Count Leaf Nodes in a Binary Tree
Count the number of leaf nodes present in a given binary tree. A binary tree is a data structure where each node has at most two children, known as the left child and the right child. A node is considered a leaf node if both of its child nodes are NULL.
Input:
The first line of input contains an integer 'T', representing the number of test cases. Each test case consists of a line containing elements of the binary tree in level order. If a node is null, it is represented by -1.
Output:
For each test case, output the number of leaf nodes present in the binary tree.
Example:
Input:
1
20 10 35 5 15 30 42 -1 13 -1 -1 -1 -1 -1 -1 -1
Output:
4
Explanation:
In the above example, nodes 5, 30, 42, and 13 are leaf nodes because they have no child nodes.
Constraints:
1 <= T <= 100
1 <= N <= 10^3
1 <= data <= 10^9
- Time Limit: 1 second
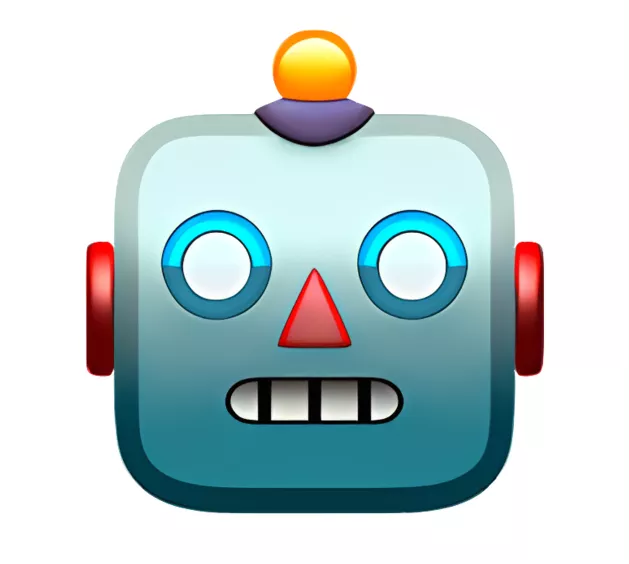
AnswerBot
4mo
Count the number of leaf nodes in a binary tree where each node has at most two children.
Traverse the binary tree and count nodes with both children as NULL.
Use recursion to check each node in the tre...read more
Help your peers!
Add answer anonymously...
Samsung Software Developer Intern interview questions & answers
A Software Developer Intern was asked 7mo agoQ. Suppose an array of length n sorted in ascending order is rotated between 1 and ...read more
A Software Developer Intern was asked 7mo agoQ. What are the ACID properties in DBMS?
A Software Developer Intern was asked Q. Sum of Digits Problem Statement Given an integer 'N', continue summing its digit...read more
Popular interview questions of Software Developer Intern
A Software Developer Intern was asked 6mo agoQ1. Suppose an array of length n sorted in ascending order is rotated between 1 and ...read more
A Software Developer Intern was asked 6mo agoQ2. What are the ACID properties in DBMS?
A Software Developer Intern was asked Q3. Sum of Digits Problem Statement Given an integer 'N', continue summing its digit...read more
Stay ahead in your career. Get AmbitionBox app
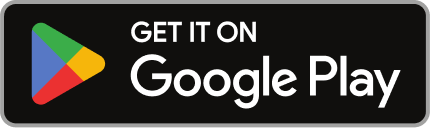
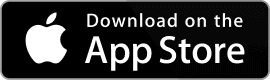
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
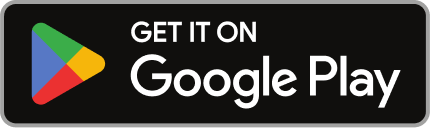
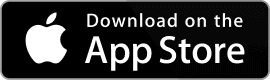