPs is a programming paradigm that focuses on objects and their interactions.
Method is a blo...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
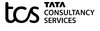
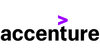
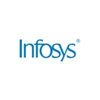
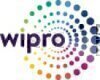
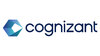
Top Interview Questions for Java Developer Related Skills
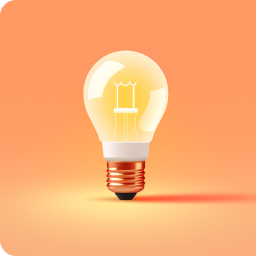
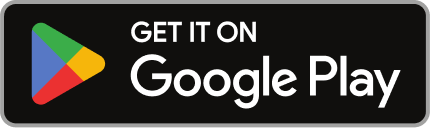
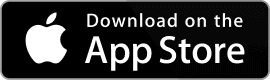
Reviews
Interviews
Salaries
Users
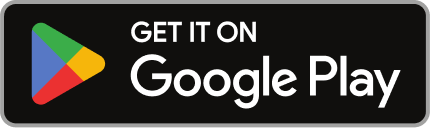
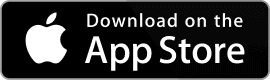