React Developer
100+ React Developer Interview Questions and Answers
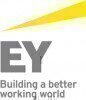
Asked in Ernst & Young

slice() returns a shallow copy of a portion of an array without modifying the original array, while splice() changes the contents of an array by removing or replacing existing elements.
slice() does not modify the original array, while splice() does
slice() returns a new array, while splice() returns the removed elements
slice() takes start and end index as arguments, while splice() takes start index, number of elements to remove, and optional elements to add as arguments
Example...read more
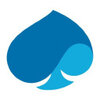
Asked in Capgemini Engineering

Q. What are the differences between let, const, and var? Provide code examples and explanations.
Let, Const, and Var are used to declare variables in JavaScript with different scoping and reassignment abilities.
Var has function scope and can be redeclared and reassigned.
Let has block scope and can be reassigned but not redeclared.
Const has block scope and cannot be reassigned or redeclared.
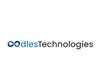
Asked in OodlesTechnologies

No, const does not make the value immutable.
const only makes the variable itself immutable, not the value it holds.
For objects and arrays, const prevents reassignment but allows mutation of properties or elements.
To make a value truly immutable, you can use Object.freeze() or other techniques.
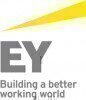
Asked in Ernst & Young

Use the built-in sort() method to sort an array of integers in JavaScript.
Use the sort() method with a compare function to sort the array in ascending order.
For descending order, modify the compare function to return b - a instead of a - b.
Example: const numbers = [4, 2, 5, 1, 3]; numbers.sort((a, b) => a - b);
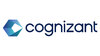
Asked in Cognizant

Q. How do you optimize a React App?
Optimize React apps by improving performance, reducing load times, and enhancing user experience through various techniques.
Use React.memo to prevent unnecessary re-renders of functional components.
Implement code splitting with React.lazy and Suspense to load components only when needed.
Utilize the useCallback and useMemo hooks to memoize functions and values, reducing recalculations.
Optimize images and assets to decrease load times, using formats like WebP.
Leverage the React...read more
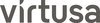
Asked in Virtusa Software Services

Q. How Promise works? What is Promise.all. Write code for both.
Promises are a way to handle asynchronous operations in JavaScript. Promise.all is used to execute multiple promises concurrently.
Promises represent a value that may not be available yet
They have three states: pending, fulfilled, and rejected
Promise.all takes an array of promises and returns a new promise that resolves when all promises in the array have resolved
If any promise in the array is rejected, the returned promise is rejected with the reason of the first promise that...read more
React Developer Jobs
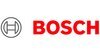
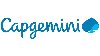
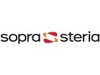
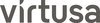
Asked in Virtusa Software Services

Q. What are Hooks in React? Explain useState and useEffect hooks.
Hooks are functions that allow you to use state and other React features without writing a class.
useState is a hook that allows you to add state to functional components.
useEffect is a hook that allows you to perform side effects in functional components.
Hooks can only be used in functional components.
Hooks must be called at the top level of a functional component.
Hooks can be used to replace lifecycle methods in class components.
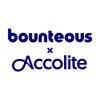
Asked in Bounteous x Accolite

Functional Components are simple functions that take props as input and return JSX, while Class Components are ES6 classes that extend React.Component and have additional features like state and lifecycle methods.
Functional Components are simpler and easier to read/write compared to Class Components.
Functional Components do not have access to state or lifecycle methods, while Class Components do.
Functional Components are stateless, while Class Components can be stateful.
Funct...read more
Share interview questions and help millions of jobseekers 🌟
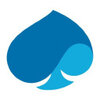
Asked in Capgemini Engineering

Q. Explain the lifecycle methods in React class components.
React class components have a lifecycle that includes mounting, updating, and unmounting phases with specific methods for each.
Mounting: Methods called when a component is being created and inserted into the DOM. Key methods: constructor(), render(), componentDidMount().
Updating: Methods called when a component's state or props change. Key methods: shouldComponentUpdate(), render(), componentDidUpdate().
Unmounting: Method called when a component is being removed from the DOM....read more
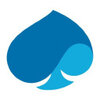
Asked in Capgemini Engineering

Q. How can you optimize a React App?
Optimizing a React app involves reducing bundle size, using lazy loading, and optimizing rendering performance.
Reduce bundle size by code splitting and using dynamic imports
Use lazy loading to load components only when needed
Optimize rendering performance by using shouldComponentUpdate and PureComponent
Use React.memo to memoize functional components
Avoid unnecessary re-renders by using useMemo and useCallback
Use performance profiling tools like React DevTools and Chrome DevTo...read more
Asked in Patona Technologies

