You are given a string. What is the minimum number of characters that need to be inserted to convert it into a palindrome?
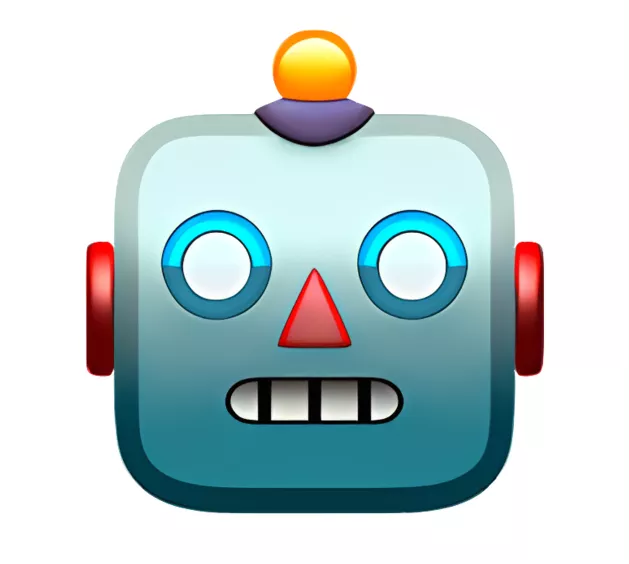
AnswerBot
4mo
The minimum number of characters needed to convert a string into a palindrome is the length of the string minus the length of the longest palindromic subsequence of the string.
Find the longest palindr...read more
Help your peers!
Add answer anonymously...
Bharti Airtel Software Engineer interview questions & answers
A Software Engineer was asked Q. Can you explain how JavaScript prototype sorting functions work?
A Software Engineer was asked Q. Why is React faster than other frameworks?
A Software Engineer was asked Q. Implement a Promise polyfill from scratch.
Popular interview questions of Software Engineer
A Software Engineer was asked Q1. Can you explain how JavaScript prototype sorting functions work?
A Software Engineer was asked Q2. Why is React faster than other frameworks?
A Software Engineer was asked Q3. Implement a Promise polyfill from scratch.
>
Bharti Airtel Software Engineer Interview Questions
Stay ahead in your career. Get AmbitionBox app
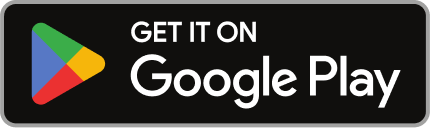
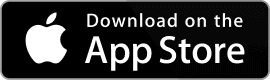
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
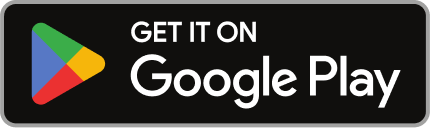
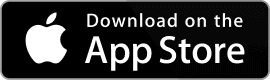