Javascript Developer
40+ Javascript Developer Interview Questions and Answers
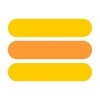
Asked in Cybercom Creation

Q. If you have 3 nested for loops, how many times will the innermost loop run?
Nested for loops multiply their iterations, leading to exponential growth in total runs based on their ranges.
A single for loop runs 'n' times if it iterates from 0 to n-1.
For nested loops, the total runs are the product of their individual iterations.
Example: 3 loops, each running 5 times: 5 * 5 * 5 = 125 total runs.
If the outer loop runs 'a' times and the inner loops run 'b' and 'c' times, total runs = a * b * c.
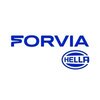
Asked in Forvia Hella

Q. Can you tell me about promise, async in javascript
Promises and async/await are used in JavaScript to handle asynchronous operations and avoid callback hell.
Promises are objects representing the eventual completion or failure of an asynchronous operation.
Async/await is a syntactic sugar built on top of promises, making asynchronous code look synchronous.
Promises can be chained using .then() and .catch() to handle success and error cases.
Async functions return a promise, allowing you to use await to pause execution until the p...read more
Javascript Developer Interview Questions and Answers for Freshers
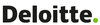
Asked in Deloitte

Q. 1. Is .map and .filter pure functions ? 2. What should not be blocked in Node.js ? 3. Is For.. in.. loop for iterating through object or arrays ?
1. Yes, .map and .filter are pure functions. 2. Network calls should not be blocked in Node.js. 3. For..in.. loop is for iterating through objects.
1. Yes, .map and .filter are pure functions as they do not modify the original array and always return a new array with the desired transformations or filtered elements.
2. Network calls should not be blocked in Node.js as it can lead to performance issues and hinder the event-driven nature of Node.js.
3. For..in.. loop is used for i...read more
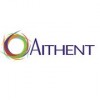
Asked in Aithent Technologies

Q. difference between var, let and const, es6 concepts, state, props in react, arrow function, funciton key word, scope in javascript
var, let, and const are used for variable declaration in JavaScript. ES6 concepts include arrow functions and block-scoped variables.
var is function-scoped, let and const are block-scoped
let and const support block-level scoping, reducing the risk of variable hoisting
const variables cannot be reassigned, but their properties can be modified
ES6 introduces arrow functions for concise syntax and lexical scoping
State and props are key concepts in React for managing component data...read more
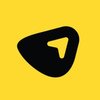
Asked in Uplers

Q. Map reduce and filter in JavaScript
Map, reduce and filter are array methods in JavaScript used for manipulating and transforming data.
Map creates a new array by applying a function to each element of the original array.
Reduce reduces an array to a single value by applying a function to each element.
Filter creates a new array with all elements that pass the test implemented by the provided function.
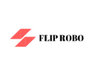
Asked in Flip Robo Technologies

Q. What is the difference between the forEach and map methods?
forEach and map are array methods in JavaScript. forEach executes a provided function once for each array element. Map creates a new array with the results of calling a provided function on every element in the calling array.
forEach does not return anything, it just iterates over the array and executes the provided function for each element.
Map returns a new array with the results of calling a provided function on every element in the calling array.
Map is useful when you want...read more
Javascript Developer Jobs
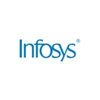
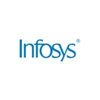
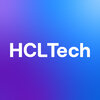
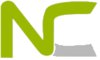
Asked in Northcorp Software

Q. How do you check if a number is even in JavaScript?
Check if a number is even by using the modulo operator.
Use the modulo operator (%) to check if the number divided by 2 has a remainder of 0.
If the remainder is 0, then the number is even.
Example: if(num % 2 === 0) { // number is even }

Asked in Celebal Technologies

Q. How would you design an NLP engine?
Designing a NLP engine involves defining the problem, selecting appropriate algorithms, and training the model.
Define the problem and identify the data sources
Select appropriate algorithms for tasks such as tokenization, part-of-speech tagging, and named entity recognition
Train the model using labeled data and evaluate its performance
Continuously improve the model by incorporating feedback and updating the algorithms
Share interview questions and help millions of jobseekers 🌟
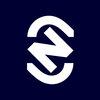
Asked in Nofinite

Q. How do you declare a variable in JavaScript?
Variables in JavaScript are declared using the 'var', 'let', or 'const' keywords.
Use 'var' to declare a variable with function scope.
Use 'let' to declare a variable with block scope.
Use 'const' to declare a constant variable.
Example: var x = 5; let y = 'hello'; const PI = 3.14;
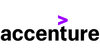
Asked in Accenture

