
Asked in UnitedHealth
Word Presence in Sentence
Determine if a given word 'W' is present in the sentence 'S' as a complete word. The word should not merely be a substring of another word.
Input:
The first line contains an integer 'T', the number of test cases.
The first line of each test case contains the sentence 'S'.
The second line of each test case contains the word 'W'.
Output:
For each test case, output "Yes" if the word 'W' is present in the sentence 'S' as a complete word; otherwise, output "No".
Each output should be printed on a new line.
Example:
Input:
T = 1
S = "hello world"
W = "hello"
Output:
Yes
Constraints:
1 ≤ T ≤ 50
1 ≤ |S|, |W| ≤ 10000
- 'S' and 'W' comprise lowercase letters only
Note: Words are separated by spaces in sentence 'S'.
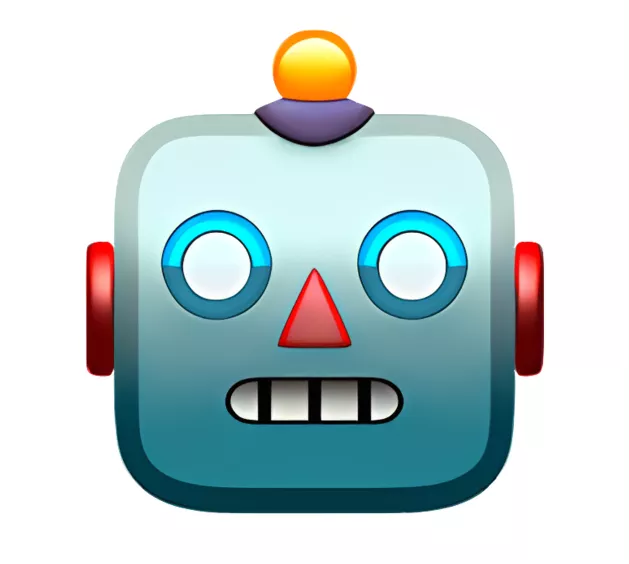
AnswerBot
4mo
Check if a given word is present in a sentence as a complete word, not a substring.
Split the sentence into words using spaces as delimiters.
Check if the given word matches any of the words in the sent...read more
Help your peers!
Add answer anonymously...
Interview Questions Asked to Software Engineer Intern at Other Companies
Top Skill-Based Questions for UnitedHealth Software Engineer Intern
Algorithms Interview Questions and Answers
250 Questions
Data Structures Interview Questions and Answers
250 Questions
SQL Interview Questions and Answers
250 Questions
Web Development Interview Questions and Answers
250 Questions
Stay ahead in your career. Get AmbitionBox app
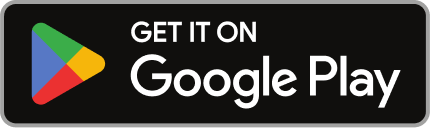
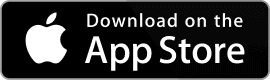
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
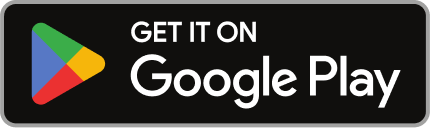
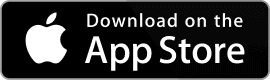