Matrix Rank Calculation
Given a matrix ARR
of dimensions N * M
, your task is to determine the rank of the matrix ARR
.
Explanation:
The rank of a matrix is defined as:
(a) The maximum number of linearly independent column vectors in the matrix, or
(b) The maximum number of linearly independent row vectors in the matrix.
Both definitions are equivalent.
Linear independence is defined as follows:
In vector space theory, a set of vectors is considered linearly dependent if there is a nontrivial linear combination of the vectors that equates to the zero vector. If no such linear combination exists, then the vectors are linearly independent.
Input:
The first line contains a single integer ‘T’ denoting the number of test cases.
Each test case consists of:
- The first line contains two space-separated integers, ‘N’ and ‘M’, representing the number of rows and the number of columns respectively.
- The next ‘N’ lines each contain ‘M’ integers that represent the matrix elements.
Output:
For each test case, return the rank of the matrix.
Example:
Input:
2
3 3
1 2 3
4 5 6
7 8 9
2 2
1 2
3 4
Output:
2
2
Constraints:
1 <= T <= 10
1 <= N, M <= 500
-10^4 <= ARR[i][j] <= 10^4
Where ARR[i][j]
denotes the matrix element at the jth column in the ith row of ARR
.
Time Limit: 1 sec
Note:
You are not required to print anything; it has already been taken care of. Just implement the given function and return the answer.
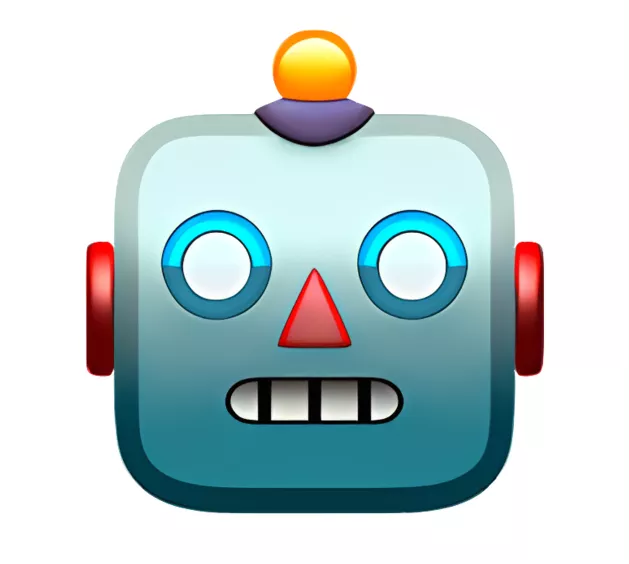
AnswerBot
4mo
Calculate the rank of a matrix based on linearly independent column or row vectors.
Determine the number of linearly independent column vectors or row vectors in the matrix.
Use Gaussian elimination or ...read more
Help your peers!
Add answer anonymously...
Microsoft Corporation Full Stack Developer interview questions & answers
A Full Stack Developer was asked Q. Who is the chairman of ISRO?
A Full Stack Developer was asked Q. What is the full form of UNESCO?
A Full Stack Developer was asked Q. Alternating Largest Problem Statement Given a list of numbers, rearrange them su...read more
Popular interview questions of Full Stack Developer
A Full Stack Developer was asked Q1. Who is the chairman of ISRO?
A Full Stack Developer was asked Q2. What is the full form of UNESCO?
A Full Stack Developer was asked Q3. Alternating Largest Problem Statement Given a list of numbers, rearrange them su...read more
>
Microsoft Corporation Full Stack Developer Interview Questions
Stay ahead in your career. Get AmbitionBox app
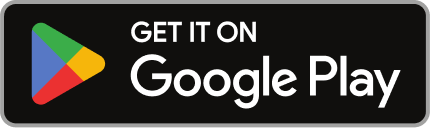
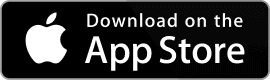
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
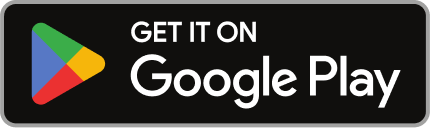
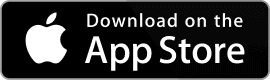