SDE-2
200+ SDE-2 Interview Questions and Answers
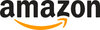
Asked in Amazon

Q. Container with Most Water Problem Statement
Given a sequence of 'N' space-separated non-negative integers A[1], A[2], ..., A[i], ..., A[n], where each number in the sequence represents the height of a line draw...read more
Find two lines to form a container with maximum water area on a Cartesian plane.
Iterate through the array from both ends to find the maximum area.
Calculate the area using the formula: (min(height[left], height[right]) * (right - left)).
Update the pointers based on the height of the lines to maximize the area.
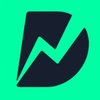
Asked in Dunzo

Q. Minimum Cost to Reduce Array Problem Statement
You are given an array ARR
containing N
positive integers. Your task is to reduce the size of this array to 1 by repetitively merging adjacent elements. Each time ...read more
Find the minimum cost to reduce an array to a single element by merging adjacent elements with their sum.
Iteratively merge adjacent elements to minimize total cost
Choose pairs to merge strategically to reduce cost
Calculate sum of adjacent elements and update array size
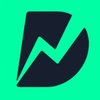
Asked in Dunzo

Q. Number of Subsequences with Even and Odd Sum
Your task is to determine the number of subsequences with odd sums and the number of subsequences with even sums from a given array of positive integers. As resultin...read more
Count the number of subsequences with odd and even sums in a given array of positive integers modulo 10^9 + 7.
Use dynamic programming to keep track of the count of subsequences with odd and even sums.
Consider the parity of each element in the array to determine the count of subsequences with odd and even sums.
Apply modulo 10^9 + 7 to handle large resulting numbers.
Example: For input [1, 2, 3], there are 3 subsequences with odd sums and 4 subsequences with even sums.
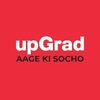
Asked in upGrad

Q. Sort 0 and 1 Problem Statement
Given an integer array ARR
of size N
containing only integers 0 and 1, implement a function to sort this array. The solution should scan the array only once without using any addi...read more
Sort an array of 0s and 1s in linear time without using additional arrays.
Use two pointers approach to swap 0s to the left and 1s to the right.
Keep track of the leftmost index of 1s to place 0s before it.
Iterate through the array once and swap elements accordingly.
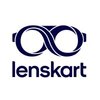
Asked in Lenskart

Q. LRU Cache Implementation Problem
Design and implement a Least Recently Used (LRU) cache data structure. This cache must support the following operations efficiently:
get(key)
: Return the value associated with ...read more
Implement a Least Recently Used (LRU) cache data structure that supports get and put operations efficiently.
Design a data structure that maintains a cache with a specified capacity.
Implement get(key) function to return value associated with key or -1 if key doesn't exist.
Implement put(key, value) function to insert/update key-value pair in cache.
Invalidate least recently used item when cache reaches its capacity.
Handle different types of operations efficiently based on input....read more
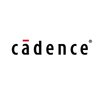
Asked in Cadence Design Systems

Q. Minimum Number of Jumps Problem
Given an array ARR
of N
integers, determine the minimum number of jumps required to reach the last index of the array (i.e., N - 1
). From any index i
, you can jump to an index i ...read more
The problem involves finding the minimum number of jumps required to reach the last index of an array, where each element indicates the maximum distance that can be jumped from that position.
Use a greedy approach to keep track of the farthest reachable index and the current end of the jump.
Iterate through the array, updating the farthest reachable index and incrementing the jump count when necessary.
Return the jump count as the minimum number of jumps needed to reach the last...read more
SDE-2 Jobs
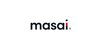

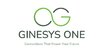
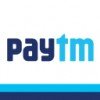
Asked in Paytm

Q. Problem: Sort an Array of 0s, 1s, and 2s
Given an array/list ARR
consisting of integers where each element is either 0, 1, or 2, your task is to sort this array in increasing order.
Input:
The input starts with...read more
The task is to sort an array of 0s, 1s, and 2s in increasing order.
Use a three-pointer approach to partition the array into three sections: 0s, 1s, and 2s.
Initialize three pointers: low, mid, and high. low points to the start of the array, mid points to the current element being processed, and high points to the end of the array.
While mid <= high, if ARR[mid] is 0, swap ARR[low] and ARR[mid], increment low and mid. If ARR[mid] is 1, increment mid. If ARR[mid] is 2, swap ARR[m...read more
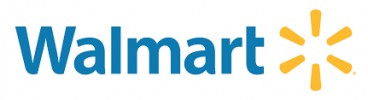
Asked in Walmart

