React Js Frontend Developer
70+ React Js Frontend Developer Interview Questions and Answers for Freshers
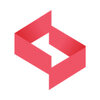
Asked in Simform

Q. 1. What is difference between abstract class and interface ?
Abstract class can have implementation while interface cannot. A class can implement multiple interfaces but only extend one abstract class.
Abstract class can have constructors while interface cannot.
Abstract class can have non-abstract methods while interface can only have abstract methods.
A class can implement multiple interfaces but only extend one abstract class.
Abstract class can have instance variables while interface cannot.
Abstract class is used for code reusability w...read more
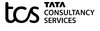
Asked in TCS

Q. What is the difference between a primary key and a unique key?
Primary key uniquely identifies a record in a table, while unique key ensures that all values in a column are distinct.
Primary key is used to enforce entity integrity, while unique key enforces domain integrity.
A table can have only one primary key, but multiple unique keys.
Primary key can't have null values, while unique key can have null values.
Primary key is automatically indexed, while unique key may or may not be indexed.
Asked in Plan.Net TechNest

Q. What is an arrow function in JavaScript?
Arrow functions are a concise way to write functions in JavaScript.
Arrow functions have a shorter syntax compared to regular functions.
They do not have their own 'this' value, instead, they inherit 'this' from the surrounding context.
Arrow functions are always anonymous and cannot be used as constructors.
They are commonly used in React components for event handlers and callback functions.
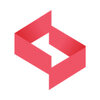
Asked in Simform

Q. 5. Why we require interface and what is interface in java ?
Interfaces in Java provide a way to achieve abstraction and multiple inheritance.
Interfaces define a set of methods that a class must implement.
They allow for loose coupling between classes.
Interfaces can be used to achieve polymorphism.
Java does not support multiple inheritance, but interfaces provide a way to achieve it.
Interfaces are used extensively in Java frameworks like Spring and Hibernate.
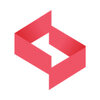
Asked in Simform

Q. How do you join three different tables in SQL?
To join three different tables in SQL, you can use the JOIN keyword along with the appropriate join conditions.
Use the JOIN keyword to combine tables based on a common column
Specify the join conditions using the ON keyword
You can join more than two tables by chaining multiple JOIN statements
Different types of joins include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN
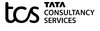
Asked in TCS

Q. What is the difference between overloading and overriding?
Overloading is having multiple methods with the same name but different parameters. Overriding is implementing a method in a subclass that already exists in the parent class.
Overloading is compile-time polymorphism while overriding is runtime polymorphism.
Overloading is used to provide different ways of calling the same method with different parameters.
Overriding is used to provide a specific implementation of a method in a subclass that is already defined in the parent class...read more
React Js Frontend Developer Jobs
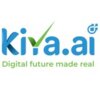
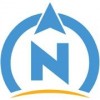
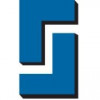
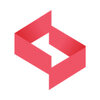
Asked in Simform

Q. Is exception handling required in a large project, or is it optional?
Yes, exception handling is required in a big project.
Exception handling helps in identifying and resolving errors during runtime.
It improves the stability and reliability of the application.
Exceptions can occur due to various reasons like network issues, server errors, or user input errors.
Handling exceptions gracefully prevents the application from crashing and provides a better user experience.
It allows for proper error logging and debugging, making it easier to identify an...read more
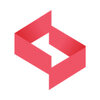
Asked in Simform

Q. What are the access specifiers in Java?
Access specifiers in Java are keywords that determine the visibility of a class, method, or variable.
There are four access specifiers in Java: public, private, protected, and default.
Public: accessible from anywhere in the program.
Private: accessible only within the same class.
Protected: accessible within the same class, subclasses, and same package.
Default: accessible within the same package only.
Share interview questions and help millions of jobseekers 🌟
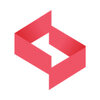
Asked in Simform

Q. What is Encapsulation in Java, and can you provide an example?
Encapsulation is a mechanism of wrapping data and code acting on the data together as a single unit.
Encapsulation is used to hide the implementation details of an object from the outside world.
It helps in achieving data abstraction and data hiding.
In Java, encapsulation is achieved by declaring the variables of a class as private and providing public getter and setter methods to access and modify the data.
Example: A bank account class with private variables like account numbe...read more
Asked in Softops Technologies

Q. How to find index of the 3rd element of an array like let arr=["one", "two", "three", "four", "five"] Now find the index of 5th element of an array without using index of method
Finding index of elements in an array in JavaScript
To find the index of the 3rd element, use arr.indexOf('three')
To find the index of the 5th element without using indexOf, use arr[4]
Arrays in JavaScript are zero-indexed, so the 5th element is at index 4
Asked in Dcj Group

