Front end Developer
100+ Front end Developer Interview Questions and Answers for Freshers
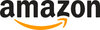
Asked in Amazon

Q. Check If Linked List Is Palindrome
Given a singly linked list of integers, determine if the linked list is a palindrome.
Explanation:
A linked list is considered a palindrome if it reads the same forwards and b...read more
Check if a given singly linked list of integers is a palindrome or not.
Create a function to reverse the linked list.
Use two pointers to find the middle of the linked list.
Compare the first half of the linked list with the reversed second half to determine if it's a palindrome.
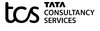
Asked in TCS

Q. Allocate Books Problem Statement
Given an array of integers arr
, where arr[i]
represents the number of pages in the i-th
book, and an integer m
representing the number of students, allocate all the books in suc...read more
Allocate books to students in a way that minimizes the maximum number of pages assigned to a student.
Iterate through possible allocations and calculate the maximum pages assigned to a student.
Find the minimum of these maximums to get the optimal allocation.
Return the minimum pages allocated in each test case, or -1 if not possible.
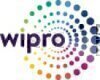
Asked in Wipro

Q. Segregate Odd-Even Problem Statement
In a wedding ceremony at NinjaLand, attendees are blindfolded. People from the bride’s side hold odd numbers, while people from the groom’s side hold even numbers. For the g...read more
Rearrange a linked list such that odd numbers appear before even numbers while preserving the order.
Create two separate linked lists for odd and even numbers
Traverse the original list and append nodes to respective odd/even lists
Combine the odd and even lists while maintaining the order
Return the rearranged linked list
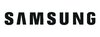
Asked in Samsung

Q. Triplets with Given Sum Problem
Given an array or list ARR
consisting of N
integers, your task is to identify all distinct triplets within the array that sum up to a specified number K
.
Explanation:
A triplet i...read more
The task is to identify all distinct triplets within an array that sum up to a specified number.
Iterate through the array and use nested loops to find all possible triplets.
Check if the sum of the triplet equals the specified number.
Print the valid triplets or return -1 if no such triplet exists.
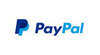
Asked in PayPal

Q. Find Magic Index in Sorted Array
Given a sorted array A consisting of N integers, your task is to find the magic index in the given array, where the magic index is defined as an index i such that A[i] = i.
Exam...read more
Find the magic index in a sorted array where A[i] = i.
Iterate through the array and check if A[i] = i for each index i.
Since the array is sorted, you can optimize the search using binary search.
Return the index if found, else return -1.
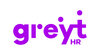
Asked in greytHR

Q. Most Frequent Non-Banned Word Problem Statement
Given a paragraph consisting of letters in both lowercase and uppercase, spaces, and punctuation, along with a list of banned words, your task is to find the most...read more
Find the most frequent word in a paragraph that is not in a list of banned words.
Split the paragraph into words and convert them to uppercase for case-insensitivity.
Count the frequency of each word, excluding banned words.
Return the word with the highest frequency in uppercase.
Front end Developer Jobs
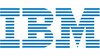
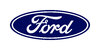
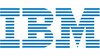
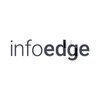
Asked in Info Edge

Q. Matching Prefix Problem Statement
Given an integer N
representing the number of strings in an array Arr
composed of lowercase English alphabets, determine a string S
of length N
such that it can be used to dele...read more
Given an array of strings, find the lexicographically smallest string that can be used as a prefix to minimize the total cost of deleting prefixes from the strings.
Iterate through the array to find the common prefix among all strings
Choose the lexicographically smallest common prefix
Delete the common prefix from each string in the array to minimize the total cost
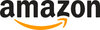
Asked in Amazon

Q. Alien Dictionary Problem Statement
You are provided with a sorted dictionary (by lexical order) in an alien language. Your task is to determine the character order of the alien language from this dictionary. Th...read more
Given a sorted alien dictionary in an array of strings, determine the character order of the alien language.
Iterate through the words in the dictionary to build a graph of character dependencies.
Perform a topological sort on the graph to determine the character order.
Return the character array representing the order of characters in the alien language.
Share interview questions and help millions of jobseekers 🌟
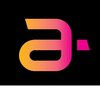
Asked in Amdocs

Q. Palindrome String Validation
Determine if a given string 'S' is a palindrome, considering only alphanumeric characters and ignoring spaces and symbols.
Note:
The string 'S' should be evaluated in a case-insensi...read more
Validate if a given string is a palindrome after removing special characters, spaces, and converting to lowercase.
Remove special characters and spaces from the input string
Convert the string to lowercase
Check if the modified string is equal to its reverse to determine if it's a palindrome
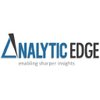
Asked in Analytic Edge

Q. Postorder Successor Problem Statement
You are provided with a binary tree consisting of 'N' distinct nodes and an integer 'M'. Your task is to find and return the postorder successor of 'M'.
Note:
The postorder...read more
Find the postorder successor of a node in a binary tree.
Traverse the binary tree in postorder to get the sequence.
Find the index of the node 'M' in the postorder sequence.
Return the element at the next index as the postorder successor.
If 'M' is the last element in the sequence, return -1 as there is no successor.
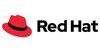
Asked in Red Hat