Q. What is the difference between == and ===?
The '==' operator checks for equality after type coercion, while '===' checks for equality without type coercion.
The '==' operator compares two values after converting them to a common type.
The '===' operator compares two values without type conversion, so they must be of the same type to be considered equal.
Example: 1 == '1' will return true because the values are equal after type coercion, but 1 === '1' will return false because they are of different types.
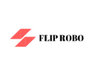
Asked in Flip Robo Technologies

Q. What is the map method?
The map method creates a new array with the results of calling a provided function on every element in the original array.
The map method does not modify the original array.
The provided function takes three arguments: currentValue, index, and array.
The map method returns a new array with the same length as the original array.
Example: const numbers = [1, 2, 3]; const doubledNumbers = numbers.map(num => num * 2); // [2, 4, 6]
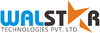
Asked in Walstar Technologies

Q. What is event delegation?
Event delegation is a technique where a single event listener is added to a parent element to handle events on its child elements.
Improves performance by reducing the number of event listeners
Useful for dynamically added elements
Event bubbling is utilized
Example: Click event on a list of items
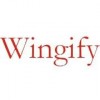
Asked in Wingify

Q. What is callback hell in JavaScript?
Callback hell refers to the situation where multiple nested callbacks make the code difficult to read and maintain.
Occurs when multiple asynchronous operations are nested within each other
Can lead to deeply nested code structure which is hard to follow
Can be avoided by using promises or async/await
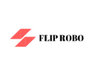
Asked in Flip Robo Technologies

Q. What is the useState hook used for in React?
useState is a hook in React that allows functional components to have state.
useState is used to manage state in functional components
It returns an array with two elements: the current state value and a function to update it
Example: const [count, setCount] = useState(0);
The initial state value is passed as an argument to useState
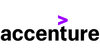
Asked in Accenture

Q. What is event bubbling?
Event bubbling is the propagation of an event from the innermost element to the outermost element in the DOM tree.
When an event occurs on an element, it first runs the handlers on that element, then on its parent, and so on up to the document object.
It allows for event delegation, where a single event handler can be attached to a parent element to handle events for all its children.
Event.stopPropagation() can be used to stop the event from bubbling up the DOM tree.
Asked in Trrigensol Technologies

Q. Do you have experience with ServiceNow?
ServiceNow is a cloud-based platform that provides IT service management, IT operations management, and IT business management solutions.
ServiceNow is commonly used for IT service management, helping organizations streamline their IT processes.
It offers features such as incident management, change management, problem management, and asset management.
ServiceNow also provides IT operations management capabilities, including monitoring, event management, and automation.
The platf...read more
Asked in Coder Infosys

Q. What are the differences between HTML and HTML5?
HTML5 is the latest version of HTML, introducing new features, elements, and APIs for modern web development.
New semantic elements: HTML5 introduced elements like <header>, <footer>, <article>, and <section> for better structure.
Multimedia support: HTML5 provides native support for audio and video with <audio> and <video> tags, eliminating the need for plugins.
Canvas element: HTML5 includes the <canvas> element for dynamic, scriptable rendering of 2D shapes and bitmap images....read more
Asked in Applied Cloud Computing

Q. Promises , differences between var let and const
Promises are used for asynchronous programming in JavaScript. var is function-scoped, let is block-scoped, and const is block-scoped and cannot be reassigned.
Promises are used to handle asynchronous operations in JavaScript.
var is function-scoped, let is block-scoped, and const is block-scoped and cannot be reassigned.
Example: var x = 10; let y = 20; const z = 30;
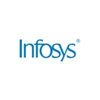
Asked in Infosys

Q. Are you familiar with agile methodologies?
Yes, Agile is a project management methodology that emphasizes iterative development and collaboration.
Agile is a project management methodology that focuses on iterative development, collaboration, and flexibility.
It involves breaking down projects into smaller tasks and completing them in short iterations called sprints.
Agile promotes frequent communication and feedback among team members to adapt to changing requirements.
Common Agile frameworks include Scrum, Kanban, and E...read more
Asked in Bloowatch

Q. Dynamic forms using JavaScript and emberJS
Dynamic forms can be created using JavaScript and emberJS to allow for interactive and customizable user input.
Use JavaScript to dynamically generate form elements based on user input or predefined conditions
Leverage emberJS to handle form data binding and validation
Implement event listeners and callbacks to update form elements and perform actions based on user input
Asked in Acutech Solutions

Q. What is a component in React?
A reusable piece of UI that can be composed with other components to form a complete UI.
Components are the building blocks of React applications.
They can be either functional or class-based.
They can have state, props, and lifecycle methods.
They can be reused and composed to create complex UIs.
Examples include buttons, forms, and navigation menus.
Asked in Applied Cloud Computing

