Programmer Analyst
400+ Programmer Analyst Interview Questions and Answers
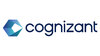
Asked in Cognizant

Q. What is meant by Dependency Injection in .NET?
Dependency injection is a design pattern used to remove dependencies between objects and make them more modular.
It allows objects to be created and configured externally, rather than within a class.
It helps to reduce coupling between classes and makes code more testable.
There are three types of dependency injection: constructor injection, property injection, and method injection.
Example: Instead of creating a database connection object within a class, it can be injected from ...read more
Asked in Strada Global

Q. What is the difference between method overloading and overriding?
Method overloading is having multiple methods with the same name but different parameters, while method overriding is having a subclass method with the same name and parameters as the superclass method.
Method overloading is used to provide different ways of calling the same method with different parameters.
Method overriding is used to provide a specific implementation of a method in a subclass that is already defined in the superclass.
Method overloading is resolved at compile...read more
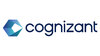
Asked in Cognizant

Q. How would you implement moving images on Flipkart?
Moving images can be added in Flipkart by using HTML and CSS animations.
Use HTML
tag to insert the image
Apply CSS animations to create the moving effect
Use keyframes to define the animation behavior

Asked in Fidelity International

Q. What do you do when a batch fails in production?
I would investigate the cause of the failure and take appropriate actions to resolve it.
Check the error logs to identify the root cause of the failure
Determine if the issue can be resolved by restarting the batch or if a code fix is required
Communicate the issue and resolution plan to relevant stakeholders
Implement the fix and re-run the batch
Perform post-mortem analysis to identify ways to prevent similar failures in the future
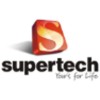
Asked in Supertech

Q. If you could rebuild the brand from the start, how would you do it?
I would focus on creating a strong brand identity and building a loyal customer base.
Develop a clear brand message and mission statement
Create a visually appealing and memorable logo
Offer exceptional customer service to build loyalty
Utilize social media and other marketing channels to reach target audience
Collaborate with influencers and industry leaders to increase brand visibility
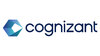
Asked in Cognizant

Q. Can you mention some data structures? Explain Stack and Queue?
Data structures are ways to organize and store data. Stack and Queue are two commonly used data structures.
Stack is a Last-In-First-Out (LIFO) data structure where the last element added is the first one to be removed.
Queue is a First-In-First-Out (FIFO) data structure where the first element added is the first one to be removed.
Stack is used in undo-redo functionality, while Queue is used in scheduling tasks.
Examples of Stack include browser history, call stack in programmin...read more
Programmer Analyst Jobs
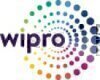
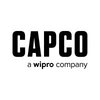
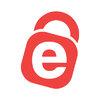
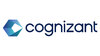
Asked in Cognizant

Q. Tell me what you know about AWS cloud.
AWS cloud is a platform that provides a wide range of cloud computing services.
AWS stands for Amazon Web Services
It offers services like computing, storage, databases, analytics, and more
AWS provides a pay-as-you-go pricing model
Examples of AWS services include EC2, S3, RDS, and Lambda
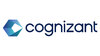
Asked in Cognizant

Q. What are OOPS concepts and how can they be used in problem solving?
OOPS concepts refer to Object-Oriented Programming principles like inheritance, encapsulation, polymorphism, and abstraction.
Inheritance allows a class to inherit properties and behavior from another class.
Encapsulation involves bundling data and methods that operate on the data into a single unit.
Polymorphism allows objects of different classes to be treated as objects of a common superclass.
Abstraction focuses on hiding the implementation details and showing only the necess...read more
Share interview questions and help millions of jobseekers 🌟
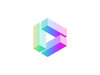
Asked in Metawallah

Q. Which types of testing are important for web testing?
Functional, Performance, Security and Compatibility testing are important for web testing.
Functional testing ensures that the website functions as expected
Performance testing checks the website's speed and response time
Security testing checks for vulnerabilities and ensures data protection
Compatibility testing ensures the website works on different browsers and devices
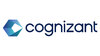
Asked in Cognizant

Q. Write a program to swap two variables without using temporary variables.
Swapping two variables without using temporary variables.
Use arithmetic operations to swap values
X = X + Y
Y = X - Y
X = X - Y
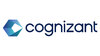
Asked in Cognizant

Q. How do you find the inverse of a number given the difference and sum of its digits?
To find the inverse of a number, calculate the difference and sum of its digits.
Iterate through each digit of the number to calculate the sum and difference.
Reverse the order of the digits to get the inverse number.
For example, if the number is 123, the sum of digits is 1+2+3=6 and the difference is 3-2-1=0. The inverse would be 321.
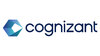
Asked in Cognizant

