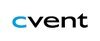
Asked in Cvent
Detect Cycle in Undirected Graph Problem Statement
You are provided with an undirected graph composed of 'N' vertices and 'M' edges, where vertices are labeled from 1 to 'N'.
Your task is to determine if there exists a cycle in the graph.
A cycle is defined as a path that begins and ends at the same vertex, traversing the edges only once.
Example:
Input:
N = 3, Edges = [[1, 2], [2, 3], [1, 3]]
Output:
Yes
Explanation:
The graph has 3 vertices and edges forming a cycle between vertices 1, 2, and 3. Thus, a cycle exists.
Constraints:
1 ≤ T ≤ 10
1 ≤ N ≤ 5000
0 ≤ M ≤ min(5000, (N * (N - 1)) / 2)
1 ≤ edges[i][0] ≤ N
1 ≤ edges[i][1] ≤ N
- There are no parallel edges between two vertices.
- There are no self-loops (an edge connecting a vertex to itself).
- The graph can be disconnected.
Input:
The first line contains an integer 'T' indicating the number of test cases. Each test case begins with two space-separated integers, ‘N’ (number of vertices) and ‘M’ (number of edges). The following ‘M’ lines describe the edges with two space-separated integers, representing an edge in the graph.
Output:
For each test case, return “Yes” if there is a cycle in the graph, else return “No”.
Note:
You do not need to print the output; it is handled automatically. Implement the function to determine the cycle presence.
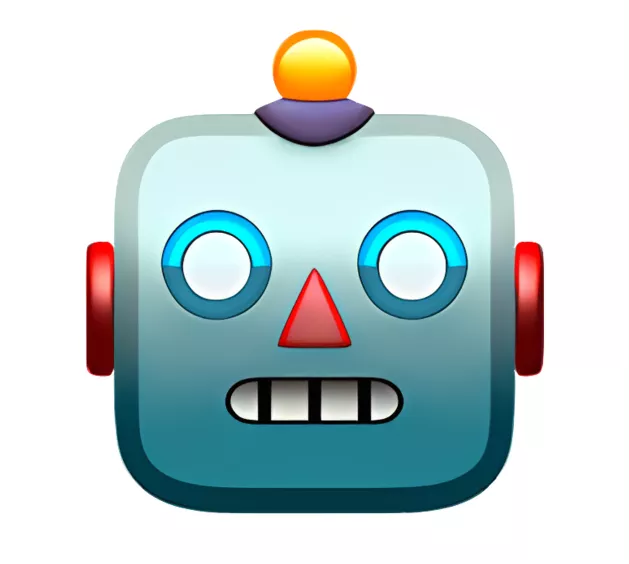
Detect cycle in an undirected graph by checking for a path that begins and ends at the same vertex.
Use Depth First Search (DFS) to traverse the graph and detect cycles.
Maintain a visited set to keep t...read more
Top Software Engineer Interview Questions Asked at Cvent
Interview Questions Asked to Software Engineer at Other Companies
Top Skill-Based Questions for Cvent Software Engineer
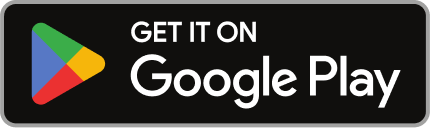
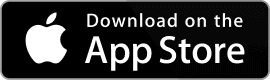
Reviews
Interviews
Salaries
Users
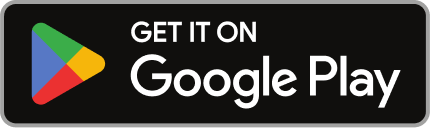
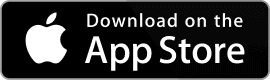