Random Point Generator in a Circle
Mr. Schrodinger needs to generate points that are uniformly distributed inside a specific circle for testing his hypothesis. Your task is to implement a function that generates random points evenly distributed within the given circle.
Example:
Input:
The first line contains an integer 'T' which indicates the number of test cases.
Each test case consists of one line containing three space-separated integers 'X', 'Y', 'R' denoting the coordinates of the circle's center and its radius.
Output:
For each test case, return 1 if the points are correctly uniformly distributed, otherwise 0.
Constraints:
1 ≤ T ≤ 5
-10^9 ≤ X, Y ≤ 10^9
1 ≤ R ≤ 10^9
Note: A point on the circle's circumference is considered inside the circle. You do not need to handle input or output; those aspects are managed for you. Focus on implementing the function.
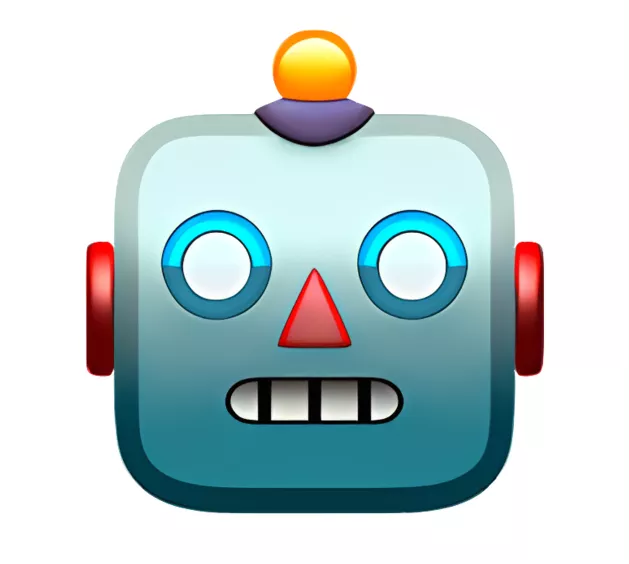
AnswerBot
4mo
Implement a function to generate random points uniformly distributed inside a circle.
Generate random points using polar coordinates
Ensure points fall within the circle by checking if distance from cen...read more
Help your peers!
Add answer anonymously...
>
Trilogy Innovations Software Developer Intern Interview Questions
Stay ahead in your career. Get AmbitionBox app
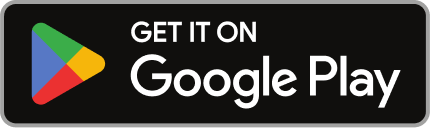
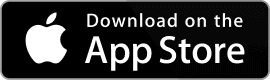
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
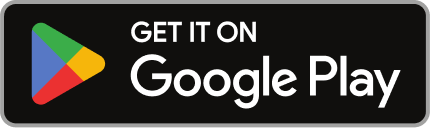
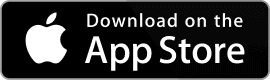