Angular Frontend Developer
200+ Angular Frontend Developer Interview Questions and Answers
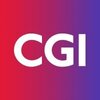
Asked in CGI Group

Q. parameter and argument difference in JavaScript ? 2. Closures and hoisting in Javascript ? 3. Var, let and Const difference ?
Understanding JavaScript concepts like parameters, closures, and variable declarations is crucial for effective coding.
Parameters are variables listed as part of a function's definition, while arguments are the actual values passed to the function.
Example: function add(a, b) { return a + b; } - 'a' and 'b' are parameters; add(2, 3) - '2' and '3' are arguments.
Closures are functions that remember their lexical scope even when the function is executed outside that scope.
Example...read more
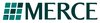
Asked in Merce Technologies

Q. How do you call an API in Angular? Write the code for this.
To call an API in Angular, use HttpClient module to make HTTP requests.
Import HttpClientModule in app.module.ts
Inject HttpClient in the component/service where API call needs to be made
Use HttpClient methods like get(), post(), put(), delete() to make API calls
Subscribe to the Observable returned by HttpClient methods to handle the response
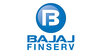
Asked in Bajaj Finserv

Q. What is the JavaScript code for finding repeating alphabets?
Use JavaScript to find repeating alphabets in an array of strings.
Loop through each string in the array
For each string, loop through each character and check if it is repeated
Store the repeating alphabets in a separate array
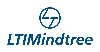
Asked in LTIMindtree

Q. How many ways can we communicate between Angular components?
There are multiple ways to communicate in Angular components, including Input, Output, ViewChild, and Services.
Input - parent component can pass data to child component using @Input decorator
Output - child component can emit events to parent component using @Output decorator
ViewChild - parent component can access child component using @ViewChild decorator
Services - components can communicate through shared services
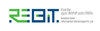
Asked in Reserve Bank Information Technology

Q. what promiss and obs and angular qustion and javascript program
Promises and Observables in Angular and JavaScript programs
Promises are used for asynchronous operations in JavaScript, representing a value that may be available now, in the future, or never
Observables are used in Angular for handling asynchronous data streams, allowing for easier management of multiple values over time
Promises have a single value, while Observables can emit multiple values over time
Promises use .then() for handling success and error callbacks, while Observa...read more
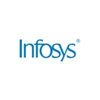
Asked in Infosys

Q. What is the best way to optimize Angular application performance?
Optimize Angular app performance by using lazy loading, change detection strategies, and efficient data handling.
Use Lazy Loading: Load feature modules only when needed to reduce initial load time. Example: Use 'loadChildren' in routing.
Implement Change Detection Strategies: Use OnPush strategy to minimize checks. This can improve performance by reducing unnecessary checks.
Optimize Template Expressions: Avoid complex expressions in templates. Pre-compute values in the compone...read more
Angular Frontend Developer Jobs
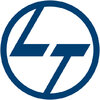
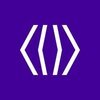
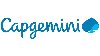
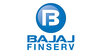
Asked in Bajaj Finserv

Q. What are the best practices for making a webpage responsive?
Responsive web design ensures optimal viewing across devices by adapting layout and content to different screen sizes.
Use flexible grid layouts that adjust based on screen size, e.g., CSS Grid or Flexbox.
Implement media queries to apply different styles for various screen widths, e.g., @media (max-width: 600px) { ... }.
Utilize responsive images with the 'srcset' attribute to serve different image sizes based on device resolution.
Adopt a mobile-first approach by designing for ...read more
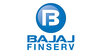
Asked in Bajaj Finserv

Q. What are the differences between JavaScript (JS) and TypeScript (TS)?
TypeScript is a superset of JavaScript that adds static typing and other features for better development experience.
TypeScript is statically typed, while JavaScript is dynamically typed. Example: In TS, you can define a variable as 'let num: number = 5;'.
TypeScript supports interfaces and enums, which help in defining contracts and enumerations. Example: 'interface User { name: string; age: number; }'.
TypeScript provides better tooling and IDE support, including autocompletio...read more
Share interview questions and help millions of jobseekers 🌟
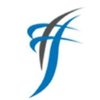
Asked in Samcom Technobrains

Q. What are the new features in the latest Angular version?
Some new features in the latest Angular version include Ivy rendering engine, Bazel support, and differential loading.
Ivy rendering engine for improved performance and smaller bundle sizes
Bazel support for faster builds and better dependency management
Differential loading for serving different bundles to modern and legacy browsers
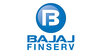
Asked in Bajaj Finserv

Q. What is data binding in the context of software development?
Data binding is a technique that connects the UI to the data model, enabling automatic synchronization between them.
Types of data binding: One-way binding (e.g., displaying data) and two-way binding (e.g., form inputs).
Example of one-way binding: Using interpolation to display a variable in the template: {{ variableName }}.
Example of two-way binding: Using ngModel in forms to bind input values to a component property.
Data binding enhances user experience by ensuring that the ...read more
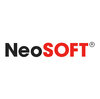
Asked in NeoSOFT

