Quick Sort Implementation
Sort a given array of integers in ascending order using the Quick Sort algorithm.
Quick Sort is a divide and conquer algorithm that involves selecting a pivot element and partitioning the array into two sub-arrays: one containing elements less than the pivot, and the other containing elements greater than the pivot. The sub-arrays are then sorted recursively.
Example:
Input:
array = [4, 2, 1, 5, 3]
Output:
[1, 2, 3, 4, 5]
Explanation:
After the first level of partitioning with 3 as pivot, the array becomes [2, 1, 3, 4, 5]. After the second level with 1 as pivot on the left and 5 on the right, the sorted array is [1, 2, 3, 4, 5].
Constraints:
- 1 ≤ T ≤ 10
- 1 ≤ N ≤ 103
- -109 ≤ ARR[i] ≤ 109
- The input contains T test cases, where each test case consists of an integer N and an array ARR with N elements.
- Time limit: 1 second.
Note: You do not need to print anything; just implement the function as required.
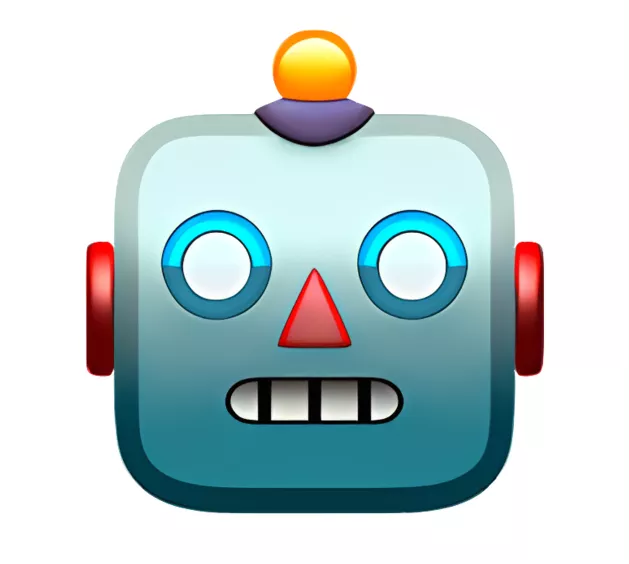
Implement Quick Sort to sort an array of integers in ascending order.
Choose a pivot element from the array
Partition the array into two parts: elements smaller than the pivot and elements larger than t...read more
Protium Finance Software Developer interview questions & answers
Popular interview questions of Software Developer
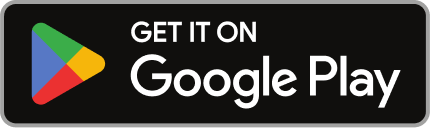
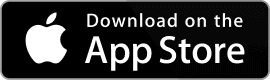
Reviews
Interviews
Salaries
Users
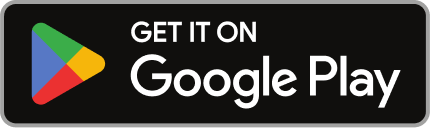
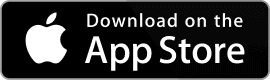