Queue Using Stacks Implementation
Design a queue data structure following the FIFO (First In First Out) principle using only stack instances.
Explanation:
Your task is to complete predefined functions to support all standard queue operations efficiently using stacks.
The queue should handle the following operations:
enQueue(data)
: Insert an integer 'data' at the end of the queue.deQueue()
: Remove and return the integer from the front of the queue. Return -1 if the queue is empty.peek()
: Return the integer at the front without removing it. Return -1 if the queue is empty.isEmpty()
: Return true if the queue is empty, otherwise false.
Input:
The first line contains an integer 'T', the number of queries.
Each of the next 'T' lines contains a query:
Type '1 val' to insert 'val' into the queue.
Type '2' to remove and return the front element.
Type '3' to return the front element.
Type '4' to check if the queue is empty.
Output:
There is no output needed for query type 1.
For query type 2, return the removed element from the queue.
For query type 3, return the front element from the queue.
For query type 4, return ‘true’ or ‘false’ based on queue's emptiness.
Example:
Suppose the input is:
T = 5 queries.
1 5 (enQueue 5)
1 10 (enQueue 10)
3 (peek the front element)
2 (deQueue the front element)
4 (check if empty)
Corresponding Output:
5 (from peek)
5 (from deQueue)
false (queue is not empty)
Constraints:
1 <= T <= 1000
1 <= type <= 4
1 <= data <= 10^9
- The queue's maximum capacity can be considered infinite.
Note:
- You are not required to display the output as it has already been handled. Just implement the functions.
- Use stack data structures only for this implementation.
- Leverage inbuilt stack functionalities in languages like C++ and Java if available.
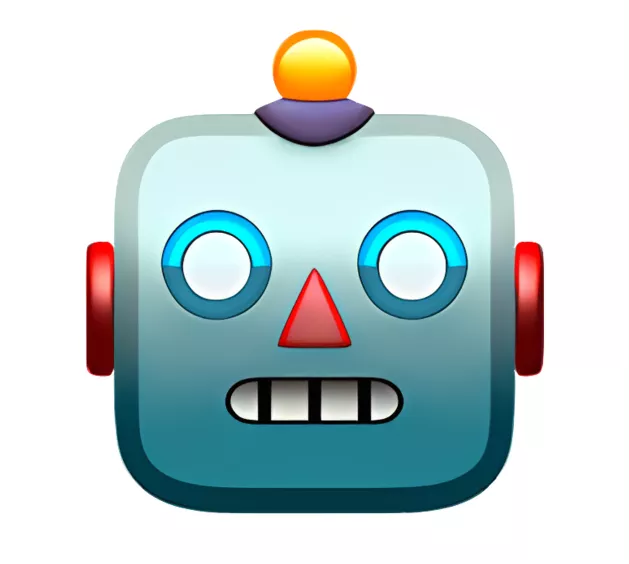
AnswerBot
4mo
Implement a queue using stacks following FIFO principle.
Use two stacks to simulate a queue - one for enqueueing and one for dequeueing.
When dequeueing, if the dequeue stack is empty, transfer all elem...read more
Help your peers!
Add answer anonymously...
Stay ahead in your career. Get AmbitionBox app
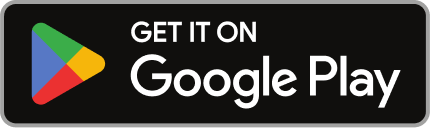
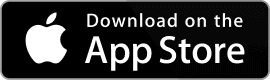
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
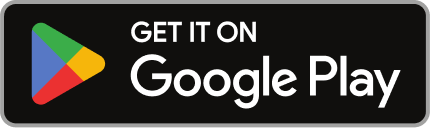
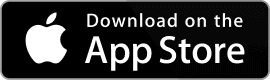