Closest Pair of Points Problem Statement
Given an array containing 'N' points in a plane, your task is to find the distance between the closest pair of points.
Explanation:
The distance between two points, (x1, y1) and (x2, y2), is calculated using the formula: [(x1 - x2) ^ 2] + [(y1 - y2) ^ 2].
Input:
N
x1 y1
x2 y2
...
xN yN
Output:
The smallest distance between the closest pair of points among the given 'N' points.
Example:
Input:
4
0 0
1 1
-1 -1
2 2
Output:
2
Constraints:
- 2 ≤ 'N' ≤ 105
- -105 ≤ 'x' ≤ 105
- -105 ≤ 'y' ≤ 105
- Time Limit: 1 second
Note:
You do not need to print anything; it has already been taken care of. Simply implement the provided function.
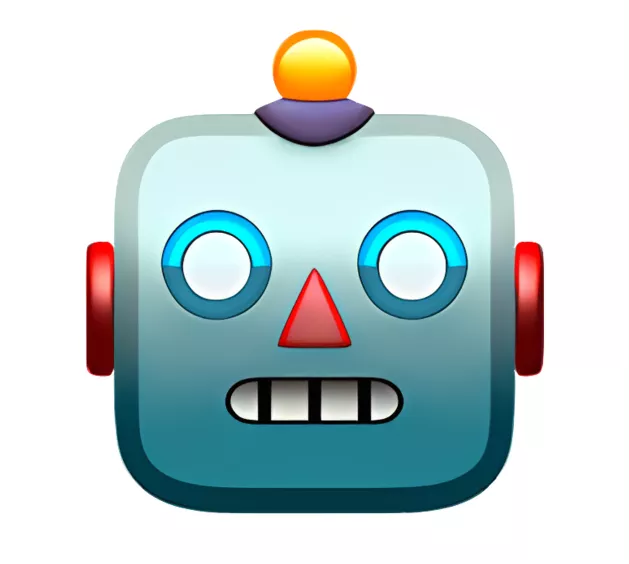
AnswerBot
4mo
Find the distance between the closest pair of points in a plane given an array of points.
Calculate the distance between each pair of points using the formula provided.
Keep track of the minimum distanc...read more
Help your peers!
Add answer anonymously...
Snapdeal Software Developer interview questions & answers
A Software Developer was asked Q. Given two linked lists that merge at a point, find the merging point.
A Software Developer was asked Q. Given an M x N matrix, how many rectangles are there?
A Software Developer was asked Q. Find a four-digit number in the form aabb that is a perfect square.
Popular interview questions of Software Developer
A Software Developer was asked Q1. Given two linked lists that merge at a point, find the merging point.
A Software Developer was asked Q2. Given an M x N matrix, how many rectangles are there?
A Software Developer was asked Q3. Find a four-digit number in the form aabb that is a perfect square.
Stay ahead in your career. Get AmbitionBox app
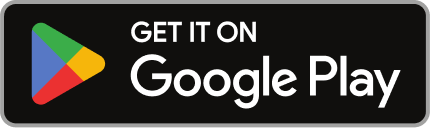
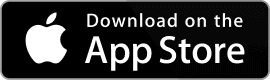
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
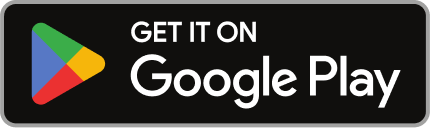
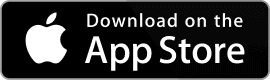