Software Engineer II
100+ Software Engineer II Interview Questions and Answers
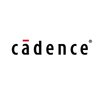
Asked in Cadence Design Systems

Q. There are fifteen horses and a racing track that can run five horses at a time. You have to figure out the top 3 horses out of those and you don't have any timer machine to measure. How will you find the top 3...
read moreDivide the horses into groups of 5 and race them. Take the top 2 from each race and race them again. Finally, race the top 2 horses to determine the top 3.
Divide the horses into 3 groups of 5 and race them.
Take the top 2 horses from each race and race them again.
Finally, race the top 2 horses to determine the top 3.

Asked in Moonfrog Labs

Q. Given an array arr[] containing N integers. In one step, any element of the array can either be changed by +1,-1,0. Find the minimum number of steps required such that the final product of all modified elements...
read moreFind minimum steps to make product of array equal to 1 by changing elements by +1,-1,0.
Calculate number of negative elements and number of zeroes in array.
If number of negative elements is even, add 1 to all elements.
If number of negative elements is odd, add 1 to all elements except the smallest negative element.
Count the number of steps taken to modify the array elements.
Return the count of steps taken.
Software Engineer II Interview Questions and Answers for Freshers
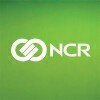
Asked in NCR Corporation

Q. What are interfaces and what is difference between an interface and abstract class
Interfaces define a contract for behavior, while abstract classes provide partial implementation.
Interfaces only define method signatures, while abstract classes can have both abstract and concrete methods.
A class can implement multiple interfaces, but can only inherit from one abstract class.
Interfaces are used for loose coupling and flexibility in design.
Abstract classes are used for code reuse and to enforce a common implementation among subclasses.

Asked in Moonfrog Labs

Q. Given an array arr[] containing N integers, print the next greater element for every element.
Given an array, print the next greater element for every element.
Iterate through the array from right to left
Use a stack to keep track of the greater elements
Pop elements from the stack until a greater element is found or the stack is empty
If a greater element is found, it is the next greater element for the current element
If the stack is empty, there is no greater element for the current element
Store the next greater element in a result array
Reverse the result array to get t...read more
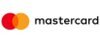
Asked in MasterCard

Q. If I give you a coding problem to solve in a language you don’t know, can you solve it if you are allowed to use Google search?
Yes, I can solve a coding problem in a language I don't know with Google search.
Utilize Google search to understand syntax and concepts in the unfamiliar language
Break down the problem into smaller parts and search for solutions to each part
Refer to online resources, forums, and documentation for guidance
Practice writing and testing code snippets in the new language before attempting the full solution
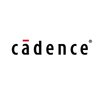
Asked in Cadence Design Systems

Q. What is the difference between C++ and Objective-C, and where would you use each?
C++ is a general-purpose programming language while Objective C is a superset of C used for iOS and macOS development.
C++ is widely used for developing applications, games, and system software.
Objective C is primarily used for iOS and macOS development.
C++ supports both procedural and object-oriented programming paradigms.
Objective C is an object-oriented language with dynamic runtime features.
C++ has a larger standard library compared to Objective C.
Objective C uses a Smallt...read more
Software Engineer II Jobs



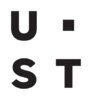
Asked in UST

Q. How do you find the sum of even numbers from a given ArrayList using the Stream API?
Using stream API to find the sum of even numbers from an ArrayList
Convert the ArrayList to a stream using the stream() method
Filter the stream to keep only the even numbers using the filter() method
Use the mapToInt() method to convert the stream of even numbers to an IntStream
Finally, use the sum() method to calculate the sum of the even numbers
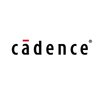
Asked in Cadence Design Systems

