Full Stack Web Developer
50+ Full Stack Web Developer Interview Questions and Answers
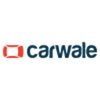
Asked in Carwale

Q. What is the time complexity of adding an element to a Singly Linked List?
Time complexity to add an element to a Singly Linked List is O(1) if added at the end using Tail pointer, and O(n) if added anywhere in the middle.
Adding an element at the end of a Singly Linked List using the Tail pointer is a constant time operation, hence O(1).
Adding an element anywhere in the middle of a Singly Linked List requires traversing the list to find the insertion point, resulting in a time complexity of O(n).
For example, if we have a Singly Linked List with 5 el...read more
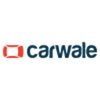
Asked in Carwale

Q. JSON How to optimize JSON? Wrote a Py code to convert optimized JSON into normal JSON. An alternative method of storing JSON data in another DS
Optimizing JSON involves minimizing redundant data, using efficient data structures, and compressing data when necessary.
Minimize redundant data by using references or IDs instead of repeating the same information multiple times.
Use efficient data structures like arrays or dictionaries to store JSON data in a more organized and accessible way.
Compress JSON data using techniques like gzip or deflate to reduce file size and improve loading times.
Example: Instead of storing the ...read more
Full Stack Web Developer Interview Questions and Answers for Freshers
Asked in QSC, LLC

Q. In what other ways can you solve the same problems?
I can solve the same problems by using different algorithms, frameworks, or libraries.
Utilizing different algorithms such as dynamic programming, greedy algorithms, or divide and conquer
Exploring alternative frameworks like React.js, Angular, or Vue.js
Leveraging different libraries such as lodash, moment.js, or axios
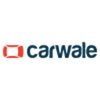
Asked in Carwale

Q. React What's the difference between using NextJs and ReactJs? Routing in NextJs. VDOM? Event Listeners. Some Hooks in NextJs.
NextJs is a framework built on top of ReactJs, providing server-side rendering and other features for easier development.
NextJs is a framework built on top of ReactJs, providing server-side rendering, routing, and other features for easier development.
ReactJs is a JavaScript library for building user interfaces, while NextJs is a framework that adds functionality like server-side rendering and routing to React applications.
NextJs simplifies the process of building React appli...read more
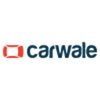
Asked in Carwale

Q. What is Time Complexity? Give examples.
Time complexity measures the amount of time an algorithm takes to complete as a function of the input size.
Time complexity is expressed using Big O notation (e.g., O(n), O(log n)).
O(1) - Constant time: Example - Accessing an element in an array by index.
O(n) - Linear time: Example - Finding an element in an unsorted array.
O(n^2) - Quadratic time: Example - Bubble sort algorithm.
O(log n) - Logarithmic time: Example - Binary search in a sorted array.
Asked in Data Dynamics Software Solutions

Q. You are building a dashboard that fetches live data every 10 seconds. How would you implement this and ensure memory is managed correctly?
Implement a live data fetching dashboard with efficient memory management using techniques like polling and cleanup.
Use setInterval to fetch data every 10 seconds: setInterval(fetchData, 10000);
Implement cleanup logic to clear previous data before fetching new data to avoid memory leaks.
Utilize WebSockets for real-time data updates if the server supports it, reducing the need for frequent polling.
Consider using a state management library (like Redux) to manage data efficientl...read more
Full Stack Web Developer Jobs
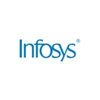
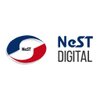
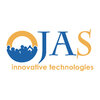
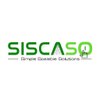
Asked in SISCASO

Q. How do you send data from the frontend to the backend?
Data can be sent from frontend to backend using HTTP requests such as POST or GET.
Use AJAX or fetch API to send data asynchronously
Include data in the request body or URL parameters
Backend should have an API endpoint to receive the data
Use appropriate data format such as JSON or form data
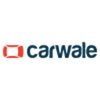
Asked in Carwale