Q. Jumping Numbers Problem Statement
Given a positive integer N
, your goal is to find all the Jumping Numbers that are smaller than or equal to N
.
A Jumping Number is one where every adjacent digit has an absolute...read more
Find all Jumping Numbers smaller than or equal to a given positive integer N.
Iterate through numbers from 0 to N and check if each number is a Jumping Number.
For each number, check if the absolute difference between adjacent digits is 1.
Include all single-digit numbers as Jumping Numbers.
Output the list of Jumping Numbers that are smaller than or equal to N.
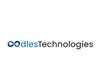
Asked in OodlesTechnologies

Q. Is JavaScript single-threaded or multi-threaded?
Javascript is single-threaded.
Javascript runs on a single thread called the event loop.
This means that it can only execute one task at a time.
However, it can delegate tasks to other threads using web workers.
This allows for parallel processing without blocking the main thread.
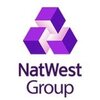
Asked in NatWest Group

Q. Are functional components and class components the same?
Functional components and class components are not the same in React. Functional components are simpler and more lightweight, while class components have additional features like state and lifecycle methods.
Functional components are stateless and are just JavaScript functions that return JSX.
Class components have access to state and lifecycle methods like componentDidMount and componentDidUpdate.
Functional components are easier to read and test, while class components can be ...read more
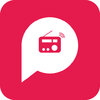
Asked in Pocket FM

Q. How would you optimize API calls on input change using debouncing?
Debounce the API call on input change to optimize performance.
Implement a debounce function to delay the API call until the user has finished typing.
Set a time interval for the debounce function to wait before making the API call.
Cancel the previous API call if a new input change occurs before the time interval is up.
Use a loading spinner to indicate to the user that the API call is in progress.
Consider using a caching mechanism to store the API response for future use.
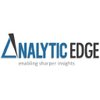
Asked in Analytic Edge

The process of building a front-end project involves planning, designing, coding, testing, and deploying.
Gather requirements and create a project plan
Design the user interface using tools like Figma or Sketch
Code the front-end using HTML, CSS, and JavaScript
Test the project for bugs and usability
Deploy the project to a web server or hosting platform
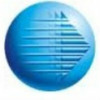
Asked in Protechsoft Technologies

Q. What is the 'this' operator?
The 'this' operator refers to the object that is currently executing the code.
The value of 'this' depends on how a function is called.
In a method, 'this' refers to the object that owns the method.
In a regular function, 'this' refers to the global object (window in a browser).
In an event handler, 'this' refers to the element that received the event.
The value of 'this' can be explicitly set using call(), apply(), or bind() methods.
Asked in Zedex Info Pvt Ltd

Q. How can you center three divs vertically and horizontally using CSS?
To center 3 divs vertically and horizontally, use flexbox and align-items/justify-content properties.
Wrap the 3 divs in a parent container
Set the parent container's display property to flex
Set the parent container's align-items and justify-content properties to center
Asked in TailNode

Q. How can you count the number of words in a given array of strings using higher-order functions?
Count the number of words in given strings of array using higher order function.
Use the map function to transform each string into an array of words
Use the reduce function to count the total number of words in all strings
Handle edge cases such as empty strings or strings with leading/trailing spaces
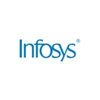
Asked in Infosys

Q. What are React lifecycle methods?
React lifecycle methods are functions that are called at different stages of a component's life.
Mounting: constructor(), render(), componentDidMount()
Updating: render(), componentDidUpdate()
Unmounting: componentWillUnmount()
Error Handling: componentDidCatch()
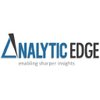
Asked in Analytic Edge

I built a responsive web application for managing tasks and deadlines.
Used HTML, CSS, and JavaScript to create the frontend
Implemented drag and drop functionality for task organization
Integrated a calendar API for deadline tracking
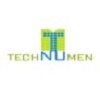
Asked in Technumen

Q. What is interceptor and where using and how to add interceptor for only selected requests?
Interceptors are middleware functions that can be used to intercept and modify HTTP requests and responses.
Interceptors can be used in Angular to modify requests before they are sent to the server or responses before they are delivered to the application.
To add an interceptor for only selected requests, you can create a custom interceptor class that implements the HttpInterceptor interface and then provide it in the HTTP_INTERCEPTORS multi-provider array.
You can then use cond...read more
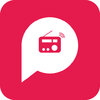
Asked in Pocket FM

Q. Write code to implement memoization.
Memoization optimizes function calls by caching results for previously computed inputs.
Memoization stores results of expensive function calls and returns cached results when the same inputs occur again.
Example: A Fibonacci function can be optimized using memoization to avoid redundant calculations.
In JavaScript, you can create a memoize function that takes another function as an argument and returns a new function.
Use an object or Map to store the results of function calls, u...read more
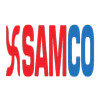
Asked in SAMCO Securities

