Ninja Theater Seating Arrangement Problem
As the technical lead of the renowned ninja theater, your task is to implement a system that ensures social distancing by maximizing the distance between seated individuals. This involves determining the best seating arrangement for incoming theater-goers.
Input:
The input begins with an integer 'T' representing the number of test cases.
For each test case:
- The first line contains a single integer ‘N’, indicating the number of seats arranged in a row in the theater.
- The following line contains a single integer, ‘M’, which represents the number of queries.
- Each of the next ‘M’ lines consists of two space-separated integers, ‘type’ and ‘seatNum’.
- For queries of the first type, ‘seatNum’ will be -1.
- For queries of the second type, it corresponds to the seat number of the departing person.
Output:
For each test case, output the results of queries of the first type. Return all the seat numbers a person should choose to maintain maximum distance from the nearest individual, separated by spaces. Each test case's result is on a new line.
Example:
Input:
1
5
3
1 -1
1 -1
2 0
Output:
0 2
Constraints:
- 1 ≤ T ≤ 5
- 1 ≤ N ≤ 109
- 1 ≤ M ≤ 100
- 1 ≤ type ≤ 2
- 0 ≤ seatNum ≤ N-1
Note:
No need to print; simply implement the function as specified. Ensure the seating maximizes the distance from the nearest seated person.
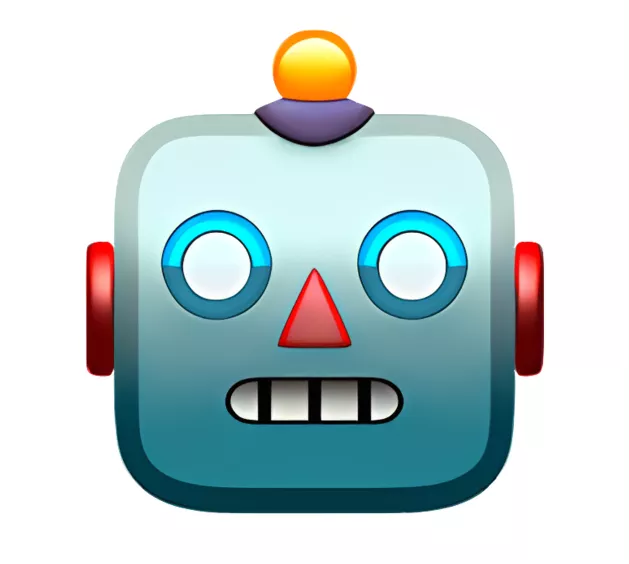
AnswerBot
4mo
Implement a system to maximize social distancing in a theater seating arrangement by determining the best seating arrangement for incoming theater-goers.
Create a function that takes the number of seat...read more
Help your peers!
Add answer anonymously...
Sanchhaya Education Software Developer Intern interview questions & answers
A Software Developer Intern was asked Q. Langford Pairing Problem Statement Given a positive integer N, you are required ...read more
A Software Developer Intern was asked Q. Ninja Theater Seating Arrangement Problem As the technical lead of the renowned ...read more
A Software Developer Intern was asked Q. Bridge in Graph Problem Statement Given an undirected graph with V vertices and ...read more
Popular interview questions of Software Developer Intern
A Software Developer Intern was asked Q1. Langford Pairing Problem Statement Given a positive integer N, you are required ...read more
A Software Developer Intern was asked Q2. Ninja Theater Seating Arrangement Problem As the technical lead of the renowned ...read more
A Software Developer Intern was asked Q3. Bridge in Graph Problem Statement Given an undirected graph with V vertices and ...read more
>
Sanchhaya Education Software Developer Intern Interview Questions
Stay ahead in your career. Get AmbitionBox app
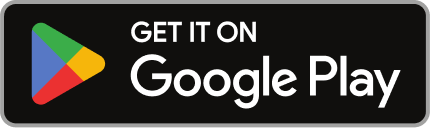
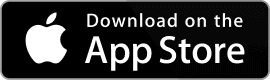
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
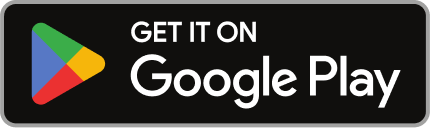
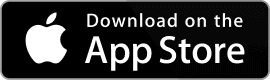