Q. Maximum Depth of a Binary Tree Problem Statement
Given the root node of a binary tree with N
nodes, where each node contains integer values, determine the maximum depth of the tree. The depth is defined as the ...read more
Find the maximum depth of a binary tree given its level order traversal.
Traverse the tree level by level and keep track of the depth using a queue data structure.
For each level, increment the depth by 1 until all nodes are processed.
Return the maximum depth encountered during traversal as the final result.
Example: For input [5, 10, 15, 20, -1, 25, 30, -1, 40, -1, -1, -1, -1, 45], the maximum depth is 7.
Share interview questions and help millions of jobseekers 🌟
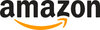
Asked in Amazon

Q. Sliding Window Maximum Problem Statement
You are given an array/list of integers with length 'N'. A sliding window of size 'K' moves from the start to the end of the array. For each of the 'N'-'K'+1 possible wi...read more
Sliding window maximum problem where we find maximum element in each window of size K.
Use a deque to store indices of elements in decreasing order within the window.
Pop elements from the deque that are out of the current window.
Add the maximum element to the result for each window.
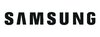
Asked in Samsung

Q. Reverse Linked List Problem Statement
Given a Singly Linked List of integers, your task is to reverse the Linked List by altering the links between the nodes.
Input:
The first line of input is an integer T, rep...read more
Reverse a singly linked list by altering the links between nodes.
Iterate through the linked list and reverse the links between nodes
Use three pointers to keep track of the current, previous, and next nodes
Update the links between nodes to reverse the list
Return the head of the reversed linked list
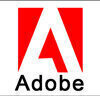
Asked in Adobe

Q. Wildcard Pattern Matching Problem Statement
Implement a wildcard pattern matching algorithm to determine if a given wildcard pattern matches a text string completely.
The wildcard pattern may include the charac...read more
Implement a wildcard pattern matching algorithm to determine if a given wildcard pattern matches a text string completely.
Create a dynamic programming matrix to store intermediate results
Handle cases for '?' and '*' characters separately
Check if the characters in the pattern and text match accordingly
Return 'True' if the text matches the pattern, otherwise 'False'
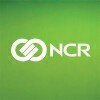
Asked in NCR Corporation

Q. Job Sequencing Problem Statement
You are provided with a N x 2 2-D array called Jobs
consisting of N
jobs. In this array, Jobs[i][0]
represents the deadline of the i-th job, while Jobs[i][1]
indicates the profi...read more
Maximize profit by scheduling jobs within their deadlines.
Sort the jobs based on their profits in descending order.
Iterate through the sorted jobs and schedule them based on their deadlines.
Keep track of the maximum profit achievable by scheduling jobs within their deadlines.
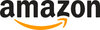
Asked in Amazon

Q. Rat In a Maze Problem Statement
Given a N * N
maze with a rat placed at position MAZE[0][0]
, find and print all possible paths for the rat to reach its destination at MAZE[N-1][N-1]
. The rat is allowed to move ...read more
The problem involves finding all possible paths for a rat to reach its destination in a maze.
Use backtracking to explore all possible paths in the maze.
Mark visited cells to avoid revisiting them.
Return the path once the destination is reached.
Handle edge cases like blocked cells and out-of-bounds movements.
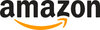
Asked in Amazon

Q. Validate Binary Search Tree Problem Statement
Given a binary tree with 'N' nodes, determine if it is a Binary Search Tree (BST). Return true
if the tree is a BST, otherwise return false
.
Example:
Input:
1 2 3 4...read more
Validate if a given binary tree is a Binary Search Tree (BST) or not.
Check if the left subtree of a node contains only nodes with values less than the node's value.
Check if the right subtree of a node contains only nodes with values greater than the node's value.
Ensure that both the left and right subtrees are also binary search trees.
Traverse the tree in-order and check if the elements are in sorted order.
Use a recursive function to validate each node's value against its sub...read more

Asked in IQVIA

Q. Minimum Knight Moves Problem
Given a square chessboard of size N x N
, determine the minimum number of moves a knight requires to reach a target position from a starting position.
Input:
The first line of input...read more
The problem involves finding the minimum number of moves a knight requires to reach a target position from a starting position on a chessboard.
Use Breadth First Search (BFS) algorithm to find the shortest path from starting position to target position.
Consider all possible moves of the knight from its current position to explore the chessboard.
Keep track of visited positions to avoid revisiting the same position.
Calculate the minimum number of steps needed to reach the target...read more
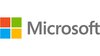
Asked in Microsoft Corporation