Q. What is the difference between class container and class composition?
Class container is a class that holds objects of other classes, while class composition is a way to combine multiple classes to create a new class.
Class container holds objects of other classes, acting as a collection or container.
Class composition combines multiple classes to create a new class with its own behavior and attributes.
In class container, the objects are typically stored in a data structure like an array or a list.
In class composition, the classes are combined by...read more
Share interview questions and help millions of jobseekers 🌟
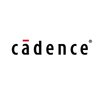
Asked in Cadence Design Systems

Q. What is a malloc function, where is it used, and how does it differ from new?
malloc is a function used in C programming to dynamically allocate memory. It is used in low-level programming and is different from new.
malloc is used to allocate memory on the heap in C programming.
It is used when the size of memory needed is not known at compile time.
malloc returns a void pointer to the allocated memory block.
Example: int* ptr = (int*) malloc(5 * sizeof(int));
new is used in C++ programming to dynamically allocate memory.
It is used with classes and objects ...read more
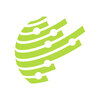
Asked in kipi.ai

Q. What is the difference between a stored procedure and a function?
Stored procedures are used to perform a set of actions, while functions return a single value.
Stored procedures are precompiled and stored in the database, while functions are compiled at runtime.
Functions can be used in SQL statements, while stored procedures cannot be used in SQL statements.
Functions can be called from within stored procedures, but stored procedures cannot be called from within functions.
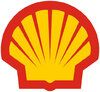
Asked in Shell

Q. What was most challenging development and why you opted for customization over OOB feature of Salesforce. Lightning and sales cloud questions.
Developing a custom solution for complex business processes in Sales Cloud
The business process required complex automation and customization
The OOB features of Sales Cloud were not sufficient to meet the requirements
Developed custom Lightning components and Apex code to automate the process
The solution was thoroughly tested and validated before deployment
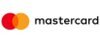
Asked in MasterCard

Q. What are software development design patterns? Can you explain singleton design pattern and code an example to show that?
Software development design patterns are reusable solutions to common problems encountered in software design.
Design patterns help in creating maintainable, scalable, and efficient software.
Singleton design pattern ensures a class has only one instance and provides a global point of access to it.
Example: Implementing a singleton class in Java -
public class Singleton { private static Singleton instance; private Singleton() {} public static Singleton getInstance() { if(instance...read more
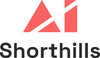
Asked in Shorthills AI

Q. Write a program in your preferred language to perform several core programming tasks.
I would use Python to perform basic programming tasks such as sorting, searching, and manipulating data structures.
Use built-in functions like sorted() and filter() for sorting and filtering data
Use loops and conditional statements for searching and manipulating data structures
Use libraries like NumPy and Pandas for advanced data manipulation and analysis
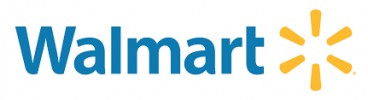
Asked in Walmart

Q. Round 1 : DSA: 1)print all pairs with a target sum in an array (with frequency of elements). 2) Array to BST. 3) Top view of Binary Tree. 4) Minimum number of jumps to reach the end of an array
DSA questions on array and binary tree manipulation
For printing pairs with target sum, use a hash table to store frequency of elements and check if complement exists
For converting array to BST, use binary search to find the middle element and recursively build left and right subtrees
For top view of binary tree, use a queue to traverse the tree level by level and store horizontal distance of nodes
For minimum number of jumps, use dynamic programming to find minimum jumps requir...read more
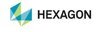
Asked in Hexagon Capability Center

Q. What is the difference between virtual and override keywords?
Virtual keyword is used to declare a method in a base class that can be overridden in a derived class. Override keyword is used in the derived class to override the implementation of the virtual method.
Virtual keyword is used in the base class to declare a method that can be overridden in the derived class
Override keyword is used in the derived class to override the implementation of the virtual method
Virtual methods provide a way for a base class to define a default implemen...read more
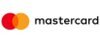
Asked in MasterCard