Q. What are closures in JavaScript and how do they work? Additionally, what is hoisting in JavaScript, and how does it behave with different variable declarations such as var, let, and const? Furthermore, what is...
read moreClosures capture variable scope, hoisting affects variable declarations, and call by reference vs. value defines how data is passed.
Closures are functions that retain access to their lexical scope even when executed outside that scope.
Example of closure: function outer() { let x = 10; return function inner() { return x; }; } const innerFunc = outer(); innerFunc(); // returns 10
Hoisting is JavaScript's behavior of moving declarations to the top of their containing scope during...read more
Asked in Dcj Group

Q. What are the hooks in React.js, and what is the difference between the useState hook and the useReducer hook? Additionally, can you describe your experience creating a shopping cart during the technical round?
Hooks in React allow functional components to manage state and side effects, enhancing component functionality.
Hooks are functions that let you use state and other React features in functional components.
useState: A hook that allows you to add state to functional components. Example: const [count, setCount] = useState(0);
useReducer: A hook for managing complex state logic, similar to Redux. Example: const [state, dispatch] = useReducer(reducer, initialState);
useState is simpl...read more
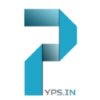
Asked in PyPs In Technologies

Q. Create an Admin (child component) with a form. The data submitted by the form should be displayed on the parent component (app.js).
Create an Admin component with a form that submits data to the parent component.
Create a child component called Admin
Add a form to the Admin component
Handle form submission in the Admin component
Pass the submitted data to the parent component using props
Render the submitted data in the parent component
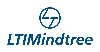
Asked in LTIMindtree

Q. what is closuer? is normal function call as closuer or not
A closure is a function that has access to its own scope, as well as the scope in which it was defined.
A closure allows a function to access variables from its outer function even after the outer function has finished executing.
Closures are created whenever a function is defined within another function.
Example: function outerFunction() { let outerVar = 'I am outer'; return function innerFunction() { console.log(outerVar); }; }
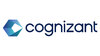
Asked in Cognizant

Q. What is lazy loading and how can we implement it in our project?
Lazy loading is a technique used to defer loading non-essential resources until they are needed.
Lazy loading helps improve performance by only loading resources when they are required.
In React, lazy loading can be implemented using React.lazy() and Suspense components.
Example: const MyComponent = React.lazy(() => import('./MyComponent'));
Example:
Loading...}>
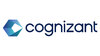
Asked in Cognizant

Q. What is React routing, and how does it differ from conventional routing methods?
React routing is a way to handle navigation in a React application by defining routes and rendering components based on the URL.
React routing allows for declarative routing, where routes are defined using JSX elements.
It enables dynamic routing based on the URL, allowing for different components to be rendered based on the route.
React Router is a popular library for handling routing in React applications.
Unlike conventional routing methods, React routing is more efficient as ...read more
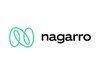
Asked in Nagarro

Q. How do you convert from portrait mode to landscape mode using CSS?
CSS can be used to adapt layouts for portrait and landscape orientations using media queries and flexible units.
Media Queries: Use CSS media queries to apply different styles based on the orientation. Example: `@media (orientation: landscape) { /* styles */ }`.
Flexbox: Utilize flexbox properties to create responsive layouts that adjust based on the screen orientation. Example: `display: flex; flex-direction: row;` for landscape.
Grid Layout: CSS Grid can help rearrange element...read more
Asked in Kiaan Technology

Q. What are React Hooks and how do they function within React applications?
React Hooks are functions that let you use state and other React features without writing a class.
Hooks allow functional components to manage state and lifecycle events.
Common hooks include useState for state management and useEffect for side effects.
Example of useState: const [count, setCount] = useState(0);
Example of useEffect: useEffect(() => { document.title = `Count: ${count}`; }, [count]);
Custom hooks can be created to encapsulate reusable logic.
Asked in Kiaan Technology

Q. What is the difference between viewport width (vw), pixels (px), and allCSS units?
Viewport width (vw), pixels (px), and other CSS units differ in how they measure size and responsiveness in web design.
1. Pixels (px): Absolute unit; 1px is 1/96th of an inch. Example: width: 100px sets a fixed width.
2. Viewport Width (vw): Relative unit; 1vw is 1% of the viewport's width. Example: width: 50vw sets width to 50% of the viewport.
3. Other CSS units: Includes em, rem, percentages, etc. Example: width: 10em is based on the font size of the element.
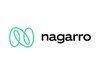
Asked in Nagarro

Q. What is the difference between writing a custom hook as a React component and as a function?
Custom hooks can be created as functions or components, each serving different purposes in React.
Custom hooks as functions: They encapsulate reusable logic and can use other hooks internally.
Example: function useFetch(url) { const [data, setData] = useState(null); useEffect(() => { fetch(url).then(...); }, [url]); return data; }
Custom hooks as components: They can manage state and lifecycle methods but are less common and can lead to confusion.
Example: function FetchComponent...read more
Asked in Numeric Infosystems

Q. For loop, event listener in react,what is usesState , what is use effect ,hooks in react
useState and useEffect are hooks in React used for managing state and side effects respectively.
useState is a hook used to add state to functional components in React
useEffect is a hook used to perform side effects in functional components
Hooks in React allow functional components to have state and lifecycle methods similar to class components
Asked in Aisensy Communications

