Front end Developer
1000+ Front end Developer Interview Questions and Answers
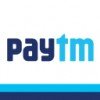
Asked in Paytm

Data spooling is a process where data is temporarily stored in a buffer before being sent to an output device.
Data spooling helps in managing the flow of data between different devices by storing it temporarily.
It allows for efficient processing of data by decoupling the input/output operations.
Examples of data spooling include print spooling, where print jobs are stored in a queue before being sent to the printer.
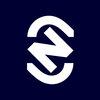
Asked in Nofinite

Q. What is JavaScript?
JavaScript is a versatile, high-level programming language primarily used for web development to create interactive and dynamic content.
JavaScript is an interpreted, high-level language that supports object-oriented, imperative, and functional programming styles.
It is dynamically typed, meaning variables can hold values of any type without explicit declaration (e.g., let x = 5; x = 'Hello';).
JavaScript runs in the browser, allowing developers to create interactive web pages (...read more
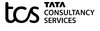
Asked in TCS

Q. Do you know about React middlewares? Why are they used?
React middlewares are functions that intercept and modify requests and responses in a React application.
Middlewares are used to add additional functionality to an application without modifying the core code.
They can be used for authentication, logging, error handling, and more.
Examples of React middlewares include Redux Thunk, Redux Saga, and React Router.
They are typically implemented using higher-order functions.
Middlewares can be chained together to create a pipeline of fu...read more
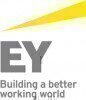
Asked in Ernst & Young

Hoisting in JavaScript is a behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase.
Variable declarations are hoisted to the top of their scope, but not their assignments.
Function declarations are fully hoisted, meaning they can be called before they are declared.
Hoisting can lead to unexpected behavior if not understood properly.
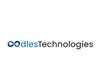
Asked in OodlesTechnologies

Types of data binding in Angular include interpolation, property binding, event binding, two-way binding.
Interpolation: {{ data }}
Property binding: [property]="data"
Event binding: (event)="function()"
Two-way binding: [(ngModel)]="data"
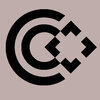

Q. You are given data in key-value pairs (an object). You need to insert this data into a form by default. The form contains label and input tags. When the application runs, the data should render in the input fie...
read moreImplement a dynamic form in React that renders input fields based on key-value pairs.
Use React's state to manage form data dynamically.
Map over the key-value pairs to create input fields and labels.
Example: const formData = { name: 'John', age: 30 };
Use controlled components to handle input changes.
Example: <input value={formData.name} onChange={e => setFormData({...formData, name: e.target.value})} />
Ensure the form updates automatically if the data changes.
Front end Developer Jobs
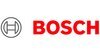
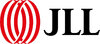
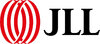
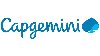
Asked in Capgemini

Q. What is life cycle method What hooks you have used What is map filter and reduce How to make JavaScript asynchronous What is the difference between redux thunk and redux saga What is the benefit of arrow functi...
read moreLife cycle methods are methods that are automatically called at different stages of a component's life cycle in React.
ComponentDidMount is called after the component has been rendered to the DOM.
ComponentDidUpdate is called after the component's state or props have been updated.
ComponentWillUnmount is called before the component is removed from the DOM.
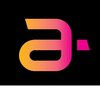
Asked in Amdocs

Promises are objects representing the eventual completion or failure of an asynchronous operation.
Promises have three states: pending, fulfilled, and rejected.
Pending: initial state, neither fulfilled nor rejected.
Fulfilled: operation completed successfully.
Rejected: operation failed.
Promises can be chained using .then() to handle success and failure.
Example: const promise = new Promise((resolve, reject) => { ... });
Share interview questions and help millions of jobseekers 🌟
Asked in Navalt

Q. What are performance optimization techniques used in React?
Performance optimization techniques in React improve rendering speed and user experience.
Code splitting to load only necessary components
Memoization to prevent unnecessary re-renders
Virtualization for long lists to improve rendering performance
Using shouldComponentUpdate or React.memo for functional components
Minimizing unnecessary re-renders by using PureComponent or React.PureComponent
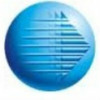
Asked in Protechsoft Technologies

Q. What is the 'this' operator?
The 'this' operator refers to the object that is currently executing the code.
The value of 'this' depends on how a function is called.
In a method, 'this' refers to the object that owns the method.
In a regular function, 'this' refers to the global object (window in a browser).
In an event handler, 'this' refers to the element that received the event.
The value of 'this' can be explicitly set using call(), apply(), or bind() methods.
Asked in Pivotrics

Q. What is the difference between display: none and visibility: hidden?
display none removes element from layout, visibility hidden hides element but still takes up space
display none removes the element from the layout completely
visibility hidden hides the element but still takes up space
display none will not render the element, while visibility hidden will render the element but make it invisible
Asked in AnglerFox WebSolutions

