Java Technical Lead
20+ Java Technical Lead Interview Questions and Answers
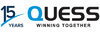
Asked in Quess

Q. Explain the internals of JVM. Where are classes, variables, and objects stored in JVM?
Classes, variables, and objects are stored in different areas of JVM's memory.
Classes are stored in the method area of JVM's memory.
Variables are stored in the stack or heap depending on their scope and lifetime.
Objects are stored in the heap area of JVM's memory.
Static variables are stored in the method area.
Local variables are stored in the stack.
Instance variables are stored in the heap.
Primitive data types are stored in the stack.
References to objects are stored in the st...read more
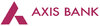
Asked in Axis Bank

Q. Exceptions code snippets to guess output. How to convert checked exception to unchecked exception
To convert checked exception to unchecked exception, use RuntimeException or create a custom unchecked exception.
Use RuntimeException to wrap checked exceptions and throw them as unchecked exceptions
Create a custom unchecked exception by extending RuntimeException class
Use try-catch block to catch checked exceptions and throw them as unchecked exceptions
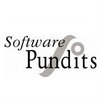
Asked in Software Pundits

Q. What is streams and how to get distinct objects Whats are conventions we follow for rest api Spring mvc error handling and spring boot configuration annotations
Streams in Java and conventions for REST API. Spring MVC error handling and Spring Boot configuration annotations.
Streams are a sequence of elements that can be processed in parallel or sequentially.
To get distinct objects, use the distinct() method.
Conventions for REST API include using HTTP methods, status codes, and resource naming.
Spring MVC error handling can be done using @ExceptionHandler annotation.
Spring Boot configuration annotations include @Configuration, @EnableA...read more
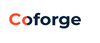
Asked in Coforge

Q. What is the difference between a stream and a parallel stream?
Stream is sequential while parallel stream is concurrent
Stream is a sequence of elements that can be processed sequentially
Parallel stream is a sequence of elements that can be processed concurrently
Parallel stream can improve performance for large datasets
Parallel stream uses multiple threads to process elements in parallel
Stream is suitable for small datasets or when order of processing is important
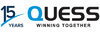
Asked in Quess

Q. Design patterns used in Microservice architecture. API Gateway
Design patterns like API Gateway, Circuit Breaker, Service Registry, and Service Discovery are commonly used in Microservice architecture.
API Gateway pattern is used to provide a single entry point for all clients to access the microservices.
Circuit Breaker pattern is used to prevent cascading failures in microservices.
Service Registry pattern is used to keep track of all the available services in the microservice architecture.
Service Discovery pattern is used to locate the a...read more
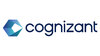
Asked in Cognizant

Q. Why do we need two-way SSL?
2 way SSL is needed for mutual authentication between client and server.
2 way SSL ensures that both client and server are authenticated
It provides an extra layer of security by verifying the identity of both parties
It is commonly used in financial transactions, healthcare, and government applications
Java Technical Lead Jobs
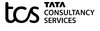
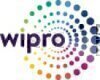
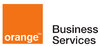
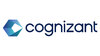
Asked in Cognizant

Q. What is Controller Advice?
Controller Advice is a mechanism in Spring MVC to handle exceptions globally.
It is used to handle exceptions across multiple controllers.
It can be used to add common data to the model.
It can be used to customize the response status code and message.
It can be used to redirect to a custom error page.
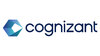
Asked in Cognizant

Q. How do you use grouping in streams?
Grouping by in streams in Java
Use the 'groupingBy' method from the 'Collectors' class
Pass a lambda expression to specify the grouping criteria
The result is a Map with the grouping criteria as keys and the grouped elements as values
Share interview questions and help millions of jobseekers 🌟
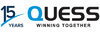
Asked in Quess

Q. What is a marker interface?
A marker interface is an interface with no methods used to mark a class as having a certain property or behavior.
Marker interfaces are used to provide metadata about a class.
They are often used in frameworks and libraries to indicate that a class should be treated in a certain way.
Examples include Serializable, Cloneable, and Remote interfaces in Java.
Marker interfaces can also be used to enforce design patterns, such as the Decorator pattern.
Marker interfaces are different f...read more
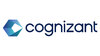
Asked in Cognizant