Q. 4.How to create custom Pipe? 5.Directives in angular 6.Binding in angular
Answers to questions related to Angular frontend development
To create a custom pipe, use the @Pipe decorator and implement the PipeTransform interface
Directives in Angular are used to manipulate the DOM and add behavior to elements
Binding in Angular is used to connect the component class with the template
Examples of binding include property binding, event binding, and two-way binding
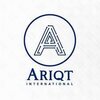
Asked in Ariqt International

Q. How is JavaScript considered both single-threaded and asynchronous?
JavaScript is single-threaded due to its event loop, yet it handles asynchronous operations using callbacks, promises, and async/await.
JavaScript runs on a single thread, meaning it can execute one command at a time.
Asynchronous operations allow JavaScript to perform tasks like fetching data without blocking the main thread.
The event loop manages asynchronous callbacks, enabling JavaScript to handle events and I/O operations efficiently.
Example: Using 'setTimeout' allows a fu...read more
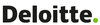
Asked in Deloitte

Q. What are the different types of loading in Angular?
Angular supports various loading strategies to optimize application performance and user experience.
Eager Loading: All modules are loaded at the start. Example: Importing all feature modules in the root module.
Lazy Loading: Modules are loaded on demand. Example: Using the 'loadChildren' property in the route configuration.
Preloading: Loads modules in the background after the application has been loaded. Example: Using PreloadAllModules strategy in routing.
OnPush Change Detect...read more
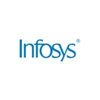
Asked in Infosys

Q. What is a pipe in Angular, and what are its types?
A pipe in Angular is used to transform data before displaying it in the template.
Pipes are used in templates to format data before displaying it to the user
There are built-in pipes like DatePipe, UpperCasePipe, LowerCasePipe, etc.
Custom pipes can also be created by implementing the PipeTransform interface
Pipes can be chained together to perform multiple transformations on the data
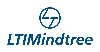
Asked in LTIMindtree

Q. Create a reactive form and save data in API with proper validation.
Create a reactive form in Angular to save data in API with validation
Create a reactive form using FormBuilder in Angular
Add form controls with proper validation rules using Validators
Handle form submission and send data to API using HttpClient
Implement error handling for API requests
Display success or error messages to the user
Asked in Synergy Softwares

Q. How do you handle large-scale responses through APIs in Angular?
Handling large-scale API responses in Angular involves efficient data management, pagination, and lazy loading techniques.
Pagination: Implement pagination to load data in chunks, reducing the initial load time. For example, use Angular Material's paginator component.
Lazy Loading: Use lazy loading to load modules only when needed, which can help in managing large datasets efficiently.
Data Caching: Implement caching strategies to store previously fetched data, minimizing API ca...read more

Asked in SplendorNet Technologies

Q. What is Angular? Explain the folder structure.
Angular is a JavaScript framework for building web applications. It uses TypeScript and follows the MVC architecture.
Angular has a modular architecture with components, services, and modules.
The folder structure includes app, assets, environments, and node_modules folders.
The app folder contains the main application code, including components, services, and modules.
The assets folder contains static files like images and fonts.
The environments folder contains environment-speci...read more
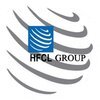
Asked in HFCL Limited

Q. What is the difference between arrow functions and normal functions?
Arrow functions provide a concise syntax and lexical scoping of 'this', differing from traditional function expressions.
Syntax: Arrow functions use a shorter syntax, e.g., const add = (a, b) => a + b; vs function add(a, b) { return a + b; }.
Lexical 'this': Arrow functions do not have their own 'this' context; they inherit 'this' from the parent scope, unlike normal functions.
Implicit return: If the function body consists of a single expression, arrow functions can return it i...read more
Asked in AdBorn Solutions

Q. Explain how standalone components work in Angular 14.
Standalone components in Angular 14 allow for independent component usage without needing a module.
Standalone components can be declared using the `@Component` decorator with the `standalone: true` property.
They can be used directly in templates without being part of an NgModule.
Example: `@Component({ selector: 'app-standalone', standalone: true, template: '<h1>Hello</h1>' })`.
Standalone components can import other standalone components or modules directly.
They simplify the c...read more
Asked in AdBorn Solutions

Q. What happens when you trigger changeDetectorRef.detectChange()?
Calling detectChanges() triggers Angular's change detection for the component, updating the view with any changes in data.
detectChanges() checks the component and its children for changes in data bindings.
It updates the view immediately, reflecting any changes in the component's state.
Example: If a property in the component changes, calling detectChanges() will update the UI without waiting for the next change detection cycle.
Useful in scenarios where Angular's automatic chan...read more
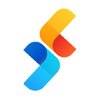
Asked in Successive Technologies

Q. Which map should be used for autocomplete?
The map to use for auto complete is the Trie data structure.
Trie is a tree-like data structure that efficiently stores and retrieves strings.
It is commonly used for auto complete functionality as it allows for fast prefix matching.
Each node in the Trie represents a character, and the edges represent the next possible characters.
By traversing the Trie, all possible completions for a given prefix can be found.
Example: Searching for 'app' in a Trie containing 'apple', 'applicati...read more
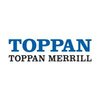