Q. What is the difference between session storage and local storage?
Session storage is temporary and data is cleared when the browser is closed, while local storage persists even after the browser is closed.
Session storage is temporary and data is cleared when the browser is closed
Local storage persists even after the browser is closed
Session storage is limited to the current tab or window, while local storage is shared across tabs and windows of the same origin
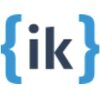
Asked in Interview Kickstart

Q. What is Promises ? Write a Polyfill of Promise.all()
Promises are objects representing the eventual completion or failure of an asynchronous operation.
Promises are used to handle asynchronous operations in JavaScript.
Promise.all() takes an array of promises and returns a single promise that resolves when all of the input promises have resolved.
A polyfill for Promise.all() can be implemented using a combination of Promise and Array.prototype.reduce().
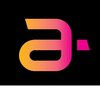
Asked in Amdocs

The CSS Box Model is a fundamental concept in CSS that defines the layout and spacing of elements on a webpage.
The Box Model consists of content, padding, border, and margin.
Content: The actual content of the box, such as text or images.
Padding: Space between the content and the border.
Border: The border surrounding the padding and content.
Margin: Space outside the border, separating the element from other elements.
Example: div { width: 200px; padding: 20px; border: 1px solid...read more
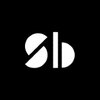
Asked in Sketch Brahma Technologies

Q. What is CSS Flexbox and its attributes?
CSS Flexbox is a layout module that helps in creating flexible and responsive layouts.
Flexbox is used to align and distribute space among items in a container.
It has properties like display, flex-direction, justify-content, align-items, and flex-wrap.
Flexbox is widely used in modern web development for creating responsive designs.
Example: display: flex; flex-direction: row; justify-content: center; align-items: center;
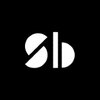
Asked in Sketch Brahma Technologies

Q. What is SCSS/Sass and what is its use?
Scss/Sass is a CSS preprocessor that adds features like variables, nesting, and mixins to make writing CSS easier and more efficient.
Scss/Sass stands for Syntactically Awesome Style Sheets
It is a CSS preprocessor that compiles into regular CSS
It adds features like variables, nesting, and mixins to make writing CSS easier and more efficient
It also allows for more advanced features like functions and loops
Example: $primary-color: #007bff; .button { background-color: $primary-co...read more
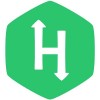
Asked in HackerRank

Q. When should you use functional components versus class components, other than for state management?
Functional components are simpler and easier to test, while class components offer more features and lifecycle methods.
Use functional components for simple UI components
Use class components for more complex UI components
Functional components are easier to read and maintain
Class components offer more lifecycle methods and state management options
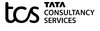
Asked in TCS

Q. What is React.js?
React.js is a JavaScript library used for building user interfaces by creating reusable UI components.
React.js allows developers to create interactive user interfaces efficiently.
It uses a virtual DOM for optimal performance by updating only the necessary components.
React components can be reused across different parts of an application.
It follows a unidirectional data flow, making it easier to manage state and data.
React.js is maintained by Facebook and a community of develo...read more
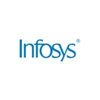
Asked in Infosys

Q. What is a controlled component versus an uncontrolled component?
Controlled components are React components whose value is controlled by React, while uncontrolled components are not.
Controlled components are typically used for forms and inputs
Uncontrolled components are typically used for simple inputs like text fields
Controlled components use the 'value' prop to set the value of the component
Uncontrolled components use the 'defaultValue' prop to set the initial value of the component
Controlled components allow for more control and validat...read more
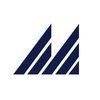
Asked in Manhattan Associates

Dependency injection in Angular is a design pattern where components are given their dependencies rather than creating them themselves.
In Angular, dependency injection is achieved by declaring the dependencies in the constructor of a component or service.
It helps in making components more modular, reusable, and easier to test.
For example, if a component needs a service to fetch data from an API, instead of creating an instance of the service within the component, the service ...read more
Asked in Appitsimple Infotek

Q. What is the difference between position absolute, relative, and other position values in CSS?
Position absolute is positioned relative to its closest positioned ancestor, while position relative is positioned relative to its normal position in the document flow.
Position absolute elements are taken out of the normal document flow.
Position relative elements are still in the normal document flow but can be moved from their original position using top, bottom, left, and right properties.
Position fixed elements are positioned relative to the viewport and do not move when t...read more
Asked in Zedex Info Pvt Ltd

