Front end Developer
1000+ Front end Developer Interview Questions and Answers
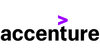
Asked in Accenture

Q. How can you center align a div in CSS using only one line of code?
Use flexbox to center align a div in just one line in CSS.
Set the parent container's display property to flex.
Use the justify-content property with the value 'center' to horizontally center the div.
Use the align-items property with the value 'center' to vertically center the div.
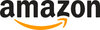
Asked in Amazon

Q. String Palindrome Verification
Given a string, your task is to determine if it is a palindrome considering only alphanumeric characters.
Input:
The input is a single string without any leading or trailing space...read more
Check if a given string is a palindrome considering only alphanumeric characters.
Remove non-alphanumeric characters from the input string.
Compare the string with its reverse to check for palindrome.
Handle edge cases like empty string or single character input.
Use two pointers approach for efficient comparison.
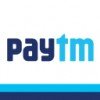
Asked in Paytm

Q. Priority CPU Scheduling Problem
Given 'N' processes with their “burst times”, where the “arrival time” for all processes is ‘0’, and the ‘priority’ of each process, your task is to compute the “waiting time” an...read more
Implement Priority CPU Scheduling algorithm to compute waiting time and turn-around time for processes.
Implement a function that takes in burst times, priorities, and number of processes as input
Sort the processes based on priority, with lower process ID as tiebreaker
Calculate waiting time and turn-around time for each process based on the scheduling algorithm
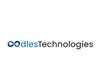
Asked in OodlesTechnologies

Q. Is JavaScript single-threaded or multi-threaded?
Javascript is single-threaded.
Javascript runs on a single thread called the event loop.
This means that it can only execute one task at a time.
However, it can delegate tasks to other threads using web workers.
This allows for parallel processing without blocking the main thread.
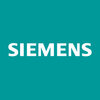
Asked in Siemens

Components are building blocks of Angular applications, while directives are used to add behavior to DOM elements.
Components have a template, styles, and behavior encapsulated together, while directives are used to manipulate the behavior of existing DOM elements.
Components are typically used to create reusable UI elements, while directives are used to add custom behavior to existing elements.
Components can have their own view encapsulation, while directives do not have their...read more
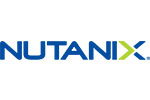
Asked in Nutanix

Q. Implement a calculator class that supports chaining operations like cal.add(2).sub(3).mul(4).delay(2000).add(4).
Implement a calculator class with chaining methods and delay function.
Create a Calculator class with add, sub, mul methods that return the instance of the class.
Implement a delay method that uses setTimeout and returns the instance of the class.
Use a queue to store the operations and execute them in order after the delay.
Return the result of the operations when the equals method is called.
Front end Developer Jobs
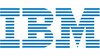
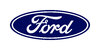
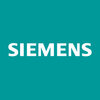
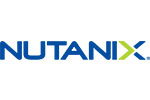
Asked in Nutanix

Q. Given a nested structure of checkboxes (parents > children > grandchildren, etc.), write JavaScript code such that when any checkbox is clicked, all its descendants are updated to the same value as that parent...
read moreJavaScript code to synchronize checkbox states in a nested structure.
Use event delegation to handle checkbox clicks efficiently.
Recursively traverse the DOM to find child checkboxes.
Set the checked property of child checkboxes based on the parent checkbox state.
Example: If a parent checkbox is checked, all its children should also be checked.
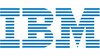
Asked in IBM

Q. Describe your approach to creating a function that returns an object containing methods for accessing, adding, and deleting elements from an array.
Create a function that manages an array with methods to get, add, and delete elements.
Define a function that returns an object with methods.
Use an array to store elements internally.
Implement a 'getElements' method to return the array.
Implement an 'addElement' method to push a new element to the array.
Implement a 'deleteElement' method to remove an element by value.
Share interview questions and help millions of jobseekers 🌟

Asked in Gainsight

You can import all exports of a file as an object in JavaScript using the 'import * as' syntax.
Use the 'import * as' syntax followed by the object name and 'from' keyword to import all exports of a file as an object.
For example: import * as myExports from './myFile.js';
You can then access the exports using dot notation, like myExports.myFunction().
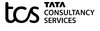
Asked in TCS

Q. How does React Native work internally, specifically regarding memory allocation and rendering JavaScript code on Android and iOS devices?
React Native works by using a bridge to communicate between JavaScript and native code on iOS and Android devices.
React Native uses a JavaScript thread to handle logic and a separate thread for UI rendering.
The bridge allows for communication between the JavaScript thread and native code on the device.
React Native uses a virtual DOM to optimize rendering performance.
Memory allocation is handled by the device's operating system.
React Native also provides tools for debugging an...read more
Asked in Appinlay

