UI Developer
200+ UI Developer Interview Questions and Answers

Asked in Frrole

Q. How can we reverse a string in JavaScript in one line?
The string can be reversed in one line using the split(), reverse(), and join() methods.
Use the split() method to convert the string into an array of characters
Use the reverse() method to reverse the order of the array elements
Use the join() method to convert the reversed array back into a string
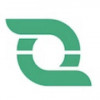
Asked in Infrrd

Q. What are closures in JavaScript? Explain using an appropriate example.
Closures in JavaScript are functions that have access to variables from their outer scope even after the outer function has finished executing.
Closures allow functions to access variables from their parent function's scope
They are created whenever a function is defined within another function
Closures can be used to create private variables and functions
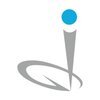
Asked in Infogain

Q. What is Redux and how does it work behind the scenes (code flow)?
Redux is a predictable state container for JavaScript apps. It helps manage the state of an application in a single immutable state tree.
Redux stores the entire state of an application in a single immutable state tree.
The state tree is read-only, and changes are made by dispatching actions.
Reducers specify how the state changes in response to actions.
The store holds the state tree, allows access to state via getState(), and allows state to be updated via dispatch(action).
Midd...read more
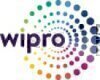
Asked in Wipro

Q. Does the JavaScript ES6 spread operator create a deep copy of an object?
The ES6 spread operator creates a shallow copy, not a deep copy, of an object.
The spread operator (...) copies properties from one object to another.
It creates a shallow copy, meaning nested objects are still referenced.
Example: const obj1 = { a: 1, b: { c: 2 } }; const obj2 = { ...obj1 }; // obj2.b is the same reference as obj1.b
Modifying nested properties in obj2 will affect obj1: obj2.b.c = 3; // obj1.b.c is now 3.
For deep copying, use methods like JSON.parse(JSON.stringif...read more
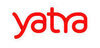
Asked in Yatra

Q. What is the difference between __proto__ and prototype?
The __proto__ is a property that points to the prototype of an object, while prototype is a property of a constructor function.
The __proto__ property is used to access the prototype of an object.
The prototype property is used to add properties and methods to a constructor function.
The __proto__ property is deprecated and should not be used in production code.
Changes to the prototype of a constructor function will affect all instances of that constructor function.
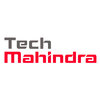
Asked in Tech Mahindra

Q. How do you fix cumulative layout shift issues?
To fix cumulative layout shift issues, ensure all images and media have dimensions specified in HTML, use CSS aspect ratio boxes, and prioritize loading critical resources.
Specify dimensions for all images and media in HTML to prevent layout shifts.
Use CSS aspect ratio boxes to reserve space for images before they load.
Prioritize loading critical resources to avoid sudden layout changes.
UI Developer Jobs
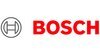
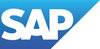
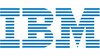
Asked in Brain Vision Technology

Q. what is angular? , what is use of javascript?, what is hosting? , what is closer? etc
Angular is a JavaScript framework for building web applications. JavaScript is a programming language used for creating interactive web pages. Hosting refers to the process of making a website accessible on the internet. A closure is a function that has access to variables in its outer scope.
Angular is a front-end framework used for building dynamic web applications.
JavaScript is a programming language that allows developers to create interactive web pages and web application...read more
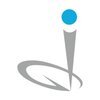
Asked in Infogain

Q. How are you managing state in your current applications?
I manage state using React's useState hook and context API for global state management.
Using React's useState hook to manage local component state
Utilizing React's context API for global state management
Implementing Redux for complex state management scenarios
Share interview questions and help millions of jobseekers 🌟
Asked in Aggregate Intelligence

Q. What are the steps involved in creating a to-do list application using React.js?
Creating a to-do list app in React involves setting up components, state management, and event handling.
1. Set up a new React project using Create React App: `npx create-react-app todo-app`.
2. Create components: Define components like `TodoList`, `TodoItem`, and `AddTodo` for structure.
3. Manage state: Use React's `useState` hook to manage the list of to-dos.
4. Implement add functionality: Create a form in `AddTodo` to input new tasks and update state.
5. Display tasks: Map th...read more
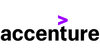
Asked in Accenture

Q. What is HTML5? What is css3? How to achieve media queries?
HTML5 is the latest version of the HTML standard, CSS3 is the latest version of the CSS standard, and media queries are used in CSS to apply different styles based on device characteristics.
HTML5 is the fifth and latest version of the HTML standard used for structuring content on the web.
CSS3 is the latest version of the CSS standard used for styling web pages.
Media queries in CSS allow for responsive design by applying different styles based on device characteristics like sc...read more
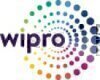
Asked in Wipro

Q. How can variable values be swapped in a single statement in JavaScript?
In JavaScript, variable values can be swapped using destructuring assignment, a concise and elegant method.
Use destructuring assignment: [a, b] = [b, a];
This method allows swapping without a temporary variable.
Example: let x = 1, y = 2; [x, y] = [y, x]; // x is now 2, y is now 1.
Destructuring is supported in ES6 and later versions.
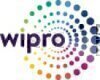
Asked in Wipro