Q. What is the Decorator design pattern?
Decorator pattern adds behavior to an object dynamically without affecting its existing behavior.
It is a structural pattern
It involves a component interface, concrete component, decorator interface, and concrete decorator
Decorators wrap around components to add new behavior
It allows for flexible and dynamic behavior modification
Example: Java I/O streams use decorator pattern
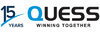
Asked in Quess

Q. Usage of Kafka and Kafka Streams
Kafka is a distributed streaming platform used for building real-time data pipelines and streaming applications.
Kafka is used for building real-time data pipelines and streaming applications
Kafka Streams is a client library for building applications and microservices that process streams of data
Kafka provides fault-tolerant storage and processing of streams of records
Kafka Streams allows for stateful and stateless processing of data
Kafka can be used for various use cases such...read more
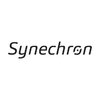
Asked in Synechron

Q. Find the occurrence of each element of an array using Streams API.
Using Streams API to find occurrence of each element in an array of strings
Use Arrays.stream() to convert the array to a stream
Use Collectors.groupingBy() to group elements by their occurrences
Use Collectors.counting() to count the occurrences of each element
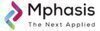
Asked in Mphasis

Q. What are the idempotent methods in a REST API call?
Idempotent methods in REST API calls are operations that can be repeated multiple times without changing the result beyond the initial application.
GET method is idempotent as it retrieves data and does not change the state of the server
PUT and DELETE methods are also idempotent as they perform the same operation regardless of how many times they are called
POST method is not idempotent as it creates a new resource each time it is called
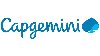
Asked in Capgemini

Q. What are the new features in Java 8?
Java 8 introduced lambda expressions, streams, default methods, and more.
Lambda expressions for functional programming
Streams for efficient processing of large data sets
Default methods to add new functionality to existing interfaces
Date and Time API for improved handling of date and time
Optional class to avoid null pointer exceptions
Nashorn JavaScript engine for improved performance
Parallel array sorting for faster sorting of large arrays
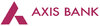
Asked in Axis Bank

Q. Explain the internal workings of CopyOnWriteArrayList.
CopyOnWriteArrayList is a thread-safe variant of ArrayList that creates a new copy on modification.
1. Thread-Safety: It allows concurrent read operations without locking, making it suitable for multi-threaded environments.
2. Copy on Modification: When a write operation (like add or set) occurs, it creates a new copy of the underlying array.
3. Performance: Read operations are fast, but write operations can be costly due to array copying.
4. Use Case: Ideal for scenarios with mo...read more
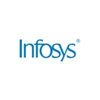
Asked in Infosys

Q. How can we improve microservice security?
Improving microservice security involves implementing authentication, authorization, encryption, and monitoring.
Implement strong authentication mechanisms such as OAuth or JWT
Use role-based access control for authorization
Encrypt sensitive data in transit and at rest
Implement monitoring and logging to detect and respond to security incidents
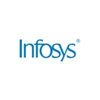
Asked in Infosys

Q. How would you sort strings based on city?
Sort strings based on city names.
Use Arrays.sort() method with a custom Comparator to sort strings based on city names.
Create a Comparator that compares the city names of two strings.
Example: String[] cities = {"New York", "London", "Paris"};
Example: Arrays.sort(cities, (a, b) -> a.compareTo(b));
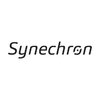
Asked in Synechron

Q. Explain the producer-consumer problem in Kafka.
Producer-consumer pattern in Kafka involves producers sending messages to topics and consumers reading from those topics asynchronously.
Producers publish messages to Kafka topics, which act as message queues.
Consumers subscribe to topics and process messages independently.
Kafka ensures message durability and fault tolerance through replication.
Example: A web application (producer) sends user activity logs to a Kafka topic, while a data processing service (consumer) reads and ...read more
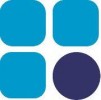
Asked in Zeus Learning

Q. How do you find the second largest element in an array?
Find 2nd max element in an array of strings.
Sort the array in descending order
Return the element at index 1
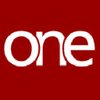
Asked in One Network Enterprises

