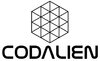
Asked in Codalien Technologies
Construct Complete Binary Tree Problem Statement
Given an array or list 'ARR' containing values for 'N' nodes of a binary tree, your task is to construct a complete binary tree in a level order fashion. The elements will be filled in level-by-level starting from level 0.
A Complete Binary Tree is defined such that all levels are fully filled except, possibly, the last one, which should be filled from left to right as much as possible.
Example:
Input:
ARR = [5, 6, 10, 2, 3, 5, 9]
Output:
5 6 10 2 3 5 9 -1 -1 -1 -1 -1 -1 -1 -1
Explanation:
The binary tree constructed from the provided array would look like this:
- Level 1: The root node is 4.
- Level 2: The left child of 5 is 6; the right child of 5 is 10.
- Level 3: The left child of 6 is 2; the right child of 6 is 3; the left child of 10 is 5; the right child of 10 is 9.
- Level 4: All children of level 3 nodes are null (-1 for each).
Output is written as a single line with values separated by spaces. The tree traversal ends when all nodes at the last level are null (-1).
Constraints:
1 <= T <= 100
1 <= N <= 3000
0 <= ARR[i] <= 10^6
- Time limit: 1 sec
Note:
You do not need to print anything; it is already managed. Implement the function to return the root of the binary tree.
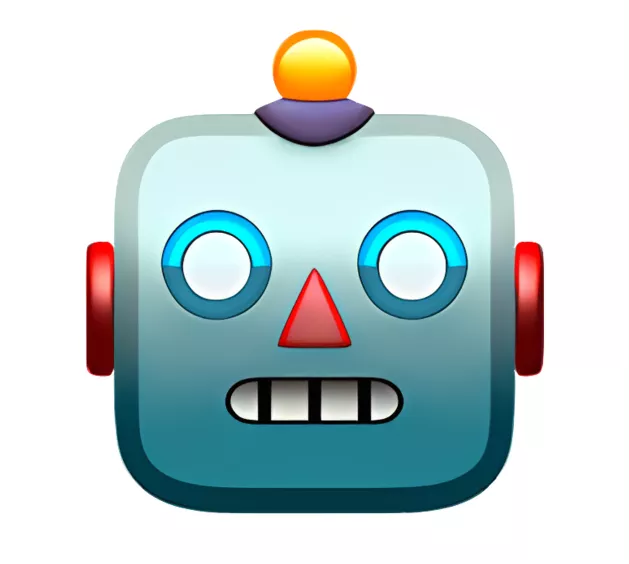
Construct a complete binary tree in level order fashion from given array of values for nodes.
Create a queue to store nodes
Start with the first element as the root node
Pop the front node from the queue...read more
Top Software Engineer Interview Questions Asked at Codalien Technologies
Interview Questions Asked to Software Engineer at Other Companies
Top Skill-Based Questions for Codalien Technologies Software Engineer
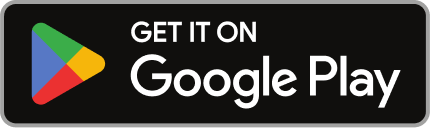
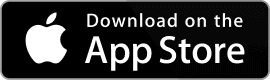
Reviews
Interviews
Salaries
Users
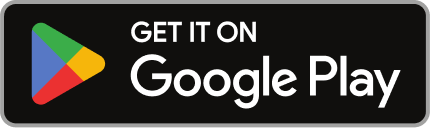
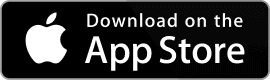