Embedded Software Developer
50+ Embedded Software Developer Interview Questions and Answers
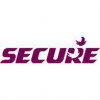
Asked in Secure Meters

Q. How many possible outcomes are there when determining the maximum of three numbers?
There are 4 possible result cases when finding the maximum of three numbers.
The maximum number is the same as the first number.
The maximum number is the same as the second number.
The maximum number is the same as the third number.
The maximum number is different from all three numbers.
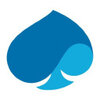
Asked in Capgemini Engineering

Q. Write a C program to check if a number is a power of 2.
This program checks if a given number is a power of 2 using bitwise operations.
A number is a power of 2 if it has exactly one bit set in its binary representation.
You can use the expression (n & (n - 1)) == 0 to check this.
Example: 4 (binary 100) is a power of 2, while 6 (binary 110) is not.
The program should handle edge cases like 0 and negative numbers.
Embedded Software Developer Interview Questions and Answers for Freshers
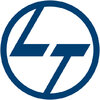
Asked in Larsen & Toubro Limited

Q. What is the difference between volatile const int a and const volatile int b?
volatile constant and constant volatile are different in terms of read and write access.
volatile constant means the value can be changed by external factors but cannot be modified by the program
constant volatile means the value cannot be changed by external factors but can be modified by the program
the order of volatile and constant keywords matter
example: volatile const int a = 5; const volatile int *b = &a;
a can be changed by external factors but cannot be modified by the p...read more
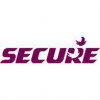
Asked in Secure Meters

Q. How can you sort an array in place, using minimal additional memory?
Short an array without using another array, with minimum parameters
Use a sorting algorithm like bubble sort or insertion sort
Iterate through the array and compare adjacent elements, swapping them if necessary
Repeat the process until the array is sorted
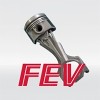
Asked in FEV

Q. what is interrupt service routine, watchdog timer, lambda function, baud rate, CAN frame format, spi, Autosar related questions some
Interrupt service routine handles interrupts, watchdog timer resets system if it hangs, lambda function is an anonymous function, baud rate is data transfer rate, CAN frame format is used in Controller Area Network, SPI is Serial Peripheral Interface, Autosar is an automotive software architecture.
ISR is a function that handles interrupts from hardware devices
Watchdog timer resets the system if it hangs or stops responding
Lambda function is an anonymous function that can be p...read more
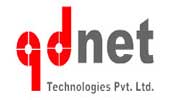
Asked in QDnet Technologies

Q. How do you interface a microprocessor kit with I/O devices?
Interfacing microprocessor kit with I/O devices involves selecting appropriate communication protocol and configuring the microprocessor pins.
Select appropriate communication protocol (e.g. SPI, I2C, UART)
Configure microprocessor pins for communication
Write code to communicate with I/O devices
Test and debug the interface
Embedded Software Developer Jobs
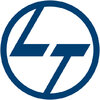
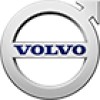
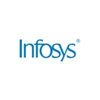
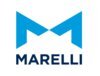
Asked in Marelli

Q. What are the use cases of unions in the C programming language?
Unions in C are used to store different data types in the same memory location.
Unions are used to save memory by allowing different data types to share the same memory location.
They are commonly used in situations where only one of the data types needs to be accessed at a time.
For example, a union can be used to store an integer and a float, with only one being accessed depending on the context.
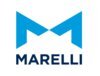
Asked in Marelli

Q. What is the purpose of using a 120-ohm resistor in the Controller Area Network (CAN) protocol?
A 120-ohm resistor is used in the CAN protocol to match the characteristic impedance of the network and reduce signal reflections.
The 120-ohm resistor helps to match the characteristic impedance of the CAN network, which is typically 120 ohms.
It reduces signal reflections that can cause data corruption and communication errors.
The resistor is placed at both ends of the CAN bus to ensure proper termination.
Without the resistor, the signal quality and reliability of the CAN com...read more
Share interview questions and help millions of jobseekers 🌟
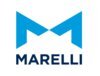
Asked in Marelli

Q. What is Pulse Width Modulation (PWM), and how does it work?
PWM is a technique used to encode analog signal using digital pulses of varying widths.
PWM is commonly used in controlling the speed of motors, brightness of LEDs, and audio signals.
It works by varying the width of the pulse in a fixed time period, known as the duty cycle.
The average voltage of the signal is determined by the duty cycle - higher duty cycle means higher average voltage.
PWM is achieved by rapidly switching a digital signal on and off at a specific frequency.
It ...read more
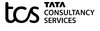
Asked in TCS

Q. Factorial of a number. Check validity of the number when scanning latter from user
Validating user input for factorial calculation
Use a loop to calculate the factorial of the number
Check if the input is a positive integer
Handle edge cases such as 0 and 1
Use try-except block to catch non-integer inputs
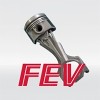
Asked in FEV

