Full Stack Software Developer
300+ Full Stack Software Developer Interview Questions and Answers
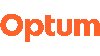
Asked in Optum Global Solutions

Q. What are the types of relationships in Database Management Systems (DBMS)?
Types of relationships in DBMS include one-to-one, one-to-many, and many-to-many relationships.
One-to-one relationship: Each record in one table is related to only one record in another table.
One-to-many relationship: Each record in one table can be related to multiple records in another table.
Many-to-many relationship: Multiple records in one table can be related to multiple records in another table.
Examples: One-to-one - Employee and EmployeeDetails tables, One-to-many - De...read more
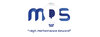
Asked in MDS Digital Media Private Limited

Q. Java vs JavaScript Linux commands Jquery How JS communicates with serverside
Questions on Java vs JavaScript, Linux commands, Jquery, and JS communication with serverside.
Java is a statically typed language, while JavaScript is dynamically typed.
Linux commands are used to interact with the operating system and perform tasks like file management and networking.
Jquery is a JavaScript library used for DOM manipulation and event handling.
JS communicates with serverside using AJAX, which stands for Asynchronous JavaScript and XML.
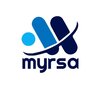
Asked in Myrsa Technology Solutions

Q. What are the differences between JavaScript and NodeJS?
JavaScript is a programming language used for web development, while Node.js is a runtime environment for executing JavaScript code outside of a web browser.
JavaScript is primarily used for client-side scripting, while Node.js is used for server-side scripting.
JavaScript is executed in a web browser, while Node.js is executed on a server.
Node.js provides additional modules and libraries for server-side development, such as file system access and networking capabilities.
JavaSc...read more
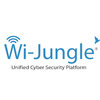
Asked in WiJungle

Q. Given two line segments with coordinates a1, b1 and a2, b2, determine if they intersect.
To determine if two line segments with coordinates a1,b1 & a2,b2 intersect, we need to check if the line segments intersect.
Calculate the slopes of both line segments
Check if the slopes are equal, if yes, check if the y-intercepts are equal
If slopes are not equal, calculate the intersection point of the two lines
Check if the intersection point lies on both line segments
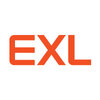
Asked in EXL Service

Q. AWS architecture used in current project and why used these services only. What could have been better.
AWS architecture in projects optimizes scalability, reliability, and cost-effectiveness using various cloud services.
EC2 Instances: Used for scalable computing capacity; for example, we can spin up instances based on traffic demands.
S3 Storage: Chosen for its durability and scalability to store static assets like images and backups efficiently.
RDS: Utilized for managed relational databases, providing automated backups and scaling options, which simplifies database management....read more
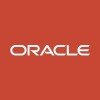
Asked in Oracle

Q. Explain the Singleton design pattern and implement a thread-safe version.
Singleton design pattern ensures a class has only one instance and provides a global point of access to it.
Ensure a private static instance variable in the class.
Provide a public static method to access the instance, creating it if necessary.
Use synchronized keyword or double-checked locking to make it thread-safe.
Full Stack Software Developer Jobs
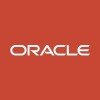
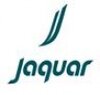
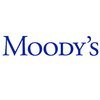
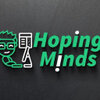
Asked in Hoping Minds

Q. Which technologies excite you?
I am excited about technologies that enable seamless integration and automation.
APIs and microservices
DevOps tools like Docker and Kubernetes
Automation tools like Selenium and Jenkins
Cloud computing platforms like AWS and Azure
Machine learning and AI technologies
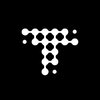
Asked in Turing

Q. How do you optimize the performance of a Node.js app?
Optimizing performance of a Nodejs app involves various techniques to improve speed and efficiency.
Use asynchronous programming to handle multiple requests efficiently
Implement caching to reduce redundant database queries
Optimize code by removing unnecessary loops and function calls
Use a load balancer to distribute traffic evenly across multiple servers
Monitor and analyze performance using tools like New Relic or Datadog
Share interview questions and help millions of jobseekers 🌟
Asked in Cognifyz Technologies