Q. Given an array of numbers, find the smallest Prime Number.
Find the smallest prime number in a given array of numbers.
A prime number is greater than 1 and has no divisors other than 1 and itself.
Example: In the array [4, 6, 8, 3, 5], the smallest prime is 3.
To find the smallest prime, iterate through the array and check each number for primality.
Use a helper function to determine if a number is prime.
Share interview questions and help millions of jobseekers 🌟
Asked in Data Dynamics Software Solutions

Q. Design a schema for storing user roles and permissions across multiple modules of an enterprise app. How do you ensure flexibility?
Design a flexible schema for user roles and permissions in an enterprise app to manage access across multiple modules.
Use a many-to-many relationship between Users, Roles, and Permissions to allow flexibility.
Create a 'Users' table with user details and a 'Roles' table for different roles like Admin, Editor, Viewer.
Implement a 'Permissions' table that defines specific actions like 'create', 'read', 'update', 'delete'.
Link Roles to Permissions through a 'RolePermissions' table...read more
Asked in Data Dynamics Software Solutions

Q. You're building a user profile form in React with multiple fields (name, email, address). How would you manage the form state efficiently and handle validation?
Efficiently manage form state and validation in React using hooks and libraries like Formik or React Hook Form.
Use React's useState or useReducer for managing form state.
Example: const [formData, setFormData] = useState({ name: '', email: '', address: '' });
Implement controlled components to sync input values with state.
Example: <input value={formData.name} onChange={e => setFormData({...formData, name: e.target.value})} />
Use validation libraries like Yup for schema-based va...read more
Asked in Data Dynamics Inc

Q. How do you deploy a full stack app to Heroku or Vercel?
Deploying a full stack app involves setting up the environment, configuring settings, and pushing code to Heroku or Vercel.
1. Prepare your app: Ensure your app is production-ready, with necessary configurations for both frontend and backend.
2. Create a Heroku/Vercel account: Sign up and log in to your account on the respective platform.
3. Install CLI tools: For Heroku, install the Heroku CLI; for Vercel, install the Vercel CLI.
4. Initialize your project: For Heroku, run 'hero...read more
Asked in Data Dynamics Software Solutions

Q. What are HTTP status codes 301, 401, 403, and 500?
HTTP status codes indicate the result of a server's response to a client's request, each serving a specific purpose.
301: Moved Permanently - Indicates that a resource has been permanently moved to a new URL. Example: Redirecting from oldsite.com to newsite.com.
401: Unauthorized - Indicates that authentication is required and has failed or has not yet been provided. Example: Accessing a protected resource without login.
403: Forbidden - Indicates that the server understands the...read more
Asked in Data Dynamics Software Solutions

Q. HOW DOSE REACT'S VIRTUL DOM WOEK What happens during a re render and how can you optimize it.
React's Virtual DOM optimizes rendering by minimizing direct DOM manipulations, enhancing performance during updates.
The Virtual DOM is a lightweight copy of the actual DOM, allowing React to efficiently determine changes.
During a re-render, React compares the new Virtual DOM with the previous one using a diffing algorithm.
Only the components that have changed are updated in the actual DOM, reducing performance costs.
React uses reconciliation to identify which parts of the UI...read more
Asked in Data Dynamics Software Solutions

Q. How would you design a REST API to manage user roles and permissions, and how would you secure its endpoints?
Design a secure REST API for managing user roles and permissions with proper authentication and authorization.
Use OAuth 2.0 for secure token-based authentication.
Implement role-based access control (RBAC) to restrict access to endpoints.
Validate user permissions before processing requests, e.g., check if a user has 'admin' role for certain actions.
Use HTTPS to encrypt data in transit, preventing eavesdropping.
Rate limit API requests to mitigate brute force attacks.
Asked in Data Dynamics Software Solutions

Q. What are CORS issues and how do you resolve them in an Express + React setup?
CORS issues arise when a web application requests resources from a different origin, leading to security restrictions.
CORS stands for Cross-Origin Resource Sharing, a security feature implemented by browsers.
When a React app (frontend) tries to access an API (backend) on a different domain, CORS policies may block the request.
To resolve CORS issues in an Express app, use the 'cors' middleware: `const cors = require('cors'); app.use(cors());`
You can configure CORS to allow spe...read more
Asked in Data Dynamics Software Solutions