Q. What are Hooks? What all Hooks have you used in your project?
Hooks are functions that allow you to use state and other React features without writing a class.
useState() - for managing state in functional components
useEffect() - for performing side effects in functional components
useContext() - for consuming context in functional components
useReducer() - for managing complex state in functional components
useCallback() - for memoizing functions in functional components
useMemo() - for memoizing values in functional components
useRef() - fo...read more
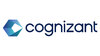
Asked in Cognizant

Q. What is Lazy Loading, Suspense. How do they work?
Lazy Loading and Suspense are techniques used to improve performance by loading components and data only when needed.
Lazy Loading delays the loading of non-critical resources until they are needed, reducing initial load time.
Suspense allows components to wait for data to load before rendering, improving user experience.
Lazy Loading and Suspense can be used together to optimize performance and user experience.
Example: A website with a large image gallery can use Lazy Loading t...read more
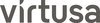
Asked in Virtusa Software Services

Q. Features of ES6. Explain Spread Operator and Rest Parameter by writing code. Give example for Object Destructuring.
ES6 features: Spread Operator, Rest Parameter, Object Destructuring
Spread Operator: allows an iterable to be expanded into individual elements
Rest Parameter: allows a function to accept an indefinite number of arguments as an array
Object Destructuring: allows extracting properties from an object and assigning them to variables
Asked in Patona Technologies

Q. How do you implement responsiveness in an application based on screen sizes?
Responsiveness in app can be achieved using CSS media queries and responsive design principles.
Use CSS media queries to apply different styles based on screen sizes
Design the app layout to be flexible and adapt to different screen sizes
Use responsive units like percentages and viewport units for sizing elements
Test the app on different devices and screen sizes to ensure responsiveness
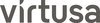
Asked in Virtusa Software Services

Q. What is the significance of the 'this' keyword in JS?
The 'this' keyword in JS refers to the object that is currently executing the code.
The value of 'this' depends on how a function is called.
In a method, 'this' refers to the object that the method belongs to.
In a regular function, 'this' refers to the global object (window in a browser).
In an event handler, 'this' refers to the element that triggered the event.
The value of 'this' can be explicitly set using call(), apply(), or bind() methods.
Asked in Patona Technologies

Q. Explain the workflow of Redux.
Redux manages application state through a unidirectional data flow using actions, reducers, and the store.
1. **Store**: Centralized state container that holds the application state. Example: `const store = createStore(reducer);`
2. **Actions**: Plain JavaScript objects that describe what happened. Example: `{ type: 'ADD_TODO', payload: { text: 'Learn Redux' } }`
3. **Reducers**: Pure functions that take the current state and an action, returning a new state. Example: `function ...read more
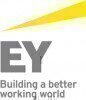
Asked in Ernst & Young

A first-class function in JavaScript is a function that can be treated like any other variable.
Can be passed as an argument to other functions
Can be returned from other functions
Can be assigned to variables
Can be stored in data structures
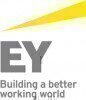
Asked in Ernst & Young

React uses a diffing algorithm called Virtual DOM to efficiently update the actual DOM based on changes in state or props.
Virtual DOM is a lightweight copy of the actual DOM.
React compares the Virtual DOM with the previous Virtual DOM to identify the minimal number of changes needed to update the actual DOM.
This process is known as reconciliation and helps in optimizing performance by reducing unnecessary re-renders.
Example: If a component's state changes, React will update t...read more
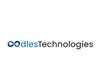
Asked in OodlesTechnologies

Custom Hooks are reusable functions in React that allow you to extract logic from components.
Custom Hooks are functions that start with the word 'use' and can call other Hooks if needed.
They are used to share stateful logic between components without using higher-order components or render props.
Custom Hooks can be used to handle common tasks like fetching data, managing form state, or subscribing to events.
They promote code reusability and make it easier to separate concerns...read more
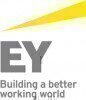
Asked in Ernst & Young

Hoisting is a JavaScript mechanism where variable and function declarations are moved to the top of their containing scope.
Variable declarations are hoisted to the top of their scope but not their assignments.
Function declarations are fully hoisted, meaning they can be called before they are declared.
Hoisting can lead to unexpected behavior if not understood properly.
Asked in Patona Technologies

Q. How can you implement security in a React app?
Implement security in React app by using authentication, authorization, and secure coding practices.
Implement user authentication using libraries like Firebase Authentication or JSON Web Tokens (JWT).
Implement authorization by defining roles and permissions for different user types.
Use secure coding practices to prevent common vulnerabilities like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF).
Implement HTTPS to ensure secure communication between the client...read more
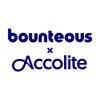
Asked in Bounteous x Accolite

