SDE-2
200+ SDE-2 Interview Questions and Answers
Asked in Tower Research Capital LLC

Q. Minimum Cost to Reduce Array
Given an array ARR
of size N
containing positive integers, the task is to reduce the size of the array to 1 by performing a specific operation multiple times. In one operation, you ...read more
Find the minimum cost to reduce an array to one element by merging adjacent elements.
Iterate through the array and merge adjacent elements with the smallest sum each time.
Keep track of the total cost as you merge elements.
Repeat the merging process until only one element remains in the array.
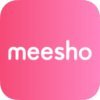
Asked in Meesho

Q. Remove Consecutive Duplicates from String
Given a string STR
consisting of both lower and upper case characters, your task is to remove consecutive duplicate characters and return the newly formed string.
Input...read more
The task is to remove consecutive duplicate characters from a given string and return the new string.
Iterate through the characters of the string
Compare each character with the next character
If they are the same, skip the next character
If they are different, append the current character to the new string
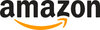
Asked in Amazon

Q. Next Greater Element Problem Statement
You are given an array arr
of length N
. For each element in the array, find the next greater element (NGE) that appears to the right. If there is no such greater element, ...read more
The task is to find the next greater element for each element in an array to its right, if no greater element exists, return -1.
Use a stack to keep track of elements for which the next greater element is not found yet.
Iterate through the array from right to left and pop elements from the stack until a greater element is found.
Store the next greater element for each element in a separate array.
If the stack is empty after iterating through the array, it means there is no greate...read more
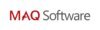
Asked in MAQ Software

Q. Reverse Only Letters Problem Statement
You are given a string S
. The task is to reverse the letters of the string while keeping non-alphabet characters in their original position.
Example:
Input:
S = "a-bcd"
Ou...read more
Reverse the letters of a string while keeping non-alphabet characters in their original position.
Iterate through the string and store the non-alphabet characters in their original positions.
Reverse the letters of the string using two pointers approach.
Combine the reversed letters with the non-alphabet characters to get the final reversed string.
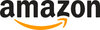
Asked in Amazon

Q. Binary Tree Conversion to Greater Tree
Given a binary tree with 'N' nodes, transform it into a Greater Tree. In this transformation, each node's value should be updated to the sum of its original value and the ...read more
Convert a binary tree into a Greater Tree by updating each node's value to the sum of its original value and the values of all nodes greater than or equal to it.
Traverse the tree in reverse inorder (right, root, left) to update the nodes' values.
Keep track of the sum of nodes visited so far to update each node's value.
Recursively update the node's value by adding the sum of greater nodes to it.
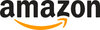
Asked in Amazon

Q. Print Permutations - String Problem Statement
Given an input string 'S', you are tasked with finding and returning all possible permutations of the input string.
Input:
The first and only line of input contains...read more
Return all possible permutations of a given input string.
Use recursion to generate all possible permutations of the input string.
Swap characters at different positions to generate permutations.
Handle duplicate characters by skipping swapping if the characters are the same.
SDE-2 Jobs
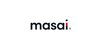

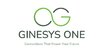
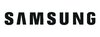
Asked in Samsung

Q. Triplets with Given Sum Problem
Given an array or list ARR
consisting of N
integers, your task is to identify all distinct triplets within the array that sum up to a specified number K
.
Explanation:
A triplet i...read more
The task is to find all distinct triplets in an array that sum up to a specified number.
Iterate through all possible triplets using three nested loops.
Check if the sum of the triplet equals the target sum.
Print the triplet if found, else print -1.
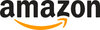
Asked in Amazon

Q. Word Break Problem Statement
You are given a non-empty string without spaces, referred to as 'sentence', and a list of non-empty strings representing a dictionary. Your task is to construct and return all possi...read more
Given a sentence and a dictionary, construct and return all possible sentences by inserting spaces to form words from the dictionary.
Use backtracking to generate all possible combinations of words from the dictionary to form sentences.
Check if a substring of the sentence matches any word in the dictionary, if so, recursively call the function with the remaining substring.
Continue this process until the entire sentence is segmented into words from the dictionary.
Return all val...read more
Share interview questions and help millions of jobseekers 🌟
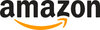
Asked in Amazon

