Friends Pairing Problem
The task is to determine the total number of ways to pair up 'N' friends or leave some of them single, following these rules:
- Each friend can either pair with one other friend or stay single.
- A friend can only be part of one pair.
The result should be computed modulo 1000000007 (109+7) due to potentially large outputs.
Example:
Input:
T = 2
N = 3
N = 4
Output:
4
10
Constraints:
- 1 <= T <= 100
- 1 <= N <= 104
Note:
1. Compute the result using modulo 109 + 7.
2. Pairs {A, B} and {B, A} are identical.
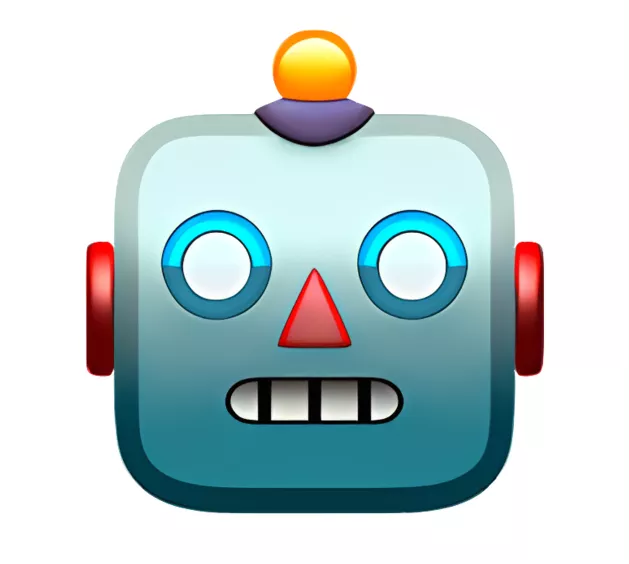
AnswerBot
4mo
The task is to determine the total number of ways to pair up 'N' friends or leave some of them single.
Use dynamic programming to solve the problem efficiently.
Consider the base cases when N is 1, 2, a...read more
Help your peers!
Add answer anonymously...
PayPal Full Stack Developer interview questions & answers
A Full Stack Developer was asked Q. Group Anagrams Together Given an array/list of strings STR_LIST, group the anagr...read more
A Full Stack Developer was asked Q. Rearrange String Problem Statement Given a string ‘S’, your task is to rearrange...read more
A Full Stack Developer was asked Q. Divide Two Integers Problem Statement You are given two integers dividend and di...read more
Popular interview questions of Full Stack Developer
A Full Stack Developer was asked Q1. Group Anagrams Together Given an array/list of strings STR_LIST, group the anagr...read more
A Full Stack Developer was asked Q2. Rearrange String Problem Statement Given a string ‘S’, your task is to rearrange...read more
A Full Stack Developer was asked Q3. Divide Two Integers Problem Statement You are given two integers dividend and di...read more
Stay ahead in your career. Get AmbitionBox app
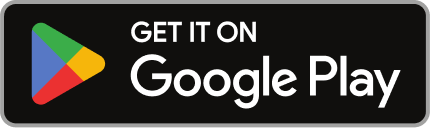
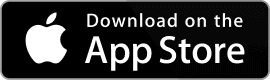
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
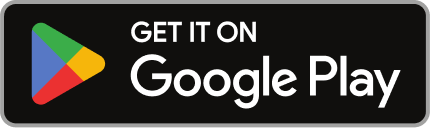
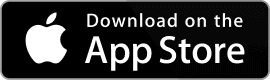