Front end Developer
100+ Front end Developer Interview Questions and Answers for Freshers
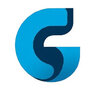
Asked in GrapplTech

Q. What is the Flexbox layout in CSS, and how does it work?
Flexbox is a CSS layout model that allows for responsive design by distributing space along a single axis.
Flexbox stands for 'Flexible Box' and is designed for one-dimensional layouts.
It uses properties like 'display: flex;' to create a flex container.
Items within the container can be aligned and distributed using properties like 'justify-content' and 'align-items'.
Example: 'justify-content: center;' centers items horizontally.
Flexbox allows for flexible item sizes, adapting ...read more
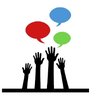
Asked in The Sparks Foundation

Q. In HTML, is span a tag?
Yes, span is a tag in HTML used to apply styles to inline elements.
Span is an inline element used to apply styles to a specific section of text or content.
It does not add any extra space or line breaks.
Example: <span style='color: red;'>This text will be red</span>
Asked in NCheng

Q. What is the full form of WWW?
World Wide Web
Stands for World Wide Web
A system of interlinked hypertext documents accessed via the Internet
Commonly used to browse websites and access information
Asked in Thiran360AI

Q. What is the difference between props and hooks in React, and what are their use cases?
Props are used to pass data to components, while hooks manage state and lifecycle in functional components.
Props are immutable and passed from parent to child components.
Example: <ChildComponent name='John' /> - 'name' is a prop.
Hooks, like useState and useEffect, allow functional components to manage state and side effects.
Example: const [count, setCount] = useState(0); - 'count' is state managed by a hook.
Props are used for data flow, while hooks enable stateful logic in fu...read more
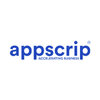
Asked in Appscrip

Q. How can you make a website responsive without using media queries?
Using flexible units like percentages and viewport units, along with fluid layouts and max-width properties.
Use percentages for widths instead of fixed pixels
Use viewport units like vw and vh for font sizes and container dimensions
Implement fluid layouts that adjust based on screen size
Set max-width properties to prevent content from becoming too wide on larger screens
Asked in Clickminds

Q. What is the lifestyle of React in terms of its development and usage?
React's lifecycle consists of phases that manage component creation, updating, and unmounting for efficient rendering.
Mounting: Components are created and inserted into the DOM. Example: constructor() and render() methods.
Updating: Components re-render in response to state or props changes. Example: componentDidUpdate() lifecycle method.
Unmounting: Components are removed from the DOM. Example: componentWillUnmount() for cleanup tasks.
Hooks: React 16.8 introduced hooks like us...read more
Front end Developer Jobs
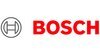
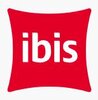
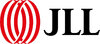
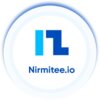
Asked in Nirmitee.io

Q. What is the difference between a function and a component?
Functions are reusable blocks of code, while components are self-contained UI elements in frameworks like React.
Functions are defined using the 'function' keyword or arrow syntax, e.g., 'const add = (a, b) => a + b;'.
Components are typically defined as classes or functions in frameworks like React, e.g., 'const MyComponent = () => <div>Hello</div>;'.
Functions can return values or perform actions, while components return JSX or HTML to render UI.
Components can manage their own...read more
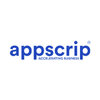
Asked in Appscrip

Q. Describe how you would create three boxes and align them to the center of the screen using front-end technologies.
Create and center three boxes on the screen using HTML and CSS.
Use HTML to create three div elements for the boxes.
Apply CSS to style the boxes with width, height, and background color.
Use Flexbox or CSS Grid to center the boxes on the screen.
Example CSS: .container { display: flex; justify-content: center; align-items: center; height: 100vh; }
Example HTML: <div class='box'></div> for each box.
Share interview questions and help millions of jobseekers 🌟
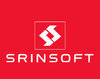
Asked in SrinSoft Technologies

Q. Introduce yourself, write sql query to list out employees above age of 23
Experienced front end developer with SQL skills. SQL query to list employees above age 23.
Use SELECT statement to retrieve data from employees table
Use WHERE clause to filter employees above age 23
Example: SELECT * FROM employees WHERE age > 23;
Asked in Kiaan Technology

Q. How would you create a responsive layout without using a CSS framework?
Creating a responsive layout involves using flexible grids, media queries, and fluid images to adapt to various screen sizes.
Use a fluid grid system with percentages instead of fixed units. For example, set a container width to 100% and child elements to 50%.
Implement media queries to adjust styles based on screen size. For instance, use '@media (max-width: 600px) { ... }' to change layout for mobile.
Utilize flexible images by setting max-width to 100% to ensure they scale wi...read more
Asked in RSSPA IT Solutions