Q. What is Constructor, is virtual constructor possible in C++, Virtual destructor with implementation.
Constructor is a special member function used for initializing objects. Virtual constructor is not possible in C++. Virtual destructor can be implemented.
Constructor is a special member function with the same name as the class, used for initializing objects.
Virtual constructor is not possible in C++ as constructors cannot be virtual.
Virtual destructor is possible in C++ and is used to ensure proper cleanup of resources when an object is destroyed.
Example: virtual ~ClassName()...read more
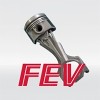
Asked in FEV

Q. What is Friend function, Getter setter, Static variable and static function with implementation
Friend function is a function that is not a member of a class but has access to its private and protected members. Getter setter are methods used to access and modify private variables. Static variables are shared among all instances of a class. Static functions are functions that can be called without an instance of the class.
Friend function allows external functions to access private and protected members of a class.
Getter setter methods are used to get and set the values o...read more
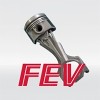
Asked in FEV

Q. Getter setter implementation, implementation of virtual function, difference between encapsulation and abstraction, focus on OOP
Understanding getter setter, virtual function, encapsulation, abstraction in OOP
Getter and setter methods are used to access and modify private class members respectively
Virtual functions in C++ allow dynamic binding and are overridden in derived classes
Encapsulation is bundling data and methods that operate on the data together
Abstraction is hiding the complex implementation details and showing only necessary features
OOP focuses on creating objects that interact with each ot...read more
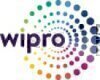
Asked in Wipro

Q. microcontroller -protocols used -debugging process -ide's used -difficult problem you faced and how u solved it
As an embedded software developer, I have experience with microcontrollers, protocols, debugging, and IDEs.
I have worked with microcontrollers such as Arduino, STM32, and PIC.
I am familiar with protocols such as UART, SPI, I2C, and CAN.
For debugging, I have used tools such as JTAG, GDB, and logic analyzers.
I have experience with IDEs such as Eclipse, Keil, and MPLAB.
One difficult problem I faced was optimizing code for a real-time system with limited resources. I solved it by...read more
Asked in Actevia Technology Services

Q. What are the different types of storage classes in programming, and how do they function?
Storage classes in programming define the scope, lifetime, and visibility of variables.
1. Automatic: Variables are created when a block is entered and destroyed when it exits. Example: 'int a;' inside a function.
2. Static: Variables retain their value between function calls. Example: 'static int count = 0;' inside a function.
3. External: Variables are accessible across multiple files. Example: 'extern int globalVar;' declared in one file and used in another.
4. Register: Sugge...read more
Asked in Actevia Technology Services

Q. What is the difference between a structure and a union in programming?
Structures store multiple data types, while unions share the same memory space for different data types.
Structures allocate separate memory for each member, allowing simultaneous access.
Unions use a single memory location for all members, saving space but limiting access to one member at a time.
Example of a structure: struct Person { char name[50]; int age; };
Example of a union: union Data { int intValue; float floatValue; char charValue; };
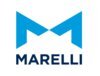
Asked in Marelli

Q. What is the purpose of the volatile keyword in programming?
The volatile keyword in programming is used to indicate that a variable's value can be changed unexpectedly.
Used to prevent compiler optimizations that assume variable values do not change outside of the program
Commonly used for variables that are modified by hardware or other threads
Example: volatile int sensorValue; // variable updated by external sensor
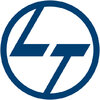
Asked in Larsen & Toubro Limited

Q. What is sub function of 19 02?
The sub function of 19 02 is not specified.
The question does not provide any context or information about the sub function of 19 02.
Without additional details, it is impossible to determine the specific sub function being referred to.
More information or clarification is needed to answer the question.
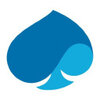
Asked in Capgemini Engineering

Q. Given a string, find the number of palindromes.
Count the number of palindromes in a given string.
Iterate through the string and check for palindromes of length 1, 2, 3, ... n
Use two pointers to check if a substring is a palindrome
Consider both even and odd length palindromes
Store the count of palindromes found
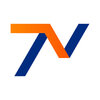
Asked in TVS Next

Q. What is your strongest programming language?
My language strength in programming is primarily in C and C++, with experience in other languages as well.
Proficient in C and C++ programming languages
Familiar with other languages such as Python and Java
Experience in developing embedded software using C and C++
Knowledge of low-level programming and hardware interfaces
Ability to write efficient and optimized code
Understanding of software development methodologies and best practices
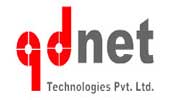
Asked in QDnet Technologies

Q. Explain LED interfacing.
LED interfacing involves connecting LEDs to a microcontroller and controlling them using software.
Connect LED to microcontroller output pin
Use software to set output pin high or low to turn LED on or off
Use PWM to control LED brightness
Use external driver circuit for high-power LEDs
Use resistors to limit current and prevent damage to LED
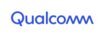
Asked in Qualcomm

