Longest Happy String Problem Statement
Given three non-negative integers X
, Y
, and Z
, determine the longest happy string. A happy string is defined as a string that contains only the letters 'a', 'b', and 'c' without having any three consecutive letters being the same.
Explanation:
You need to construct the longest string possible such that it has 'a' at most X
times, 'b' at most Y
times, and 'c' at most Z
times.
If there are multiple strings with maximum length, any of these strings can be returned.
Input:
The first line contains an integer 'T' representing the number of test cases. Each test case consists of one line containing three space-separated integers: X, Y, and Z.
Output:
For each test case, return "1" if a correct happy string can be formed, otherwise return "0".
Example:
Input:
2
1 1 7
2 2 1
Output:
1
1
Constraints:
1 ≤ T ≤ 5
0 ≤ X, Y, Z ≤ 10^3
X + Y + Z ≥ 1
Note:
You are not required to input or print anything. Focus on implementing the function to achieve the desired result.
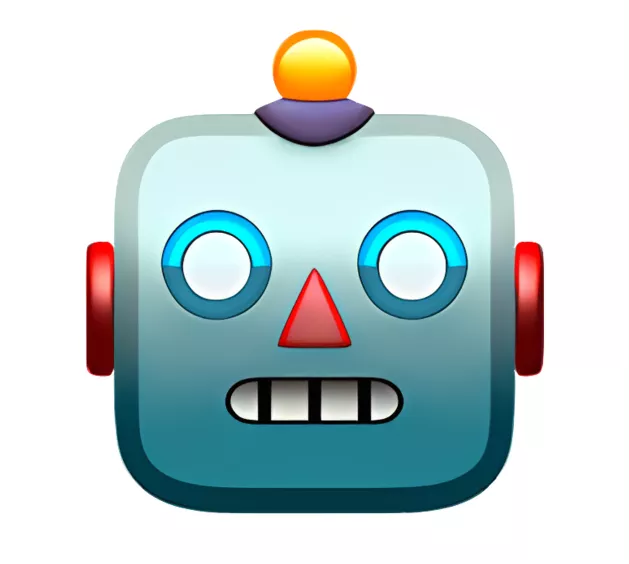
AnswerBot
4mo
The longest happy string problem involves constructing a string with 'a', 'b', and 'c' without having any three consecutive letters being the same.
Determine the maximum number of times 'a', 'b', and '...read more
Help your peers!
Add answer anonymously...
Salesforce Member Technical Staff interview questions & answers
A Member Technical Staff was asked 3mo agoQ. How do you find the median using a min-heap data structure in a stream of number...read more
A Member Technical Staff was asked Q. Design a HashMap.
A Member Technical Staff was asked Q. Deep diving into current project works
Popular interview questions of Member Technical Staff
A Member Technical Staff was asked 3mo agoQ1. How do you find the median using a min-heap data structure in a stream of number...read more
A Member Technical Staff was asked Q2. Design a HashMap.
A Member Technical Staff was asked Q3. Deep diving into current project works
>
Salesforce Member Technical Staff Interview Questions
Stay ahead in your career. Get AmbitionBox app
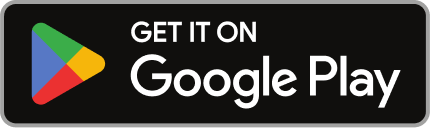
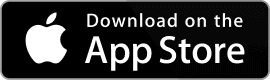
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
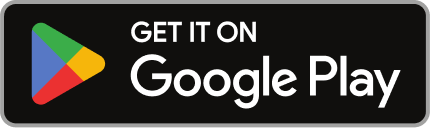
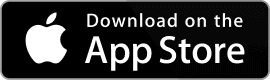