Props are read-only properties passed from a parent component to a child component in React.
Props allow data to be passed down the component tree.
Props are immutable and cannot be modified by the child component.
Props can be used to customize the behavior or appearance of a component.
Props are accessed using the dot notation, like this.props.propName.
Props can be any type of data, including strings, numbers, objects, or functions.
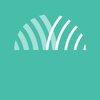
Asked in Worldline

Callbacks in JavaScript are functions passed as arguments to other functions to be executed later.
Callbacks are commonly used in event handling, asynchronous programming, and functional programming.
They allow for functions to be executed after another function has finished its execution.
Example: setTimeout(callbackFunction, 1000) will execute callbackFunction after 1 second.
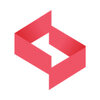
Asked in Simform

Reconciliation in ReactJS is the process of updating the DOM to match the virtual DOM after a component's state or props have changed.
Reconciliation is the algorithm React uses to update the UI efficiently.
It compares the virtual DOM with the actual DOM and only updates the parts that have changed.
Reconciliation is a key feature that helps React achieve high performance.
Example: When a user interacts with a React component, reconciliation ensures that only the necessary parts...read more
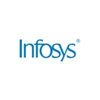
Asked in Infosys

Q. What are stateless and stateful components?
Stateless components are functional components that don't have state, while stateful components are class components that have state.
Stateless components are simpler and easier to test.
Stateful components can hold and modify state, and can trigger re-renders.
Stateless components can receive data through props.
Stateful components can have lifecycle methods.
Example of stateless component: const Button = ({ onClick }) =>
Example of stateful component: class Counter exten...read more
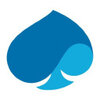
Asked in Capgemini Engineering

Q. What are state and props? What are the differences between them?
State and props are two important concepts in React. State represents the internal data of a component, while props are used to pass data from a parent component to a child component.
State is mutable and can be changed within a component.
Props are read-only and cannot be modified within a component.
State is used to manage component-specific data, while props are used for inter-component communication.
State is initialized and managed within a component, while props are passed ...read more
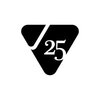
Asked in ValueLabs

Q. What are the use cases of Service Workers?
Service Workers are scripts that run in the background and can intercept network requests, cache or modify responses.
Offline support for web applications
Push notifications
Background synchronization
Reduced network usage and faster page loads
Progressive Web Apps (PWA)
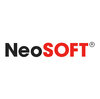
Asked in NeoSOFT

Q. What is the difference between Local Storage and Session Storage?
Local Storage is persistent storage that remains even after the browser is closed, while Session Storage is temporary and is cleared when the browser is closed.
Local Storage has no expiration date, while Session Storage is cleared when the session ends
Local Storage can store larger amounts of data compared to Session Storage
Local Storage is accessible across different browser tabs and windows, while Session Storage is limited to the current tab or window
Local Storage can be a...read more
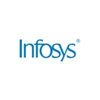
Asked in Infosys

Q. What are the lifecycle methods in React?
Lifecycle methods are methods that get called at various stages of a component's life.
componentDidMount() - called after component is mounted
shouldComponentUpdate() - determines if component should update
componentDidUpdate() - called after component is updated
componentWillUnmount() - called before component is unmounted
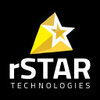
Asked in rSTAR Technologies

A higher order function is a function that takes one or more functions as arguments or returns a function as its result.
Higher order functions can be used to create abstractions and compose functions together.
Examples include map, filter, and reduce functions in JavaScript.
Higher order functions can also be used for event handling and asynchronous programming.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
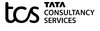
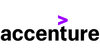
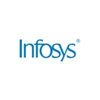
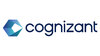
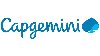
Top Interview Questions for React Developer Related Skills
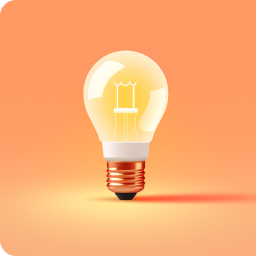
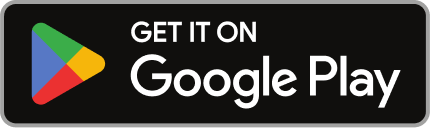
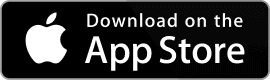
Reviews
Interviews
Salaries
Users
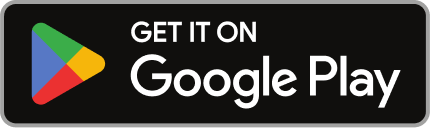
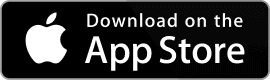