Q. What is the difference between PUT and POST methods?
PUT is used to update or replace an existing resource, while POST is used to create a new resource.
PUT is idempotent, meaning multiple identical requests will have the same effect as a single request.
POST is not idempotent, meaning multiple identical requests may have different effects.
PUT is used when the client knows the URI of the resource it wants to update.
POST is used when the client does not know the URI of the resource it wants to create.
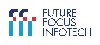
Asked in Future Focus Infotech

Q. Microservices with Kubernate deployment
Microservices with Kubernate deployment involves breaking down an application into smaller, independent services and deploying them using Kubernetes.
Microservices architecture involves breaking down a monolithic application into smaller, independent services that can be developed, deployed, and scaled independently.
Kubernetes is a container orchestration platform that automates the deployment, scaling, and management of containerized applications.
Deploying microservices with ...read more
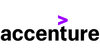
Asked in Accenture

Q. What is the use of static in the main function?
Using static in main function allows it to be called without creating an instance of the class.
Static in main function allows it to be called directly without creating an object of the class.
Static methods can be called using the class name itself, without creating an object.
Example: public static void main(String[] args) { }
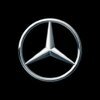

Q. Microservices concept
Microservices are a software development technique where applications are composed of small, independent services that communicate over well-defined APIs.
Microservices break down a large application into smaller, loosely coupled services
Each service is responsible for a specific function and can be developed, deployed, and scaled independently
Communication between services is typically done through APIs, often using lightweight protocols like HTTP or messaging queues
Microserv...read more
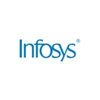
Asked in Infosys

Q. Explain the internal workings of a HashMap.
HashMap is a data structure that stores key-value pairs and uses hashing to efficiently retrieve values.
HashMap internally uses an array of linked lists to store key-value pairs.
When a key-value pair is added, the key is hashed to find the index in the array where it will be stored.
If multiple keys hash to the same index, a linked list is used to handle collisions.
Retrieving a value involves hashing the key to find the index and then traversing the linked list to find the val...read more
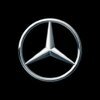

Q. How would you design a database table?
Design a database table for storing user information
Identify the necessary fields such as name, email, phone number, address, etc.
Define appropriate data types for each field (e.g. VARCHAR, INT, DATE)
Set primary key and any necessary constraints (e.g. unique, not null)
Consider normalization to avoid data redundancy and improve data integrity
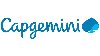
Asked in Capgemini

Q. RestAPI implementation
RestAPI implementation involves creating web services using HTTP methods to interact with data.
Use HTTP methods like GET, POST, PUT, DELETE to perform CRUD operations on resources
Implement endpoints with appropriate request and response formats (JSON, XML)
Secure API with authentication and authorization mechanisms like OAuth
Document API using tools like Swagger for easy consumption by clients
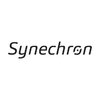
Asked in Synechron

Q. Explain the thread life cycle.
The thread life cycle in Java describes the various states a thread can be in during its execution.
New State: A thread is created but not yet started. Example: Thread t = new Thread();
Runnable State: The thread is ready to run and waiting for CPU time. Example: t.start();
Blocked State: The thread is waiting for a monitor lock to enter a synchronized block or method.
Waiting State: The thread is waiting indefinitely for another thread to perform a particular action. Example: t....read more
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
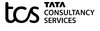
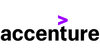
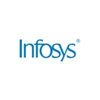
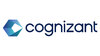
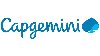
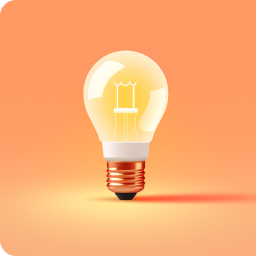
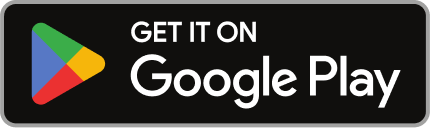
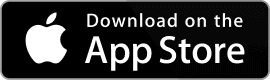
Reviews
Interviews
Salaries
Users
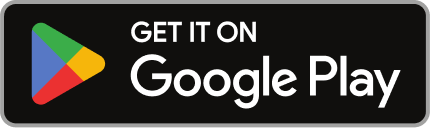
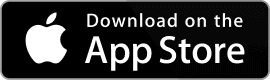