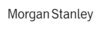
Asked in Morgan Stanley
Box Stacking Problem Statement
Consider you are provided with 'n' different types of rectangular 3D boxes. For each type of box, you have three separate arrays: height
, width
, and length
that define the dimensions of the boxes. Specifically, the ith type of box dimensions are given by height[i]
, width[i]
, and length[i]
.
Your task is to stack these boxes to achieve the maximum possible height.
Input:
The first line contains an integer ‘T’, the number of test cases.
For each test case, the input is structured as follows:
1. An integer ‘n’, the number of box types.
2. ‘n’ space-separated integers that specify the height
array.
3. ‘n’ space-separated integers that specify the width
array.
4. ‘n’ space-separated integers that specify the length
array.
Output:
For each test case, output a single integer, representing the height of the tallest stack possible.
Example:
Input:
1
3
4 1 4
6 2 5
7 3 6
Output:
15
Explanation:
The boxes can be rotated to allow any face to be the base. Using rotations, the maximum height stack can be formed with the dimensions given.
Constraints:
- 1 <= T <= 50
- 1 <= n <= 102
- 1 <= height[i] <= 102
- 1 <= width[i] <= 102
- 1 <= length[i] <= 102
- Time limit: 1 second
Note:
The following rules apply when stacking:
- You can only place a box on top of another if its base dimensions (length and width) are strictly smaller than the box beneath it.
- Boxes can be rotated to use any face as the base, and you can use multiple copies of the same box, resulting in different possible orientations for each type.
- No two boxes will have all three dimensions identical.
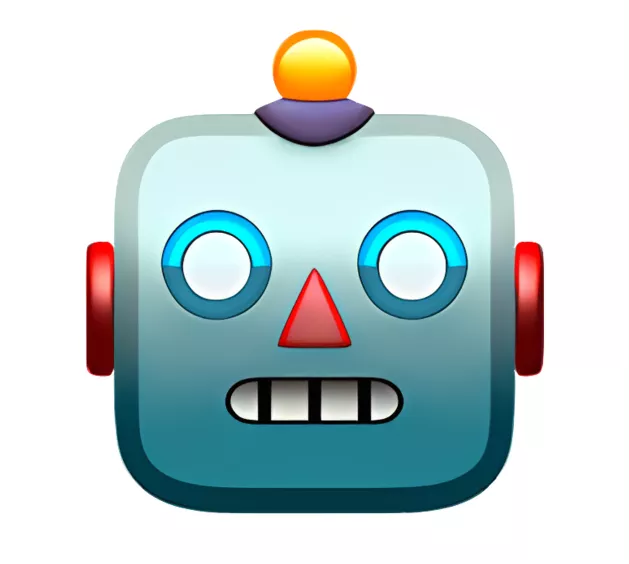
The task is to stack different types of rectangular 3D boxes to achieve the maximum possible height by following certain constraints.
Sort the boxes based on their base dimensions in non-increasing ord...read more
Top Technology Analyst Interview Questions Asked at Morgan Stanley
Interview Questions Asked to Technology Analyst at Other Companies
Top Skill-Based Questions for Morgan Stanley Technology Analyst
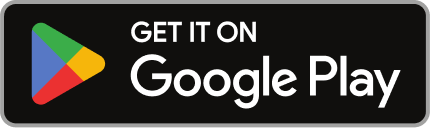
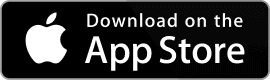
Reviews
Interviews
Salaries
Users
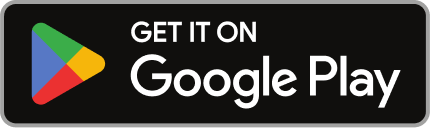
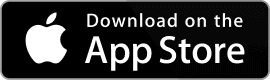