Full Stack Engineer
60+ Full Stack Engineer Interview Questions and Answers
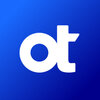
Asked in OpenText Technologies

Q. Find All Subsets
Given an array arr
consisting of 'N' distinct integers, your task is to generate all possible non-empty subsets of the given array.
You can return the subsets in any order.
Input:
The first lin...read more
Generate all possible non-empty subsets of a given array of distinct integers.
Use recursion to generate all subsets by including or excluding each element in the array.
Maintain a current subset and iterate through the array to generate subsets.
Print each subset as it is generated to get all possible non-empty subsets.
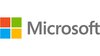
Asked in Microsoft Corporation

Q. Longest Palindromic Subsequence Problem Statement
Given a string A
consisting of lowercase English letters, determine the length of the longest palindromic subsequence within A
.
Explanation:
- A subsequence is d...read more
The task is to find the length of the longest palindromic subsequence in a given string.
Iterate through the string and create a 2D array to store the lengths of palindromic subsequences.
Use dynamic programming to fill the array based on the characters in the string.
Consider the cases where characters match or do not match to update the array values.
Return the length of the longest palindromic subsequence for each test case.
Full Stack Engineer Interview Questions and Answers for Freshers
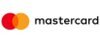
Asked in MasterCard

Q. Word Pattern Problem Statement
Given two strings S
and T
, determine if S
follows the same pattern as T
.
A full match means there is a bijection between a letter of T
and a non-empty word of S
.
Example:
Input:
S...read more
Check if two strings follow the same pattern based on bijection between letters and words.
Iterate through each letter in T and corresponding word in S to create a mapping.
Use a hashmap to store the mapping between letters and words.
Check if the mapping is consistent for all test cases.
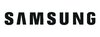
Asked in Samsung

Q. Reverse Linked List Problem Statement
Given a singly linked list of integers, return the head of the reversed linked list.
Example:
Initial linked list: 1 -> 2 -> 3 -> 4 -> NULL
Reversed linked list: 4 -> 3 -> 2...read more
Reverse a singly linked list of integers and return the head of the reversed linked list.
Iterate through the linked list and reverse the pointers to point to the previous node instead of the next node.
Keep track of the current, previous, and next nodes while traversing the linked list.
Update the head of the reversed linked list to be the last node encountered.
Ensure to handle edge cases like an empty linked list or a single node in the list.
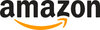
Asked in Amazon

Q. Next Smallest Palindrome Problem Statement
Given a string representation of a number 'S', determine the smallest palindrome that is strictly greater than this number 'N'.
Example:
Input:
3
2
99
3
123
4
4567
Output:
1...read more
The task is to find the smallest palindrome greater than a given number.
Iterate from the middle of the number and mirror the left side to form the palindrome.
Handle cases where the number is already a palindrome or has all 9s.
Consider odd and even length numbers separately.
Convert the string to integer for comparison and manipulation.
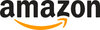
Asked in Amazon

Q. Level Order Traversal Problem Statement
Given a binary tree of integers, return the level order traversal of the binary tree.
Input:
The first line contains an integer 'T', representing the number of test cases...read more
Implement a function to return the level order traversal of a binary tree of integers.
Create a queue to store nodes for level order traversal
Start with the root node and enqueue it
While the queue is not empty, dequeue a node, print its value, and enqueue its children
Repeat until all nodes are traversed
Full Stack Engineer Jobs
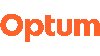
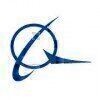
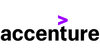
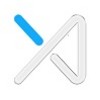
Asked in Arcesium

Q. Count Subarrays with Sum Divisible by K
Given an array ARR
and an integer K
, your task is to count all subarrays whose sum is divisible by the given integer K
.
Input:
The first line of input contains an integer...read more
Count the number of subarrays in an array whose sum is divisible by a given integer K.
Iterate through the array and keep track of the running sum modulo K.
Use a hashmap to store the frequency of remainders.
For each prefix sum, check how many previous prefix sums have the same remainder.
Return the total count of subarrays with sum divisible by K.
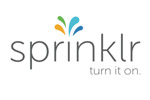
Asked in SPRINKLR

