Java Developer
300+ Java Developer Interview Questions and Answers for Freshers
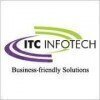
Asked in ITC Infotech

Q. What is the Java Class Library?
Java Class Library is a collection of pre-compiled classes and methods that provide ready-to-use functionality for Java developers.
Java Class Library contains classes for common tasks like input/output, networking, database access, etc.
Developers can use these classes to save time and effort by reusing existing code.
Examples include java.lang, java.util, java.io, java.net, etc.
Asked in Jforce Solutions

Q. What are the tasks involved in creating a User Interface (UI) for a CRUD (Create, Read, Update, Delete) application?
Tasks involved in creating a UI for a CRUD application
Designing the layout and structure of the UI
Implementing input forms for creating and updating data
Displaying data in a readable format for viewing and reading
Adding functionality for updating and deleting data
Implementing validation for user input to ensure data integrity
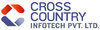
Asked in Cross Country Infotech

Q. What is a queue in programming?
A queue is a data structure that follows the FIFO (First In First Out) principle.
Elements are added to the back of the queue and removed from the front.
Common operations include enqueue (add to back) and dequeue (remove from front).
Examples include a line of people waiting for a movie or a printer queue.
Java provides the Queue interface and its implementations like LinkedList and PriorityQueue.
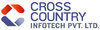
Asked in Cross Country Infotech

Q. What is a stack in programming?
A stack is a data structure that follows the Last In First Out (LIFO) principle.
Elements are added and removed from the top of the stack.
Push() adds an element to the top of the stack.
Pop() removes the top element from the stack.
Peek() returns the top element without removing it.
Used in programming for function calls, expression evaluation, and memory management.
Asked in Asite Solutions

Q. How do you connect a database with Java?
Java provides JDBC API to connect with databases.
Load the JDBC driver class
Create a connection object
Create a statement object
Execute the query
Process the result set
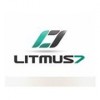
Asked in Litmus7 Systems Consulting

Q. Given two integer arrays, find consecutive sub-arrays and return the sub-array which has a sum greater than 6.
Find consecutive subarrays in two integer arrays with a sum greater than 6.
Iterate through each array to find all possible subarrays.
Calculate the sum of each subarray.
Check if the sum is greater than 6.
Return the subarrays that meet the criteria.
Example: For array [1, 2, 3, 4], subarrays like [2, 3, 4] have a sum of 9.
Java Developer Jobs
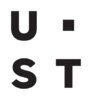
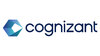
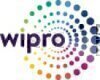
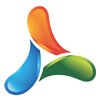
Asked in Allied Globetech

Q. What are the differences between cryptography and encryption, and which one is superior in certain contexts?
Cryptography encompasses encryption and other techniques for secure communication, while encryption specifically refers to data transformation.
Cryptography is a broader field that includes techniques like hashing, digital signatures, and key exchange.
Encryption is a specific method within cryptography that transforms data to prevent unauthorized access.
Example of encryption: AES (Advanced Encryption Standard) is widely used to secure data.
Example of cryptography: Public key i...read more
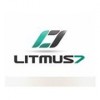
Asked in Litmus7 Systems Consulting

Q. Write a program with Java 8 to filter the employees based on salary greater than 10,000 from a list of Employee objects.
Java 8 program to filter employees with a salary greater than 10,000 using streams.
Create an Employee class with fields like name and salary.
Use a List<Employee> to store employee objects.
Utilize Java 8 Streams to filter employees based on salary.
Example: employees.stream().filter(e -> e.getSalary() > 10000).collect(Collectors.toList());
Print the filtered list of employees.
Share interview questions and help millions of jobseekers 🌟
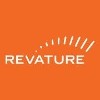
Asked in Revature

Q. What is linked lists,what is multiple inheritance,what is compiler and interpreter and where they both are used
Linked lists are data structures where each element points to the next element. Multiple inheritance is when a class inherits from more than one parent class. A compiler translates source code into machine code, while an interpreter executes code line by line.
Linked lists are used to store data in a linear sequence, with each element pointing to the next one.
Multiple inheritance allows a class to inherit attributes and methods from more than one parent class.
A compiler transl...read more
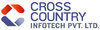
Asked in Cross Country Infotech

Q. Give me a real-life example of polymorphism.
Polymorphism is the ability of an object to take on many forms. A real-life example is a vehicle, which can be a car, a bike, or a truck.
Polymorphism allows objects of different classes to be treated as objects of a common superclass.
In the example of a vehicle, different types of vehicles can have common methods like start(), stop(), and accelerate().
Polymorphism helps in achieving code reusability and flexibility in object-oriented programming.
Another example is the use of ...read more
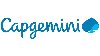
Asked in Capgemini

Q. How many teams are involved in the spiral model?
The number of teams involved in spiral model varies based on the project requirements.
Spiral model is a flexible model that allows for multiple teams to work on different phases simultaneously.
The number of teams involved can range from one to many depending on the size and complexity of the project.
Each team is responsible for a specific phase of the project, such as planning, design, implementation, and testing.
For example, a large software development project may involve m...read more
Asked in Hippo Cloud Technologies