Q. Time to Burn Tree Problem
You are given a binary tree consisting of 'N' unique nodes and a start node where the burning will commence. The task is to calculate the time in minutes required to completely burn th...read more
Calculate the time in minutes required to completely burn a binary tree starting from a given node.
Start burning from the given node and spread fire to adjacent nodes each minute
Track the time taken for each node to burn completely
Return the maximum time taken to burn the entire tree
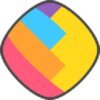
Asked in ShareChat

Q. Find Minimum in Rotated Sorted Array
You are presented with a sorted array that has been rotated an unknown number of times. The nature of this rotation is such that each element shifts to the right with the la...read more
Find the smallest element in a rotated sorted array.
Use binary search to find the pivot point where rotation occurs.
Compare the element at the pivot point to determine the smallest element.
Handle cases where the array is not rotated or has only one element.
Time complexity should be O(log(N)) and space complexity O(1).
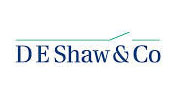
Asked in DE Shaw

Q. Minimum Steps for a Knight to Reach Target
Given a square chessboard of size 'N x N', determine the minimum number of moves a Knight requires to reach a specified target position from its initial position.
Expl...read more
Calculate the minimum number of moves a Knight requires to reach a specified target position on a chessboard.
Use breadth-first search (BFS) algorithm to find the shortest path for the Knight.
Consider all possible moves of the Knight on the chessboard.
Keep track of visited positions to avoid revisiting them.
Return the minimum number of moves required to reach the target position.
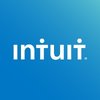
Asked in Intuit

Q. Count Pairs with Given Sum
Given an integer array/list arr
and an integer 'Sum', determine the total number of unique pairs in the array whose elements sum up to the given 'Sum'.
Input:
The first line contains ...read more
Count the total number of unique pairs in an array whose elements sum up to a given value.
Use a hashmap to store the frequency of each element in the array.
Iterate through the array and for each element, check if (Sum - current element) exists in the hashmap.
Increment the count of pairs if the complement exists in the hashmap.
Divide the count by 2 to avoid counting duplicates like (arr[i], arr[j]) and (arr[j], arr[i]) separately.
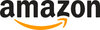
Asked in Amazon

Q. Max Submatrix Problem Statement
You are provided with a matrix MAT
consisting of integers, with dimensions N x M
(i.e., N rows and M columns). Your objective is to determine the maximum sum submatrix within thi...read more
Find the maximum sum submatrix in a given matrix.
Iterate over all possible submatrices and calculate their sums
Use Kadane's algorithm to find the maximum sum subarray in each row
Combine the sums of rows to find the maximum sum submatrix
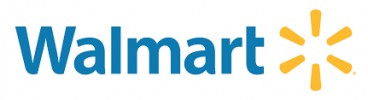
Asked in Walmart

Q. Maximum Number With Single Swap
You are given an array of N elements that represent the digits of a number. You can perform one swap operation to exchange the values at any two indices. Your task is to determin...read more
Given an array of digits, find the maximum number that can be achieved by performing at most one swap.
Iterate through the array to find the maximum digit.
If the maximum digit is already at the beginning, find the next maximum digit and swap them.
If the maximum digit is not at the beginning, swap it with the digit at the beginning.
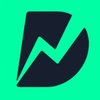
Asked in Dunzo

Q. Maximum Size Rectangle Binary Sub-Matrix with All 1s
Given a binary-valued matrix of size N x M
, your task is to determine the largest area of a submatrix that contains only 1's.
Input:
The first line contains ...read more
Find the largest area of a submatrix containing only 1's in a binary matrix.
Iterate over each cell in the matrix and calculate the maximum area of submatrix with all 1's ending at that cell.
Use dynamic programming to keep track of the maximum area ending at each cell.
Update the maximum area as you iterate through the matrix.
Return the overall maximum area found in the matrix.
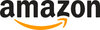
Asked in Amazon

Q. Median in a Stream Problem Statement
Your task is to determine the median of integers as they are read from a data stream. The median is the middle value in the ordered list of numbers. If the list length is ev...read more
Find median of integers in a data stream as they are read.
Use two heaps - max heap for lower half of numbers and min heap for upper half.
Keep the size of two heaps balanced to find the median efficiently.
Handle even and odd number of elements separately to calculate median.
Return vector of medians after each element is read from the stream.
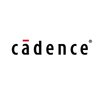
Asked in Cadence Design Systems

Q. Two Teams (Check Whether Graph is Bipartite or Not)
Determine if a given undirected graph can be divided into exactly two disjoint cliques. Print 1 if possible, otherwise print 0.
Input:
The first line contains...read more
Check if a given undirected graph can be divided into exactly two disjoint cliques.
Create an adjacency list to represent the graph
Use BFS or DFS to check if the graph is bipartite
If the graph is bipartite, it can be divided into two disjoint cliques
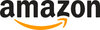
Asked in Amazon