JavaScript data types include string, number, boolean, object, function, undefined, and null.
String: 'hello'
Number: 42
Boolean: true or false
Object: { key: 'value' }
Function: function() { }
Undefined: undefined
Null: null
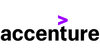
Asked in Accenture

Q. Write a function to remove duplicate elements from an array.
Remove duplicate elements from an Array
Use the Set object to remove duplicate elements
Convert the Set back to an array using the spread operator
If the array contains objects, use a custom comparison function
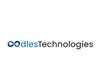
Asked in OodlesTechnologies

Async/Await is a feature in JavaScript that allows for easier handling of asynchronous code.
Async/Await is built on top of Promises and provides a more readable and concise way to work with asynchronous code.
The 'async' keyword is used to define a function as asynchronous, allowing the use of 'await' inside it to pause execution until a Promise is settled.
Using Async/Await can help avoid callback hell and make code more maintainable and easier to understand.
Example: async fun...read more
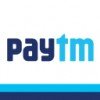
Asked in Paytm

Inheritance is a relationship between a superclass and subclass, while generalization is a relationship between entities with common characteristics.
Inheritance involves a parent-child relationship where the child class inherits attributes and methods from the parent class.
Generalization involves grouping entities with common attributes into a higher-level entity.
Inheritance is a specific form of generalization in object-oriented programming.
Example: In a database, a 'Car' en...read more
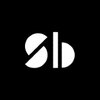
Asked in Sketch Brahma Technologies

Q. What are CSS media queries, and what is the difference between min and max?
CSS media queries are used to apply different styles based on device characteristics. Min and max define the range of values.
Media queries allow for responsive design by adapting to different screen sizes and orientations
Min-width and max-width are commonly used to define the range of screen sizes
Min-device-width and max-device-width are used to target specific device characteristics
Other media query features include min-height, max-height, orientation, and resolution
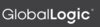
Asked in GlobalLogic

var is function scoped while let is block scoped in JavaScript.
var is function scoped, meaning it is accessible throughout the function it is declared in.
let is block scoped, meaning it is only accessible within the block it is declared in.
Using var can lead to variable hoisting issues, while let avoids this problem.
Example: var x = 10; function test() { var x = 20; console.log(x); } test(); // Output: 20
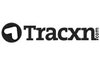
Asked in Tracxn

Q. Why is JavaScript considered a dynamic language?
JavaScript is considered a dynamic language because variables can change types based on their current value.
Variables in JavaScript can hold different types of values at different times
The type of a variable is determined at runtime, not at compile time
Dynamic typing allows for flexibility and ease of use in JavaScript programming
Example: a variable can start as a number and then be reassigned as a string
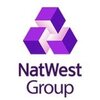
Asked in NatWest Group

Q. Are functional components and class components the same?
Functional components and class components are not the same in React. Functional components are simpler and more lightweight, while class components have additional features like state and lifecycle methods.
Functional components are stateless and are just JavaScript functions that return JSX.
Class components have access to state and lifecycle methods like componentDidMount and componentDidUpdate.
Functional components are easier to read and test, while class components can be ...read more
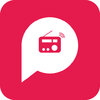
Asked in Pocket FM

Q. How would you optimize API calls on input change using debouncing?
Debounce the API call on input change to optimize performance.
Implement a debounce function to delay the API call until the user has finished typing.
Set a time interval for the debounce function to wait before making the API call.
Cancel the previous API call if a new input change occurs before the time interval is up.
Use a loading spinner to indicate to the user that the API call is in progress.
Consider using a caching mechanism to store the API response for future use.
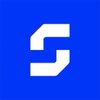
Asked in Sun Dew Solutions

Q. According to you what are the futuristic skills required for a frontend developer?
Futuristic skills for a frontend developer include AI, VR/AR, PWAs, and accessibility.
Proficiency in AI and machine learning for creating intelligent interfaces
Knowledge of VR/AR for developing immersive experiences
Expertise in Progressive Web Apps (PWAs) for building fast and reliable web applications
Understanding of accessibility guidelines and techniques for creating inclusive designs
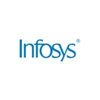
Asked in Infosys

