Reactjs Developer
30+ Reactjs Developer Interview Questions and Answers for Freshers
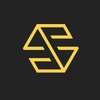
Asked in Studio Graphene

Q. Implement a counter with increment and decrement buttons. Include an input field to set the counter's initial value, and ensure increment/decrement operations are based on this input value.
Implement a counter with increment and decrement buttons and an input field to set the value.
Create a state variable to hold the counter value
Use onClick event handlers for the buttons to increment and decrement the counter
Use onChange event handler for the input field to update the counter value
Set the initial value of the counter to 0
Validate the input value to ensure it is a number
Asked in NewFangled Vision

Q. Coding Question, Find largest element in array without sort, find sum of all element in array, find count of each element in array like how many times each element occurred in array?
Find largest element, sum of all elements, and count of each element in array without sorting.
To find the largest element, iterate through the array and keep track of the maximum value.
To find the sum of all elements, iterate through the array and add each element to a running total.
To find the count of each element, use a hashmap to store the count of each element as you iterate through the array.
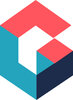
Asked in Genpact

Q. How is data passed from children to parents in React components?
Data can be passed from children to parents in React components by using callback functions.
Use callback functions to pass data from child components to parent components
Parent component passes a function as a prop to child component
Child component calls the function with the data as an argument to pass data to parent component
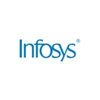
Asked in Infosys

Q. What is the difference between state and props?
States are mutable data managed within a component, while props are immutable data passed from parent to child components.
States are managed within a component and can be changed using setState method
Props are passed from parent to child components and are immutable
States are used for internal component data management, while props are used for passing data between components
Example: A counter component may have a state for the count value, while receiving a prop for the incr...read more
Asked in NewFangled Vision

Q. How do you communicate from child to parent components in React?
Using props and callbacks to communicate data from child to parent components in React.
Passing data from child to parent components using props
Using callbacks to send data from child to parent components
Using context API for global state management
Asked in Mobyink Innovation

Q. What are the different methods of synchronous programming in JavaScript?
Synchronous programming in JavaScript executes code sequentially, blocking further execution until the current task completes.
1. Function Calls: Functions execute in the order they are called. Example: `functionA(); functionB();`
2. Loops: Iterative structures like `for` and `while` run synchronously. Example: `for (let i = 0; i < 5; i++) { console.log(i); }`
3. Conditional Statements: `if` and `switch` statements execute synchronously based on conditions. Example: `if (x > 10)...read more
Reactjs Developer Jobs
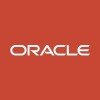
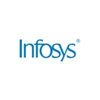
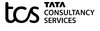
Asked in P99soft

Q. Development cycle of a student registration app
The development cycle of a student registration app involves planning, designing, developing, testing, and deploying the app.
Plan the app's features and functionality
Design the user interface and user experience
Develop the app using Reactjs and other technologies
Test the app for bugs and errors
Deploy the app to a server or hosting platform
Continuously maintain and update the app as needed
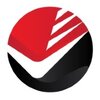
Asked in VRIZE

Q. Write code using JavaScript with functions.
This code demonstrates a simple JavaScript function that adds two numbers and returns the result.
Define a function using the 'function' keyword: function add(a, b) { return a + b; }
Call the function with arguments: let sum = add(5, 10);
Use console.log to display the result: console.log(sum);
Functions can also be assigned to variables: const add = function(a, b) { return a + b; };
Share interview questions and help millions of jobseekers 🌟
Asked in Senwell Solutions

Q. What is useState and why do we use it?
useState is a hook in React that allows functional components to have state.
useState is used to add state to functional components in React.
It returns an array with two elements - the current state value and a function to update that value.
Example: const [count, setCount] = useState(0);
Asked in NewFangled Vision

Q. Explain the position properties: Absolute, Fixed, and Relative.
Position properties in CSS for element placement.
Absolute: Element is positioned relative to its closest positioned ancestor.
Fixed: Element is positioned relative to the viewport.
Relative: Element is positioned relative to its normal position.
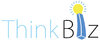
Asked in Thinkbiz Technology

Q. What is React and what makes it faster?
React is a JavaScript library for building user interfaces, known for its virtual DOM and efficient rendering.
React uses a virtual DOM to minimize actual DOM manipulation, improving performance.
It employs a reconciliation algorithm to efficiently update the UI when data changes.
React Fiber, a reimplementation of the core algorithm, enables incremental rendering for better user experience.
React's component-based architecture allows for reusable and modular code, enhancing deve...read more
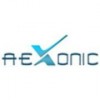
Asked in Aexonic Technologies