Q. How can you parse location and shop name from a card transaction string?
Use regex to extract location and shop name from transaction string.
Use regular expressions to match patterns for location and shop name.
Look for keywords like 'at', 'from', 'in' to identify location.
Consider variations in transaction strings and account for them in regex patterns.
Example: 'Spent $50 at Starbucks Coffee' -> Location: Starbucks Coffee, Shop Name: Starbucks Coffee
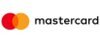
Asked in MasterCard

Q. What kind of databases you’ve worked on? And explain what is DML!
I have worked on relational databases like MySQL and PostgreSQL. DML stands for Data Manipulation Language.
Worked on MySQL and PostgreSQL databases
Familiar with writing SQL queries for data manipulation
DML stands for Data Manipulation Language

Asked in JPMorgan Chase & Co.

Q. You are provided with a stream of trade data for various companies, including the number of trades, prices, and additional information. How do you calculate the profit and loss for each company?
Calculate profit and loss by analyzing trade data for each company based on trades and prices.
Aggregate trade data by company to get total trades and prices.
Calculate total revenue by multiplying the number of trades by the trade price.
Determine costs if available; otherwise, assume a baseline cost.
Profit/Loss = Total Revenue - Total Costs.
Example: If Company A has 100 trades at $10 each, revenue = 1000. If costs = 800, profit = 200.
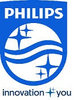
Asked in Philips Innovation Campus

Q. 1. Difference between abstract and interface 2. OOPs concepts with examples 3. Solid principles 4. some Coding questions
Interview questions for Software Engineer II
Abstract class is a class that cannot be instantiated and can have both abstract and non-abstract methods. Interface is a blueprint of a class that only has abstract methods.
OOPs concepts include inheritance, polymorphism, encapsulation, and abstraction. Example: Inheritance - a child class inheriting properties and methods from a parent class.
SOLID principles are Single Responsibility, Open-Closed, Liskov Substitution, Interface Se...read more
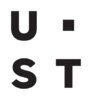
Asked in UST

Q. Which interface is used to prevent SQL injection?
Prepared Statements interface is used to prevent SQL injection.
Prepared Statements interface is used to parameterize the SQL queries.
It allows the separation of SQL code and user input.
It helps to prevent SQL injection attacks by automatically escaping special characters.
Examples: PDO, mysqli, Java PreparedStatement, etc.
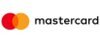
Asked in MasterCard

Q. Can you develop a simple API app that shows it is running and responds to requests?
A simple API app can be built using Flask to handle requests and display its status.
Use Flask to create a basic web server: `from flask import Flask`.
Define a route to handle GET requests: `@app.route('/status')`.
Return a JSON response indicating the API is running: `return {'status': 'running'}`.
Run the app with `app.run(debug=True)` to enable debugging.
Test the API using tools like Postman or curl.
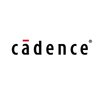
Asked in Cadence Design Systems

Q. How do you calculate the width of a tree?
The width of a tree can be calculated by finding the maximum number of nodes at any level.
Traverse the tree level by level using breadth-first search
Keep track of the maximum number of nodes at any level
Return the maximum number of nodes as the width of the tree
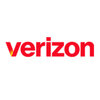
Asked in Verizon

Q. What is Redux, and what are its use cases in class-based and functional components? Please provide examples.
Redux is a predictable state container for JavaScript apps.
Redux is used for managing the state of an application.
It is commonly used with React to manage the state of components.
Redux uses a single store to manage the state of an entire application.
Actions are dispatched to the store to update the state.
Reducers are used to update the state based on the dispatched actions.
Functional components can use the useSelector hook to access the state.
Class components can use the conn...read more
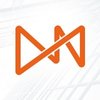
Asked in Vertafore

Q. ConcurrentModificationException. Vertical Scaling vs Horizontal Scaling. Roles and responsibilties in previous org. Strengths and weakness. Describe a situation where you became angry.
Questions on software engineering concepts, previous roles and responsibilities, and personal strengths and weaknesses.
ConcurrentModificationException: occurs when a collection is modified while being iterated over
Vertical Scaling vs Horizontal Scaling: vertical scaling involves adding more resources to a single machine, while horizontal scaling involves adding more machines to a system
Roles and responsibilities in previous org: discuss previous job titles and duties
Strengths...read more
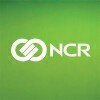
Asked in NCR Corporation

