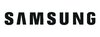
Asked in Samsung
Implement a Stack Using Two Queues
You are tasked with implementing a Stack data structure specifically designed to store integer data using two Queues. Utilize the inbuilt Queue for this purpose.
Function Requirements:
1. Constructor: Initializes the data members (queues) as needed.
2. push(data): This function takes one integer argument, pushes the element on the stack, and does not return anything.
3. pop(): Removes the element at the top of the stack and returns it. If the stack is empty, it returns -1.
4. top: Retrieves the element at the top of the stack without removing it. If the stack is empty, it returns -1.
5. size(): Returns the size of the stack at any given time.
6. isEmpty(): Returns a boolean indicating if the stack is empty.
Operations on the Stack:
Query-1: Push an integer onto the stack.
Query-2: Pop and return the top of the stack.
Query-3: Retrieve the top of the stack.
Query-4: Get the current size of the stack.
Query-5: Check if the stack is empty.
Input:
Input consists of an integer specifying the number of queries, followed by queries themselves which perform operations on the stack.
The first line contains an integer 'Q', the number of queries.
Each of the next 'Q' lines contains details of the operation:
- For push operation, the line will contain two integers indicating the operation type and data to be pushed.
- For other operations, the line will contain one integer indicating the query.
Output:
- For push operation, return nothing.
- For pop operation, output the popped data.
- For top operation, output the data at the top of the stack.
- For size operation, output the size of the stack.
- For isEmpty operation, output 'true' or 'false' (without quotes).
Each output should be on a separate line.
Constraints:
- 1 <= Q <= 1000
- -10^9 <= data <= 10^9 and data ≠ -1
- Each query type will be between 1 and 5.
Total time allowed is 1 second.
Note:
There's no need to print explicitly since output handling is managed; focus on implementing the needed functions only.
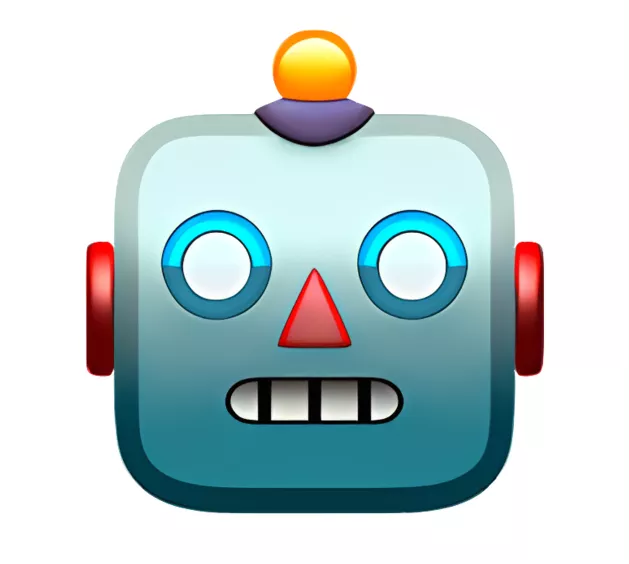
AnswerBot
4mo
Implement a Stack data structure using two Queues for integer data.
Use two Queues to simulate the Stack behavior.
Push elements by enqueueing them in one Queue.
Pop elements by dequeueing all elements f...read more
Help your peers!
Add answer anonymously...
Top Software Developer Interview Questions Asked at Samsung
Q. All 1's and 2's of array
Q. Design a ticket booking system like Bookmyshow.
Q. How do you find the second largest salary in a DBMS?
Interview Questions Asked to Software Developer at Other Companies
Top Skill-Based Questions for Samsung Software Developer
Algorithms Interview Questions and Answers
250 Questions
Data Structures Interview Questions and Answers
250 Questions
Web Development Interview Questions and Answers
250 Questions
Java Interview Questions and Answers
250 Questions
SQL Interview Questions and Answers
250 Questions
Software Development Interview Questions and Answers
250 Questions
Stay ahead in your career. Get AmbitionBox app
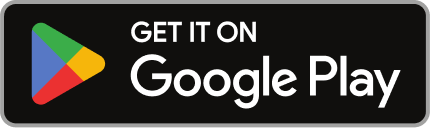
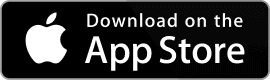
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
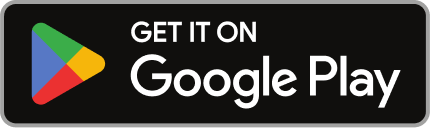
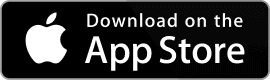