Q. How can you create a deep copy of an object in JavaScript?
A deep copy of an object in JavaScript creates a new object with all properties copied recursively.
Use JSON methods: const deepCopy = JSON.parse(JSON.stringify(originalObject));
Use a recursive function to copy properties: function deepCopy(obj) { return Array.isArray(obj) ? obj.map(deepCopy) : typeof obj === 'object' ? Object.fromEntries(Object.entries(obj).map(([k, v]) => [k, deepCopy(v)])) : obj; }
Utilize libraries like Lodash: const deepCopy = _.cloneDeep(originalObject);
F...read more
Asked in Pivotrics

Q. What is the difference between display: none and visibility: hidden?
display: None removes the element from the flow of the document, while visibility: 0 hides the element but still takes up space.
display: None removes the element from the document flow, making it invisible and not taking up any space.
visibility: 0 hides the element visually, but it still occupies space in the layout.
display: None is commonly used to hide elements completely, while visibility: 0 is used to hide elements while preserving the layout.
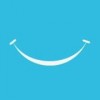
Asked in Appiness Interactive

Q. Can you write the basic HTML structure?
The basic HTML structure includes essential elements like doctype, html, head, and body for a web page.
<!DOCTYPE html> - Declares the document type and version of HTML.
<html> - The root element that wraps all content on the page.
<head> - Contains meta-information, title, and links to stylesheets.
<title> - Sets the title of the web page displayed in the browser tab.
<body> - Contains the content of the web page, such as text, images, and links.

Asked in LogiNext Solutions

Q. 1. what is react 2.create a tab strcture like when u click add css and open that tab
React is a JavaScript library for building user interfaces.
React is a popular JavaScript library for building user interfaces.
To create a tab structure in React, you can use components like Tabs and TabPanels.
When a tab is clicked, you can use state to show or hide the content of that tab.
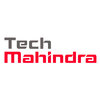
Asked in Tech Mahindra

Q. Explain the difference between authorization and authentication.
Authentication verifies a user's identity, while authorization determines what a user can access.
Authentication confirms a user's identity through credentials like username and password.
Authorization controls access to resources based on the authenticated user's permissions.
Example: Logging into a website (authentication) and then being able to view/edit specific pages based on your role (authorization).
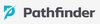
Asked in Pathfinder Global

Q. Why is React JS faster compared to other frameworks?
React uses virtual DOM and efficient rendering techniques to make it faster than other frameworks.
React uses virtual DOM which reduces the number of direct DOM manipulations, making it faster.
React uses efficient rendering techniques like shouldComponentUpdate and PureComponent to optimize rendering.
React also has a one-way data flow which makes it easier to manage and update data, improving performance.
React's modular architecture allows for code reuse and reduces redundancy...read more
Asked in Giant Wheel Studio

Q. What is Redux, and why do we use it for state management?
Redux is a predictable state container for JavaScript apps. It helps manage the state of an application in a centralized manner.
Redux is used for state management in JavaScript applications.
It provides a predictable state container, making it easier to manage and update the state of an application.
Redux follows a unidirectional data flow, where the state is updated through actions and reducers.
It allows for better separation of concerns by keeping the state separate from the ...read more
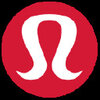
Asked in Lululemon Athletica

Q. Write code to display data in a table with options to edit and delete rows, and data should be stored in local storage.
Create a dynamic table with edit and delete options, storing data in local storage for persistence across sessions.
HTML Structure: Use a <table> element to display data, with <tr> for rows and <td> for cells.
Local Storage: Use localStorage.setItem() to save data and localStorage.getItem() to retrieve it.
Edit Functionality: Implement an edit button that populates a form with the row's data for modification.
Delete Functionality: Add a delete button that removes the row from the...read more
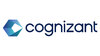
Asked in Cognizant

Q. Given a string, determine whether it is a palindrome.
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward.
Convert the given string to lowercase and remove any non-alphanumeric characters
Reverse the string and compare it with the original string to check for palindrome
Examples: 'racecar' is a palindrome, 'hello' is not a palindrome
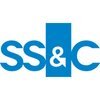
Asked in SS&C TECHNOLOGIES

Q. Write a query to retrieve the second highest salary from the database.
Retrieve the second highest salary using SQL queries with various methods.
Use the 'DISTINCT' keyword to eliminate duplicates: SELECT DISTINCT salary FROM employees ORDER BY salary DESC LIMIT 1 OFFSET 1;
Use a subquery to find the maximum salary that is less than the highest: SELECT MAX(salary) FROM employees WHERE salary < (SELECT MAX(salary) FROM employees);
Utilize the 'ROW_NUMBER()' window function: SELECT salary FROM (SELECT salary, ROW_NUMBER() OVER (ORDER BY salary DESC) ...read more
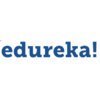
Asked in Edureka