Q. If both id and class are applied to an element, which style will take precedence?
The style of the id will apply first before the style of the class.
IDs have higher specificity than classes, so the style of the id will take precedence over the style of the class.
If both id and class have conflicting styles, the style of the id will be applied.
Example: <div id='uniqueId' class='commonClass'>...</div> - the style of 'uniqueId' will be applied.
Asked in TailNode

Q. Describe how to fetch an API with proper error handling using async/await.
To fetch an API with proper error handling and async await, use try-catch block and await keyword.
Use the fetch() function to make the API request.
Wrap the fetch() call in a try-catch block to handle errors.
Use the await keyword before the fetch() call to wait for the response.
Check the response status code to handle different scenarios.
Handle any errors or exceptions that may occur during the API request.
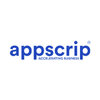
Asked in Appscrip

Q. Why are mobile numbers 10 digits long?
Mobile numbers are typically 10 digits long to ensure uniqueness and standardization for easy identification and communication.
Standardization: Having a fixed length of 10 digits makes it easier for systems to validate and process mobile numbers.
Uniqueness: With 10 digits, there are a large number of possible combinations, reducing the likelihood of duplicate numbers.
International compatibility: Many countries have adopted 10-digit mobile numbers as a standard format for easy...read more
Asked in Kiaan Technology

Q. What is the difference between relative, absolute, fixed, and sticky positioning in CSS?
CSS positioning types control how elements are placed in a document flow, affecting layout and responsiveness.
Relative: Positioned relative to its normal position. Example: `top: 10px;` moves it down from where it would normally be.
Absolute: Positioned relative to the nearest positioned ancestor (not static). Example: `position: absolute; top: 0;` places it at the top of the ancestor.
Fixed: Positioned relative to the viewport, remaining in the same place even when scrolling. ...read more
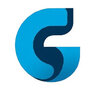
Asked in GrapplTech

Q. How would you develop a model component and apply CSS for styling it?
Developing a modal component involves creating a reusable UI element with HTML, CSS, and JavaScript for interactivity.
1. Create the HTML structure: Use a <div> for the modal with a header, body, and footer.
Example: <div class='modal'><div class='modal-header'>...</div><div class='modal-body'>...</div><div class='modal-footer'>...</div></div>
2. Style the modal with CSS: Use flexbox for centering and transitions for smooth opening/closing.
Example: .modal { display: flex; justif...read more
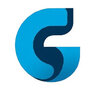
Asked in GrapplTech

Q. What does responsive design mean in the context of web development?
Responsive design ensures web applications adapt seamlessly to various screen sizes and devices for optimal user experience.
Fluid grids: Layouts that use percentage-based widths instead of fixed pixels, allowing elements to resize based on the screen size.
Media queries: CSS techniques that apply different styles based on device characteristics, such as width, height, or orientation.
Flexible images: Images that scale within their containing elements, often using CSS properties...read more
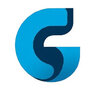
Asked in GrapplTech

Q. What is the Flexbox layout in CSS, and how does it work?
Flexbox is a CSS layout model that allows for responsive design by distributing space along a single axis.
Flexbox stands for 'Flexible Box' and is designed for one-dimensional layouts.
It uses properties like 'display: flex;' to create a flex container.
Items within the container can be aligned and distributed using properties like 'justify-content' and 'align-items'.
Example: 'justify-content: center;' centers items horizontally.
Flexbox allows for flexible item sizes, adapting ...read more
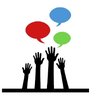
Asked in The Sparks Foundation

Q. In HTML, is span a tag?
Yes, span is a tag in HTML used to apply styles to inline elements.
Span is an inline element used to apply styles to a specific section of text or content.
It does not add any extra space or line breaks.
Example: <span style='color: red;'>This text will be red</span>
Interview Questions of Similar Designations
Interview Experiences of Popular Companies
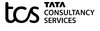
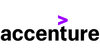
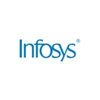
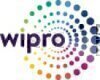
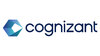
Top Interview Questions for Front end Developer Related Skills
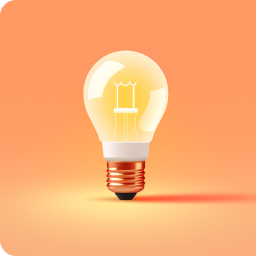
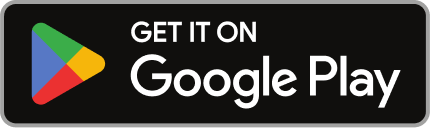
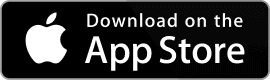
Reviews
Interviews
Salaries
Users
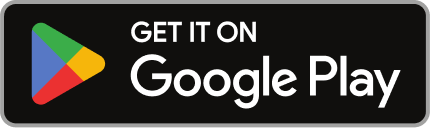
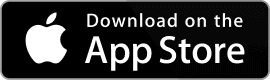