Q. Smaller Elements Count Problem Statement
Given an array of size N
, return a count array such that COUNT[i]
equals the number of elements which are smaller than ARR[i]
on its right side.
Input:
Input Format:
The...read more
Return an array where each element represents the count of smaller elements on its right side in the given array.
Iterate through the array from right to left and maintain a sorted list of elements encountered so far.
For each element, find its index in the sorted list to determine the count of smaller elements on its right side.
Use binary search or segment trees for efficient searching and updating of the sorted list.
Share interview questions and help millions of jobseekers 🌟
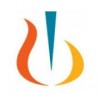
Asked in Novartis

Q. Count the Number of Ones Problem Statement
Given an integer N
, calculate the total number of times the digit '1' appears in each number from 0 to N inclusive.
Input:
T (number of test cases)
Each test case conta...read more
The task is to count the number of occurrences of the digit '1' in each number from 0 to N.
Iterate through each number from 0 to N
Convert each number to a string
Count the number of occurrences of the digit '1' in the string representation of each number
Return the total count of '1's
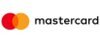
Asked in MasterCard

Q. Next Greater Node in Linked List Problem Statement
In a set of linked ninja villages, the goal is to determine if a stronger ninja exists in the nearest village linked ahead. Given a linked list of 'N' integers...read more
Given a linked list of ninja villages, find the next greater ninja strength in the nearest linked village.
Traverse the linked list and store the elements in an array.
Use a stack to keep track of elements with no next greater element found yet.
Iterate through the array to find the next greater element for each village.
Return the array of next greater elements for each village.

Asked in Hike

Q. Maximum Size Rectangle Sub-matrix with All 1's Problem Statement
You are provided with an N * M
sized binary matrix 'MAT' where 'N' denotes the number of rows and 'M' denotes the number of columns. Your task is...read more
The task is to find the maximum size of a submatrix consisting of all 1's in a given binary-valued matrix.
Iterate through each cell of the matrix
For each cell, calculate the maximum size of a submatrix with that cell as the top-left corner
Keep track of the maximum size encountered so far
Return the maximum size
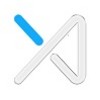
Asked in Arcesium

Q. Minimum Operations to Equalize Array
Given an integer array ARR
of length N
where ARR[i] = (2*i + 1)
, determine the minimum number of operations required to make all the elements of ARR
equal. In a single opera...read more
The minimum number of operations required to make all elements of the given array equal.
The array is generated based on the formula ARR[i] = (2*i + 1).
To equalize the array, increment one element and decrement another in each operation.
The number of operations needed is equal to the difference between the maximum and minimum elements in the array.
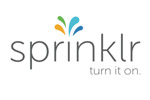
Asked in SPRINKLR

Q. Rank from Stream Problem Statement
Given an array of integers ARR
and an integer K
, determine the rank of the element ARR[K]
.
Explanation:
The rank of any element in ARR
is defined as the number of elements sma...read more
The task is to determine the rank of a specific element in an array based on the number of smaller elements before it.
Iterate through the array up to index K and count the number of elements smaller than ARR[K].
Return the count as the rank of ARR[K].
Handle multiple test cases by repeating the process for each case.
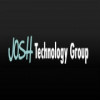
Asked in Josh Technology Group

Q. 0/1 Knapsack Problem Statement
A thief is planning to rob a store and can carry a maximum weight of 'W' in his knapsack. The store contains 'N' items where the ith item has a weight of 'wi' and a value of 'vi'....read more
The 0/1 Knapsack Problem involves maximizing the total value of items a thief can steal within a given weight limit.
Dynamic programming can be used to solve this problem efficiently.
Create a 2D array to store the maximum value that can be stolen at each weight capacity.
Iterate through the items and update the array based on whether the item is included or not.
The final answer will be the value at the last cell of the array.
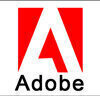
Asked in Adobe

Q. Count Distinct Substrings
You are provided with a string S
. Your task is to determine and return the number of distinct substrings, including the empty substring, of this given string. Implement the solution us...read more
Implement a function to count the number of distinct substrings in a given string using a trie data structure.
Create a trie data structure to store the substrings of the input string.
Traverse the trie to count the number of distinct substrings.
Handle empty string as a distinct substring.
Return the count of distinct substrings for each test case.
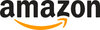
Asked in Amazon