Q. How do you ensure security in web applications (e.g., XSS, CSRF) protection?
Implementing security measures like input validation and tokenization to protect against XSS and CSRF attacks.
Use input validation and sanitization to prevent XSS. For example, use libraries like DOMPurify to clean user input.
Implement Content Security Policy (CSP) to restrict sources of content and mitigate XSS risks.
Use anti-CSRF tokens in forms and AJAX requests to ensure that requests are legitimate. For example, include a token in the form that is validated on the server...read more
Asked in Data Dynamics Software Solutions

Q. How do you manage state in a large-scale application using Redux, Context API, or another method, and why?
Managing state in large-scale applications can be achieved using Redux, Context API, or other solutions based on specific needs.
Redux is ideal for complex state management with a predictable state container, making it easier to debug and test.
Context API is suitable for simpler state management needs, allowing for easier integration without additional libraries.
For example, Redux can manage global state like user authentication, while Context API can handle theme settings.
Con...read more
Asked in Data Dynamics Software Solutions

Q. How does event delegation work in JavaScript and why is it useful?
Event delegation is a technique in JavaScript that allows handling events at a higher level in the DOM hierarchy.
Event delegation works by attaching a single event listener to a parent element instead of multiple listeners to child elements.
When an event occurs on a child element, it bubbles up to the parent, allowing the parent listener to handle it.
This reduces memory usage and improves performance, especially with many child elements.
Example: Instead of adding click listen...read more
Asked in Data Dynamics Software Solutions

Q. What is the difference between SQL joins: INNER, LEFT, RIGHT, and FULL?
SQL joins combine rows from two or more tables based on related columns, with different types defining how unmatched rows are handled.
INNER JOIN: Returns only matching rows from both tables. Example: SELECT * FROM A INNER JOIN B ON A.id = B.id.
LEFT JOIN: Returns all rows from the left table and matched rows from the right table. Unmatched rows in the right table will be NULL. Example: SELECT * FROM A LEFT JOIN B ON A.id = B.id.
RIGHT JOIN: Returns all rows from the right table...read more
Asked in Data Dynamics Inc

Q. How do you handle API versioning in a RESTful backend?
API versioning in RESTful backends ensures compatibility and smooth transitions between different API versions.
Use URI versioning: e.g., /api/v1/resource and /api/v2/resource.
Implement query parameter versioning: e.g., /api/resource?version=1.
Utilize header versioning: clients specify the version in request headers.
Maintain backward compatibility to avoid breaking changes for existing clients.
Document each version clearly to guide developers on usage and changes.
Asked in Data Dynamics Software Solutions

Q. How would you handle concurrency issues in a Node.js backend?
Concurrency in Node.js can be managed using techniques like callbacks, promises, and async/await to ensure data integrity.
Use asynchronous programming with callbacks to handle multiple requests without blocking the event loop.
Implement Promises to manage asynchronous operations more effectively, allowing for cleaner code and better error handling.
Utilize async/await syntax for a more synchronous-like flow, making it easier to read and maintain code.
Consider using a message qu...read more
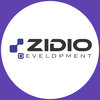
Asked in Zidio Development

Q. What additional and distinctive features did you implement?
Implemented features like real-time notifications, user roles, and advanced search for enhanced user experience.
Real-time notifications using WebSockets for instant updates on user activities.
User roles and permissions to manage access levels, ensuring data security.
Advanced search functionality with filters for improved content discovery.
Responsive design for seamless experience across devices.
Integration of third-party APIs for enhanced functionality, like payment gateways.
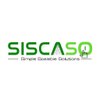
Asked in SISCASO

Q. How do you link the frontend with the backend?
The frontend and backend can be linked through APIs, where the frontend sends requests to the backend and receives responses.
Use RESTful APIs to define endpoints and methods for communication between frontend and backend
Frontend makes HTTP requests to backend APIs using libraries like Axios or Fetch
Backend processes the requests, performs necessary operations, and sends back responses
Responses can be in various formats like JSON or XML
Examples: a frontend form submitting data...read more
Asked in Data Dynamics Software Solutions