Q. Loot Houses Problem Statement
A thief is planning to steal from several houses along a street. Each house has a certain amount of money stashed. However, the thief cannot loot two adjacent houses. Determine the...read more
Determine the maximum amount of money a thief can steal from houses without looting two consecutive houses.
Use dynamic programming to keep track of the maximum money that can be stolen up to each house.
At each house, the thief can either choose to steal from the current house or skip it and steal from the previous house.
The maximum amount of money that can be stolen without looting two consecutive houses is the maximum of the two options.
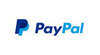
Asked in PayPal

Q. Rearrange String Problem Statement
Given a string ‘S’, your task is to rearrange its characters so that no two adjacent characters are the same. If it's possible, return any such arrangement, otherwise return “...read more
Given a string, rearrange its characters so that no two adjacent characters are the same.
Iterate through the string and count the frequency of each character.
Use a priority queue to rearrange the characters based on their frequency.
Check if it's possible to rearrange the string without any two adjacent characters being the same.
Return 'Yes' if possible, 'No' otherwise.
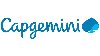
Asked in Capgemini

Q. Trailing Zeros in Factorial Problem
Find the number of trailing zeroes in the factorial of a given number N
.
Input:
The first line contains an integer T
representing the number of test cases.
Each of the followi...read more
Count the number of trailing zeros in the factorial of a given number.
Count the number of factors of 5 in the factorial of the given number N.
Divide N by 5, then by 25, then by 125, and so on, and sum up the quotients to get the total number of trailing zeros.
Example: For N=10, 10/5=2, so there are 2 factors of 5 in 10!, hence 2 trailing zeros.
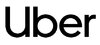
Asked in Uber

Q. Best Insert Position for a Target in a Sorted Array
You are provided with a sorted array A
of length N
consisting of distinct integers and a target integer M
. Your task is to determine the position where M
woul...read more
Find the best insert position for a target in a sorted array to maintain order.
Use binary search to find the correct position for the target integer in the sorted array.
If the target is already present in the array, return its index.
Maintain the sorted order of the array after inserting the target integer.
Handle edge cases like empty array or target being smaller than the first element or larger than the last element.
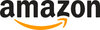
Asked in Amazon

Q. Flatten Multi-Level Linked List
You are given a multi-level linked list containing 'N' nodes. Each node has 'next' and 'child' pointers that may or may not point to other nodes. Your task is to flatten this mul...read more
Flatten a multi-level linked list into a single linked list by rearranging the pointers.
Traverse the linked list and whenever a node has a child, flatten the child list and append it after the current node.
Update the 'next' and 'child' pointers accordingly while flattening the list.
Continue this process until all nodes are rearranged into a single linked list.
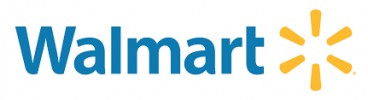
Asked in Walmart

Q. House Robber Problem Statement
Mr. X is a professional robber with a plan to rob houses arranged in a circular street. Each house has a certain amount of money hidden, separated by a security system that alerts...read more
House Robber problem - find maximum amount of money Mr. X can rob without triggering alarm in circular street.
Use dynamic programming to keep track of maximum money robbed at each house.
Consider two cases - robbing the first house and not robbing the first house.
Handle circular arrangement by considering the first and last houses separately.
Return the maximum amount of money that can be robbed without triggering the alarm.
Interview Experiences of Popular Companies
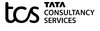
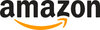
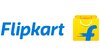
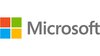
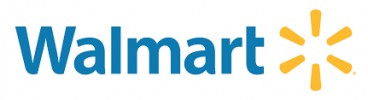
Top Interview Questions for SDE-2 Related Skills
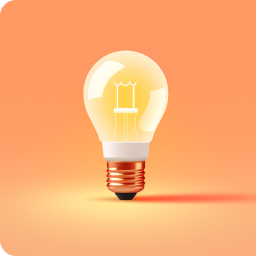
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
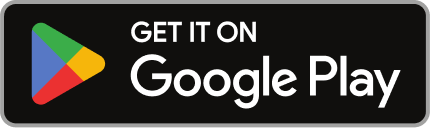
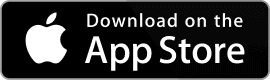
Reviews
Interviews
Salaries
Users
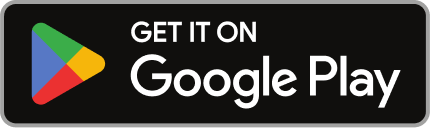
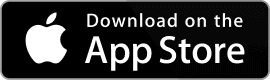