Q. What will be the output of the following code? async function run(data){ await console.log(data); } console.log(1); run(2); console.log(3);
The output will be 1, 2, 3 in order.
The console.log(1) statement will be executed first, printing 1 to the console.
The run(2) function will be called asynchronously, but since it is using await, it will wait for the console.log(2) statement to finish before moving on.
The console.log(3) statement will be executed last, printing 3 to the console.
Asked in Softops Technologies

Q. Q1 How to find factorial of the number
Factorial of a number is the product of all positive integers up to that number.
Use a loop to multiply all positive integers up to the given number.
If the given number is 0 or 1, return 1.
Recursively call the function to find the factorial of the given number.
Handle negative numbers by returning undefined or throwing an error.
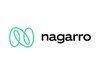
Asked in Nagarro

Q. What are meta tags in HTML? What is the importance of viewport?
Meta tags provide metadata about the HTML document, influencing SEO and responsive design, including viewport settings.
Meta tags are placed in the <head> section of an HTML document.
They provide information like character set, author, and description.
Example: <meta name='description' content='This is a sample webpage.'>
Viewport meta tag controls layout on mobile browsers.
Example: <meta name='viewport' content='width=device-width, initial-scale=1.0'>
Proper viewport settings en...read more
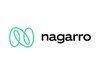
Asked in Nagarro

Q. What are useTransition and useDeferred hooks in React?
useTransition and useDeferredValue are hooks for managing UI transitions and deferred updates in React.
useTransition allows you to mark updates as non-urgent, improving user experience during state changes.
Example: const [isPending, startTransition] = useTransition();
useDeferredValue lets you defer a value update until the browser is idle, preventing blocking UI.
Example: const deferredValue = useDeferredValue(value);
Both hooks help in optimizing rendering performance and main...read more
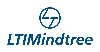
Asked in LTIMindtree

Q. What is the difference between Redux and Redux Toolkit?
Redux Toolkit is a set of tools and best practices to simplify Redux development, while Redux is a predictable state container for JavaScript apps.
Redux Toolkit provides a set of tools like createSlice, createAsyncThunk, and configureStore to simplify Redux setup and reduce boilerplate code.
Redux Toolkit includes immer library for writing immutable update logic in a more convenient way.
Redux Toolkit also includes a default setup for Redux DevTools Extension integration.
Redux ...read more
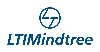
Asked in LTIMindtree

Q. What are inline-level and block-level elements?
Inline and block level elements are two types of HTML elements with different display behaviors.
Inline elements flow in the same line as surrounding content, while block level elements take up the full width available.
Examples of inline elements include <span>, <a>, and <strong>.
Examples of block level elements include <div>, <p>, and <h1>.
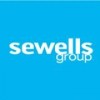
Asked in Sewells Group

Q. What are access modifiers in programming?
Access modifiers are keywords in programming that define the accessibility of classes, methods, and variables.
Access modifiers control the visibility and accessibility of classes, methods, and variables within a program.
Common access modifiers include public, private, protected, and default (package-private).
Public access modifier allows the class, method, or variable to be accessed from any other class.
Private access modifier restricts access to only within the same class.
Pr...read more
Asked in Praeclarum Tech

Q. Tell me difference between Let, Var, Cost and
Let, var, and const are used to declare variables in JavaScript, with differences in scope and mutability.
let: block-scoped, can be reassigned
var: function-scoped, can be reassigned
const: block-scoped, cannot be reassigned
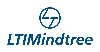
Asked in LTIMindtree

Q. What are React Hooks? Explain some of them.
React Hooks are functions that let you use state and other React features without writing a class.
React Hooks were introduced in React 16.8.
They allow you to use state and other React features in functional components.
Some commonly used hooks are useState, useEffect, useContext, and useRef.
Hooks make it easier to reuse logic across components.
Hooks can be used to manage component state, perform side effects, and more.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
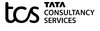
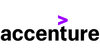
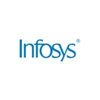
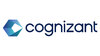
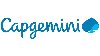
Top Interview Questions for React Js Frontend Developer Related Skills
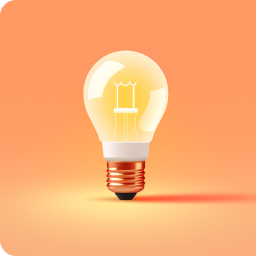
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
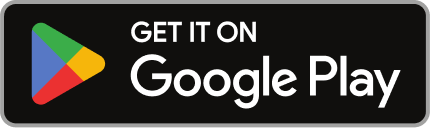
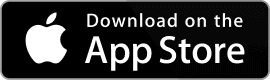
Reviews
Interviews
Salaries
Users
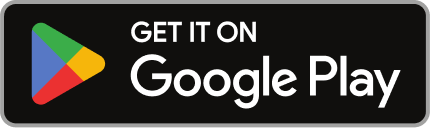
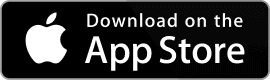