Q. You are building an Express.js middleware to authenticate API requests using a JWT token. The token is passed in the Authorization header.
Create Express.js middleware to authenticate API requests using JWT from the Authorization header.
Use the 'jsonwebtoken' library to verify the JWT token.
Extract the token from the 'Authorization' header: 'Bearer <token>'.
Check if the token is valid and not expired.
If valid, attach the user information to the request object for further use.
Send a 401 response if the token is missing or invalid.
Asked in Data Dynamics Software Solutions

Q. You need to display a list of products fetched from an API in a React component with the following requirements.
Display a list of products from an API in a React component using state and effects.
Use useState to manage the product list state. Example: const [products, setProducts] = useState([]);
Use useEffect to fetch data from the API when the component mounts. Example: useEffect(() => { fetchProducts(); }, []);
Implement a function to fetch products from the API. Example: const fetchProducts = async () => { const response = await fetch(apiUrl); const data = await response.json(); setP...read more
Asked in Essential Services Outsource

Q. How do you upload multiple files using AJAX?
Multiple files can be uploaded using AJAX by sending a FormData object containing the files.
Create a FormData object
Append each file to the FormData object
Send the FormData object using AJAX
Asked in DifferenTech Solutions

Q. How does OTP generation work in a signup system?
OTP generation in SignUp system involves generating a unique code and sending it to the user for verification.
Generate a random code using a combination of numbers and letters
Store the code in a database or cache for verification
Send the code to the user via email, SMS, or other communication channels
Validate the code entered by the user during the SignUp process
Expire the code after a certain period of time to ensure security
Asked in Data Dynamics Inc

Q. How does the React Reconciliation process work?
React Reconciliation is the process of updating the UI efficiently by comparing virtual DOM trees.
React uses a virtual DOM to minimize direct manipulation of the real DOM, which is slow.
When a component's state or props change, React creates a new virtual DOM tree.
React compares the new virtual DOM with the previous one using a diffing algorithm.
If differences are found, React updates only the changed parts of the real DOM.
This process is optimized by using keys in lists to i...read more
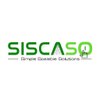
Asked in SISCASO

Q. What do you know about the POST method?
Yes, the POST method is used to send data to a server to create or update a resource.
POST is one of the HTTP methods used to send data to a server
It is commonly used to create or update a resource on the server
Data is sent in the request body
Examples include submitting a form, creating a new user account, or adding a new item to a shopping cart
Asked in Data Dynamics Software Solutions

Q. Describe how REST APIs differ from GraphQL.
REST APIs use fixed endpoints for data, while GraphQL allows flexible queries for specific data needs.
REST APIs have multiple endpoints (e.g., /users, /posts) for different resources.
GraphQL has a single endpoint (e.g., /graphql) that handles all queries.
In REST, the server defines the structure of responses; in GraphQL, clients specify the structure.
REST can lead to over-fetching or under-fetching data; GraphQL allows clients to request exactly what they need.
Example: In RES...read more
Interview Experiences of Popular Companies
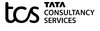
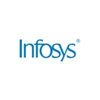
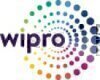
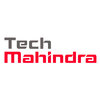
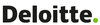
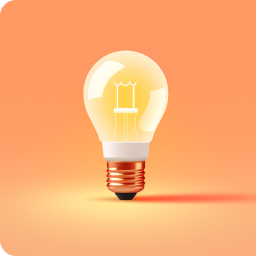
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
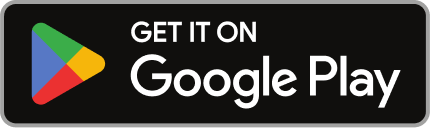
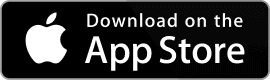
Reviews
Interviews
Salaries
Users
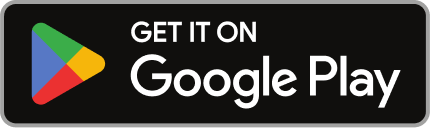
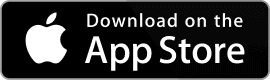