Q. What types of loops are available in JavaScript?
Types of loops in JavaScript include for, while, and do-while loops.
For loop: Executes a block of code a specified number of times.
While loop: Executes a block of code while a specified condition is true.
Do-while loop: Executes a block of code once, and then repeats the loop as long as a specified condition is true.
Asked in Aerosol IO

Q. UI stuff for report / load large stuff data
Use pagination, lazy loading, virtual scrolling, and server-side processing for efficient loading of large data in UI reports.
Implement pagination to display data in smaller chunks
Utilize lazy loading to load data only when needed
Implement virtual scrolling to render only the visible portion of data
Use server-side processing to offload heavy data processing to the server
Asked in Firmsap Consulting

Q. What are callback functions?
Callback functions are functions passed as arguments to other functions to be executed later.
Callback functions are commonly used in asynchronous programming.
They are executed after a certain task is completed.
Example: setTimeout function in JavaScript takes a callback function as an argument.

Asked in Celebal Technologies

Q. What are JavaScript libraries?
JS libraries are pre-written code that can be used to perform specific tasks in JavaScript.
Libraries can save time and effort by providing pre-written code for common tasks
Popular JS libraries include jQuery, React, and Angular
Libraries can be installed using package managers like npm or yarn
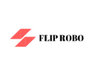
Asked in Flip Robo Technologies

Q. What is the forEach method?
The forEach method executes a provided function once for each array element.
Used to iterate over elements in an array.
Syntax: array.forEach(callback(currentValue, index, array))
Does not return a new array; returns undefined.
Example: [1, 2, 3].forEach(num => console.log(num)); // Logs 1, 2, 3
Cannot be stopped or broken; continues until all elements are processed.
Asked in Trrigensol Technologies

Q. Do you know JavaScript?
Yes, I am proficient in JavaScript and have experience in developing web applications using it.
Proficient in JavaScript programming language
Experience in developing web applications using JavaScript
Familiar with popular JavaScript libraries and frameworks like React, Angular, and Node.js
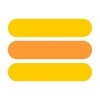
Asked in Cybercom Creation

Q. What is multiple inheritance and what type is it?
Multiple inheritance is when a class can inherit attributes and methods from more than one parent class.
Allows a class to inherit from multiple parent classes
Can lead to the diamond problem where ambiguity arises if two parent classes have a method with the same name
Languages like JavaScript do not support multiple inheritance but can achieve similar functionality using mixins
Asked in Bloowatch

Q. How is an Ember app deployed?
Ember apps can be deployed using various methods such as hosting on a server, using a cloud platform, or deploying to a content delivery network (CDN).
Ember apps can be deployed by hosting them on a server, such as Apache or Nginx.
They can also be deployed using cloud platforms like Heroku or AWS.
Another option is to deploy the app to a content delivery network (CDN) for faster and more efficient distribution.
Deployment can be automated using tools like Ember CLI or continuou...read more
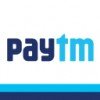
Asked in Paytm

Q. Write a polyfill for Promise.all.
A polyfill is a code that adds a feature which the browser may not support. Promise.all() polyfill can be used for older browsers.
Create a function that takes an array of promises as input
Return a new promise that resolves when all promises in the input array have resolved
If any promise in the input array rejects, reject the new promise with the reason of the first promise that rejects
Use setTimeout and recursion to check if all promises have resolved
If the browser supports P...read more
Interview Experiences of Popular Companies
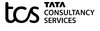
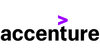
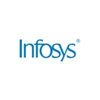
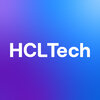
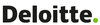
Top Interview Questions for Javascript Developer Related Skills
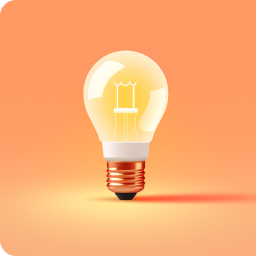
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
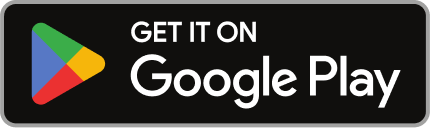
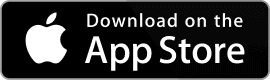
Reviews
Interviews
Salaries
Users
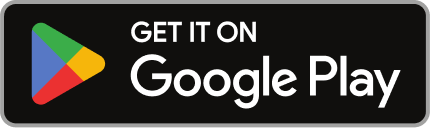
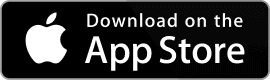