Bottom View of Binary Tree
Given a binary tree, determine and return its bottom view when viewed from left to right. Assume that each child of a node is positioned at a 45-degree angle from its parent.
Nodes in the bottom view are those that are visible when the tree is viewed from the bottom. For each horizontal distance from the root, the node that is visible in the bottom view is the one that is deepest (or the bottom-most node).
Example:
Input:
1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1
Output:
4 2 6 3 7
Explanation:
Given the tree:
1
2 3
4 -1 5 6
-1 7 -1 -1 -1 -1
-1 -1
The bottom-most node at various horizontal distances are:
- -2: 4
- -1: 2
- 0: 6
- 1: 3
- 2: 7
Therefore, the bottom view is 4 2 6 3 7
.
Constraints:
1 ≤ T ≤ 100
1 ≤ N ≤ 3000
-10^9 ≤ DATA ≤ 10^9
- Time Limit: 1 sec
Note:
- Horizontal distance of a node 'n' is calculated relative to the root. The root has a horizontal distance of 0. For any node, the horizontal distance is:
-parent's distance + 1
if it is a right child.
-parent's distance - 1
if it is a left child. - If two nodes share the same horizontal distance but are at different depths, select the deeper node.
- No need for explicit print statements, just return the correct sequence of nodes from left to right in the bottom view.
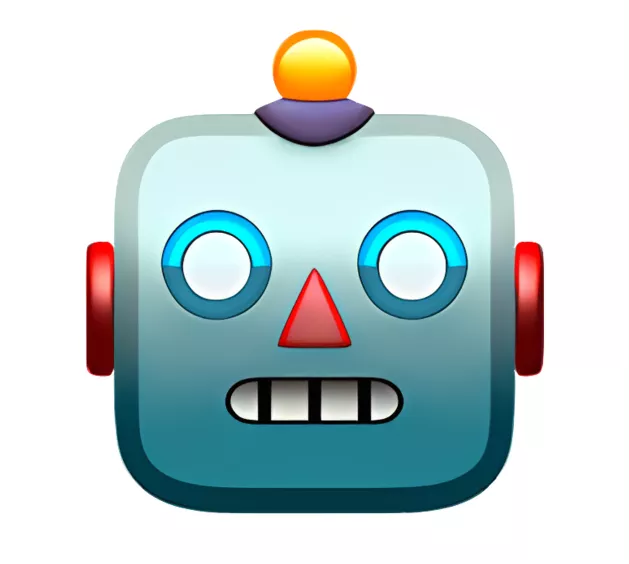
Given a binary tree, determine and return its bottom view when viewed from left to right.
Traverse the binary tree level by level and keep track of the horizontal distance and depth of each node
For eac...read more
Cognizant Software Analyst interview questions & answers
Popular interview questions of Software Analyst
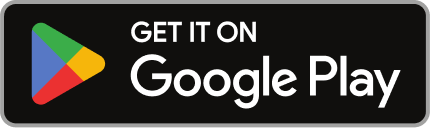
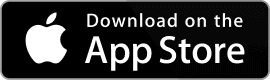
Reviews
Interviews
Salaries
Users
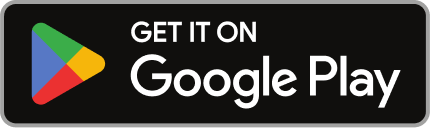
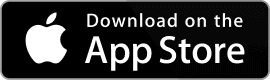