Q. Edit Distance Problem Statement
Given two strings S
and T
with lengths N
and M
respectively, your task is to find the "Edit Distance" between these strings.
The Edit Distance is defined as the minimum number of...read more
The task is to find the minimum number of operations required to convert one string into another using delete, replace, and insert operations.
Use dynamic programming to solve the problem efficiently.
Create a 2D array to store the edit distances between substrings of the two input strings.
Fill up the array based on the minimum of three possible operations: insert, delete, or replace.
Return the value at the bottom right corner of the array as the final edit distance.
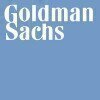
Asked in Goldman Sachs

Q. Merge Intervals Problem Statement
You are provided with 'N' intervals, each containing two integers denoting the start time and end time of the interval.
Your task is to merge all overlapping intervals and retu...read more
Merge overlapping intervals and return sorted list by start time.
Sort the intervals based on start time.
Iterate through intervals and merge overlapping ones.
Return the merged intervals sorted by start time.
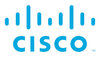
Asked in Cisco

Q. Longest Subarray Zero Sum Problem Statement
Given an array of integers arr
, determine the length of the longest contiguous subarray that sums to zero.
Input:
N (an integer, the length of the array)
arr (list of ...read more
Find the length of the longest contiguous subarray that sums to zero in an array of integers.
Iterate through the array and keep track of the running sum and its corresponding index in a hashmap.
If the running sum is seen again, calculate the length of the subarray by subtracting the current index from the index stored in the hashmap.
Update the maximum length of the subarray as you iterate through the array.
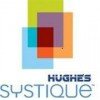
Asked in Hughes Systique Corporation

Examples of OOP concepts using code and principles
Encapsulation: Using private variables and getter/setter methods
Inheritance: Creating subclasses that inherit properties and methods from a superclass
Polymorphism: Implementing methods with the same name but different functionality in subclasses
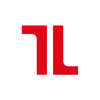
Asked in Tilicho Labs

Q. What are the definitions of Protected Routes, Private Routes, and Public Routes in web development, and could you provide code examples for each?
Protected, Private, and Public Routes manage access to web application pages based on user authentication and authorization.
Public Routes: Accessible to everyone, no authentication required. Example: Home page, About page.
Private Routes: Restricted to authenticated users. Example: User dashboard, Profile settings.
Protected Routes: Similar to private routes but may allow access based on user roles or permissions. Example: Admin panel for admins only.
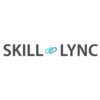
Asked in Skill Lync

Q. Tell me about your knowledge of Java and SQL.
I have extensive knowledge in Java and SQL, focusing on database interactions and backend development.
Proficient in JDBC for connecting Java applications to SQL databases.
Experience in writing complex SQL queries for data retrieval and manipulation.
Familiar with ORM frameworks like Hibernate for object-relational mapping.
Implemented stored procedures and triggers for efficient database operations.
Knowledge of database design principles and normalization techniques.
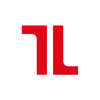
Asked in Tilicho Labs

Q. What is middleware, and how is it utilized for role-based access?
Middleware is software that connects different applications or services, often used for managing access control in web applications.
Middleware acts as a bridge between the client and server, processing requests and responses.
It can enforce role-based access control (RBAC) by checking user roles before granting access to resources.
For example, in an Express.js application, middleware can be used to verify if a user has the 'admin' role before allowing access to admin routes.
Mi...read more
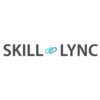
Asked in Skill Lync

Q. Write programs for all questions based on Java and SQL.
This program covers essential Java and SQL concepts for Fullstack Engineer interviews.
Understand Java fundamentals: classes, objects, inheritance, and polymorphism.
Familiarize with SQL queries: SELECT, INSERT, UPDATE, DELETE.
Practice Java collections: ArrayList, HashMap, and their use cases.
Learn about exception handling in Java: try-catch blocks.
Explore JDBC for database connectivity in Java applications.
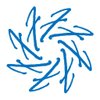
Asked in Flair Labs

