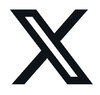
Asked in Twitter
Fibonacci Number Verification
Identify if the provided integer 'N' is a Fibonacci number.
A number is termed as a Fibonacci number if it appears in the Fibonacci sequence, where each number is the sum of the two preceding ones, with the sequence beginning from 0 and 1. The sequence can be described as:
Fn = F(n-1) + F(n-2)
Input:
The first input line contains a single integer ‘T’, representing the number of test cases.
Each test case has one integer 'N' in a new line, the number to assess if it is a Fibonacci number.
Output:
For each test case, output “YES” if the number is a Fibonacci number; otherwise, output “NO”.
Each result should be printed on a separate line.
Example:
Input:
3
2
4
8
Output:
YES
NO
YES
Constraints:
- 1 ≤ T ≤ 100
- 0 ≤ N ≤ 100000
Note: Implementation should not include printing; it is handled externally.
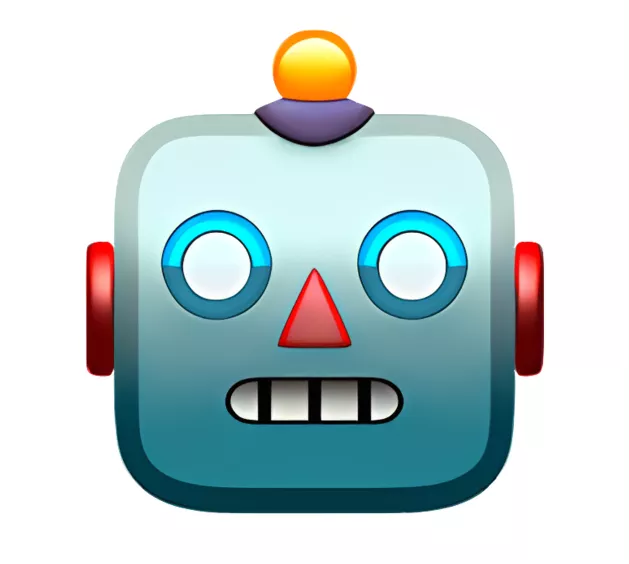
AnswerBot
4mo
Check if a given number is a Fibonacci number or not.
Iterate through the Fibonacci sequence until you find a number greater than or equal to the given number.
Check if the given number matches the Fibo...read more
Help your peers!
Add answer anonymously...
Top Software Developer Interview Questions Asked at Twitter
Q. Explain the process of calling an API.
Q. How do you test the feasibility of an idea?
Q. Design a Facebook-like application.
Interview Questions Asked to Software Developer at Other Companies
Top Skill-Based Questions for Twitter Software Developer
Algorithms Interview Questions and Answers
250 Questions
Data Structures Interview Questions and Answers
250 Questions
Web Development Interview Questions and Answers
250 Questions
Java Interview Questions and Answers
250 Questions
SQL Interview Questions and Answers
250 Questions
Software Development Interview Questions and Answers
250 Questions
Stay ahead in your career. Get AmbitionBox app
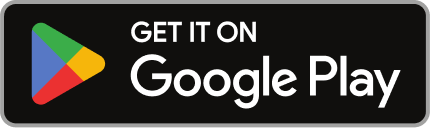
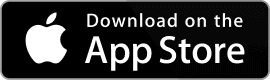
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
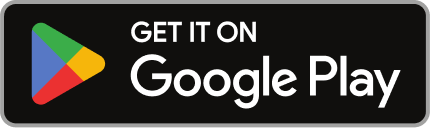
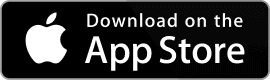