Q. Describe a small task involving fetching data from an API.
Demonstrating how to fetch data from an API using React.js with hooks.
Use the useEffect hook to fetch data when the component mounts.
Utilize the fetch API or axios for making HTTP requests.
Store the fetched data in a state variable using useState.
Handle loading and error states to improve user experience.
Example: const [data, setData] = useState([]); useEffect(() => { fetch('API_URL').then(response => response.json()).then(data => setData(data)); }, []);
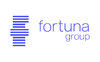
Asked in Fortuna Group

Q. What are semantic elements?
Semantic elements are HTML tags that clearly define the content they contain, making it easier for both humans and machines to understand the structure of a web page.
Semantic elements provide meaning to the content they enclose, improving accessibility and SEO.
Examples include <header>, <footer>, <nav>, <article>, <section>, <aside>, <main>, <figure>, <figcaption>.
Using semantic elements helps organize the content of a web page in a more structured and meaningful way.
Asked in Senwell Solutions

Q. What are JSX and Babel?
JSX is a syntax extension for JavaScript that allows writing HTML-like code within JavaScript. Babel is a tool used to transpile JSX code into plain JavaScript.
JSX stands for JavaScript XML and is used to write HTML-like code in React components
Babel is a JavaScript compiler that converts JSX code into plain JavaScript for browser compatibility
JSX allows developers to write UI components in a more declarative and readable way
Example:
Hello, World!in JSX gets transpiled to Re...read more
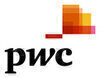
Asked in PwC

Q. What is docType in HTML?
DocType in HTML specifies the version of HTML being used in the document.
DocType declaration is not an HTML tag.
It is used to inform the web browser about the version of HTML being used.
It is placed at the very beginning of an HTML document before the <html> tag.
Example: <!DOCTYPE html> for HTML5.
Asked in Senwell Solutions

Q. What are the different hooks available in React?
Hooks are functions that let you use state and other React features without writing a class.
Hooks are introduced in React 16.8.
They allow you to use state and other React features in functional components.
Some commonly used hooks are useState, useEffect, useContext, and useReducer.
Hooks help in reusing logic across components and making code more readable.
Example: useState hook is used to add state to functional components.
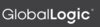
Asked in GlobalLogic

Q. Write a JavaScript function to reverse a string.
Reverse a string in JavaScript
Use the split() method to convert the string into an array
Use the reverse() method to reverse the array
Use the join() method to convert the array back to a string
Asked in Swasthya AI

Q. Write a function that converts a number to its word representation (e.g., 102 becomes 'one hundred two').
Convert a number into words
Break the number into groups of three digits
Convert each group into words (e.g. '102' becomes 'one hundred two')
Combine the words with appropriate thousand/million/billion separators
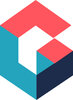
Asked in Genpact

Q. What is reconciliation?
Reconciliation is the process of updating the UI to match the current state of the application.
Reconciliation is the algorithm used by React to update the DOM efficiently.
It compares the virtual DOM with the actual DOM and only updates the parts that have changed.
Reconciliation is a key feature of React that helps in improving performance by minimizing DOM updates.
Examples of reconciliation include adding, updating, or removing elements in the UI based on changes in the appli...read more
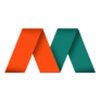
Asked in Mactosys Software Solution

Q. What are states in React?
States in React are mutable data that control the behavior of a component and determine what is rendered on the screen.
States are used to store and manage data within a component.
They are mutable and can be updated using the setState() method.
Changes in state trigger re-rendering of the component.
States are initialized in the constructor of a class component or using the useState hook in functional components.
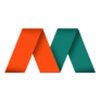
Asked in Mactosys Software Solution

Q. Write code to print a pyramid pattern.
Print pyramid pattern using nested loops
Use nested loops to print each row of the pyramid
Increment spaces and decrement stars in each row
Example: for a pyramid with 5 rows -
*
***
*****
*******
*********
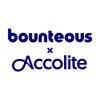
Asked in Bounteous x Accolite

Q. What are props in React?
Props are read-only properties that are passed from a parent component to a child component in React.
Props allow data to be passed between components in a unidirectional flow.
Props are immutable and cannot be modified by the child component.
Props can be used to customize the behavior or appearance of a component.
Props are accessed using the 'this.props' syntax in class components or as function arguments in functional components.
Example:
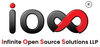
Asked in Infinite Open Source Solutions