Q. How does the browser render a webpage from the moment a user enters a URL until the page is displayed?
The browser processes a URL to display a webpage through several steps, including DNS resolution, HTTP requests, and rendering.
1. URL Input: User enters a URL in the browser's address bar.
2. DNS Resolution: The browser queries a DNS server to convert the URL into an IP address.
3. HTTP Request: The browser sends an HTTP request to the server at the resolved IP address.
4. Server Response: The server processes the request and sends back the HTML, CSS, and JavaScript files.
5. Ren...read more
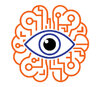
Asked in VisAI Labs

Q. What are your pros and cons?
I am a highly motivated and detail-oriented full stack developer with strong problem-solving skills.
Pros: Strong problem-solving skills
Detail-oriented
Highly motivated
Experience in full stack development
Cons: Can be overly meticulous at times
May need to work on delegating tasks more effectively
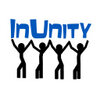
Asked in Inunity Mangaluru

Q. Name various hooks and explain their purpose.
Hooks in React are functions that allow you to use state and other React features in functional components.
useState - allows functional components to have state
useEffect - allows side effects in functional components
useContext - allows functional components to access context
useReducer - alternative to useState for more complex state logic
useMemo - memoizes a value to prevent unnecessary re-renders
useRef - allows functional components to access DOM elements or store mutable va...read more
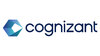
Asked in Cognizant

Q. Differentiate between an exclusive lock and a shared lock.
Exclusive lock is used when a resource is being modified and prevents other processes from accessing it. Shared lock allows multiple processes to read a resource simultaneously.
Exclusive lock is used for write operations, while shared lock is used for read operations.
Exclusive lock blocks other processes from acquiring both exclusive and shared locks on the same resource.
Shared lock allows multiple processes to acquire shared locks on the same resource simultaneously.
Exclusiv...read more
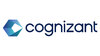
Asked in Cognizant

Q. Explain these terms - Linked List, Stack, Queue.
Linked List is a linear data structure. Stack and Queue are abstract data types.
Linked List: A collection of nodes where each node points to the next node.
Stack: A data structure where elements are added and removed from the top only.
Queue: A data structure where elements are added at the rear and removed from the front only.
Example: Browser history can be implemented using a Linked List.
Example: Undo/Redo functionality can be implemented using a Stack.
Example: Print queue ca...read more
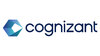
Asked in Cognizant

Q. What is the object-oriented model?
Object oriented model is a programming paradigm that uses objects to represent real-world entities.
Objects have properties and methods that define their behavior
Encapsulation, inheritance, and polymorphism are key concepts in OOP
Examples of OOP languages include Java, C++, and Python
Asked in Edovu Ventures

Q. Can you show me the codebase of a project you have worked on?
A web application for managing tasks with user authentication and real-time updates using React and Node.js.
Utilized React for the front-end, enabling a dynamic user interface.
Implemented Node.js and Express for the back-end, handling API requests.
Used MongoDB for data storage, allowing for flexible data management.
Integrated JWT for secure user authentication and session management.
Employed Socket.io for real-time updates on task status.
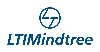
Asked in LTIMindtree

Q. Given a sorted array of integers, write a function to perform a binary search to find the index of a target value. If the target value is not found, return -1.
Binary search function to find target value in sorted array
Define function that takes sorted array and target value as input
Initialize variables for start, end, and middle indices
Use while loop to iterate until start is less than or equal to end
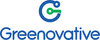
Asked in Greenovative Energy