Q. Left View of a Binary Tree
Given a binary tree, your task is to print the left view of the tree. The left view of a binary tree contains the nodes visible when the tree is viewed from the left side.
Input:
The ...read more
The task is to print the left view of a binary tree, which contains the nodes visible when the tree is viewed from the left side.
Traverse the tree level by level and keep track of the leftmost node at each level
Use a queue for level order traversal and a map to store the leftmost nodes
Print the values of the leftmost nodes stored in the map as the left view of the tree
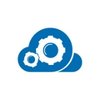
Asked in 42Gears Mobility Systems

Q. Convert Binary Tree to Mirror Tree
Convert a given binary tree into its mirror tree, where the left and right children of all non-leaf nodes are interchanged.
Input:
An integer ‘T’ denoting the number of test c...read more
Convert a binary tree into its mirror tree by interchanging left and right children of non-leaf nodes.
Traverse the tree in a recursive manner and swap the left and right children of each node.
Use a temporary variable to swap the children of each node.
Ensure to modify the tree in place without creating a new tree.
Example: For the input tree 1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1, the mirror tree will have inorder traversal as 7 4 2 1 6 3 5.
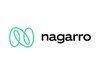
Asked in Nagarro

Q. Equilibrium Index Problem Statement
Given an array Arr
consisting of N integers, your task is to find the equilibrium index of the array.
An index is considered as an equilibrium index if the sum of elements of...read more
Find the equilibrium index of an array where sum of elements on left equals sum on right.
Iterate through the array and calculate prefix sum and suffix sum at each index.
Compare prefix sum and suffix sum to find equilibrium index.
Return the left-most equilibrium index found.

Asked in JPMorgan Chase & Co.

Q. Longest Increasing Path in Matrix Problem Statement
Given a 2-D matrix mat
with 'N' rows and 'M' columns, where each element at position (i, j) is mat[i][j]
, determine the length of the longest increasing path ...read more
The problem involves finding the length of the longest increasing path in a 2-D matrix starting from a given cell.
Use dynamic programming to keep track of the longest increasing path starting from each cell.
Implement a recursive function to explore all possible paths from a cell.
Update the length of the longest path for each cell based on the maximum path length from its neighbors.
Consider edge cases such as boundary conditions and handling negative values in the matrix.
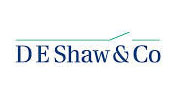
Asked in DE Shaw

Q. Peak Element Finder
For a given array of integers arr
, identify the peak element. A peak element is an element that is greater than its neighboring elements. Specifically, if arr[i]
is the peak, then both arr[i...read more
Find the peak element in an array of integers.
Iterate through the array and check if the current element is greater than its neighbors.
Handle edge cases for the first and last elements of the array.
Return the peak element found.
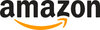
Asked in Amazon

Q. String Transformation Problem
Given a string (STR
) of length N
, you are tasked to create a new string through the following method:
Select the smallest character from the first K
characters of STR
, remove it fr...read more
Given a string, select smallest character from first K characters, remove it, and append to new string until original string is empty.
Iterate through the string, selecting the smallest character from the first K characters each time.
Remove the selected character from the original string and append it to the new string.
Repeat the process until the original string is empty.
Return the final new string formed after the operations.
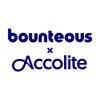
Asked in Bounteous x Accolite

Q. Topological Sort Problem Statement
Given a Directed Acyclic Graph (DAG) consisting of V
vertices and E
edges, your task is to find any topological sorting of this DAG. You need to return an array of size V
repr...read more
Implement a function to find any topological sorting of a Directed Acyclic Graph (DAG).
Use Depth First Search (DFS) to find the topological ordering of the vertices.
Start by visiting a vertex and recursively visit its adjacent vertices before adding it to the result array.
Maintain a visited array to keep track of visited vertices and avoid cycles.
Once all vertices are visited, reverse the result array to get the topological sort.
Example: For a DAG with edges 0->1, 0->3, 1->2,...read more
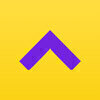
Asked in Housing.com

Q. Word Ladder Problem Statement
Given two strings, BEGIN
and END
, along with an array of strings DICT
, determine the length of the shortest transformation sequence from BEGIN
to END
. Each transformation involves ...read more
The Word Ladder problem involves finding the shortest transformation sequence from one word to another by changing one letter at a time.
Use breadth-first search to find the shortest transformation sequence.
Create a graph where each word is a node and edges connect words that differ by one letter.
Keep track of visited words to avoid revisiting them.
Return -1 if no transformation sequence is possible.
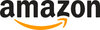
Asked in Amazon