Q. What is API? Difference between API and RestApi What is cloud? Where have you used cloud till now? Database related questions. A few html js related questions.
API stands for Application Programming Interface. It defines the methods and data formats that applications can use to communicate with each other.
API is a set of rules and protocols that allows different software applications to communicate with each other.
REST API is a type of API that follows the principles of REST (Representational State Transfer) architecture.
Cloud computing refers to the delivery of computing services over the internet, including storage, databases, net...read more
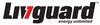
Asked in Livguard

Q. How is React optimized compared to other JavaScript frameworks?
React is optimised due to virtual DOM, one-way data binding, and component-based architecture.
Virtual DOM allows React to update only the necessary components, improving performance.
One-way data binding ensures that data flows in a single direction, reducing the risk of bugs.
Component-based architecture promotes reusability and modularity, making code easier to maintain.
React's use of JSX allows developers to write HTML-like code within JavaScript, improving readability and p...read more
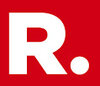
Asked in Republic TV

Q. What are the concepts of async and await in JavaScript?
Async and await simplify asynchronous programming in JavaScript, making it easier to read and write code that handles promises.
Async functions return a promise, allowing the use of await within them.
Await pauses the execution of the async function until the promise is resolved.
Using async/await makes code look synchronous, improving readability.
Example: async function fetchData() { const data = await fetch(url); return data; }
Error handling can be done using try/catch blocks ...read more
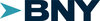
Asked in BNY

Q. Given an array, find the maximum length of a contiguous subarray with all unique elements.
Find max continous sub array with unique elements in an array
Use sliding window approach
Keep track of unique elements in the window
Update max length when a duplicate element is found
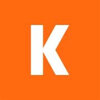
Asked in KAYAK

Q. How do you optimize a query?
Optimizing a query involves using indexes, limiting the result set, and avoiding unnecessary joins.
Use indexes on columns frequently used in the query's WHERE clause
Limit the result set by using pagination or filtering criteria
Avoid unnecessary joins by denormalizing data or using subqueries
Asked in Jitera

Q. Write code for a retry mechanism.
Implementing a retry mechanism in code to handle failures and retries automatically.
Create a function that takes a function to execute and a maximum number of retries as parameters.
Use a loop to execute the function and catch any exceptions that occur.
If an exception occurs, decrement the number of retries left and retry the function.
Example: function retry(func, maxRetries) { for(let i=0; i
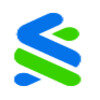
Asked in Standard Chartered

Q. The most difficult challenges faced, situation-based approach, dealing with ambiguous requirements
Dealing with ambiguous requirements and difficult challenges in a situation-based approach.
Identifying and clarifying ambiguous requirements through effective communication
Breaking down complex problems into smaller, manageable tasks
Adapting to changing priorities and tight deadlines
Collaborating with team members to brainstorm solutions
Using critical thinking and problem-solving skills to find innovative solutions
Managing expectations and balancing trade-offs between differe...read more
Interview Experiences of Popular Companies
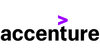
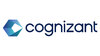

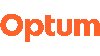
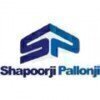
Top Interview Questions for Full Stack Engineer Related Skills
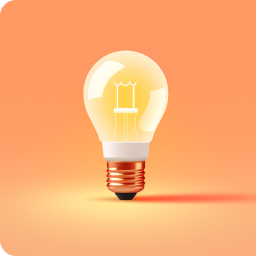
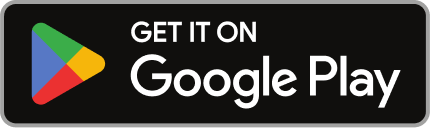
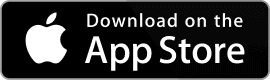
Reviews
Interviews
Salaries
Users
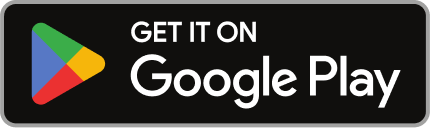
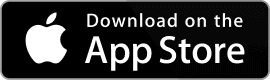