Top 10 Pyspark Interview Questions and Answers
Updated 3 Jul 2025
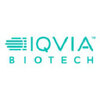
Asked in IQVIA Biotech

Q. What are the key differences between PySpark and Pandas?
Pyspark is a distributed computing framework for big data processing, while Pandas is a library for data manipulation in Python.
Pyspark is designed for big data processing and distributed computing, while Pandas is more suitable for smaller datasets ...read more
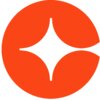
Asked in Cornerstone OnDemand

Q. Write a word count program in PySpark.
A program to count the number of words in a text file using PySpark.
Read the text file using SparkContext
Split the lines into words using flatMap
Map each word to a tuple of (word, 1)
Reduce by key to count the occurrences of each word
Save the output t...read more
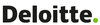
Asked in Deloitte

Q. When should you use PySpark, and when should you use Pandas?
Use Pyspark for big data processing and distributed computing, use pandas for smaller datasets and data manipulation.
Use Pyspark for handling large datasets that don't fit into memory
Use pandas for data manipulation and analysis on smaller datasets t...read more
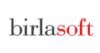
Asked in Birlasoft

Q. What are RDDs in PySpark?
RDD stands for Resilient Distributed Datasets in Pyspark, which are fault-tolerant collections of elements that can be processed in parallel.
RDDs are the fundamental data structure in Pyspark.
They are immutable and can be cached in memory for faster ...read more
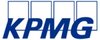
Asked in KPMG India

Q. Write PySpark code to read a CSV file and display the top 10 records.
Pyspark code to read csv file and show top 10 records.
Import the necessary libraries
Create a SparkSession
Read the CSV file using the SparkSession
Display the top 10 records using the show() method
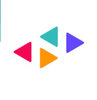
Asked in Nielsen

Q. Write a query to remove duplicate rows in PySpark based on the primary key.
Use dropDuplicates() function in pyspark to remove duplicate rows based on primary key.
Use dropDuplicates() function on the DataFrame with the primary key column specified.
Specify the subset parameter in dropDuplicates() to specify the primary key co...read more
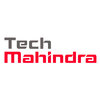
Asked in Tech Mahindra

Q. How do you filter data in PySpark?
Filtering in PySpark involves using the filter function to select rows based on specified conditions.
Use the filter function with a lambda function to specify the condition for filtering
Filter based on column values or complex conditions
Example: df.f...read more
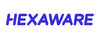
Asked in Hexaware Technologies

Q. Explain the PySpark architecture.
PySpark architecture is based on the Apache Spark architecture, with additional components for Python integration.
PySpark architecture includes Spark Core, Spark SQL, Spark Streaming, MLlib, and GraphX.
It allows Python developers to interact with Spa...read more
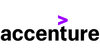
Asked in Accenture

Q. How is data processed using PySpark?
Data is processed using PySpark by creating Resilient Distributed Datasets (RDDs) and applying transformations and actions.
Data is loaded into RDDs from various sources such as HDFS, S3, or databases.
Transformations like map, filter, reduceByKey, etc...read more
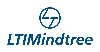
Asked in LTIMindtree

Q. How do you combine two columns in a PySpark DataFrame?
Use the withColumn method in PySpark to combine two columns in a DataFrame.
Use the withColumn method to create a new column by combining two existing columns
Specify the new column name and the expression to combine the two columns
Example: df = df.wit...read more
Pyspark Jobs
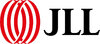
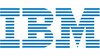
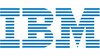
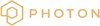
Asked in Photon Interactive

Q. Explain how you handle large data processing in PySpark.
Large data processing in Pyspark involves partitioning, caching, and optimizing transformations for efficient processing.
Partitioning data to distribute workload evenly across nodes
Caching intermediate results to avoid recomputation
Optimizing transfo...read more
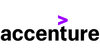
Asked in Accenture

Q. Data manipulations using pyspark
Data manipulations using pyspark involve processing and transforming large datasets using the PySpark framework.
Use PySpark functions like select, filter, groupBy, and join to manipulate data
Utilize RDDs (Resilient Distributed Datasets) and DataFrame...read more
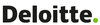
Asked in Deloitte

Q. python vs pyspark
Python is a general-purpose programming language, while PySpark is a distributed computing framework built on top of Spark for big data processing.
Python is a versatile language used for various applications, including web development, data analysis,...read more
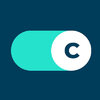
Asked in Concentrix Catalyst

Q. Describe a PySpark scenario to remove regex characters from column values.
Use Pyspark to remove regex characters from column values
Use the regexp_replace function in Pyspark to remove regex characters from column values
Specify the regex pattern to match and the replacement string
Apply the regexp_replace function to the des...read more
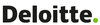
Asked in Deloitte

Q. Using PySpark, how would you find the products with sales for three consecutive years?
Use window function to find products with 3 consecutive years sales in Pyspark
Use window function to partition by product and order by year
Filter the results where the count of consecutive years is 3
Top Interview Questions for Related Skills
Interview Experiences of Popular Companies
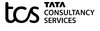
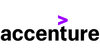
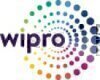
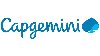
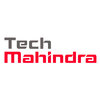
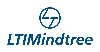
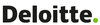
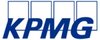
Interview Questions of Pyspark Related Designations
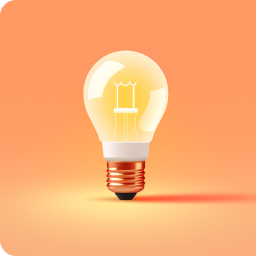
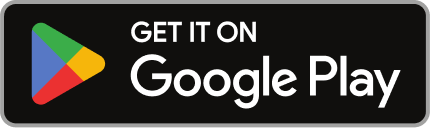
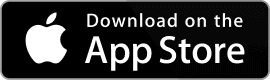
Reviews
Interviews
Salaries
Users
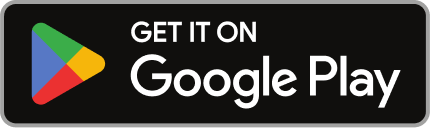
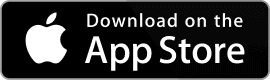