Q. what is jquery? 2.explain architecture of your project.
jQuery is a fast, small, and feature-rich JavaScript library.
jQuery simplifies HTML document traversing, event handling, and animating.
It provides a set of methods for AJAX interactions and DOM manipulation.
jQuery is cross-platform and supports a wide range of browsers.
It has a large community and a vast number of plugins available.
Project architecture depends on the specific project and its requirements.
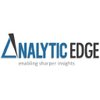
Asked in Analytic Edge

The process of building a front-end project involves planning, designing, coding, testing, and deploying.
Gather requirements and create a project plan
Design the user interface using tools like Figma or Sketch
Code the front-end using HTML, CSS, and JavaScript
Test the project for bugs and usability
Deploy the project to a web server or hosting platform

Asked in IBS Software Services

Q. How would you develop an application that displays all airports in the country on a map, allowing users to log in and report incidents at specific airports?
Develop an app to show all airports in a country on a map and allow users to report incidents after logging in.
Use a map API like Google Maps or Mapbox to display all airports in the country
Implement a login system for users to report incidents
Create a form for users to report incidents with fields like airport name, incident type, and description
Store incident reports in a database for future reference and analysis
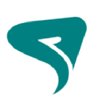
Asked in Protocol Zone

Q. what is filter method which is better to use filter method or foor loop
The filter method is a built-in JavaScript method used to create a new array with all elements that pass a certain condition.
Filter method is more concise and readable compared to for loop
Filter method is more declarative and functional programming oriented
Example: const numbers = [1, 2, 3, 4, 5]; const evenNumbers = numbers.filter(num => num % 2 === 0);
Example: const words = ['hello', 'world', 'example']; const shortWords = words.filter(word => word.length < 6);
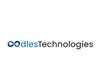
Asked in OodlesTechnologies

Data can be shared between components in Angular using services, input/output properties, and observables.
Use services to create a shared data service that can be injected into multiple components
Use input/output properties to pass data from parent to child components
Use observables to create a data stream that can be subscribed to by multiple components
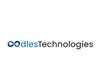
Asked in OodlesTechnologies

typeof() operator in JavaScript is used to determine the data type of a variable or expression.
typeof() returns a string indicating the type of the operand.
Example: typeof 42 will return 'number'.
Example: typeof 'hello' will return 'string'.
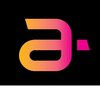
Asked in Amdocs

Components in React go through various stages like mounting, updating, and unmounting.
Components are created and inserted into the DOM during the mounting phase.
During the updating phase, components can re-render due to changes in props or state.
Components are removed from the DOM during the unmounting phase.
Lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount are used to perform actions at different stages.
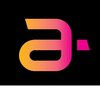
Asked in Amdocs

Prototype chaining in JavaScript is the mechanism by which objects inherit properties and methods from other objects.
In JavaScript, each object has a prototype property that points to another object. When a property or method is accessed on an object, JavaScript will look for it in the object itself first, and then in its prototype chain.
If the property or method is not found in the object, JavaScript will continue to look up the prototype chain until it finds the property or...read more
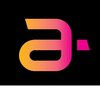
Asked in Amdocs

HTML5 is the latest version of the HTML standard with new features for web development.
Support for multimedia elements like <video> and <audio>
Canvas and SVG for graphics and animations
Improved form controls and validation
Offline storage with Local Storage and IndexedDB
Geolocation API for location-based services

Asked in Gainsight

The 'this' operator in JavaScript refers to the current context or object.
Refers to the current object or context in which a function is being executed
Can be used to access properties and methods of the current object
The value of 'this' is determined by how a function is called
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
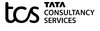
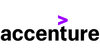
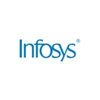
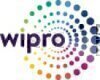
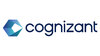
Top Interview Questions for Front end Developer Related Skills
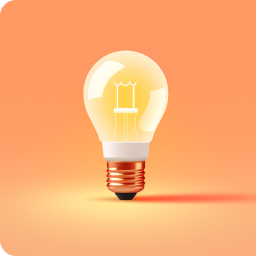
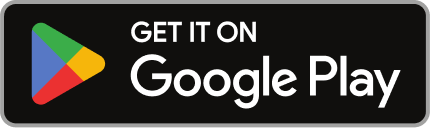
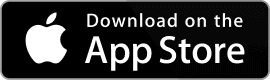
Reviews
Interviews
Salaries
Users
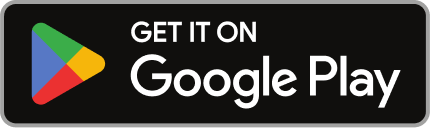
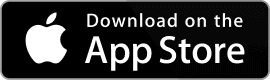