Q. What is the difference between padding and spacing?
Padding is the space inside an element, while spacing is the space around an element.
Padding is the space inside an element, between the content and the border.
Spacing refers to the space around an element, including margins and borders.
Padding can be adjusted using CSS properties like padding-top, padding-bottom, etc.
Spacing can be adjusted using CSS properties like margin-top, margin-bottom, etc.
Asked in Yipli

Q. What are Components in React?
Components in React are reusable, independent pieces of code that manage their own state and can be composed together to build complex user interfaces.
Components are the building blocks of React applications
They can be class components or functional components
Components can have their own state and lifecycle methods
Components can be reused throughout the application
Example:
, ,
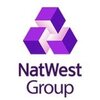
Asked in NatWest Group

Q. Why is React reusable?
React is reusable because it allows developers to create components that can be easily reused throughout an application.
React components can be easily reused in different parts of an application, saving time and effort.
Components can be composed together to build complex UIs, promoting reusability.
React's virtual DOM efficiently updates only the components that have changed, improving performance and reusability.
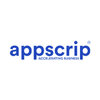
Asked in Appscrip

Q. How can you center an item without using flexbox or grid?
To center an item without flex and grid, use text-align center for inline elements and margin auto for block elements.
For inline elements, use text-align: center on the parent element
For block elements, use margin: 0 auto on the element you want to center
For inline-block elements, set text-align: center on the parent and display: inline-block on the child
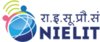
Asked in NIELIT

Q. What is the use of the 'this' keyword?
The 'this' keyword refers to the current object and is used to access its properties and methods.
Used to refer to the current object in a method or constructor
Can be used to call another constructor in the same class
Can be used to call a method of the same object
Can be used to call a method of a parent object
Can be used to bind a function to a specific object
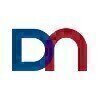
Asked in Diebold Nixdorf

Q. How do you pass data between components in Angular?
Data can be passed between components in Angular using input properties, output properties, services, and state management libraries like NgRx.
Use input properties to pass data from parent to child components
Use output properties to emit events from child to parent components
Use services to create a shared data service that can be injected into multiple components
Use state management libraries like NgRx for managing application state and sharing data between components
Asked in Athamas Technologies

Q. How can you disable a button after it's clicked?
You can make a button disabled on click by adding a click event listener and setting the 'disabled' attribute.
Add a click event listener to the button element
Inside the event listener function, set the 'disabled' attribute of the button to true
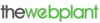
Asked in The Web Plant

Q. 1. What is the background position in CSS? 2. Write a code for nested code for the unordered list?
Background position in CSS is used to specify the starting position of a background image.
Background position can be set using keywords like top, bottom, left, right, center, or using specific pixel or percentage values.
Example: background-position: top right;
Example: background-position: 50% 50%;
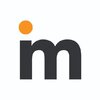
Asked in Mobikasa

Q. Design another page, similar to the one from the first round.
Design a user-friendly landing page for a fictional e-commerce website showcasing products and promotions.
Use a clean and modern layout with a prominent hero image to capture attention.
Incorporate a navigation bar with categories like 'Home', 'Shop', 'About Us', and 'Contact'.
Include a section for featured products with images, prices, and 'Add to Cart' buttons.
Add promotional banners for discounts or seasonal sales to encourage purchases.
Ensure the page is responsive, adapti...read more
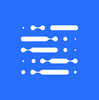
Asked in Sekel Technologies

Q. What is Next.js and what are its key features?
Next.js is a React framework for building server-side rendered and static web applications with ease.
Server-Side Rendering (SSR): Automatically renders pages on the server for better SEO and performance.
Static Site Generation (SSG): Pre-renders pages at build time, improving load times (e.g., blog posts).
API Routes: Allows building API endpoints within the application (e.g., /api/users).
File-Based Routing: Simplifies routing by using the file system (e.g., pages/about.js maps...read more
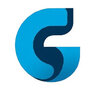
Asked in GrapplTech

Q. What are grids in CSS and how are they used?
CSS grids are a layout system that allows for responsive design using rows and columns.
CSS Grid Layout is a two-dimensional layout system, enabling control over both rows and columns.
It uses a grid container to define the grid structure with properties like 'display: grid'.
Items within the grid can be placed precisely using grid lines, areas, and cells.
Example: To create a simple grid, use 'grid-template-columns: repeat(3, 1fr);' for three equal columns.
Grid can adapt to diff...read more
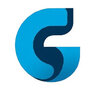
Asked in GrapplTech

Q. What are the features of ES6 in JavaScript?
ES6 introduced significant improvements to JavaScript, enhancing syntax and functionality for developers.
Arrow Functions: Shorter syntax for function expressions. Example: const add = (a, b) => a + b;
Template Literals: Multi-line strings and string interpolation. Example: const greeting = `Hello, ${name}!`;
Destructuring Assignment: Unpacking values from arrays or properties from objects. Example: const [x, y] = [1, 2];
Default Parameters: Allowing named parameters to be initia...read more
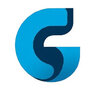
Asked in GrapplTech