Q. Check for Valid Parentheses
You are given a string STR
containing only the characters "{", "}", "(", ")", "[", and "]". Your task is to determine if the parentheses in the string are balanced.
Input:
The first ...read more
Check if the given string containing only parentheses is balanced or not.
Use a stack to keep track of opening parentheses.
Iterate through the string and push opening parentheses onto the stack.
When a closing parenthesis is encountered, pop from the stack and check if it matches the corresponding opening parenthesis.
If at the end the stack is empty, return 'YES' else return 'NO'.
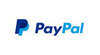
Asked in PayPal

Q. Palindrome Checker Problem Statement
Given an alphabetical string S
, determine whether it is a palindrome or not. A palindrome is a string that reads the same backward as forward.
Input:
The first line of the i...read more
Check if a given string is a palindrome or not.
Iterate through the string from both ends and compare characters.
If all characters match, return 1 indicating a palindrome.
If any characters don't match, return 0 indicating not a palindrome.
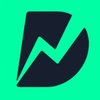
Asked in Dunzo

Q. Anagram Substring Search
Given two strings 'STR' and 'PTR', identify all starting indices of 'PTR' anagram substrings in 'STR'. Two strings are anagrams if one can be rearranged to form the other.
Input:
First ...read more
Identify starting indices of anagram substrings of 'PTR' in 'STR'.
Use a sliding window approach to check for anagrams of 'PTR' in 'STR'.
Create a frequency map of characters in 'PTR' and 'STR' to compare.
Slide the window along 'STR' and check if the frequency maps match.
Return the starting indices where anagrams are found.
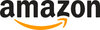
Asked in Amazon

Q. Course Schedule Problem Statement
You are enrolled as a student and must complete N
courses, numbered from 1 to N, to earn your degree.
Some courses have prerequisites, which means that to take course i
, you mu...read more
Determine if it is feasible to complete all courses with prerequisites.
Create a graph representation of the courses and prerequisites.
Check for cycles in the graph using topological sorting.
If there are no cycles, all courses can be completed.
Example: For input N=2, prerequisites=[[1, 2]], the output is 'Yes'.
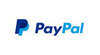
Asked in PayPal

Q. Find The Sum Of The Left Leaves Problem Statement
Given a binary tree with ‘root’, your task is to find and return the sum of all the left leaf nodes.
Example:
Input:
1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1
Outpu...read more
Find and return the sum of all the left leaf nodes in a binary tree.
Traverse the binary tree using depth-first search (DFS)
Check if a node is a leaf node and a left child
Sum up the values of all left leaf nodes
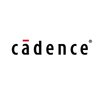
Asked in Cadence Design Systems

Q. Maximum Length Pair Chain Problem
You are provided with 'N' pairs of integers, where the first number in each pair is less than the second number, i.e., in pair (a, b) -> a < b. A pair chain is defined as a seq...read more
Find the length of the longest pair chain that can be formed using the provided pairs.
Sort the pairs based on the second number in each pair.
Iterate through the sorted pairs and keep track of the maximum chain length.
Update the maximum chain length based on the conditions given in the problem statement.
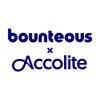
Asked in Bounteous x Accolite

Q. Median of Two Sorted Arrays
Given two sorted arrays A
and B
of sizes N
and M
, find the median of the merged array formed by combining arrays A
and B
. If the total number of elements, N + M
, is even, the median ...read more
Find the median of two sorted arrays by merging them and calculating the median of the combined array.
Merge the two sorted arrays into one sorted array.
Calculate the median of the combined array based on the total number of elements.
Return the median as the result.
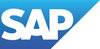
Asked in SAP

Q. Middle Node of a Linked List Problem Statement
Given the head node of a singly linked list, return a pointer to the middle node of the linked list. If the number of elements is odd, return the middle element. I...read more
Return the middle node of a singly linked list, or the latter of the two middle nodes if the number of elements is even.
Traverse the linked list with two pointers, one moving twice as fast as the other.
When the fast pointer reaches the end, the slow pointer will be at the middle node.
If the number of elements is even, return the latter of the two middle nodes.
Handle cases where there is only one node or no midpoint exists.
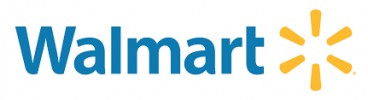
Asked in Walmart

Q. Minimum Cost to Connect Sticks
You are provided with an array, ARR
, of N
positive integers. Each integer represents the length of a stick. The task is to connect all sticks into one by paying a cost to join two...read more
Find the minimum cost to connect all sticks into one by joining two sticks at a time.
Sort the array of stick lengths in non-decreasing order.
Keep adding the two smallest sticks at a time to minimize cost.
Repeat until all sticks are connected into one.
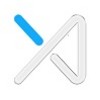
Asked in Arcesium

