Fullstack Javascript Developer
10+ Fullstack Javascript Developer Interview Questions and Answers
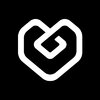
Asked in Fynd

Q. Write code to print numbers 1 to n where each number i should get printed after i seconds. Questions around let and var on the solution. Promisify the solution.
Print numbers 1 to n with a delay of i seconds for each number.
Use a loop to iterate from 1 to n
Use setTimeout function to delay the printing of each number
Use let instead of var to avoid closure issues
Promisify the solution by returning a promise
Asked in Rablo.in

Q. What is the difference between let, var, and const?
let, var and const are all used to declare variables in JavaScript, but they differ in their scope and mutability.
let has block scope and can be reassigned
var has function scope and can be reassigned
const has block scope and cannot be reassigned, but its properties can be mutated
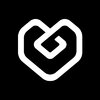
Asked in Fynd

Q. Given two linked lists, find the intersection node if they intersect.
To find the intersection node of two linked lists, iterate through both lists and compare each node until a common node is found.
Iterate through both linked lists simultaneously
Compare each node of the lists until a common node is found
If the lists have different lengths, align the starting points by advancing the longer list
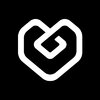
Asked in Fynd

Q. How would you use CSS to create a ring with a background color?
Create a ring with a background color using CSS by utilizing border properties and border-radius.
Use a <div> element to create the ring.
Set a fixed width and height for the <div>.
Apply a border to the <div> to create the ring effect.
Use border-radius: 50% to make the <div> circular.
Set the background color using the background property.
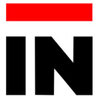
Asked in INZINT

Q. What basic JavaScript questions were you asked during the interview?
Basic JavaScript questions often cover fundamentals like variables, functions, and scope.
What is the difference between 'var', 'let', and 'const'? Example: 'var' is function-scoped, while 'let' and 'const' are block-scoped.
Explain hoisting in JavaScript. Example: Variables declared with 'var' are hoisted to the top of their scope.
What are closures? Example: A function that retains access to its lexical scope even when the function is executed outside that scope.
What is the pu...read more
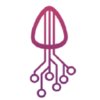
Asked in Loumus Data Systems

Q. Design a database schema for nested comments on a post.
Design a database schema for nested comments on a post
Create a table for posts with a unique ID
Create a table for comments with a unique ID, post ID, and parent comment ID
Use foreign keys to link comments to posts and parent comments
Use a recursive function to retrieve nested comments
Fullstack Javascript Developer Jobs
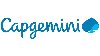
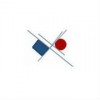
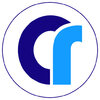
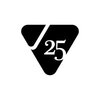
Asked in ValueLabs

Q. What is the event loop in JavaScript?
Event loop is a mechanism in JavaScript that handles asynchronous operations.
Event loop continuously checks the call stack and the task queue.
If the call stack is empty, it takes the first task from the queue and pushes it to the call stack.
Event loop is responsible for handling callbacks and promises.
Event loop prevents blocking of the main thread.
Asked in Codinova Technologies

Q. What is maps, reduce, hoisting, strings, var, let, const
Maps, reduce, hoisting, strings, var, let, const are important concepts in JavaScript development.
Maps: Used to transform elements in an array without changing the original array.
Reduce: Used to reduce an array to a single value by applying a function to each element.
Hoisting: Refers to the behavior in JavaScript where variable and function declarations are moved to the top of their containing scope.
Strings: Data type used to represent text in JavaScript.
Var: Declares a varia...read more
Share interview questions and help millions of jobseekers 🌟
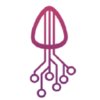
Asked in Loumus Data Systems

Q. Design a calculator in React.
A calculator app built using React
Create a component for the calculator
Use state to keep track of the input and result
Implement functions for basic arithmetic operations
Add buttons for each number and operation
Display the input and result on the screen
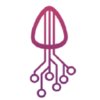
Asked in Loumus Data Systems

Q. Explain Higher Order Functions.
Higher order functions are functions that take other functions as arguments or return functions as their output.
Higher order functions can be used to create more flexible and reusable code.
They allow for functions to be composed and combined in various ways.
Examples of higher order functions include map, filter, and reduce in JavaScript.
Higher order functions can also be used for currying and partial application.
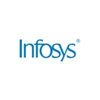
Asked in Infosys

Q. What is the event loop?
Event loops are mechanisms in programming languages that allow for asynchronous execution of code.
Event loops handle the execution of multiple tasks in a non-blocking manner.
They continuously check for tasks in the event queue and execute them one by one.
Event loops are commonly used in JavaScript to handle asynchronous operations like setTimeout, setInterval, and AJAX requests.
Asked in Codinova Technologies

Q. Implement a custom hook.
Custom hook is a reusable function in React that allows you to extract component logic into a separate function.
Create a function starting with 'use' keyword (e.g. useCustomHook)
Use useState, useEffect, useContext, or other hooks inside the custom hook
Return the necessary values or functions from the custom hook
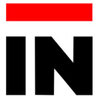
Asked in INZINT

Q. Explain the differences between bind, call, and apply in JavaScript.
Bind, call, and apply are methods in JavaScript for controlling function context and arguments.
bind(): Creates a new function with a specified 'this' context. Example: const boundFunc = func.bind(obj);
call(): Invokes a function with a specified 'this' context and arguments. Example: func.call(obj, arg1, arg2);
apply(): Similar to call, but takes an array of arguments. Example: func.apply(obj, [arg1, arg2]);
bind() returns a new function, while call() and apply() execute the fun...read more
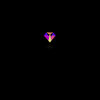
Asked in Diamondpick

Q. Given the head of a linked list, remove the nth node from the end of the list and return its head.
Remove the nth node from a linked list
Traverse the linked list to find the nth node
Update the pointers to skip over the nth node
Handle edge cases like removing the head node
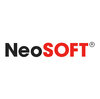
Asked in NeoSOFT

Q. What is middleware in Node.js, and what are its uses?
Middleware in Node.js is a function that has access to the request and response objects, and can modify or terminate the request-response cycle.
Middleware functions are executed sequentially in the order they are defined.
They can be used for tasks such as logging, authentication, error handling, etc.
Example: Express.js uses middleware extensively for handling requests.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
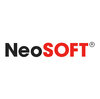
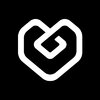
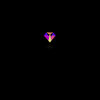
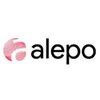
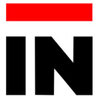
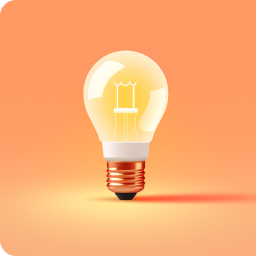
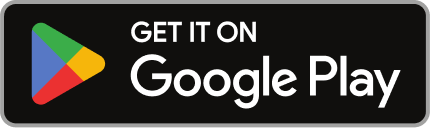
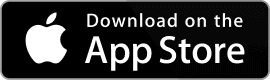
Reviews
Interviews
Salaries
Users
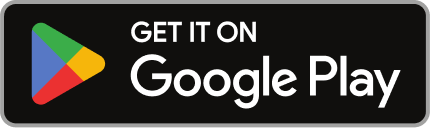
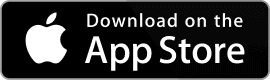