Q. what do you mean by machine learning , how you implemented your project .
Machine learning is a subset of AI that enables machines to learn from data and improve their performance.
Machine learning involves training algorithms to make predictions or decisions based on data.
Supervised learning involves training a model on labeled data to make predictions on new data.
Unsupervised learning involves finding patterns in unlabeled data.
Reinforcement learning involves training a model to make decisions based on rewards or punishments.
Examples of machine le...read more
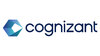
Asked in Cognizant

Q. Write a LINQ query to find all the employees with a salary greater than 25000.
LINQ query to find employees with salary > 25000
Use the 'where' clause to filter employees based on salary
Specify the condition for salary greater than 25000
Select the required employee details using 'select'
Example: var result = employees.Where(e => e.Salary > 25000).Select(e => new { e.Name, e.Department });
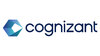
Asked in Cognizant

Q. Do you know Python? Solve the following code and explain the output.
I am familiar with Python and can solve the given code snippet to determine the output.
Identify the code snippet provided
Analyze the syntax and logic used in the code
Execute the code in a Python environment to determine the output
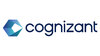
Asked in Cognizant

Q. What is NoSQL and how is it different from RDBMS?
NoSQL is a non-relational database that stores and retrieves data in a flexible and scalable manner.
NoSQL databases are schema-less and do not require a fixed table structure like RDBMS.
NoSQL databases are horizontally scalable, meaning they can handle large amounts of data and traffic.
Examples of NoSQL databases include MongoDB, Cassandra, and Redis.
RDBMS databases are vertically scalable, meaning they can handle more traffic by adding more resources to a single server.
Examp...read more
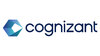
Asked in Cognizant

Q. Write a program in Java to find the middle element in a list.
Java program to find the middle element in a list.
Create a list and add elements to it.
Find the size of the list using size() method.
Calculate the middle index using size()/2.
Retrieve the middle element using get() method.
Handle cases where the list has even number of elements.
Asked in SatNav Technologies

Q. What do you know about Networking?
Networking involves connecting devices to share resources and communicate with each other.
Networking is the practice of linking devices together to share resources and information.
It involves protocols such as TCP/IP, DNS, and DHCP.
Networking can be wired or wireless, and can be used for local or wide area connections.
Examples of networking devices include routers, switches, and modems.
Networking is essential for internet connectivity and cloud computing.
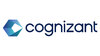
Asked in Cognizant

Q. What is the logic behind tree and graph data structures?
Trees and graphs are used to represent hierarchical and non-linear relationships respectively in data structures and algorithms.
Trees are used to represent hierarchical relationships between data points, such as in file systems or organizational structures.
Graphs are used to represent non-linear relationships between data points, such as in social networks or transportation systems.
Both trees and graphs can be traversed using various algorithms, such as depth-first search or ...read more
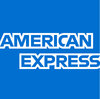
Asked in American Express

Q. How many traffic signals are there in Mumbai?
It is not possible to determine the exact number of traffic signals in Mumbai without available data.
The number of traffic signals in Mumbai can vary over time due to construction, removal, or changes in traffic management.
The Mumbai Traffic Police or Municipal Corporation may have the most accurate data on the number of traffic signals.
Estimating the number of traffic signals in Mumbai would require a comprehensive survey or analysis of the city's road network.
Factors such a...read more
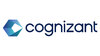
Asked in Cognizant

Q. What is the time complexity of a nested for loop?
Time complexity of nested for loop.
The time complexity is O(n^k) where n is the size of the outer loop and k is the size of the inner loop.
Nested loops can lead to exponential time complexity.
Optimizing the inner loop can improve performance.
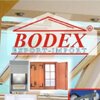
Asked in BODEX

Q. what are types of functions in js and how does they work!
Types of functions in JavaScript include named functions, anonymous functions, arrow functions, and immediately invoked function expressions (IIFE).
Named functions are defined with a function keyword followed by a function name.
Anonymous functions are defined without a function name.
Arrow functions provide a more concise syntax for writing functions.
IIFE functions are executed immediately after they are defined.
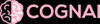
Asked in CognAI

Q. What is the difference between regression testing and sanity testing?
Regression testing is to ensure that changes made to the software do not affect existing functionality. Sanity testing is to ensure that the software is stable enough for further testing.
Regression testing is done after changes are made to the software to ensure that existing functionality is not affected.
Sanity testing is done to ensure that the software is stable enough for further testing.
Regression testing is a type of functional testing while sanity testing is a type of ...read more
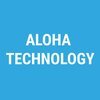
Asked in Aloha Technology