Q. What are the types of directives in Angular?
Types of directives in Angular include structural directives, attribute directives, and component directives.
Structural directives are used to add or remove elements from the DOM based on conditions (e.g. *ngIf, *ngFor)
Attribute directives are used to change the appearance or behavior of an element (e.g. ngStyle, ngClass)
Component directives are custom directives that are components themselves (e.g. @Component)
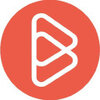
Asked in BigTime Software

Q. What are the different data types present in JavaScript?
JavaScript has several data types including string, number, boolean, object, function, undefined, and null.
String: 'hello', '123'
Number: 123, 3.14
Boolean: true, false
Object: { key: 'value' }
Function: function() { }
Undefined: undefined
Null: null
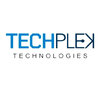
Asked in TechPlek Technologies

Q. What has been your experience focusing primarily on frontend development rather than backend?
I have focused on creating user-friendly interfaces, optimizing performance, and ensuring responsive designs in frontend development.
Developed interactive web applications using React, enhancing user engagement through dynamic content updates.
Implemented responsive design principles with CSS frameworks like Bootstrap, ensuring accessibility across devices.
Utilized tools like Figma for UI/UX design, translating wireframes into functional components.
Optimized frontend performan...read more
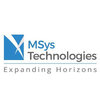
Asked in MSys Tech India

Q. What is the method for implementing inheritance in Golang?
In Golang, inheritance is implemented using composition instead of traditional inheritance.
Golang does not support traditional inheritance like other object-oriented languages.
Instead of inheritance, Golang promotes code reuse through composition.
Embedding structs in Golang allows for achieving similar behavior to inheritance.
Asked in Cognifyz Technologies

Q. Explain the difference between SQL and NoSQL databases. When would you use one over the other?
SQL databases are structured and relational, while NoSQL databases are flexible and non-relational.
SQL databases use structured query language (e.g., MySQL, PostgreSQL).
NoSQL databases are schema-less and can store unstructured data (e.g., MongoDB, Cassandra).
SQL is ideal for complex queries and transactions, while NoSQL is better for large-scale data and real-time applications.
Use SQL for applications requiring ACID compliance, like banking systems.
Use NoSQL for applications...read more
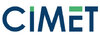
Asked in CIMET

Q. What is the importance of the key prop in React? Explain in detail.
Keys in React are important for efficient rendering and maintaining component state.
Keys help React identify which items have changed, are added, or are removed in a list of components.
Using keys correctly can improve performance by reducing unnecessary re-renders.
Keys should be unique among siblings but can be reused across different lists.
Example:
- {items.map(item =>
- {item.name} )}
Asked in Hilavsen

Q. How node.js works? Reason why node.js was familiar to work with?
Node.js is a runtime environment that allows JavaScript to be run on the server side.
Node.js uses an event-driven, non-blocking I/O model which makes it lightweight and efficient.
It is built on the V8 JavaScript engine from Google Chrome.
Node.js is commonly used for building scalable network applications.
It allows developers to use JavaScript for both client-side and server-side development.
Node.js has a large ecosystem of open source libraries and frameworks available.
Asked in Hilavsen

Q. How would you restrict unauthorised access to certain end-points? Have you worked on with MFA's?
Restricting unauthorized access to endpoints can be achieved through authentication mechanisms like JWT, OAuth, API keys, and implementing Multi-Factor Authentication (MFA).
Implementing JWT (JSON Web Tokens) for authentication and authorization
Using OAuth for secure authorization between applications
Utilizing API keys to control access to specific endpoints
Implementing Multi-Factor Authentication (MFA) for an added layer of security
Setting up role-based access control (RBAC) ...read more
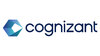
Asked in Cognizant