Q. What are checked and unchecked exceptions?
Checked exceptions are checked at compile-time while unchecked exceptions are not checked at compile-time.
Checked exceptions are those which are checked at compile-time and the programmer is forced to handle them using try-catch or throws keyword.
Unchecked exceptions are those which are not checked at compile-time and the programmer is not forced to handle them.
Examples of checked exceptions are IOException, ClassNotFoundException, SQLException, etc.
Examples of unchecked exce...read more


Q. What are wrapper classes in Java?
There is no such thing as waperclass in Java.
The term 'waperclass' is not a valid Java keyword or class name.
It is possible that the interviewer made a mistake or was testing the candidate's ability to handle unexpected questions.
As a Java developer, it is important to be familiar with the language's syntax and keywords.
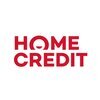
Asked in Home Credit Finance

Q. What is a collection?
A collection is a framework that provides an architecture to store and manipulate a group of objects.
Collections are used to store, retrieve, manipulate, and communicate data between objects.
They provide various data structures like lists, sets, and maps.
Collections offer methods to add, remove, and search for elements in the collection.
Examples of collections in Java include ArrayList, HashSet, and HashMap.
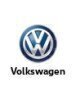
Asked in Volkswagen

Q. What is type casting in Java?
Type casting in Java is the process of converting one data type into another.
Type casting can be done implicitly or explicitly.
Implicit type casting is done automatically by the compiler when there is no loss of data.
Explicit type casting is done manually by the programmer when there is a possibility of data loss.
Type casting is useful when we want to use a variable of one data type in an expression or assignment of another data type.
Example: int num = 10; double decimalNum =...read more
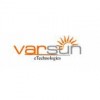
Asked in VARSUN eTechnologies

Q. Write a program to print a Christmas tree pattern using 8 and 4 stars.
Program to print Christmas tree with 8 and 4 stars
Use loops to print the tree structure
For 8 stars tree, use 4 rows and for 4 stars tree, use 3 rows
Print spaces before and after the stars to align them properly
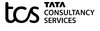
Asked in TCS

Q. What are the four pillars of OOPS?
The four pillars of OOPS are encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation: Bundling of data and methods into a single unit.
Inheritance: Ability to create new classes based on existing classes.
Polymorphism: Ability to use a single interface to represent different types of objects.
Abstraction: Hiding the implementation details and providing only the essential information.
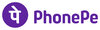
Asked in PhonePe

Q. What are the different types of status codes?
HTTP status codes indicate the outcome of a HTTP request. They are categorized into 5 classes.
1xx: Informational - Request received, continuing process
2xx: Success - Request successfully received, understood, and accepted
3xx: Redirection - Further action needed to complete the request
4xx: Client Error - Request contains bad syntax or cannot be fulfilled
5xx: Server Error - Server failed to fulfill a valid request
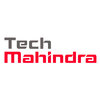
Asked in Tech Mahindra

Q. Why we need Java ? What is Polymorphism Can we use return type in constructer
Java is a widely used programming language known for its platform independence and extensive libraries.
Java is platform independent, meaning it can run on any operating system
Java has a large standard library with pre-built classes and methods for common tasks
Java is object-oriented, allowing for modular and reusable code
Java supports multithreading, enabling concurrent execution of tasks
Java has automatic memory management through garbage collection
Java is widely used in ent...read more
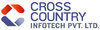
Asked in Cross Country Infotech

Q. Can we have multi-level inheritance in Java?
Yes, Java supports multi-level inheritance where a class can inherit from another class, and that class can further inherit from another class.
Java supports single inheritance, where a class can only inherit from one superclass.
However, multi-level inheritance is possible by creating a chain of classes where each class inherits from the one above it.
For example, Class A can be the superclass of Class B, and Class B can be the superclass of Class C.
Class C will inherit the pro...read more
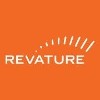
Asked in Revature

Q. Explain the given code and its expected output.
The code demonstrates Java concepts such as loops, arrays, and conditional statements.
The code initializes an array of integers and iterates through it using a for loop.
It checks each element for a specific condition (e.g., even or odd) and prints a message accordingly.
Example: If the array is {1, 2, 3, 4}, the output will be '1 is odd', '2 is even', '3 is odd', '4 is even'.