Q. Write your own memcpy function, taking care of boundary conditions.
Write a custom memcpy function with boundary condition handling.
Use pointers to copy data from source to destination.
Check for NULL pointers and zero length cases.
Use a loop to copy data byte by byte.
Handle overlapping memory regions with caution.
Asked in Actevia Technology Services

Q. How can I get and set the nth bit in a number?
Use bitwise operations to get and set the nth bit in a number efficiently.
To get the nth bit: Use bitwise AND with a mask. Example: (num & (1 << n)) != 0 checks if nth bit is set.
To set the nth bit: Use bitwise OR with a mask. Example: num | (1 << n) sets the nth bit to 1.
To clear the nth bit: Use bitwise AND with the negation of the mask. Example: num & ~(1 << n) clears the nth bit.
To toggle the nth bit: Use bitwise XOR with a mask. Example: num ^ (1 << n) toggles the nth bi...read more
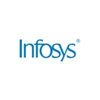
Asked in Infosys

Q. What are the latest advancements in embedded C programming?
Latest embedded C programming includes features like dynamic memory allocation, multi-threading, and object-oriented programming.
Dynamic memory allocation allows for more efficient use of memory
Multi-threading enables concurrent execution of multiple tasks
Object-oriented programming allows for better code organization and reusability
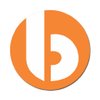
Asked in Bacancy Technology

Q. What is the difference between SPI and I2C Communication protocols?
SPI is faster, full-duplex, and uses separate data lines, while I2C is slower, half-duplex, and uses a shared data line.
SPI is faster than I2C
SPI is full-duplex, allowing data to be sent and received simultaneously
SPI uses separate data lines for communication
I2C is slower than SPI
I2C is half-duplex, meaning data can only be sent or received at a time
I2C uses a shared data line for communication
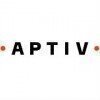
Asked in Aptiv

Q. 1. What is structured, pointer, array , union
Structured, pointer, array, and union are all data types in C programming language.
Structured data type is a collection of variables of different data types under a single name.
Pointer is a variable that stores the memory address of another variable.
Array is a collection of similar data types stored in contiguous memory locations.
Union is a special data type that allows storing different data types in the same memory location.
Example: struct employee { char name[20]; int age;...read more
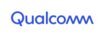
Asked in Qualcomm

Q. Given two singly linked lists, find the node at which the two lists intersect. Optimize your solution.
Find intersection point of two linked lists and optimize solution.
Traverse both lists and find their lengths
Move the head of the longer list to the same distance as the shorter list
Traverse both lists in parallel until they meet at the intersection point
Use hash table to store visited nodes for faster lookup
Use two pointers, one starting from the head of each list, and move them at the same pace until they meet at the intersection point
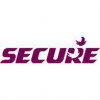
Asked in Secure Meters

Q. Write code to set or reset a bit.
Code for bit set or reset
To set a bit, use bitwise OR operator with 1 shifted left by the bit position
To reset a bit, use bitwise AND operator with the complement of 1 shifted left by the bit position
Use unsigned integer data type for bit manipulation
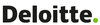
Asked in Deloitte

Q. What is the difference between a static and a dynamic library?
Static libraries are linked at compile-time, while dynamic libraries are linked at run-time.
Static libraries are included in the final executable file, increasing its size.
Dynamic libraries are loaded into memory only when needed, reducing the executable size.
Static libraries are platform-specific, while dynamic libraries can be shared across platforms.
Static libraries provide faster execution, while dynamic libraries allow for easier updates and maintenance.
Examples of stati...read more
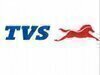
Asked in TVS Motor

Q. Why are you using a network?
Networks are essential for communication and data transfer between devices and systems.
Networks enable devices to communicate and share data with each other.
They allow for remote access and control of devices.
Networks facilitate the transfer of large amounts of data quickly and efficiently.
They enable the creation of complex systems and applications that rely on multiple devices and components.
Examples include the internet, local area networks (LANs), and wireless networks.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
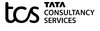
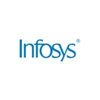
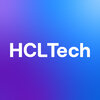
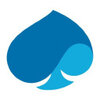

Top Interview Questions for Embedded Software Developer Related Skills
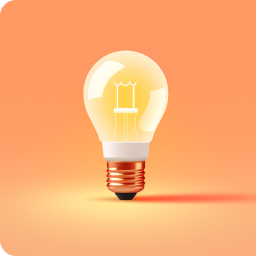
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
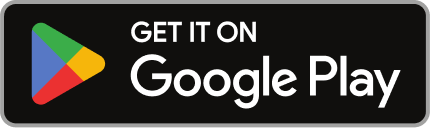
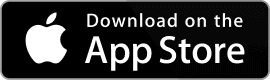
Reviews
Interviews
Salaries
Users
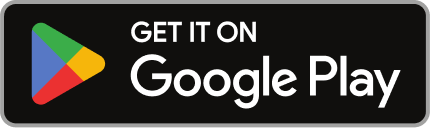
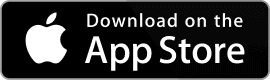