Q. What are SOLID principles, and can you explain them?
SOLID principles are a set of five design principles for writing maintainable and scalable code.
Single Responsibility Principle (SRP) - a class should have only one reason to change
Open-Closed Principle (OCP) - a class should be open for extension but closed for modification
Liskov Substitution Principle (LSP) - subtypes should be substitutable for their base types
Interface Segregation Principle (ISP) - clients should not be forced to depend on interfaces they do not use
Depend...read more
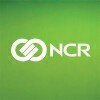
Asked in NCR Corporation

Q. Write a program to find the second largest element from a 4-digit number. For example, if the input is 1245, the program should return 4.
Program to find the second largest element from a 4-digit number
Convert the number to a string and split it into an array of characters
Sort the array in descending order
Return the second element of the array

Asked in XpressBees

Q. Given an array of integers, return indices of the two numbers such that they add up to a specific target. You may assume that each input would have exactly one solution, and you may not use the same element twi...
read moreFind indices of two numbers in an array that sum up to a given target.
Use a hash map to store the difference between the target and each element's value.
Iterate through the array and check if the current element exists in the hash map.
If it exists, return the indices of the current element and the element from the hash map.
Example: For array [2, 7, 11, 15] and target 9, return [0, 1] since 2 + 7 = 9.
Asked in Koo App

Q. 1. How is session authenticated in facebook and other websites when we login? 2. How to store large databases? Hashing related.
Session authentication in websites and storing large databases using hashing.
Session authentication in websites involves creating a unique session ID for each user upon login and storing it in a cookie or server-side session storage.
The session ID is then used to authenticate subsequent requests from the user.
Large databases can be stored using hashing techniques such as sharding, consistent hashing, and hash tables.
Sharding involves dividing the database into smaller partiti...read more

Asked in Fareportal

Q. What is a singleton design pattern?
Singleton design pattern restricts the instantiation of a class to a single instance and provides a global point of access to it.
Used when only one instance of a class is required throughout the system
Provides a global point of access to the instance
Implemented using a private constructor, static method, and static variable
Example: Logger class, Database connection class

Asked in Kaplan

Q. SOLID design principles and Design Patterns use cases
SOLID design principles and Design Patterns are used to create maintainable and scalable software.
SOLID principles help in creating loosely coupled and modular code.
Design Patterns provide reusable solutions to common software problems.
Examples of SOLID principles include Single Responsibility, Open/Closed, and Liskov Substitution.
Examples of Design Patterns include Factory, Singleton, and Observer.
Using SOLID principles and Design Patterns can improve code quality, reduce bu...read more
Interview Experiences of Popular Companies
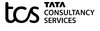
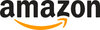
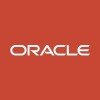

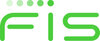
Top Interview Questions for Software Engineer II Related Skills
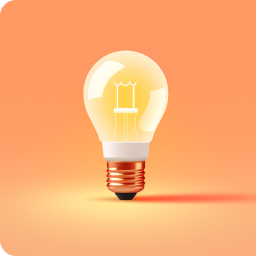
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
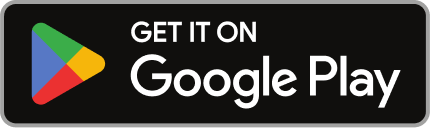
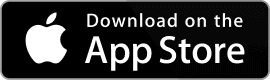
Reviews
Interviews
Salaries
Users
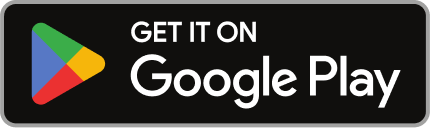
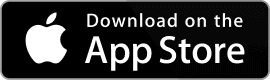