Q. How do you add a delay to an animation using jQuery?
Use the delay() method in jQuery to add delay for animation.
Use the delay() method before the animation function.
Specify the duration of the delay in milliseconds as an argument.
Example: $(element).delay(1000).fadeOut();
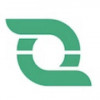
Asked in Infrrd

Q. What are the various lifecycle hooks in Angular?
Angular lifecycle hooks are methods that allow you to tap into specific points in a component's lifecycle.
ngOnChanges: Called when an input property changes
ngOnInit: Called once the component is initialized
ngDoCheck: Called during every change detection run
ngAfterContentInit: Called after content (ng-content) has been projected into the component
ngAfterContentChecked: Called after every check of the projected content
ngAfterViewInit: Called after the component's view has been ...read more
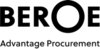
Asked in Beroe Inc

Q. Describe a React task to fetch data from an API and implement country, state, and city selection.
Fetching and selecting country, state, and city data from API using React
Use axios or fetch to get data from API
Create dropdown menus for country, state, and city selection
Use onChange event to update state based on user selection
Filter data based on selected country and state to display relevant cities
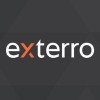
Asked in Exterro

Q. Share your screen and sort an array without using built-in JavaScript methods.
Sorting an array without using built-in JS methods
Create a custom sorting function using a sorting algorithm like bubble sort, selection sort, or insertion sort
Compare each element in the array and swap them if they are in the wrong order
Repeat the process until the array is fully sorted
Asked in ValueMatrix

Q. How do you ensure cross browser compatibility in your CSS?
To ensure cross-browser compatibility in CSS, I use various techniques and tools to test and adapt styles for different browsers.
Use CSS resets or normalize.css to create a consistent baseline across browsers.
Utilize vendor prefixes for properties that require them, e.g., '-webkit-', '-moz-', '-ms-'.
Test styles in multiple browsers (Chrome, Firefox, Safari, Edge) and devices to identify issues.
Leverage feature detection libraries like Modernizr to apply styles conditionally b...read more
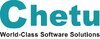
Asked in Chetu

Q. What are the basic concepts of UI/UX design and development?
UI/UX design focuses on enhancing user satisfaction through effective design and usability principles.
User-Centered Design: Prioritizing user needs and preferences in the design process. Example: Conducting user interviews.
Usability: Ensuring the product is easy to use. Example: Simplifying navigation in a mobile app.
Accessibility: Designing for users with disabilities. Example: Using alt text for images.
Visual Hierarchy: Organizing content to guide users' attention. Example:...read more
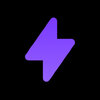
Asked in BlogHunch

Q. What are the essential elements of effective design?
Effective design combines usability, aesthetics, and functionality to create a seamless user experience.
User-Centric Approach: Design should prioritize the needs and preferences of users. For example, conducting user research to inform design decisions.
Consistency: Maintain uniformity in design elements like colors, fonts, and layouts. For instance, using the same button style across the application.
Accessibility: Ensure that designs are usable for people with disabilities. A...read more

Asked in Ranosys Technologies

Q. What is meant by "sticky" in the context of position?
In UI design, 'sticky' refers to elements that remain fixed in place during scrolling, enhancing user navigation.
Sticky positioning is achieved using CSS with 'position: sticky'.
An element with 'position: sticky' toggles between 'relative' and 'fixed' based on the scroll position.
Example: A sticky header remains visible at the top of the viewport while scrolling down a page.
Sticky elements can be used for navigation menus, sidebars, or call-to-action buttons.
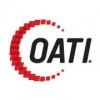
Asked in Open Access Technology India

Q. What is Ajax and How does ajax works
Ajax is a technique used to update parts of a web page without reloading the entire page.
Ajax stands for Asynchronous JavaScript and XML.
It allows for asynchronous communication between the client and server.
Ajax uses XMLHttpRequest object to send and receive data from the server.
It can update the content of a web page dynamically without refreshing the whole page.
Ajax is commonly used for creating interactive web applications.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
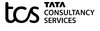
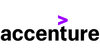
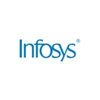
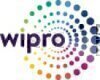
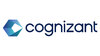
Top Interview Questions for UI Developer Related Skills
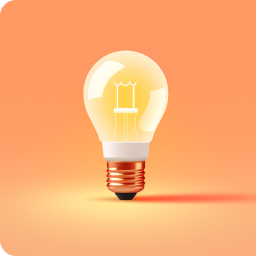
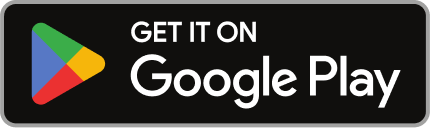
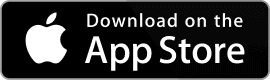
Reviews
Interviews
Salaries
Users
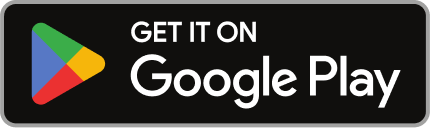
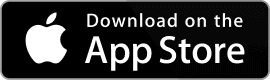