Longest Path In Directed Graph Problem Statement
Given a Weighted Directed Acyclic Graph (DAG) comprising 'N' nodes and 'E' directed edges, where nodes are numbered from 0 to N-1, and a source node 'Src'. Your task is to find the longest distances from the source node 'Src' to all the nodes in the graph.
Return an array of 'N' integers where the ith integer represents the longest distance from the node 'i' to the source node 'Src'. If a node is not reachable from the source node, return -1 for that node.
Input:
The first line of input contains an integer 'T', the number of test cases. Each test case begins with a line containing three space-separated integers 'N', 'E', and 'Src': the number of nodes, the number of edges, and the source node, respectively. The following 'E' lines each contain three space-separated integers 'U', 'V', 'W', indicating a directed edge from node U to node V with weight W.
Output:
For each test case, print 'N' space-separated integers, where the ith integer gives the maximum distance of node 'i' from the source node 'Src'. Print the result for each test case on a new line.
Example:
Input:
N = 5, E = 7, Src = 0
Output:
0 3 10 15 54
Explanation:
The maximum distance computations are as follows:
- Distance of node 0 from node 0 is 0 (self-loop).
- Distance of node 1 from node 0 is 3, via path 0 -> 1.
- Distance of node 2 from node 0 is 10, via path 0 -> 2.
- Distance of node 3 from node 0 is 15, via path 0 -> 2 -> 3.
- Distance of node 4 from node 0 is 54, via path 0 -> 1 -> 4.
Constraints:
- 1 <= T <= 50
- 1 <= N <= 104
- 0 <= E <= 104
- 0 <= Src <= N-1
- 0 <= U, V <= N-1
- 1 <= W <= 105
Note:
You do not need to print anything; focus on implementing the function to get the required results.
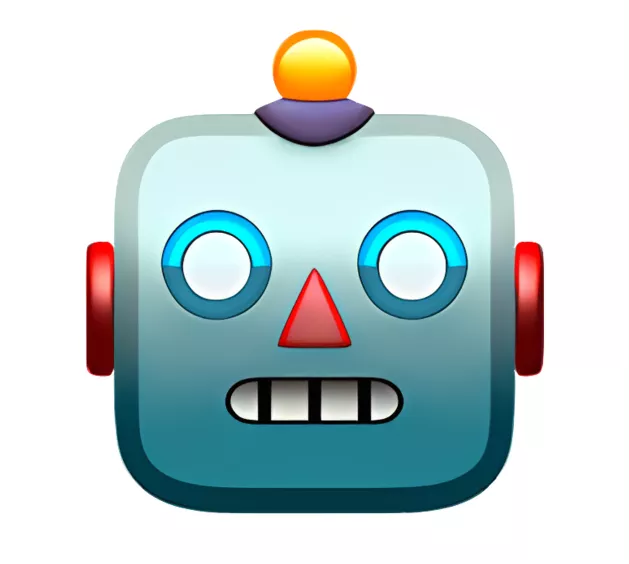
The task is to find the longest distances from a source node to all nodes in a weighted directed acyclic graph.
Implement a function that takes the number of nodes, edges, source node, and edge weights...read more
Salesforce Associate Software Engineer interview questions & answers
Popular interview questions of Associate Software Engineer
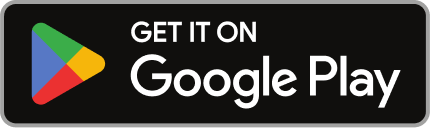
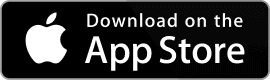
Reviews
Interviews
Salaries
Users
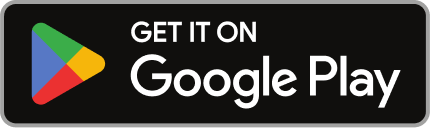
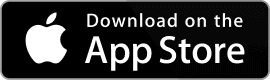