Q. Write a program in your preferred language.
I wrote a program in Python to calculate the factorial of a number.
Used a while loop to multiply the number with its previous value until it reaches 1
Implemented error handling for negative numbers and non-integer inputs
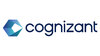
Asked in Cognizant

Q. What is space complexity?
Space complexity refers to the amount of memory used by an algorithm.
It is a measure of how much memory an algorithm needs to solve a problem.
It is usually expressed in terms of the amount of memory used by the algorithm as a function of the size of the input.
Space complexity can be affected by the data structures used in the algorithm.
For example, an algorithm that uses a hash table will have a higher space complexity than one that uses a simple array.
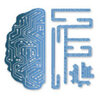
Asked in Feynn Labs

Q. What is the difference between supervised learning and unsupervised learning?
Supervised learning uses labeled data to train a model, while unsupervised learning uses unlabeled data to find patterns.
Supervised learning requires a target variable to be predicted, while unsupervised learning does not.
Supervised learning algorithms include linear regression, logistic regression, and decision trees.
Unsupervised learning algorithms include clustering, principal component analysis, and anomaly detection.
Supervised learning is used in applications such as ima...read more
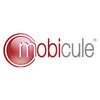
Asked in Mobicule Technologies

Q. 1.What is android activity life cycle. 2.What is collections in java. ..etc
Android activity life cycle is the sequence of states an activity goes through.
The life cycle includes states like onCreate(), onStart(), onResume(), onPause(), onStop(), and onDestroy().
Each state has a specific purpose and can be used to manage the activity's behavior.
Collections in Java are data structures that store and manipulate groups of objects.
Examples of collections include ArrayList, LinkedList, HashSet, and HashMap.
Collections provide methods for adding, removing,...read more
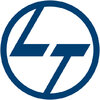
Asked in Larsen & Toubro Limited

Q. What is the value of the three-sigma limit in statistics?
The three-sigma limit in statistics is a range that includes 99.7% of the data points in a normal distribution.
The three-sigma limit is also known as the 99.7% confidence interval.
It is calculated by adding and subtracting three times the standard deviation from the mean.
For example, if a data set follows a normal distribution with a mean of 50 and a standard deviation of 5, the three-sigma limit would be between 35 and 65.
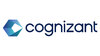
Asked in Cognizant

Q. Implement a min stack program in 5 minutes.
A min stack program is implemented to find the minimum element in a stack in constant time.
Create two stacks, one for storing the elements and the other for storing the minimum values.
Push the elements onto the stack and also push the minimum value onto the minimum stack if it is smaller than the current minimum.
When popping an element from the stack, also pop the top element from the minimum stack if it is the same as the popped element.
The minimum value can be obtained by p...read more
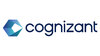
Asked in Cognizant

Q. If given the name 'pulsar', the function should return 'bike'; otherwise, it should return 'car'. Can you implement this function?
This function checks if the input name is 'pulsar' and returns 'bike' or 'car' accordingly.
Use a simple conditional statement to check the name.
Example: If name == 'pulsar', return 'bike'.
Else, return 'car'.
This can be implemented in various programming languages.
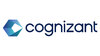
Asked in Cognizant

Q. what is linked list and array list what is your preference for data manupulation and all
Linked list and array list are data structures used for storing and manipulating collections of data. Linked list allows for dynamic size and efficient insertion/deletion, while array list provides fast random access.
Linked list is a data structure where each element points to the next element in the list. Example: singly linked list, doubly linked list.
Array list is a data structure that stores elements in contiguous memory locations. Example: ArrayList in Java, List in C#.
L...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
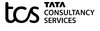
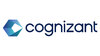
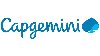
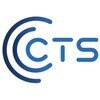
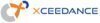
Top Interview Questions for Programmer Analyst Related Skills
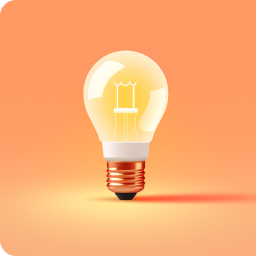
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
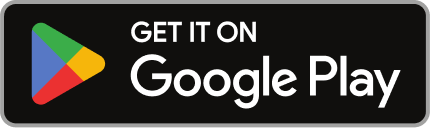
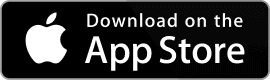
Reviews
Interviews
Salaries
Users
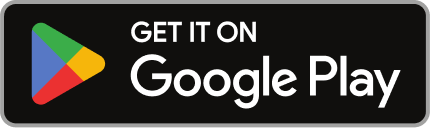
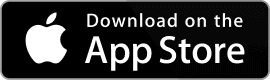