Q. How do you implement change detection for heavy components in Angular?
Optimize change detection in Angular for heavy components using strategies like OnPush, manual detection, and trackBy.
Use ChangeDetectionStrategy.OnPush to limit checks to input-bound properties.
Implement manual change detection using ChangeDetectorRef.detectChanges() when necessary.
Utilize trackBy in ngFor to optimize rendering of lists, reducing unnecessary checks.
Leverage Observables and async pipe to automatically manage subscriptions and change detection.
Consider using t...read more
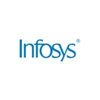
Asked in Infosys

Q. Write a JavaScript function to remove duplicate elements from an array.
Use JavaScript to remove duplicate elements from an array of strings.
Create a new Set from the array to automatically remove duplicates
Convert the Set back to an array to get the final result
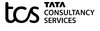
Asked in TCS

Q. How do you communicate between two non-related components?
Using a shared service or event emitter to facilitate communication between non-relatable components.
Create a shared service with methods to send and receive data between components
Use event emitters to emit events and pass data between components
Utilize RxJS subjects to create an observable data stream for communication
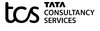
Asked in TCS

Q. What is angular , why SPA came in picture
Angular is a JavaScript framework for building web applications. SPA came in picture for better user experience.
Angular is a client-side framework developed by Google.
It allows developers to build dynamic, single-page web applications.
SPA (Single Page Application) came in picture to provide a better user experience by reducing page reloads and improving performance.
SPA loads all the necessary resources (HTML, CSS, JS) at once and dynamically updates the content as the user in...read more
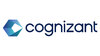
Asked in Cognizant

Q. Given an API URL, fetch data from the API, render it in the UI, and create a custom pipe to transform the ID into the format 1**8.
Fetch data from an API and display it in Angular, using a custom pipe to format IDs.
Use HttpClient to make API calls: `this.http.get(apiUrl).subscribe(data => this.data = data);`
Create a custom pipe using Angular CLI: `ng generate pipe idFormatter`.
In the pipe, implement `transform(value: number): string` to convert ID to 1**8 format.
Use the pipe in the template: `{{ id | idFormatter }}` to display formatted IDs.
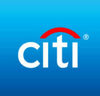
Asked in Citicorp

Q. How do you build form validations in Angular?
Form validations in Angular are built using Angular forms and validators.
Use Angular forms to create form controls and group them together
Apply built-in validators like required, minlength, maxlength, pattern, etc.
Create custom validators for complex validation requirements
Display error messages based on validation status
Use reactive forms for more control and flexibility
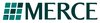
Asked in Merce Technologies

Q. What is the difference between reactive forms and template-driven forms?
Reactive forms are more flexible and allow for complex form validation and handling, while template driven forms are simpler and rely on directives in the template.
Reactive forms are defined programmatically in the component class using form controls, form groups, and form arrays.
Template driven forms are defined in the HTML template using ngModel directive and template variables.
Reactive forms offer more control over form validation and submission logic.
Template driven forms...read more
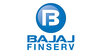
Asked in Bajaj Finserv

Q. What is the method to center a div using SCSS?
Centering a div in SCSS can be achieved using various techniques like flexbox, grid, or margin auto.
Use Flexbox: Set display: flex; and justify-content: center; align-items: center; on the parent.
Example: .parent { display: flex; justify-content: center; align-items: center; } .child { width: 50%; }
Use Grid: Set display: grid; and place the child in the center.
Example: .parent { display: grid; place-items: center; } .child { width: 50%; }
Use Margin Auto: Set width on the chil...read more

Asked in SplendorNet Technologies

Q. What is the purpose of using services?
Services are used to share data and functionality across components in Angular applications.
Services are singleton objects that can be injected into components, directives, and other services.
They are used to share data and functionality across components.
Services can be used to make HTTP requests, handle authentication, and perform other tasks.
They can also be used to encapsulate complex business logic and keep components lean.
Services can be provided at the component level ...read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
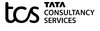
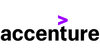
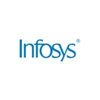
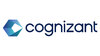
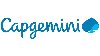
Top Interview Questions for Angular Frontend Developer Related Skills
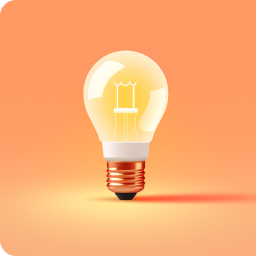
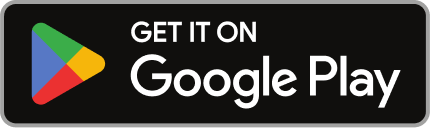
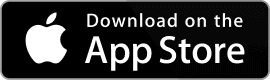
Reviews
Interviews
Salaries
Users
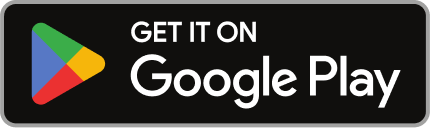
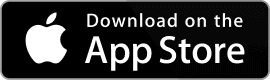