Implement Stack with Linked List
Your task is to implement a Stack data structure using a Singly Linked List.
Explanation:
Create a class named Stack
which supports the following operations, each in O(1) time:
getSize
: Returns the current size of the stack as an integer.isEmpty
: Returns a boolean indicating whether the stack is empty.push
: Accepts an integer and places it on top of the stack. Does not return anything.pop
: Removes the top element of the stack. Does not return anything and takes no action if the stack is empty.getTop
: Returns the top element of the stack as an integer or -1 if the stack is empty.
Input:
The first line of the input will contain the number of queries, T
.
The next T
lines will contain the queries, which can be of the following five types:
- '1': Print the current size of the stack.
- '2': Determine whether the stack is empty and print "true" if yes, "false" otherwise.
- '3 val': Push
val
(a non-negative integer) onto the top of the stack. No output should be printed. - '4': Remove the top element of the stack. No output should be printed.
- '5': Print the top element of the stack, or -1 if the stack is empty.
Output:
For each test case, print the result of each query on a separate line. If the query is '3' or '4', do not print anything.
Example:
Input:
5
3 10
5
1
2
4
Output:
10
1
false
Constraints:
- 1 <= T <= 10^6
- 1 <= Q <= 5
- 1 <= Data <= 2^31 - 1
Where Q
is the type of query and Data
represents the values being pushed onto and popped from the stack.
Time Limit: 1 second
Note:
You do not need to print anything as it has already been taken care of. Your task is to implement the function as specified.
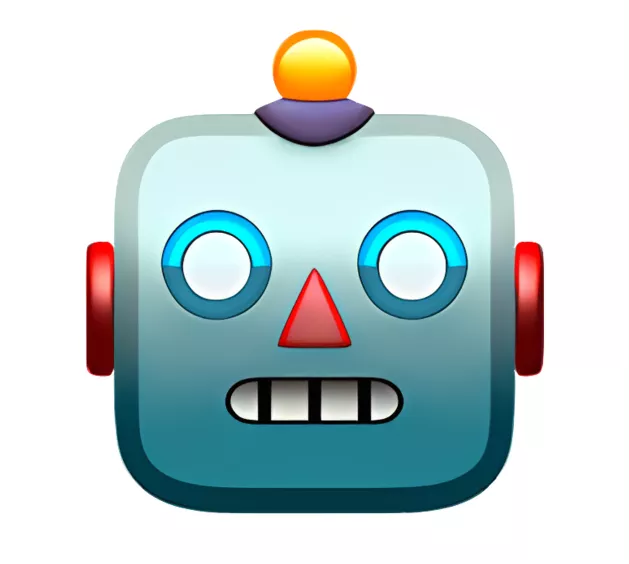
Implement a Stack data structure using a Singly Linked List with operations in O(1) time.
Create a class named Stack with getSize, isEmpty, push, pop, and getTop methods.
Use a Singly Linked List to sto...read more
OodlesTechnologies Software Developer interview questions & answers
Popular interview questions of Software Developer
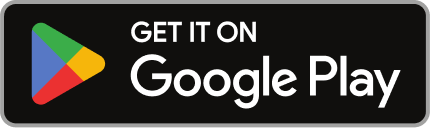
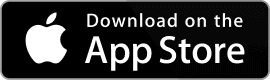
Reviews
Interviews
Salaries
Users
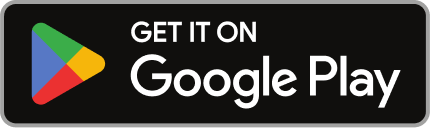
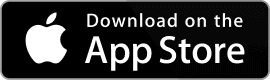