Design a HashSet
Create a HashSet data structure without using any built-in hash table libraries.
Functions Description:
1) Constructor: Initializes the data members as required.
2) add(value): Inserts an element into the HashSet. Takes one argument, the value to be added, and returns nothing.
3) contains(value): Checks if the element exists in the HashSet. Takes one argument, the value to be searched for. Returns true if the element exists, otherwise returns false.
4) remove(value): Removes an element from the HashSet. Takes one argument, the value to be removed. Returns the removed element, or -1 if the element does not exist or the HashSet is empty.
Query Operations:
Query-1 (1): Inserts an element into the HashSet.
Query-2 (2): Returns a boolean indicating whether the element is present.
Query-3 (3): Removes the element from the HashSet.
Input:
The first line contains an integer ‘Q’, the number of queries.
Each of the next ‘Q’ lines contains two integers, representing the type of operation and the value.
Output:
For Query-1: Do not return anything.
For Query-2: Return true or false based on the presence of the element.
For Query-3: Return the element being removed, or -1 if not present.
Constraints:
- 1 <= Q <= 103
- 1 <= query type <= 3
- 0 <= VALUE <= 106
- Time limit: 1 second
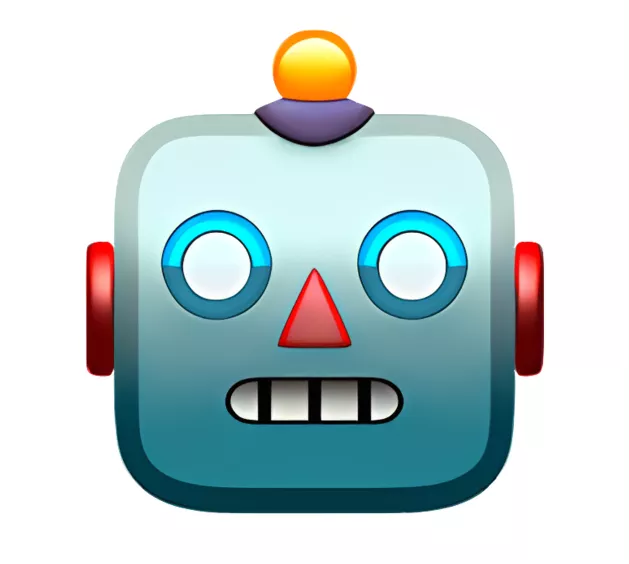
AnswerBot
4mo
Design a HashSet data structure with add, contains, and remove functions.
Implement a HashSet using an array and handling collisions using chaining or open addressing.
Use a hash function to map values ...read more
Help your peers!
Add answer anonymously...
Goldman Sachs Software Analyst interview questions & answers
A Software Analyst was asked Q. Describe how to implement two stacks using a single array.
A Software Analyst was asked Q. Design a stack that supports push, pop, top, and retrieving the minimum element ...read more
A Software Analyst was asked Q. What is the difference between SQL and NoSQL databases?
Popular interview questions of Software Analyst
A Software Analyst was asked Q1. Describe how to implement two stacks using a single array.
A Software Analyst was asked Q2. Design a stack that supports push, pop, top, and retrieving the minimum element ...read more
A Software Analyst was asked Q3. What is the difference between SQL and NoSQL databases?
>
Goldman Sachs Software Analyst Interview Questions
Stay ahead in your career. Get AmbitionBox app
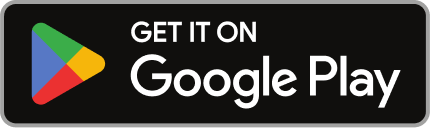
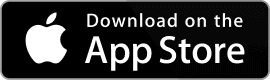
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
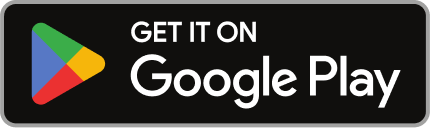
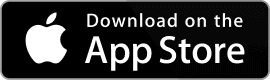