Q. What is the difference between an error and an exception?
Errors are severe issues that cannot be handled programmatically, while exceptions are issues that can be handled.
Errors are typically caused by the environment or system, while exceptions are caused by the program itself.
Errors are usually fatal and cannot be recovered from, while exceptions can be caught and handled.
Examples of errors include out of memory errors or stack overflow errors, while examples of exceptions include null pointer exceptions or arithmetic exceptions.
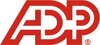
Asked in Automatic Data Processing (ADP)

Q. What is the code to print a right-angled triangle of numbers based on a given input number?
Use nested loops to print a right-angled triangle of numbers based on input.
Use two nested loops to control the rows and columns of the triangle.
Increment the number to be printed in each row.
Example: If input is 5, the output would be: 1, 12, 123, 1234, 12345
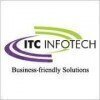
Asked in ITC Infotech

Q. Types of datatypes in Java
Java has several types of datatypes including primitive and reference types.
Primitive datatypes include int, double, boolean, char, etc.
Reference datatypes include classes, interfaces, arrays, etc.
Examples: int age = 25; String name = "John"; int[] numbers = {1, 2, 3};
Wrapper classes like Integer, Double, Boolean, etc. are used to wrap primitive types.
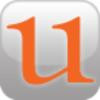
Asked in Unimity Solutions

Q. Write a program to find pairs of numbers that sum to 8.
Program to find pairs of numbers that sum up to 8
Use a nested loop to iterate through the array and find pairs
Check if the sum of the pair is equal to 8
Store and display the pairs that satisfy the condition
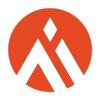
Asked in Apexon

Q. Write a program to find the numbers divisible by 3 in a string and reverse their indexes. For example, if the input is String s = "PHONE_NUMBER", the output should be String s = "PHONE_NUMBER".
This program identifies digits divisible by 3 in a string and reverses their positions.
Identify characters in the string that are divisible by 3 (e.g., '3', '6', '9').
Store the indexes of these characters for later use.
Reverse the order of the characters at the identified indexes.
Reconstruct the string with the reversed characters in their new positions.
Example: For input '123456789', output will be '129456783'.
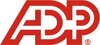
Asked in Automatic Data Processing (ADP)

Q. How can a Spring Boot project be created to develop an API that returns specified data?
A Spring Boot project can be created to develop an API by setting up a new Spring Boot project, defining API endpoints, and implementing the necessary logic.
Create a new Spring Boot project using Spring Initializr
Define API endpoints using @RestController annotation
Implement the logic to return specified data in the API endpoints
Use @GetMapping, @PostMapping, @PutMapping, @DeleteMapping annotations to map HTTP methods to controller methods
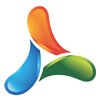
Asked in Allied Globetech

Q. What are the methods for encrypting and decrypting data within the OSI model?
Encryption and decryption methods can be applied at various layers of the OSI model to secure data transmission.
Application Layer: Use of SSL/TLS for encrypting HTTP traffic (HTTPS).
Transport Layer: Implementation of secure protocols like DTLS for UDP traffic.
Network Layer: IPsec can encrypt data packets for secure communication over IP.
Data Link Layer: WPA2 encrypts data in wireless networks to protect against eavesdropping.
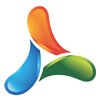
Asked in Allied Globetech

Q. What are the uses of Data Structures and Algorithms (DSA) in programming?
Data Structures and Algorithms optimize data management and processing, enhancing performance and efficiency in programming tasks.
Efficient data storage: Arrays, linked lists, and trees help organize data for quick access.
Optimized searching: Algorithms like binary search reduce time complexity when finding elements in sorted data.
Improved performance: Using appropriate data structures can significantly speed up operations, e.g., hash tables for fast lookups.
Resource manageme...read more
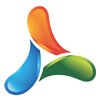
Asked in Allied Globetech

Q. How does OOPs work in Java? Explain its benefits with real-life examples.
OOP in Java organizes code into objects, enhancing modularity, reusability, and maintainability through real-world modeling.
Encapsulation: Hiding internal state, e.g., a 'BankAccount' class with private balance.
Inheritance: Creating subclasses, e.g., 'Dog' inherits from 'Animal', reusing properties.
Polymorphism: Methods can take many forms, e.g., 'draw()' method in 'Shape' class for 'Circle' and 'Square'.
Abstraction: Simplifying complex systems, e.g., a 'Car' class with metho...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
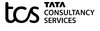
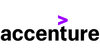
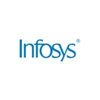
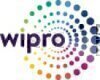
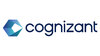
Top Interview Questions for Java Developer Related Skills
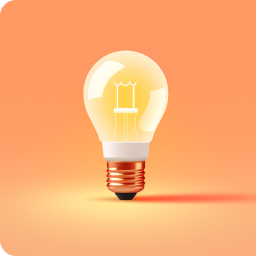
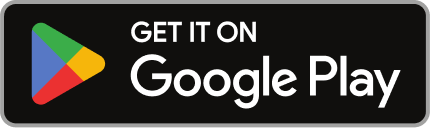
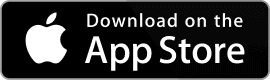
Reviews
Interviews
Salaries
Users
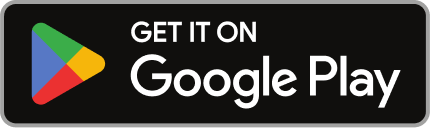
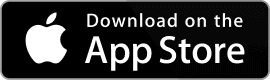