Q. Minimum Operations to Equalize Array
Given an integer array ARR
of length N
where ARR[i] = (2*i + 1)
, determine the minimum number of operations required to make all the elements of ARR
equal. In a single opera...read more
The minimum number of operations required to make all elements of the given array equal is calculated by finding the median of the array elements.
Calculate the median of the array elements to determine the target value for all elements to be equal.
Find the absolute difference between each element and the target value, sum these differences to get the minimum number of operations.
For odd length arrays, the median is the middle element. For even length arrays, the median is the...read more
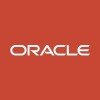
Asked in Oracle

Q. Partition Equal Subset Sum Problem
Given an array ARR
consisting of 'N' positive integers, determine if it is possible to partition the array into two subsets such that the sum of the elements in both subsets i...read more
The problem is to determine if it is possible to partition an array into two subsets with equal sum.
Use dynamic programming to solve this problem efficiently.
Create a 2D array to store the subset sum possibilities.
Check if the total sum of the array is even before attempting to partition.
Iterate through the array and update the subset sum array accordingly.
Return true if the subset sum array contains a sum equal to half of the total sum.
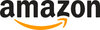
Asked in Amazon

Q. The Ninja Port Problem
Ninja is in a city with 'N' colonies, where each colony contains 'K' houses. He starts at house number "sourceHouse" in colony number "sourceColony" and wants to reach house number "desti...read more
The Ninja Port Problem involves finding the minimum time for a ninja to travel between colonies and houses using secret paths and teleportation.
Calculate the minimum time considering teleportation within colonies, traveling between colonies, and secret paths.
Keep track of the number of secret paths used and ensure they are one-way.
Consider the constraints provided to optimize the solution.
Example: N=2, K=3, S=1, P=1, sourceHouse=1, sourceColony=1, destinationHouse=3, destinat...read more
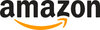
Asked in Amazon

Q. Add Two Numbers Represented by Linked Lists
Your task is to find the sum list of two numbers represented by linked lists and return the head of the sum list.
Explanation:
The sum list should be a linked list re...read more
Add two numbers represented by linked lists and return the head of the sum list.
Traverse both linked lists simultaneously while keeping track of carry from previous sum
Create a new linked list to store the sum of the two numbers
Handle cases where one linked list is longer than the other by padding with zeros
Update the sum and carry values accordingly while iterating through the linked lists
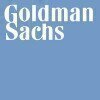
Asked in Goldman Sachs

Q. Arithmetic Progression Queries Problem Statement
Given an integer array ARR
of size N
, perform the following operations:
- update(l, r, val):
Add (val + i)
to arr[l + i]
for all 0 ≤ i ≤ r - l
.
- rangeSum(l, r):...read more
Implement update and rangeSum operations on an integer array based on given queries.
Implement update(l, r, val) by adding (val + i) to arr[l + i] for all i in range (0, r - l).
Implement rangeSum(l, r) to return the sum of elements in the array from index l to r.
Handle queries using 1-based indexing.
Ensure constraints are met for input values.
Output the sum of arr[l..r] for each rangeSum operation.
Interview Experiences of Popular Companies
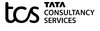
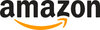
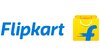
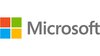
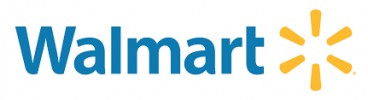
Top Interview Questions for SDE-2 Related Skills
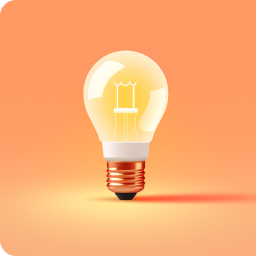
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
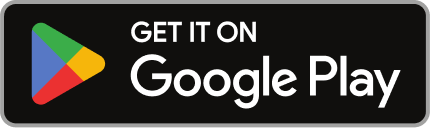
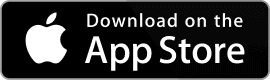
Reviews
Interviews
Salaries
Users
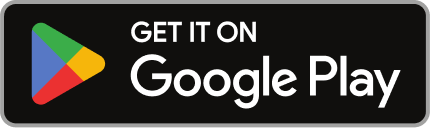
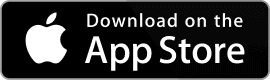