Q. How can you center three divs vertically and horizontally using CSS?
To center 3 divs vertically and horizontally, use flexbox and align-items/justify-content properties.
Wrap the 3 divs in a parent container
Set the parent container's display property to flex
Set the parent container's align-items and justify-content properties to center
Asked in Navalt

Q. What is the difference between Server-Side Rendering and Client-Side Rendering?
Server-Side Rendering is rendering the web page on the server and sending the fully rendered page to the client, while Client-Side Rendering is rendering the web page on the client's browser using JavaScript.
Server-Side Rendering generates the HTML on the server and sends it to the client, resulting in faster initial page load.
Client-Side Rendering loads a basic HTML page first and then uses JavaScript to render the content on the client's browser, which can result in slower ...read more
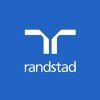
Asked in Randstad

Q. What challenges have you faced in modernizing legacy systems, and how do you handle the integration of new technologies within an established global infrastructure?
Modernizing legacy systems and integrating new technologies in a global infrastructure.
Identify key pain points in the legacy system that need modernization
Develop a clear roadmap for integration of new technologies while minimizing disruption
Collaborate with stakeholders to ensure alignment with business goals
Implement gradual changes and conduct thorough testing to mitigate risks
Provide training and support for users to adapt to the new technologies
Asked in Cumin 360 Technologies

Q. How would you design the database for a chat application?
A chat app db should have tables for users, conversations, messages, and attachments.
Create a table for users with their unique ID, username, and password.
Create a table for conversations with their unique ID, name, and participants.
Create a table for messages with their unique ID, conversation ID, sender ID, and message content.
Create a table for attachments with their unique ID, message ID, and attachment URL.
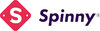
Asked in Spinny

Q. Implement event bubbling on three nested divs: grandparent, parent, and child.
Event bubbling can be implemented by attaching event listeners to the child, parent, and grandparent divs.
Add event listeners to the child, parent, and grandparent divs
Use the event.stopPropagation() method to stop the event from bubbling up to the parent and grandparent divs
Handle the event in each div's event listener function
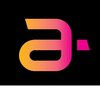
Asked in Amdocs

Media elements in HTML are used to embed audio and video content on a webpage.
Media elements include <audio> and <video> tags in HTML.
They allow for the playback of audio and video files directly on a webpage.
Attributes like src, controls, autoplay, and loop can be used to customize the behavior of media elements.
Example: <video src='video.mp4' controls></video>
Asked in TailNode

Q. How can you count the number of words in a given array of strings using higher-order functions?
Count the number of words in given strings of array using higher order function.
Use the map function to transform each string into an array of words
Use the reduce function to count the total number of words in all strings
Handle edge cases such as empty strings or strings with leading/trailing spaces
Asked in Techpile Technology

Q. Is it possible to apply multiple colors to text using only one HTML tag and CSS, without including additional tags?
Yes, you can apply multiple colors to text using CSS with a single tag by utilizing techniques like gradients or text shadows.
Use CSS gradients: `background: linear-gradient(to right, red, blue); -webkit-background-clip: text; color: transparent;`
Apply text-shadow for a multi-colored effect: `text-shadow: 1px 1px red, 2px 2px blue;`
Utilize the `mask-image` property for advanced color effects: `mask-image: linear-gradient(to right, red, blue);`
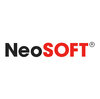
Asked in NeoSOFT

Lifecycle hooks in Angular are methods that allow you to tap into specific points in a component's lifecycle.
Lifecycle hooks include ngOnInit, ngOnChanges, ngDoCheck, ngOnDestroy, etc.
ngOnInit is used for initialization logic, ngOnChanges for reacting to input changes, ngDoCheck for custom change detection, ngOnDestroy for cleanup tasks, etc.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
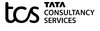
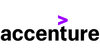
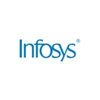
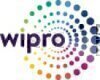
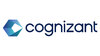
Top Interview Questions for Front end Developer Related Skills
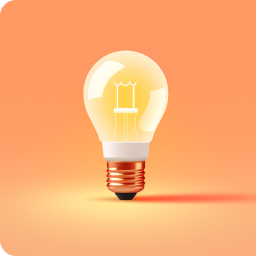
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
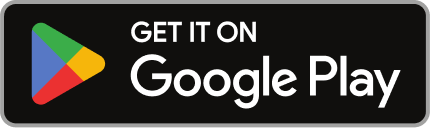
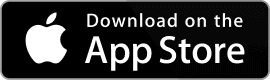
Reviews
Interviews
Salaries
Users
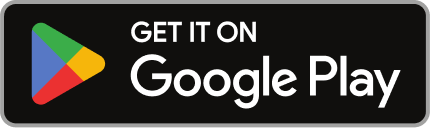
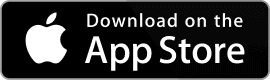