Q. What is closure in JavaScript?
Closure is a feature in JavaScript that allows a function to access variables from its outer scope even after the function has finished executing.
Closure is created when a function is defined inside another function.
The inner function has access to the variables and parameters of the outer function.
The inner function forms a closure with the outer function, preserving the state of the outer function's variables.
Closures are commonly used to create private variables and functi...read more
Asked in Senwell Solutions

Q. What are the differences between the Real DOM and the Virtual DOM?
Real DOM is the actual representation of the UI, while Virtual DOM is a lightweight copy used by React for efficient updates.
Real DOM updates are slow and involve direct manipulation of the UI, which can lead to performance issues.
Virtual DOM is a JavaScript object that represents the Real DOM, allowing React to optimize updates.
When a component's state changes, React updates the Virtual DOM first, then compares it with the Real DOM (diffing).
Only the changed elements are upd...read more
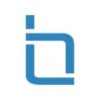
Asked in LeewayHertz Technologies

Q. Tell me about the useEffect hooks.
useEffect is a React hook that manages side effects in functional components, enabling lifecycle-like behavior.
useEffect runs after every render by default, allowing you to perform side effects like data fetching.
You can control when useEffect runs by passing a dependency array as the second argument.
Example: useEffect(() => { fetchData(); }, [dependency]); runs fetchData only when 'dependency' changes.
Cleanup can be handled by returning a function from useEffect, which runs ...read more
Asked in Classforma AI

Q. What is state in React?
State in React is a JavaScript object that stores data relevant to a component.
State is mutable and can be updated using the setState() method
State is local and specific to a component
Changes to state trigger re-rendering of the component
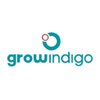
Asked in Grow Indigo

Q. What is React and why should it be used?
React is a JavaScript library for building user interfaces.
React is used for creating interactive and dynamic user interfaces.
It allows for building reusable UI components.
React uses a virtual DOM for efficient rendering.
React can be used for single-page applications, mobile apps, and more.
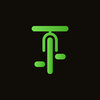
Asked in Pedals Up

Q. What is useState?
useState is a hook in React that allows functional components to have state.
useState is a built-in hook in React.
It allows functional components to have state.
It returns an array with two elements: the current state value and a function to update the state.
The initial state can be passed as an argument to useState.
The state can be of any data type, such as a string, number, boolean, or object.
Example: const [count, setCount] = useState(0);
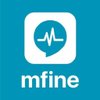
Asked in Mfine

Q. How early can you start?
The candidate can start immediately or as soon as required.
Candidate can start immediately if needed
Availability can be discussed during the interview process
Flexibility in start date is appreciated
Asked in Devkraft Technologies

Q. lifecyle of a component
The lifecycle of a component refers to the different stages a component goes through from creation to deletion.
Mounting: When a component is created and inserted into the DOM.
Updating: When a component's state or props change.
Unmounting: When a component is removed from the DOM.
Examples of lifecycle methods include componentDidMount, componentDidUpdate, and componentWillUnmount.
Lifecycle methods can be used to perform actions at specific stages of a component's lifecycle.
Interview Experiences of Popular Companies
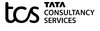
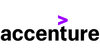
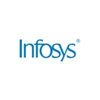
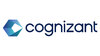
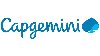
Top Interview Questions for Reactjs Developer Related Skills
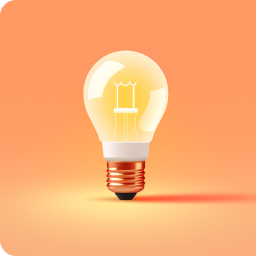
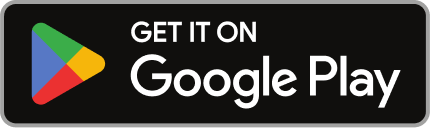
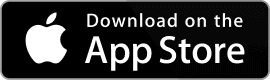
Reviews
Interviews
Salaries
Users
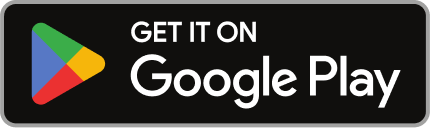
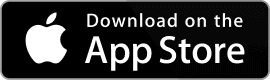