Q. Define the terms OSI, TCP, and IP.
OSI, TCP & IP are networking protocols used for communication between devices on a network.
OSI (Open Systems Interconnection) is a conceptual model that defines how data is transmitted over a network.
TCP (Transmission Control Protocol) is a protocol that ensures reliable transmission of data between devices.
IP (Internet Protocol) is a protocol that handles the addressing and routing of data packets between devices on a network.
TCP/IP is a suite of protocols that are commonly ...read more
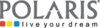
Asked in Polaris Consulting

Q. What is a String?? Difference between String Buffer and String Builder?
A String is a sequence of characters. String Buffer and String Builder are mutable and faster alternatives to String.
String is an immutable class in Java.
String Buffer and String Builder are mutable classes.
String Buffer is synchronized and thread-safe.
String Builder is not synchronized and faster than String Buffer.
Both String Buffer and String Builder have methods to append, insert, delete, and reverse strings.
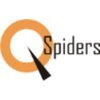
Asked in QSpiders

Q. What is a Join? List its different types.?
A Join is used to combine rows from two or more tables based on a related column between them.
Types of Joins: Inner Join, Left Join, Right Join, Full Outer Join, Cross Join
Inner Join: Returns rows when there is at least one match in both tables
Left Join: Returns all rows from the left table and the matched rows from the right table
Right Join: Returns all rows from the right table and the matched rows from the left table
Full Outer Join: Returns rows when there is a match in on...read more
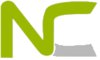
Asked in Northcorp Software

Q. Can you explain the differences between REST and GraphQL APIs?
REST and GraphQL are two different approaches for building APIs, each with unique characteristics and use cases.
REST is resource-based, while GraphQL is query-based.
In REST, multiple endpoints are used for different resources; in GraphQL, a single endpoint handles all queries.
REST returns fixed data structures; GraphQL allows clients to specify exactly what data they need.
Example: In REST, to get user data, you might call /users/1; in GraphQL, you can request specific fields ...read more
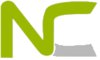
Asked in Northcorp Software

Q. How do you ensure the performance and scalability of a full stack application?
To ensure performance and scalability, I focus on efficient architecture, optimization techniques, and monitoring tools.
Use microservices architecture to isolate services and scale independently.
Implement caching strategies (e.g., Redis, Memcached) to reduce database load.
Optimize database queries and use indexing to speed up data retrieval.
Utilize load balancers to distribute traffic evenly across servers.
Employ asynchronous processing for long-running tasks (e.g., using mes...read more
Asked in Driksha Infotech

Q. What is the difference between GET and POST methods in HTTP?
GET retrieves data from a server, while POST sends data to be processed.
GET requests data from a specified resource, e.g., fetching user data from /users.
POST submits data to be processed, e.g., submitting a form at /submit.
GET requests can be cached, while POST requests are not cached.
GET requests have length limitations (URL length), while POST can handle larger amounts of data.
GET parameters are visible in the URL, while POST parameters are included in the request body.
Interview Experiences of Popular Companies
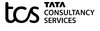
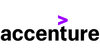
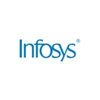
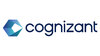
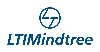
Top Interview Questions for Full Stack Software Developer Related Skills
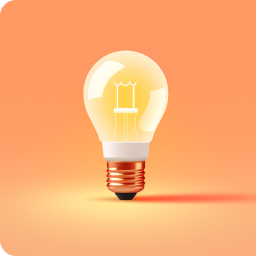
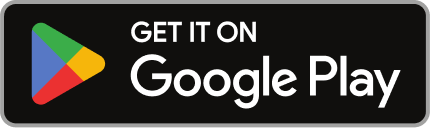
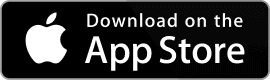
Reviews
Interviews
Salaries
Users
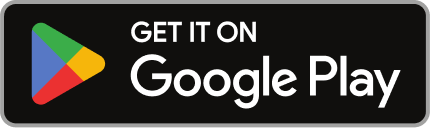
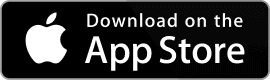