Q. What is the code for adding elements to an array?
Adding elements to an array can be done using methods like push, unshift, and splice in JavaScript.
1. Using push(): Adds one or more elements to the end of an array. Example: let fruits = ['apple', 'banana']; fruits.push('orange'); // ['apple', 'banana', 'orange']
2. Using unshift(): Adds one or more elements to the beginning of an array. Example: let fruits = ['apple', 'banana']; fruits.unshift('orange'); // ['orange', 'apple', 'banana']
3. Using splice(): Adds elements at a s...read more
Asked in Zedex Info Pvt Ltd

Q. What is the difference between block and inline-block elements?
Block elements take up the full width available, while inline-block elements only take up as much width as necessary.
Block elements start on a new line and take up the full width available (e.g. <div>).
Inline-block elements do not start on a new line and only take up as much width as necessary (e.g. <span>).
Block elements can have margins and padding applied to all four sides, while inline-block elements only have horizontal margins and padding.
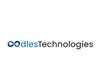
Asked in OodlesTechnologies

Q. What is the return type of getElementsByClassName in JavaScript?
getElementsByClassName returns a live HTMLCollection of elements with the specified class name.
Returns an HTMLCollection, which is an array-like object.
HTMLCollection is live, meaning it updates automatically with DOM changes.
Example: const elements = document.getElementsByClassName('myClass');
Access elements using index: elements[0], elements[1], etc.
Use Array.from() to convert HTMLCollection to an array for easier manipulation.
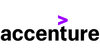
Asked in Accenture

Q. What is event bubbling?
Event bubbling is the process in which an event triggered on a nested element is also triggered on its parent elements.
Events in JavaScript propagate from the innermost element to the outermost element.
During event bubbling, the event is first handled by the innermost element and then propagated to its parent elements.
This allows for event delegation, where a single event handler can be used to handle events on multiple elements.
Event.stopPropagation() can be used to stop the...read more
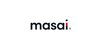
Asked in MASAI School

Q. Explain web development in simple terms that a 5-year-old child can understand.
Web development is like building a house on the internet where people can visit and do things.
Web development is creating websites and web applications using programming languages like HTML, CSS, and JavaScript.
It involves designing how the website looks and works, and making sure it works well on different devices like computers and phones.
Examples of web development include creating a website for a business, an online store, or a social media platform.
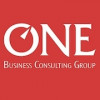
Asked in ONE BCG

Q. They usually asked me about OOPs concepts and coding. Can you elaborate?
Object-Oriented Programming (OOP) concepts are fundamental for structuring code in a modular and reusable way.
Encapsulation: Bundling data and methods that operate on the data within one unit (class). Example: A 'Car' class with properties like 'color' and methods like 'drive()'.
Inheritance: Mechanism to create a new class using properties and methods of an existing class. Example: 'ElectricCar' inherits from 'Car'.
Polymorphism: Ability to present the same interface for diffe...read more
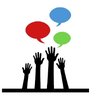
Asked in The Sparks Foundation

Q. In css box module or components
The CSS box model is a design concept that describes how elements are rendered on a web page.
The box model consists of content, padding, border, and margin.
The content area is where the actual content of the element is displayed.
Padding is the space between the content and the border.
Border is a line that surrounds the padding and content.
Margin is the space outside the border, separating the element from other elements.
The box model allows for precise control over the layout...read more
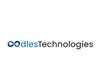
Asked in OodlesTechnologies

Q. Javascript typeof() method
The typeof() method in JavaScript returns the data type of a variable or expression.
typeof() returns a string indicating the type of the operand.
It can be used with variables, literals, or expressions.
Common return values include 'number', 'string', 'boolean', 'object', 'function', and 'undefined'.
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
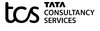
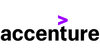
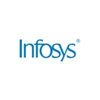
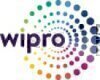
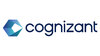
Top Interview Questions for Front end Developer Related Skills
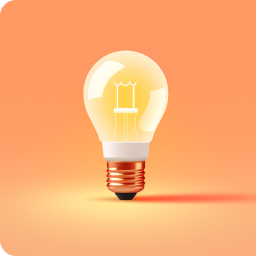
Calculate your in-hand salary
Confused about how your in-hand salary is calculated? Enter your annual salary (CTC) and get your in-hand salary
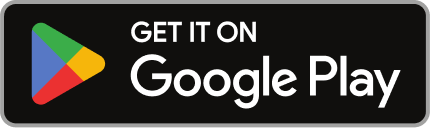
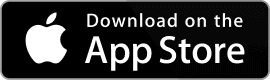
Reviews
Interviews
Salaries
Users
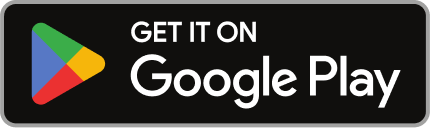
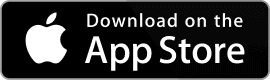